AWS Identity and Access Management (AWS IAM) plays a big role in AWS security because it empowers you to control access by creating users and groups, assigning specific permissions and policies to specific users, setting up multi-factor authentication for additional security, and more. This Boto3 IAM tutorial will cover using the Boto3 library (AWS SDK for Python) to manage AWS IAM service through the APIs.
If you’re new to the Boto3 library, check the Introduction to Boto3 library article.
Table of contents
What is AWS IAM?
AWS Identity and Access Management (IAM) is a web service that helps you manage security and control access to AWS resources. You can specify permissions to control which users or roles can access specific services and their actions under which conditions.
AWS IAM is a global service, so you don’t have to create different users or groups within each AWS region. AWS IAM covers all AWS regions.
IAM has everything you need to maintain access management to your AWS cloud resources, but it is your responsibility to configure it right. You should define how secure your access control procedures should be, how much you want to restrict users from accessing certain resources, how complex a password policy must be, or whether users should use multi-factor authentication, for example.
How to connect to AWS IAM using Boto3?
The Boto3 library provides you with two ways to access APIs for managing AWS IAM service:
- The
client
that allows you to access the low-level API data. For example, you can access API response data in JSON format. - The
resource
that allows you to use AWS services in a higher-level object-oriented way. For more information on the topic, look at AWS CLI vs. botocore vs. Boto3.
Here’s how you can instantiate the Boto3 IAM client to start working with Amazon EC2 APIs:
import boto3
iam_client = boto3.client("iam")
You can similarly instantiate the Boto3 EC2 resource:
import boto3
iam_resource = boto3.resource("iam")
Managing IAM policies using Boto3
To define a level of access to one or AWS service, you need to define an IAM policy. A policy is a JSON document that contains a set of rules defined in one or more statements. Each policy grants a specific set of permissions to AWS APIs, and it can be attached to one or more IAM identities (user, group, and role).
There are two types of policies:
- Managed policies can be created and attached to multiple entities. AWS has several built-in managed policies that cover various use cases. You can assign many managed policies to IAM users, roles, or groups. You can also create your own managed policies according to your needs.
- Inline policies are directly applied to IAM entities (users or roles) and don’t have distinctive ARNs. Those policies can not be reused.
AWS recommends using managed policies instead of inline policies to standardize policies management and make them reusable.
When working with IAM, you need to understand its terminology:
- Identities are IAM resources that define users, groups, and roles. You can attach a policy to an IAM identity.
- Entities are objects that AWS uses for authentication, for example, IAM users or roles.
- Principals are people or applications that use IAM users or roles in AWS accounts to make any actions or API calls.
IAM policy allows or denies actions to one or more principals on certain resources.
There are two main categories of IAM policies:
- Identity-based policies answer the question, “Which API calls can this identity make to which resources?”
- Resource-based policies define “Which identities can perform which actions with me?”
Here’s an example of an identity-based policy:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": "cognito-idp:AdminAddUserToGroup",
"Resource": "<resource-arn>"
}
]
}
And an example of resource-based policy:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"AWS": "<user-arn>"
},
"Action": "cognito-idp:AdminAddUserToGroup",
"Resource": "<resource-arn>"
}
]
}
How to create IAM policy?
To create an IAM policy, you must use the create_policy() method of the Boto3 IAM client.
The best way to define a policy is to use the Python dictionary. You can convert a defined policy to a string representation by using the json.dumps() method afterward:
import boto3
import json
client = boto3.client('iam')
policy_json = {
"Version": "2012-10-17",
"Statement": [
{
"Sid": "CognitoPostConfirmationAddToGroup",
"Effect": "Allow",
"Action": "cognito-idp:AdminAddUserToGroup",
"Resource": "arn:aws:cognito-idp:ap-northeast-1:585584209241:userpool/ap-northeast-1_p48aaPoti"
}
]
}
response = client.create_policy(
PolicyName='CognitoPostConfirmationAddToGroup',
PolicyDocument=json.dumps(policy_json)
)
print(response['Policy'])
The returned response
object will contain additional information about the created policy.
Here’s an execution output:
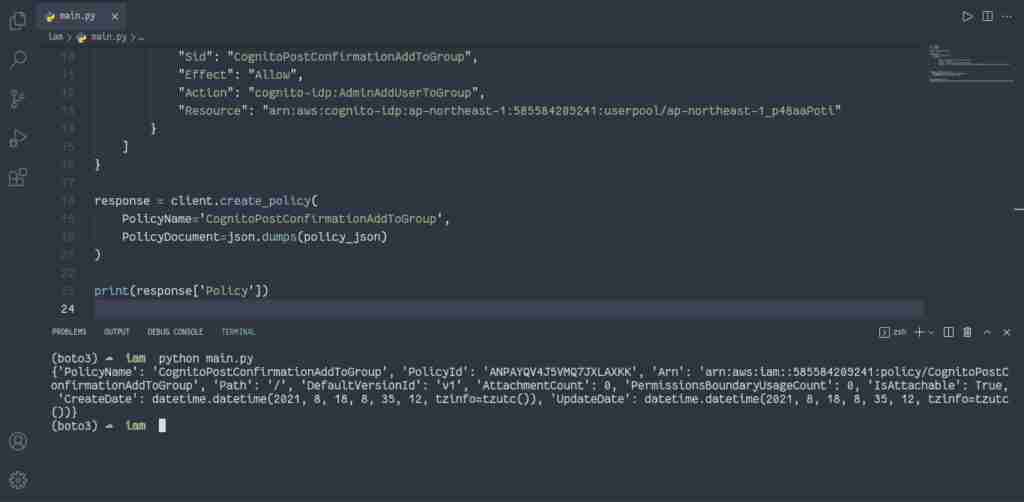
After execution of the create_policy()
method, you’ll see a created policy in the AWS console:

How to list IAM policies?
To get a list of all IAM policies available in your AWS account, you need to use the all() method of the policies collection of the IAM resource.
Note: Please remember that this method output might contain many policies in large AWS accounts.
import boto3
IAM_RESOURCE = boto3.resource('iam')
policies = IAM_RESOURCE.policies.all()
print('All account policies')
for policy in policies:
print(f' - {policy.policy_name}')
Here’s an execution output:
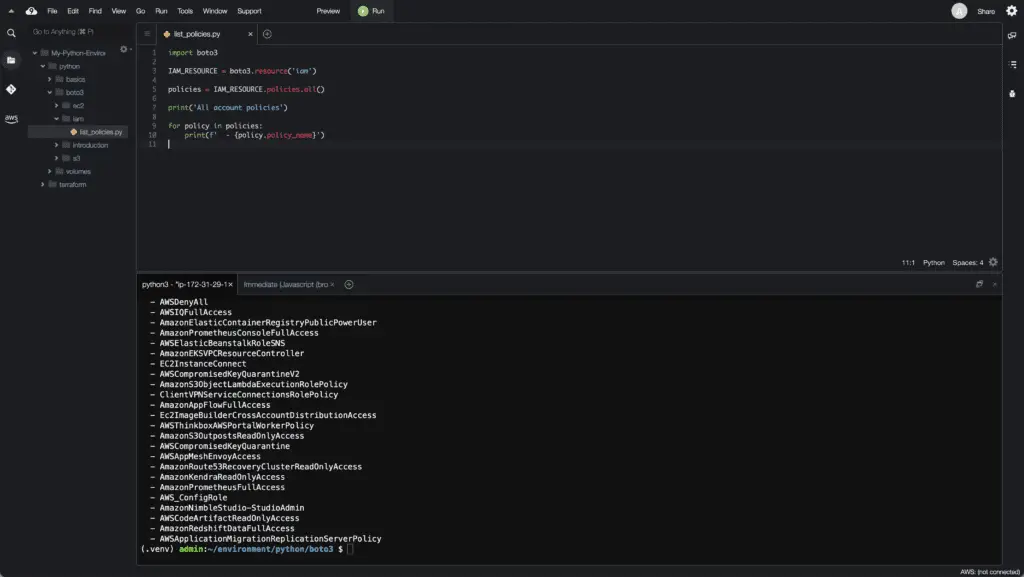
Alternatively, you can use the list_policies() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.list_policies(
Scope='All'
)
paginator = client.get_paginator("list_policies")
for page in paginator.paginate():
for policy in page['Policies']:
print(f' - {policy["PolicyName"]}')
In the example above, we need to use the get_paginator() method because the list_policies()
method limits the returned result to 100 policies by default.
You can provide any arguments supported by the list_policies()
method to the paginate()
method. We’ll cover this later in the filtering section.
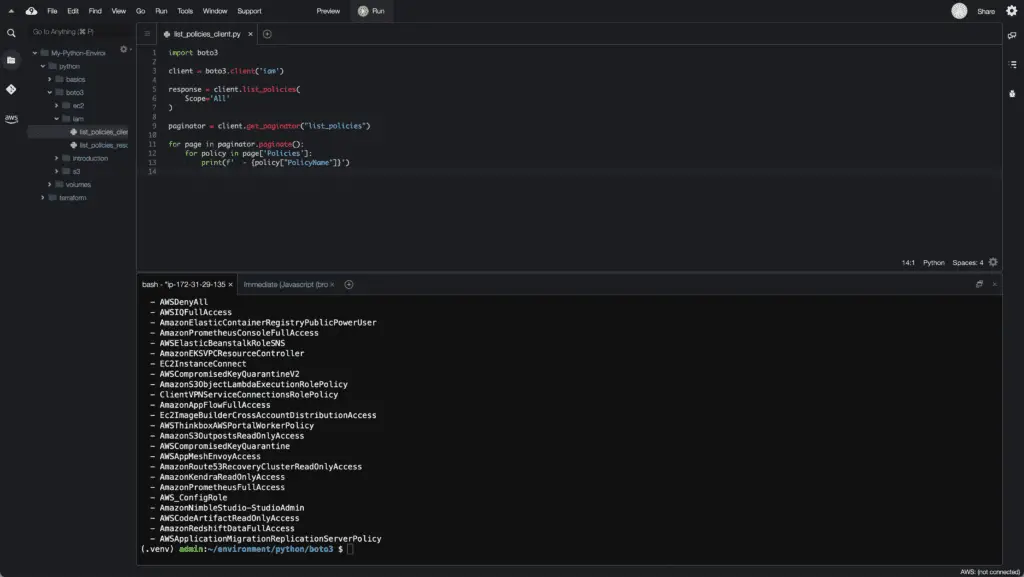
How to filter IAM policies?
To filter IAM policies available in your AWS account, you need to use the filter() method of the policies collection of the IAM resource. This method allows you to filter by the following criteria:
Scope
– policy scope (accepts valuesAll
,AWS
, andLocal
)OnlyAttached
– allows getting attached (True
) or detached (False
) policiesPathPrefix
– allows searching policies by the common path prefix- PolicyUsageFilter – specifies policy type (
PermissionsPolicy
orPermissionsBoundary
)
Let’s filter AWS permission policies with the common path prefix /service-role/
:
import boto3
IAM_RESOURCE = boto3.resource('iam')
policies = IAM_RESOURCE.policies.filter(
Scope='AWS',
PolicyUsageFilter='PermissionsPolicy',
PathPrefix='/service-role/'
)
print('Search results:')
for policy in policies:
print(f' - {policy.policy_name}')
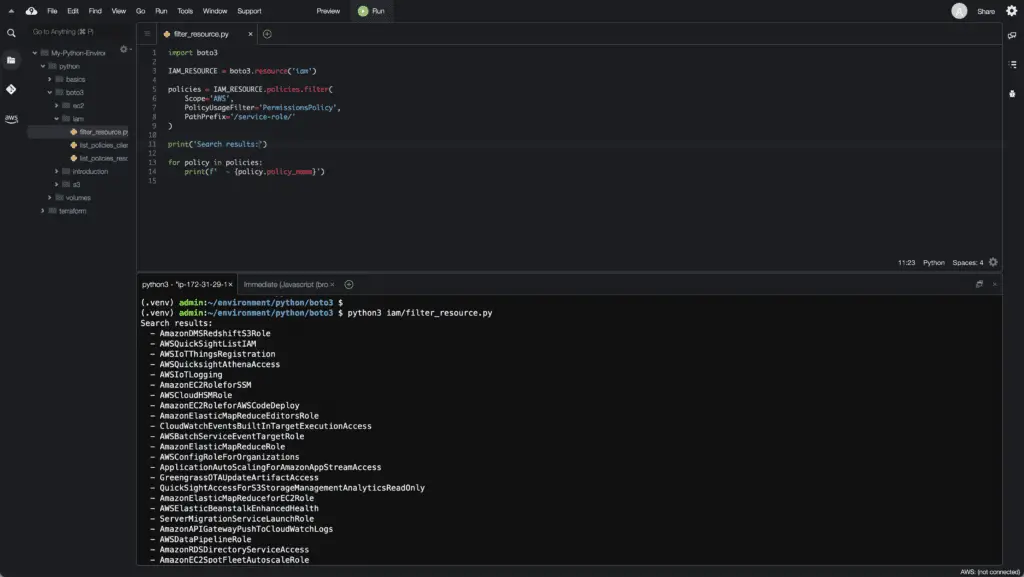
Alternatively, to filter IAM policies, you need to use the list_policies() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.list_policies(
Scope='AWS',
PolicyUsageFilter='PermissionsPolicy',
PathPrefix='/service-role/'
)
policies = response['Policies']
print([policy['PolicyName'] for policy in policies])
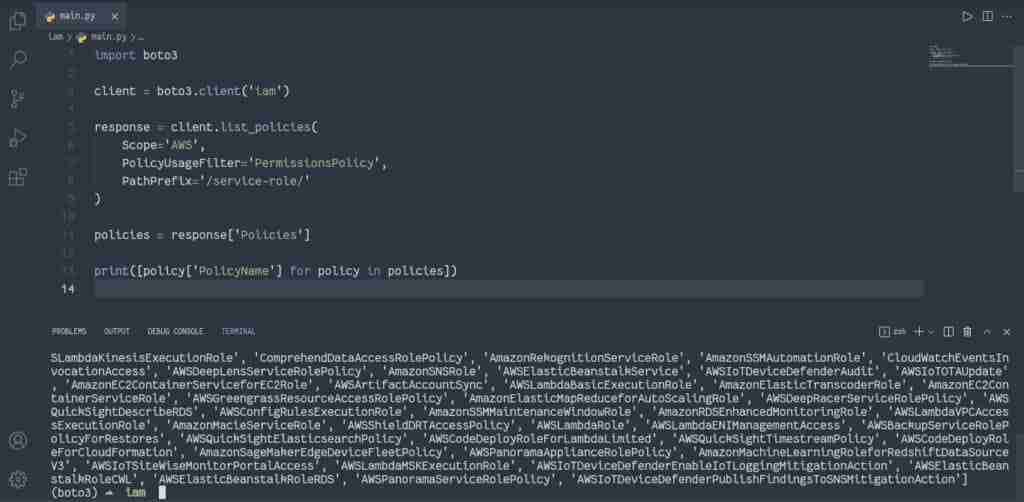
How to describe IAM policy?
To describe the IAM policy, you need to use the get_policy() method of the IAM client. This method returns the information about the specified managed policy in a Python dictionary, including the policy’s default version and the total number of IAM users, groups, and roles attached to that policy.
import boto3
client = boto3.client('iam')
response = client.get_policy(
PolicyArn='arn:aws:iam::585584209241:policy/CognitoPostConfirmationAddToGroup'
)
print(response['Policy'])

How to delete the IAM policy?
To delete an IAM policy, you must use the delete() method if the Policy class of the IAM resource.
Note: Before deleting a managed policy, you must detach the policy from all associated users, groups, or roles. In addition, you must delete all policy versions using the delete_policy_version() method.
import boto3
IAM_RESOURCE = boto3.resource('iam')
policy = IAM_RESOURCE.Policy(
'arn:aws:iam::585584209241:policy/demo-policy'
)
policy.delete()
print('Policy has been deleted')
Here’s an execution output:

Alternatively, to delete an IAM policy, you can use the delete_policy() method of the IAM client.
import boto3
client = boto3.client('iam')
response = client.delete_policy(
PolicyArn='arn:aws:iam::585584209241:policy/CognitoPostConfirmationAddToGroup'
)
print(response)
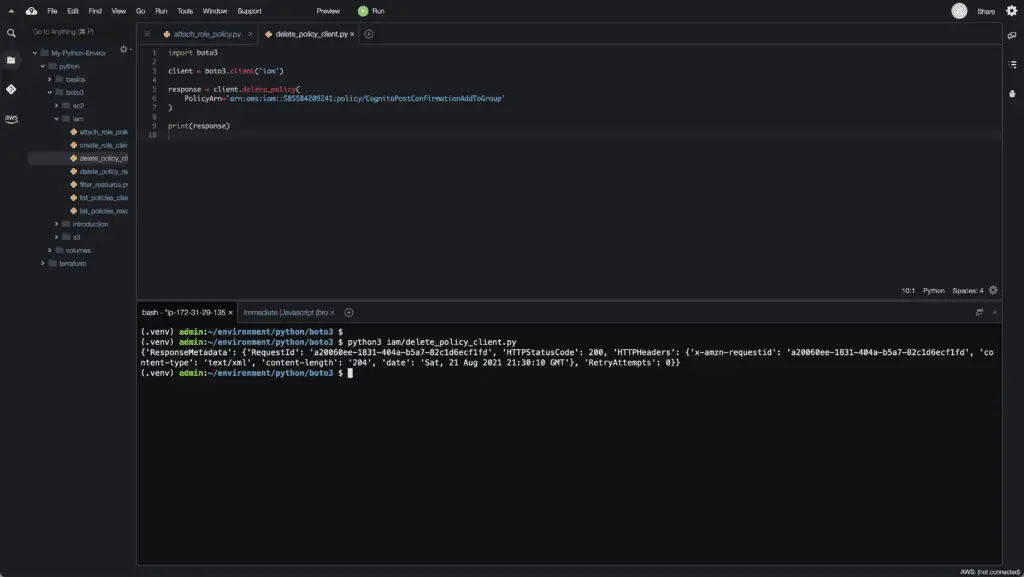
How to attach IAM policy to a role?
To attach an IAM policy to a role, you must use the attach_policy() method of the Role class of the IAM resource.
import boto3
ROLE_NAME = 'DEMO-Role'
resource = boto3.resource('iam')
role = resource.Role(ROLE_NAME)
role.attach_policy(
PolicyArn='arn:aws:iam::aws:policy/AmazonS3FullAccess'
)
print('Policy has been attached to the IAM role')
Here’s an execution output:
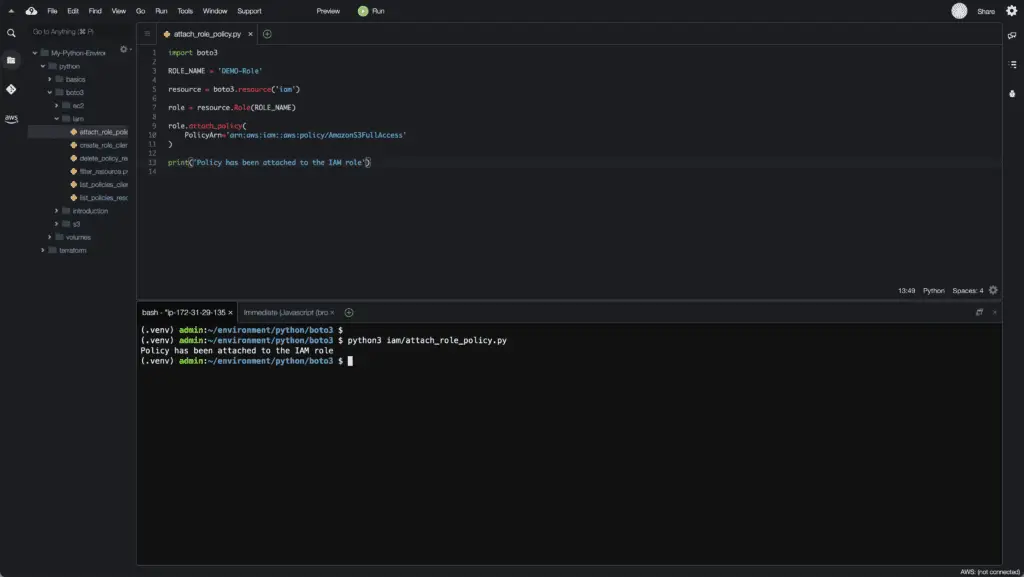
How to detach IAM policy from the role?
Todetach the IAM policy from the role, you need to use the detach_policy() method of the Role class of the IAM resource:
import boto3
ROLE_NAME = 'DEMO-Role'
resource = boto3.resource('iam')
role = resource.Role(ROLE_NAME)
role.detach_policy(
PolicyArn='arn:aws:iam::aws:policy/AmazonS3FullAccess'
)
print('Policy has been detached from the IAM role')

Managing IAM roles using Boto3
The IAM role contains a set of permissions an IAM entity can perform and what other IAM entities (users or roles) can assume that role. A role is not directly linked to a person or a service. Rather it can be assumed by any resource that the role grants permission to. Role credentials are always temporary and rotated periodically by the AWS Session Token Service(STS).
Instead of assigning permissions to an entity directly, roles allow an entity to be granted permissions temporarily (on an as-needed basis) to perform tasks. This enforces the least privilege principle, which is based on identity and time, as you can restrict entities to the minimum amount of access needed and the minimum amount of time needed to complete a task.
A role is both a principal and identity in AWS and primarily aims to grant temporary permissions to perform API calls in an account. To use a role, it has to be assumed.
Each role has trust relationships determining the entities that can assume the role. It also has a set of permissions that define which privileges entities get after they assume the role.
How to create IAM role?
To create an IAM role, you need to use the create_role() method of the IAM client. This method requires a policy that defines who can assume (use) this role.
For this example, let’s create a role that can be assumed by the TestUser
.
import boto3
import json
client = boto3.client('iam')
assume_role_policy = {
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::585584209241:user/TestUser"
},
"Action": "sts:AssumeRole"
}
]
}
response = client.create_role(
RoleName='TestRole',
AssumeRolePolicyDocument=json.dumps(assume_role_policy)
)
print(response)
Later, we can attach policies to the Role so that whenever TestUser
assumes TestRole
, the user can have the permissions from Role.

How to list IAM roles?
To list all IAM roles, you need to use the all()
method of the roles collection of the IAM resource.
import boto3
IAM_RESOURCE = boto3.resource('iam')
roles = IAM_RESOURCE.roles.all()
print('Account roles:')
for role in roles:
print(f' - {role.role_name}')
Here’s an execution output:
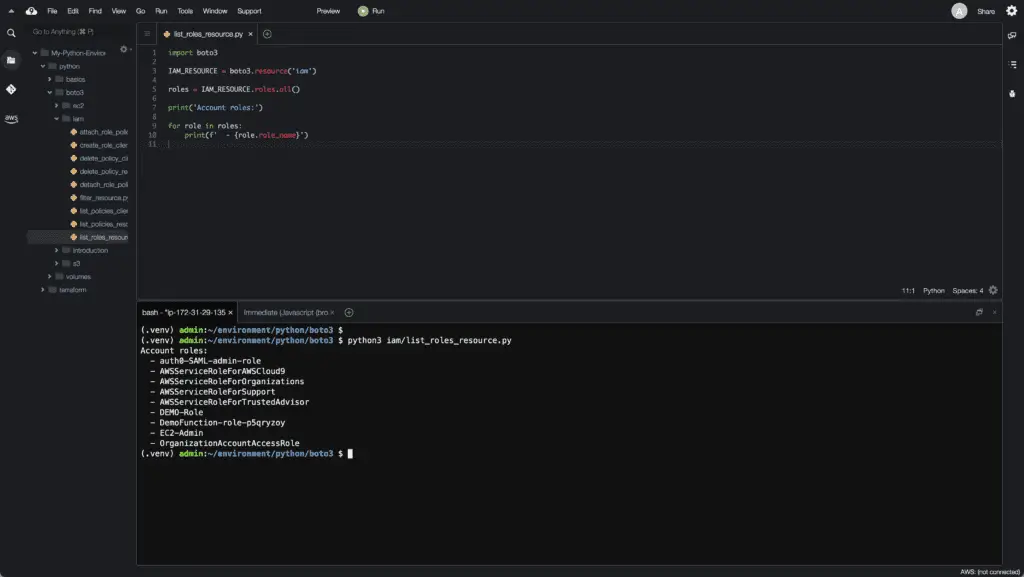
Alternatively, you can use thelist_roles() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.list_roles()
roles = response['Roles']
print([role['RoleName'] for role in roles])

How to filter IAM roles?
To filter IAM roles, you need to use the filter()
method of the roles collection of the IAM resource. This method accepts the PathPrefix
argument to filter the list of roles.
import boto3
IAM_RESOURCE = boto3.resource('iam')
policies = IAM_RESOURCE.roles.filter(
PathPrefix='/service-role/'
)
print('Search results:')
for policy in policies:
print(f' - {policy.role_name}')
Here’s an execution output:
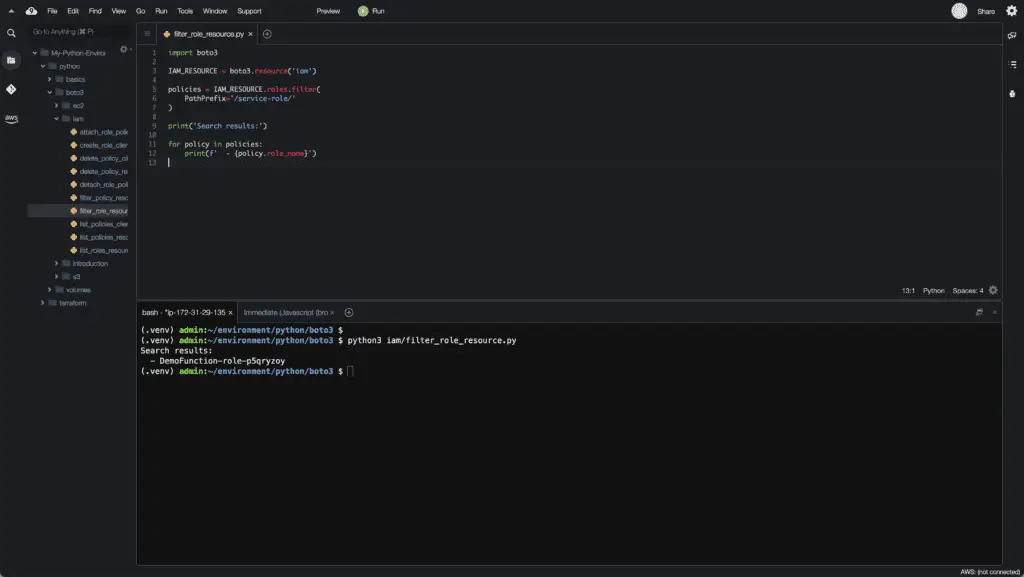
Alternatively, you can use thelist_roles() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.list_roles(
PathPrefix='/service-role/'
)
roles = response['Roles']
print([role['RoleName'] for role in roles])
Here’s an execution output:

How to describe IAM role?
To describe the IAM role, you can use the get_role() method of the IAM client. This method retrieves information about the specified role, including the role’s path, GUID, ARN, and the role’s trust policy granting permission to assume the role:
import boto3
client = boto3.client('iam')
response = client.get_role(
RoleName='TestRole'
)
print(response)
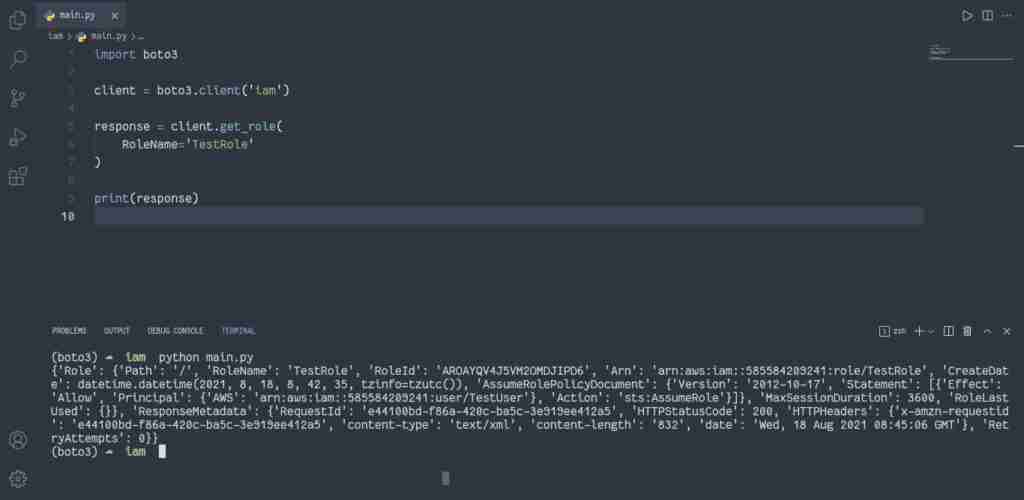
How to delete IAM role?
To delete the IAM role, you need to use the delete() method of the Role class of the IAM resource.
Note: Before deleting a role, you must detach all policies from the role.
import boto3
IAM_RESOURCE = boto3.resource('iam')
role = IAM_RESOURCE.Role(
'DEMO-Role'
)
role.delete()
print('IAM role has been deleted')
Here’s an execution output:

Alternatively, you can use the delete_role() method of the IAM client.
import boto3
client = boto3.client('iam')
response = client.delete_role(
RoleName='TestRole'
)
print(response)
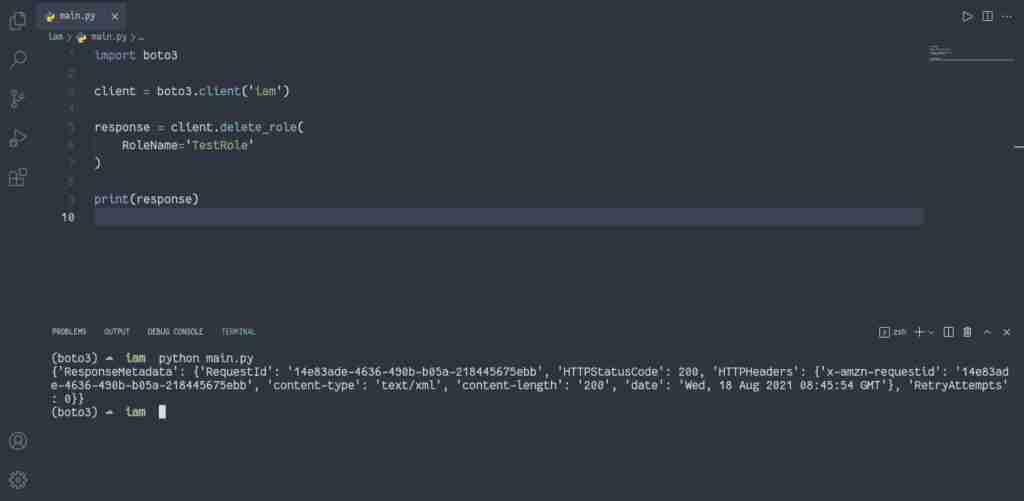
Managing IAM groups using Boto3
An IAM group is a collection of users and permissions assigned to those users. Groups provide a convenient way to manage users with similar needs by categorizing them to their requirements. Then, you can manage permissions for all those users simultaneously through the group.
How to create IAM group?
To create the IAM group, you need to use thecreate_group() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.create_group(
GroupName='TestGroup'
)
print(response)
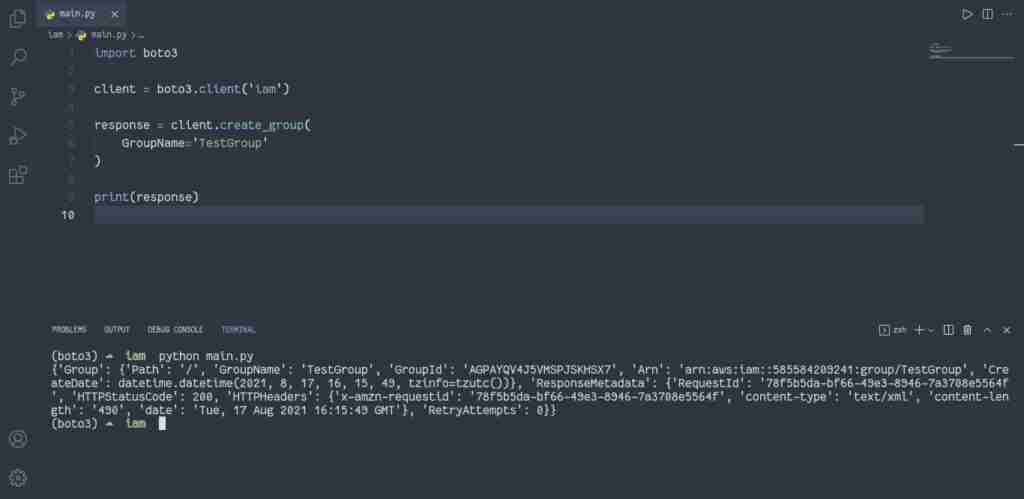
How to list IAM groups?
To list IAM groups, you need to use the all()
method of groups collection of the IAM resource:
import boto3
IAM_RESOURCE = boto3.resource('iam')
groups = IAM_RESOURCE.groups.all()
print('IAM groups in the account:')
for group in groups:
print(f' - {group.name}')
Here’s an execution output:
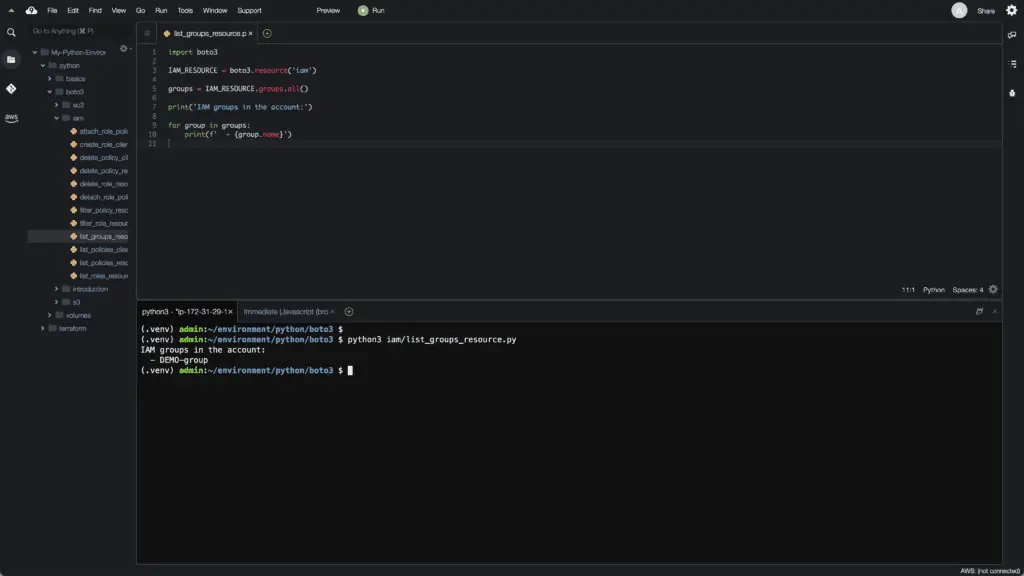
Alternatively, you can use thelist_groups() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.list_groups()
groups = response['Groups']
print([group['GroupName'] for group in groups])

How to filter IAM groups?
To filter IAM groups, you need to use the filter()
method of groups collection of the IAM resource.
Similar to the roles and policies, IAM groups support filtering by PathPrefix
.
import boto3
IAM_RESOURCE = boto3.resource('iam')
groups = IAM_RESOURCE.groups.filter(
PathPrefix='/'
)
print('IAM groups in the account:')
for group in groups:
print(f' - {group.name}')
Here’s an execution output:
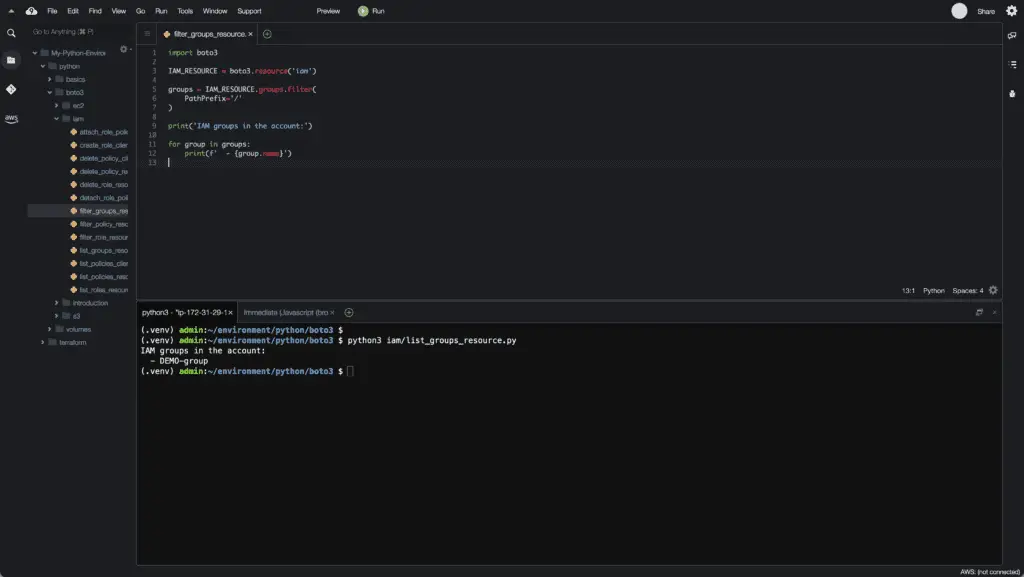
Alternatively, you can use thelist_groups() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.list_groups(
PathPrefix='/'
)
groups = response['Groups']
print([group['GroupName'] for group in groups])

How to describe IAM groups?
To describe IAM groups, you need to use theget_group() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.get_group(
GroupName='TestGroup'
)
print(response['Group'])

How to delete IAM group?
To delete the IAM group, you need to use the delete() method of the Group class of the IAM resource:
import boto3
IAM_RESOURCE = boto3.resource('iam')
group = IAM_RESOURCE.Group('DEMO-Group')
group.delete()
print('IAM group has been deleted')
Here’s an execution output:
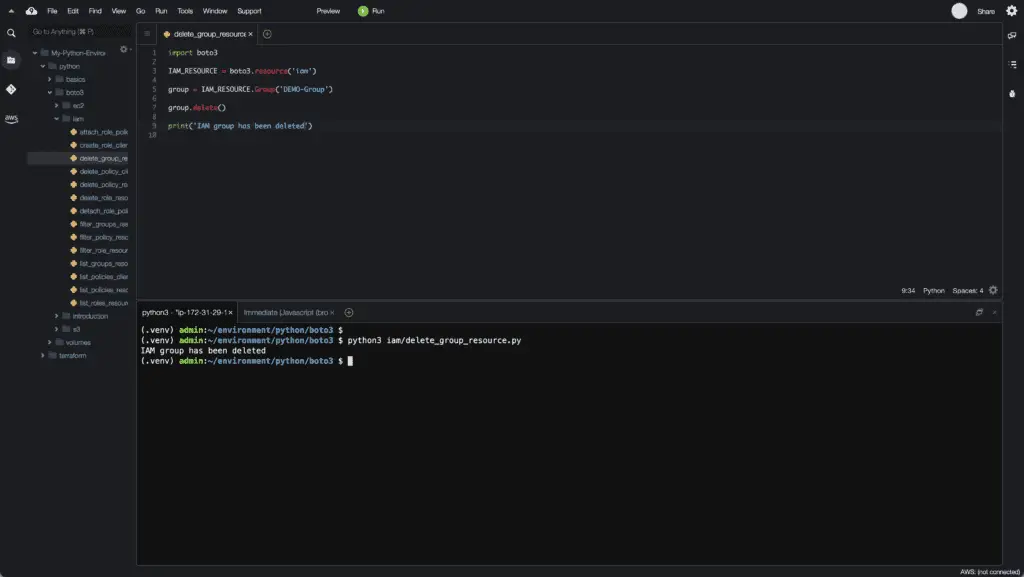
Alternatively, you can use thedelete_group() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.delete_group(
GroupName='TestGroup'
)
print(response)
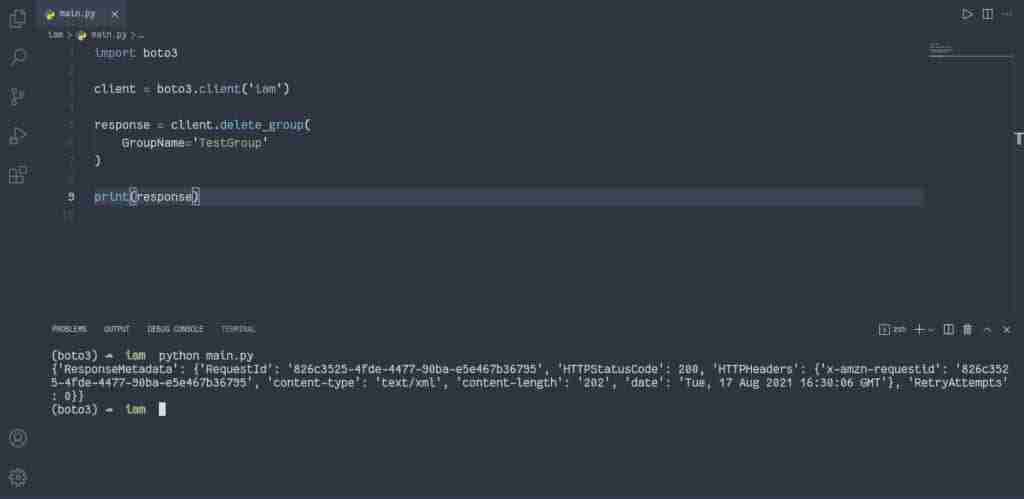
Managing IAM instance profiles using Boto3
By assigning Instance Profiles, you can provide your EC2 instances required permissions to access AWS resources without using API Access Keys. Instance Profile provides a mechanism to assign an IAM role to an instance.
How to create IAM instance profile?
To create an IAM instance profile, you need to use thecreate_instance_profile() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.create_instance_profile(
InstanceProfileName='TestInstanceProfile'
)
print(response['InstanceProfile'])
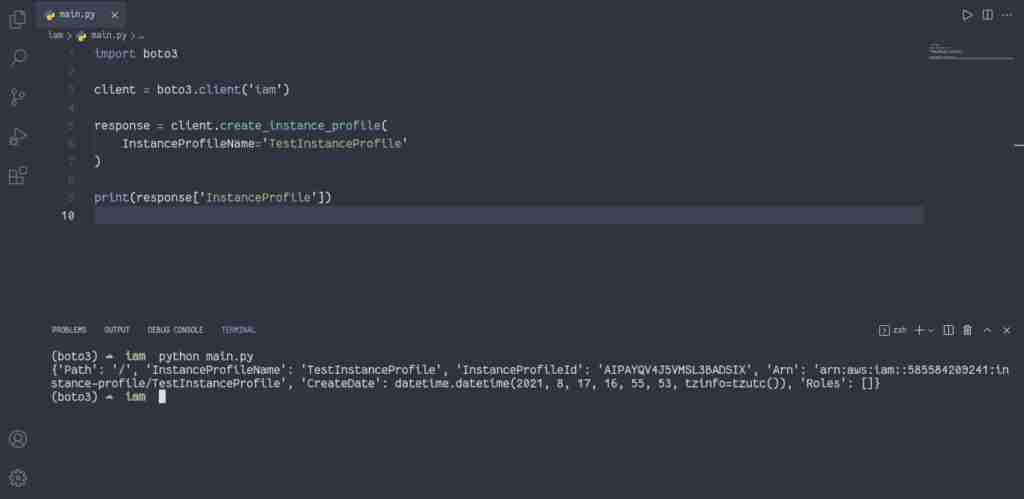
How to add role to IAM instance profile?
To add a role to the IAM Instance Profile, you need to use the add_role() method of the InstanceProfile class of the IAM resource:
import boto3
IAM_RESOURCE = boto3.resource('iam')
instance_profile = IAM_RESOURCE.InstanceProfile(
'TestInstanceProfile'
)
instance_profile.add_role(
RoleName='EC2-Admin'
)
print('IAM role has been added to the Instance Profile')
Here’s an execution output:

Alternatively, you can use the add_role_to_instance_profile() method of the IAM client to add the required IAM role to the instance profile.
import boto3
client = boto3.client('iam')
response = client.add_role_to_instance_profile(
InstanceProfileName='TestInstanceProfile',
RoleName='TestRole'
)
print(response)
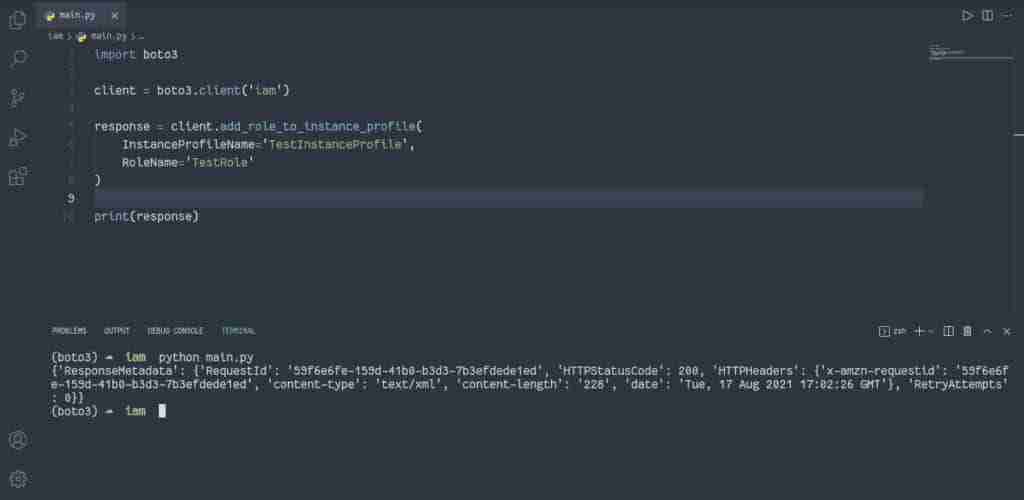
How to list IAM instance profiles?
To list IAM Instance Profiles, you need to use the all()
method of the instance_profiles collection of the IAM resource:
import boto3
IAM_RESOURCE = boto3.resource('iam')
instance_profiles = IAM_RESOURCE.instance_profiles.all()
print('IAM Instance Profiles:')
for instance_profile in instance_profiles:
print(f' - {instance_profile.name}')
Here’s an execution output:

Alternatively, you can use thelist_instance_profiles() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.list_instance_profiles()
instance_profiles = response['InstanceProfiles']
print([instance_profile['InstanceProfileName'] for instance_profile in instance_profiles])
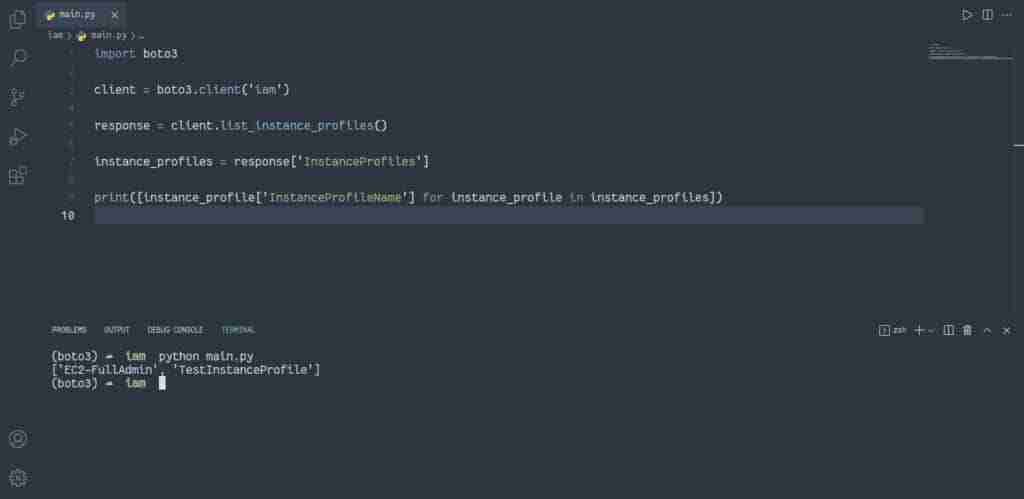
How to filter IAM instance profiles?
To filter IAM instance profiles, you need to use the filter()
method of instance_profiles collection of the IAM resource:
Similar to the roles, policies, and groups, IAM Instance Profiles support filtering by PathPrefix
.
import boto3
IAM_RESOURCE = boto3.resource('iam')
instance_profiles = IAM_RESOURCE.instance_profiles.filter(
PathPrefix='/'
)
print('IAM Instance Profile search results:')
for instance_profile in instance_profiles:
print(f' - {instance_profile.name}')
Here’s an execution output:
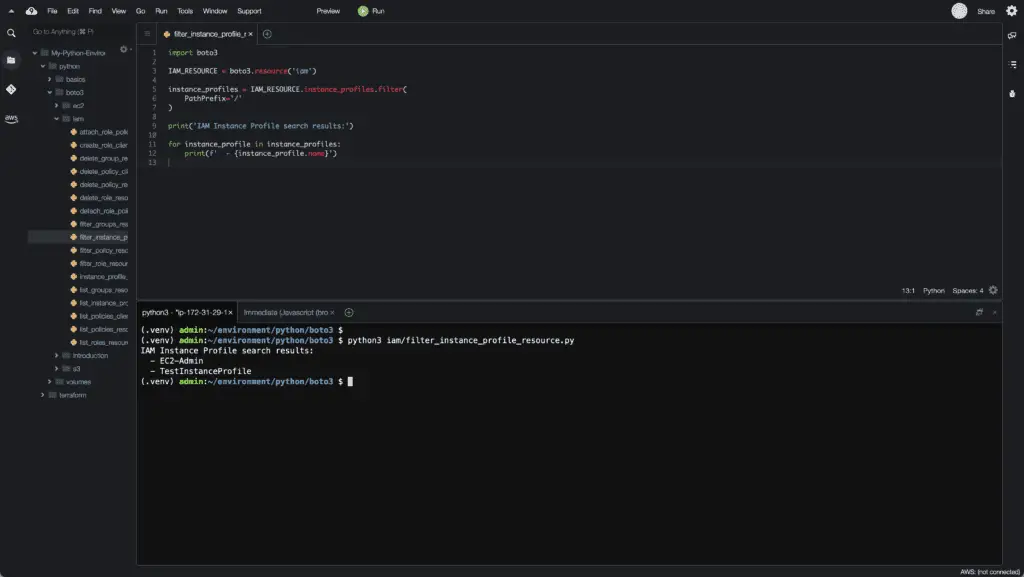
Alternatively, you can use thelist_instance_profiles() method of the IAM client to achieve the same result:
import boto3
client = boto3.client('iam')
response = client.list_instance_profiles(
PathPrefix='/'
)
instance_profiles = response['InstanceProfiles']
print([instance_profile['InstanceProfileName'] for instance_profile in instance_profiles])

How to describe IAM instance profile?
To describe the IAM instance profile, you need to use the get_instance_profile() method of the IAM client to retrieve the information about the specified instance profile, including the instance profile’s path, GUID, ARN, and role.
import boto3
client = boto3.client('iam')
response = client.get_instance_profile(
InstanceProfileName='TestInstanceProfile'
)
print(response)
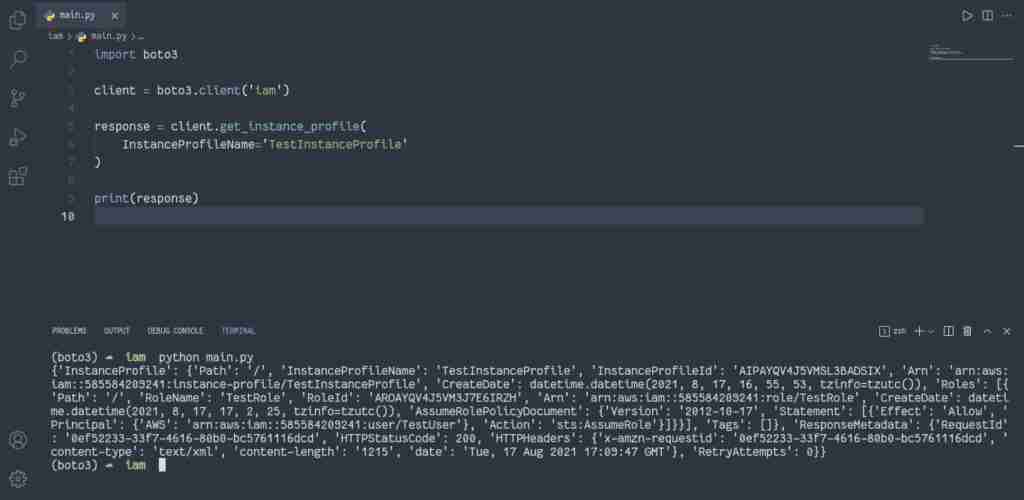
How to delete IAM instance profile?
To delete the IAM instance profile, you must use the delete() method of the InstanceProfile class of the IAM resource.
Notes:
- Before deleting the IAM Instance Profile, you must remove the role from the Instance Profile. Otherwise, you’ll get the following Python exception:
DeleteConflictException: An error occurred (DeleteConflict) when calling the DeleteInstanceProfile operation: Cannot delete entity, must remove roles from instance profile first.
- Ensure you do not have any Amazon EC2 instances running with the instance profile you are about to delete. Deleting a role or instance profile associated with a running instance will break any applications running on the instance.
import boto3
IAM_RESOURCE = boto3.resource('iam')
instance_profile = IAM_RESOURCE.InstanceProfile(
'TestInstanceProfile'
)
print('Detaching roles from Instance Profile...')
for attached_role in instance_profile.roles_attribute:
instance_profile.remove_role(
RoleName=attached_role['RoleName']
)
instance_profile.delete()
print('IAM Instance Profile has been deleted')
Here’s an execution output:
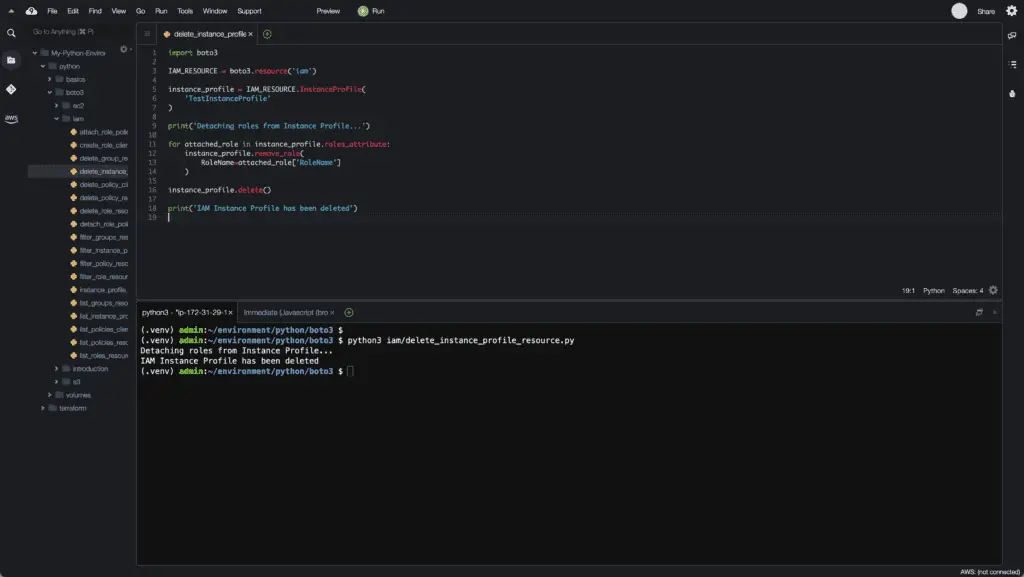
Alternatively, you can use theremove_role_from_instance_profile() anddelete_instance_profile() methods if the IAM clientto delete the Instance Profile:
import boto3
client = boto3.client('iam')
role_removed = client.remove_role_from_instance_profile(
InstanceProfileName='TestInstanceProfile',
RoleName='TestRole'
)
if role_removed:
response = client.delete_instance_profile(
InstanceProfileName='TestInstanceProfile'
)
print(response)
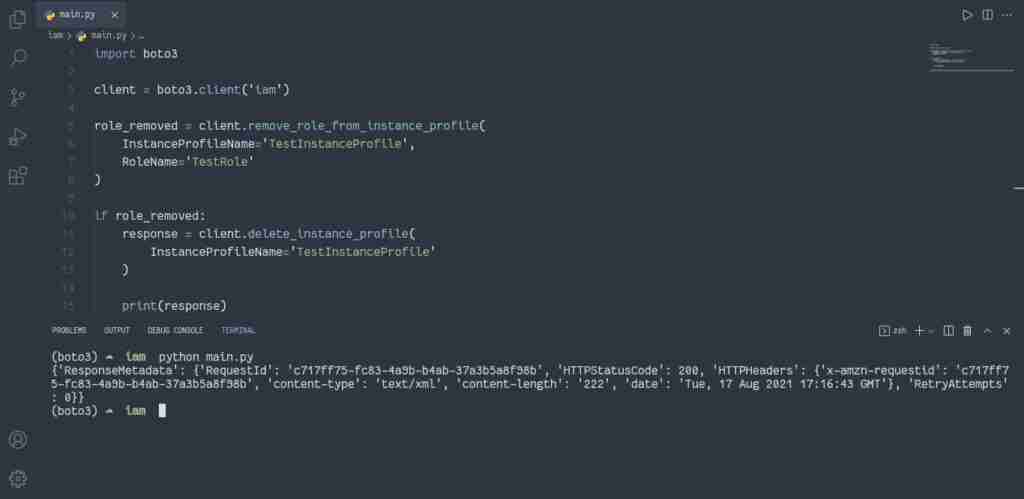
How to attach IAM instance profile to the EC2 instance?
To attach the IAM instance profile to the EC2 instance, you need to use the associate_iam_instance_profile() method of the EC2 client.
Note: You cannot associate more than one IAM instance profile with an EC2 instance.
import boto3
iam_client = boto3.client('iam')
ec2_client = boto3.client('ec2')
def get_instance_profile(instance_profile_name):
response = iam_client.get_instance_profile(
InstanceProfileName=instance_profile_name
)
return response['InstanceProfile']
instance_profile = get_instance_profile('TestInstanceProfile')
response = ec2_client.associate_iam_instance_profile(
IamInstanceProfile={
'Arn': instance_profile['Arn'],
'Name': instance_profile['InstanceProfileName']
},
InstanceId='i-0286635ef38b83dff'
)
print(response)

How to detach IAM instance profile from the EC2 instance?
To detach the IAM Instance Profile from the EC2 instance, you need to use the describe_iam_instance_profile_associations() method of the EC2 client. As soon as an EC2 instance can have only one association at a time, you can filter the EC2 instance by the instance ID and get the first association from theassociations’ list.
import boto3
client = boto3.client('ec2')
describe_response = client.describe_iam_instance_profile_associations(
Filters=[
{
'Name': 'instance-id',
'Values': ['i-0286635ef38b83dff']
}
]
)
associations = describe_response['IamInstanceProfileAssociations']
association_id = associations[0]['AssociationId']
response = client.disassociate_iam_instance_profile(
AssociationId=association_id
)
print(response)
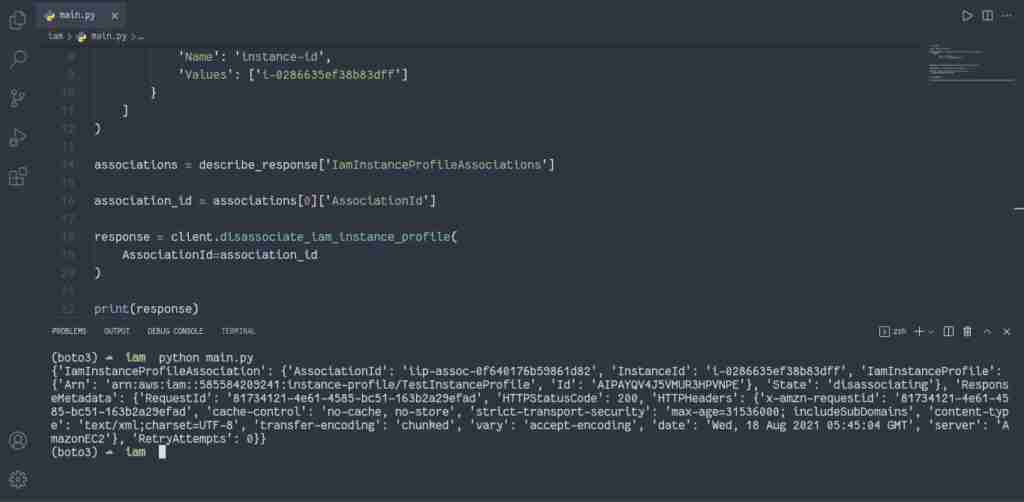
Managing IAM users using Boto3
An IAM user is an entity you create in AWS to represent the person or application that can interact with AWS services.
How to create IAM user?
To create an IAM user, you need to use thecreate_user() method of the IAM client:
import boto3
client = boto3.client('iam')
response = client.create_user(
UserName='IAMTestUser'
)
print(response)
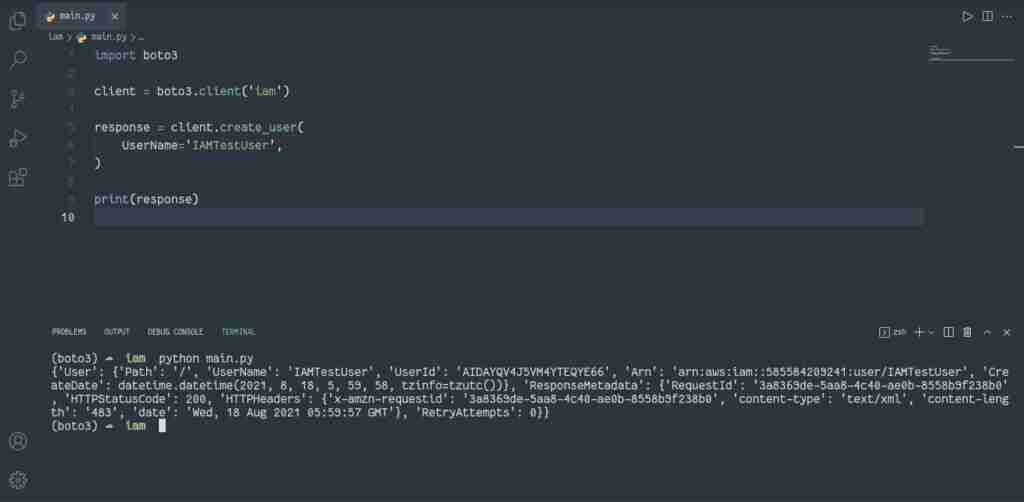
How to list IAM users?
To list all IAM users in the AWS account, you need to use the all()
method of users collection of the IAM resource:
import boto3
IAM_RESOURCE = boto3.resource('iam')
users = IAM_RESOURCE.users.all()
print('All account users')
for user in users:
print(f' - {user.name}')
Here’s an execution output:

Alternatively, you can use thelist_users() method of the IAM client to achieve the same result:
import boto3
client = boto3.client('iam')
response = client.list_users()
users = response['Users']
print('All account users')
for user in users:
print(f' - {user["UserName"]}')
Here’s an execution output:
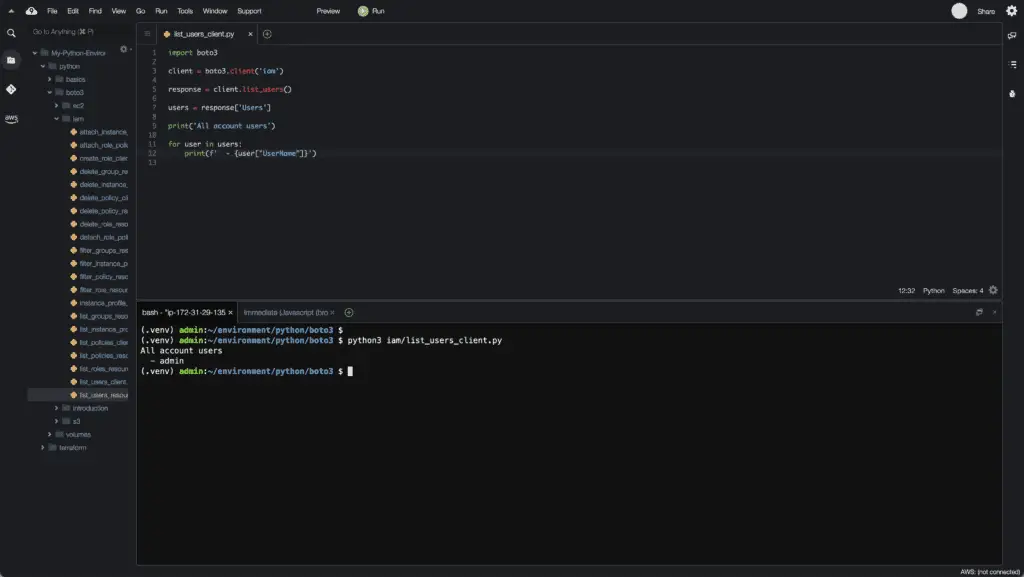
How to filter IAM users?
To filter IAM users, you need to use the filter()
method of the users collection of the IAM resource. This method allows you to filter users by their Path Prefix:
import boto3
IAM_RESOURCE = boto3.resource('iam')
users = IAM_RESOURCE.users.filter(
PathPrefix='/'
)
print('IAM users search results:')
for user in users:
print(f' - {user.name}')
Here’s an execution output:

Alternatively, you can use thelist_users() method of the IAM client to achieve the same result:
import boto3
client = boto3.client('iam')
response = client.list_users(
PathPrefix='/test/'
)
users = response['Users']
print([user['UserName'] for user in users])

How to describe IAM user?
To describe the IAM user, you need to use the get_user() method of the IAM client that retrieves information about the specified IAM user, including the user’s creation date, path, unique ID, and ARN.
If you do not specify a user name, IAM will return the user information of the currently authenticated user.
import boto3
client = boto3.client('iam')
response = client.get_user()
print(response)
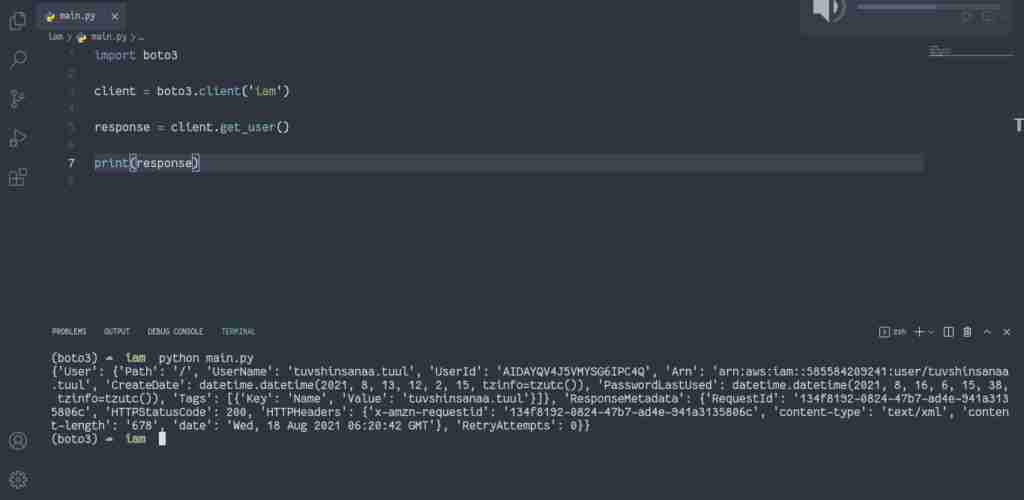
Here’s an example of describing an IAM user based on the user name:
import boto3
client = boto3.client('iam')
response = client.get_user(
UserName='IAMTestUser'
)
print(response)
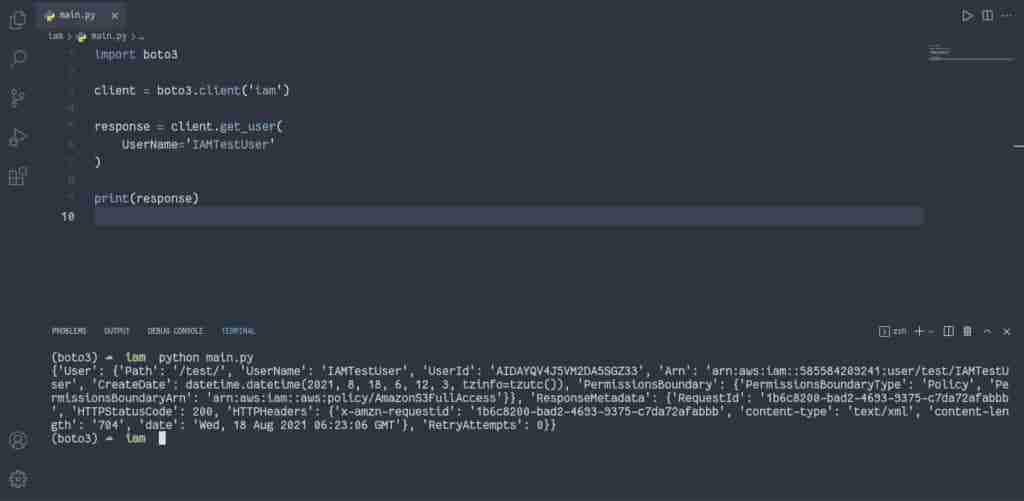
How to delete IAM user?
To delete an IAM user, you must use the delete() method of the User class of the IAM resource.
Before deleting an IAM user, you must remove the following items first:
- User Login Profile ( LoginProfile.delete() )
- Access keys ( AccessKey.delete() )
- Signing certificate ( SigningCertificate.delete() )
- SSH public key ( delete_ssh_public_key() )
- Git credentials ( delete_service_specific_credential() )
- Multi-factor authentication (MFA) device ( deactivate_mfa_device() , delete_virtual_mfa_device() )
- Inline policies ( delete_user_policy() )
- Attached managed policies ( User.detach_policy() )
- Group memberships ( User.remove_group() )
Here’s an example:
import boto3
IAM_RESOURCE = boto3.resource('iam')
user = IAM_RESOURCE.User(
'IAMTestUser'
)
user.delete()
print('IAM user has been deleted')
Here’s an execution output:
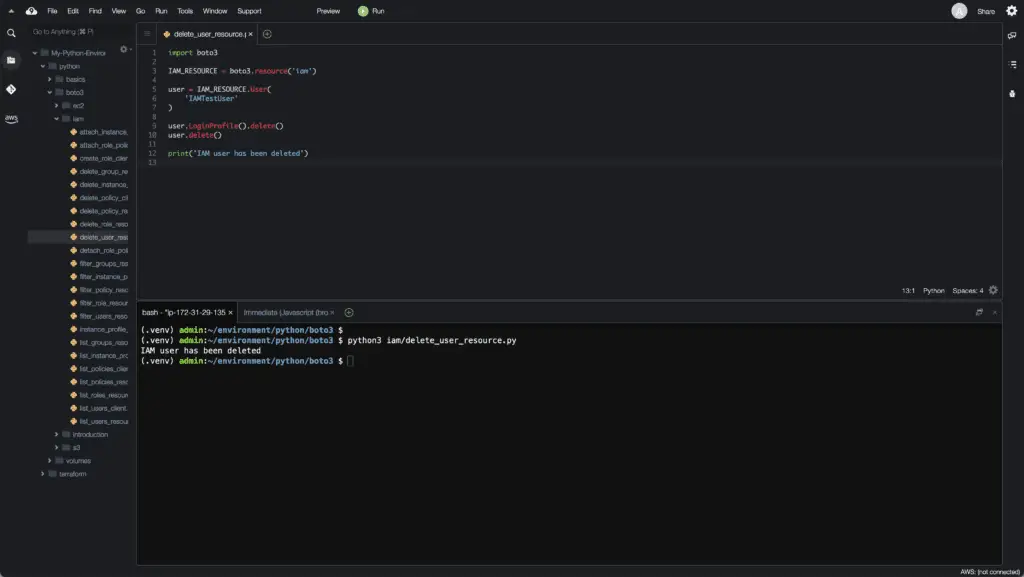
Alternatively, you can use the delete_user() method of the IAM client to delete the specified IAM user.
import boto3
client = boto3.client('iam')
response = client.delete_user(
UserName='IAMTestUser'
)
print(response)
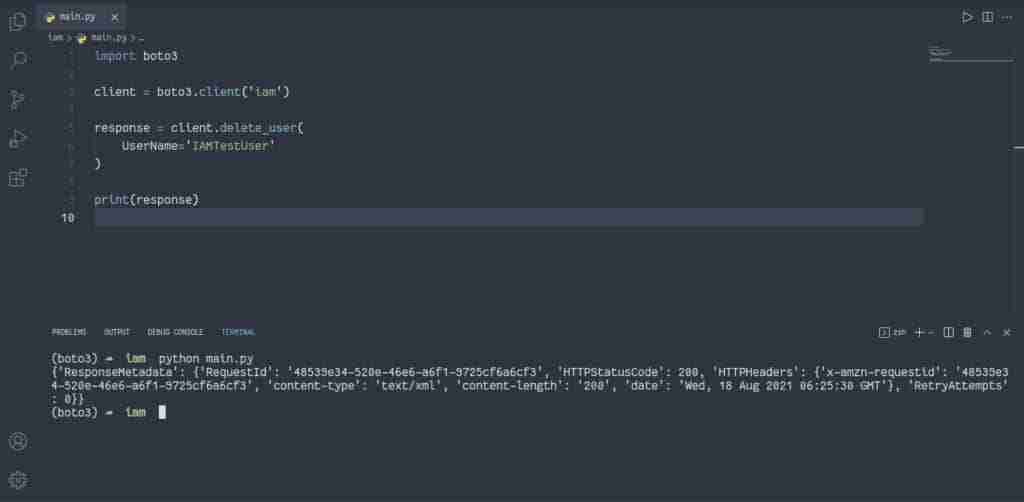
How to add IAM user to group?
To add the IAM user to a group, you need to use the add_user() method of the Group class of the IAM resource:
import boto3
IAM_RESOURCE = boto3.resource('iam')
user = IAM_RESOURCE.User(
'admin'
)
group = IAM_RESOURCE.Group('Admin-Group')
group.add_user(UserName=user.name)
print('IAM user has been added to the group')
Here’s an execution output:
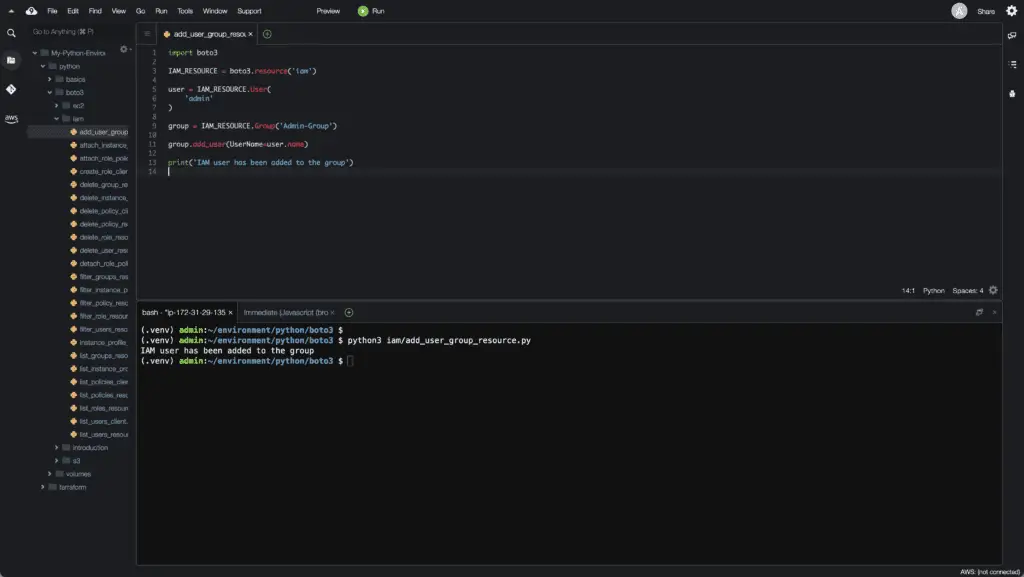
Alternatively, you can use theadd_user_to_group() method of the IAM client to add an IAM user to a group:
import boto3
client = boto3.client('iam')
response = client.add_user_to_group(
GroupName='TestGroup',
UserName='IAMTestUser'
)
print(response)
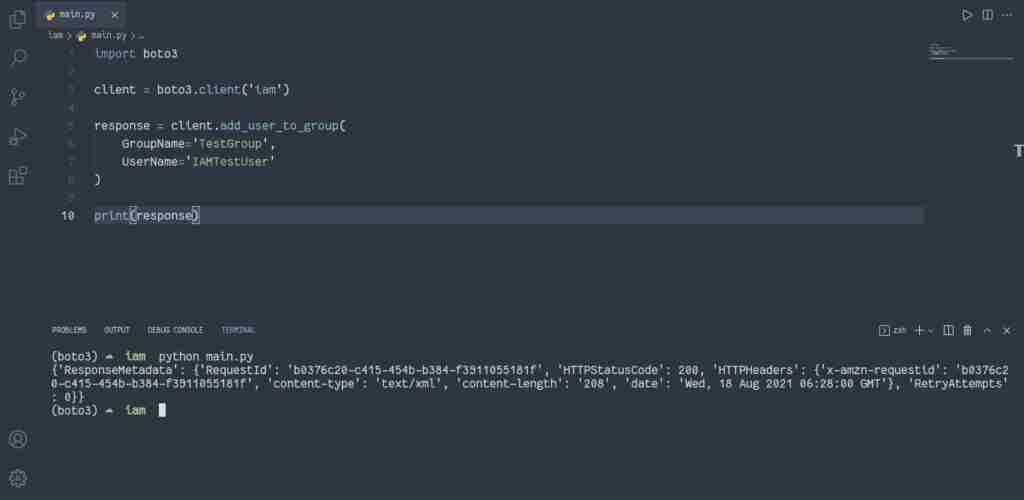
How to remove IAM user from group?
To remove the IAM user from a group, you must use the remove_user() method of the Group class of the IAM resource.
import boto3
IAM_RESOURCE = boto3.resource('iam')
user = IAM_RESOURCE.User(
'admin'
)
group = IAM_RESOURCE.Group('Admin-Group')
group.remove_user(UserName=user.name)
print('IAM user has been removed from the group')
Here’s an execution output:
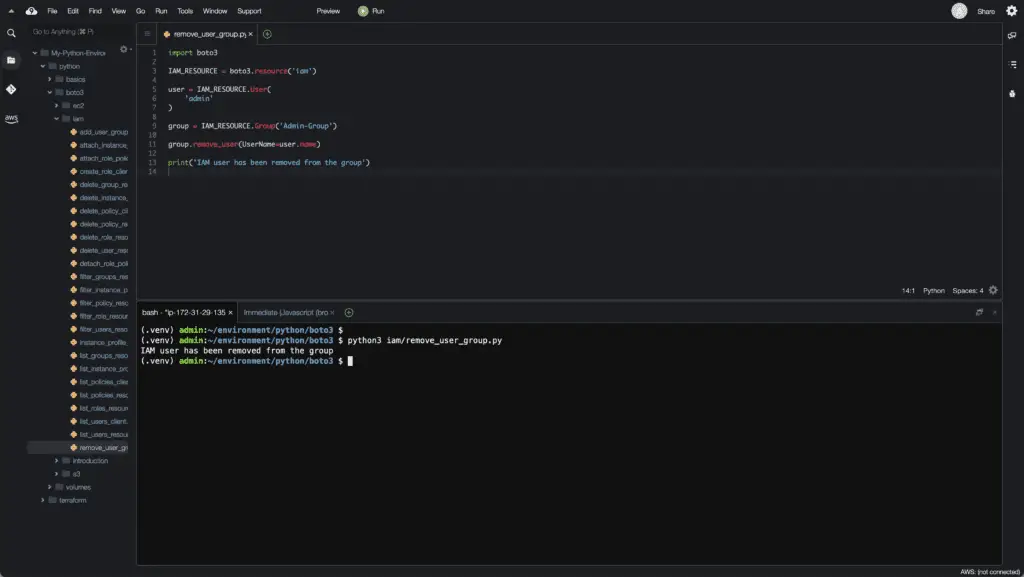
Alternatively, you can use theremove_user_from_group() method of the IAM client to achieve the same result:
import boto3
client = boto3.client('iam')
response = client.remove_user_from_group(
GroupName='TestGroup',
UserName='IAMTestUser'
)
print(response)
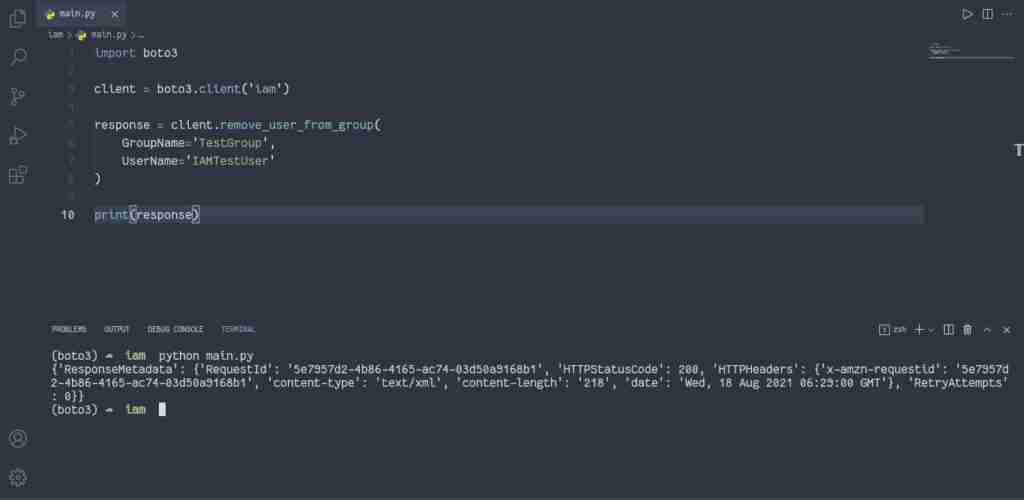
How to attach IAM policy to the user?
Toattach the IAM policy to the user, you must use theattach_policy() method of the User class of the IAM resource.
import boto3
iam = boto3.resource('iam')
user = iam.User('IAMTestUser')
response = user.attach_policy(
PolicyArn='arn:aws:iam::aws:policy/AmazonS3FullAccess'
)
print(response)

How to detach IAM policy from the user?
Todetach the IAM policy from the user, you need to use thedetach_policy()method of the User class of the IAM resource.
import boto3
iam = boto3.resource('iam')
user = iam.User('IAMTestUser')
response = user.detach_policy(
PolicyArn='arn:aws:iam::aws:policy/AmazonS3FullAccess'
)
print(response)
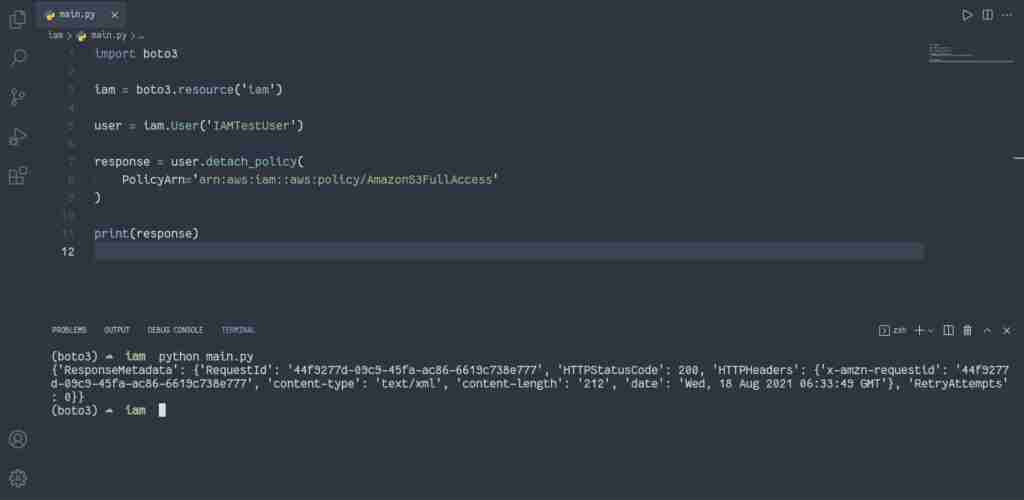
How to change IAM user password?
To change the IAM user password, you need to use the change_password() method that changes the password of the IAM user calling the operation. The account root user password is not affected by this operation. For more details, please check out the documentation.
import boto3
client = boto3.client('iam')
response = client.change_password(
OldPassword='string',
NewPassword='string'
)
print(response)
Managing IAM user API access keys
AWS API Access Keys are long-term credentials for IAM users. You can use Access Keys to get programmatic access to the AWS cloud using the AWS CLI or AWS SDKs.
How to create IAM Access Keys?
To create IAM Access Keys, you must use the create_access_key() method that creates new AWS Access and Secret Access Keys for the specified user.
import boto3
client = boto3.client('iam')
response = client.create_access_key(
UserName='IAMTestUser'
)
print(response)
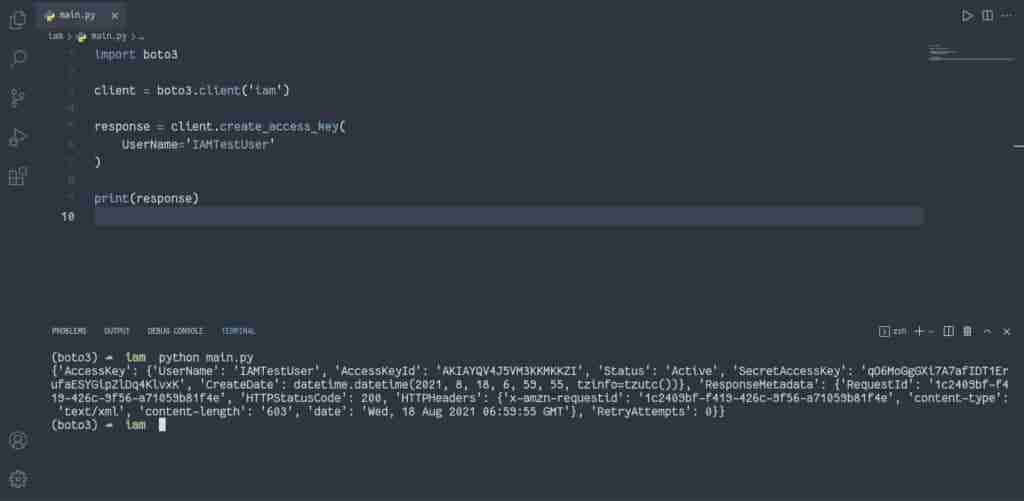
How to list IAM access keys?
To list IAM access keys, you can use the list_access_keys() method of the IAM client that returns information about the Access Key ID associated with the specified IAM user.
import boto3
client = boto3.client('iam')
response = client.list_access_keys(
UserName='IAMTestUser',
)
print(response)
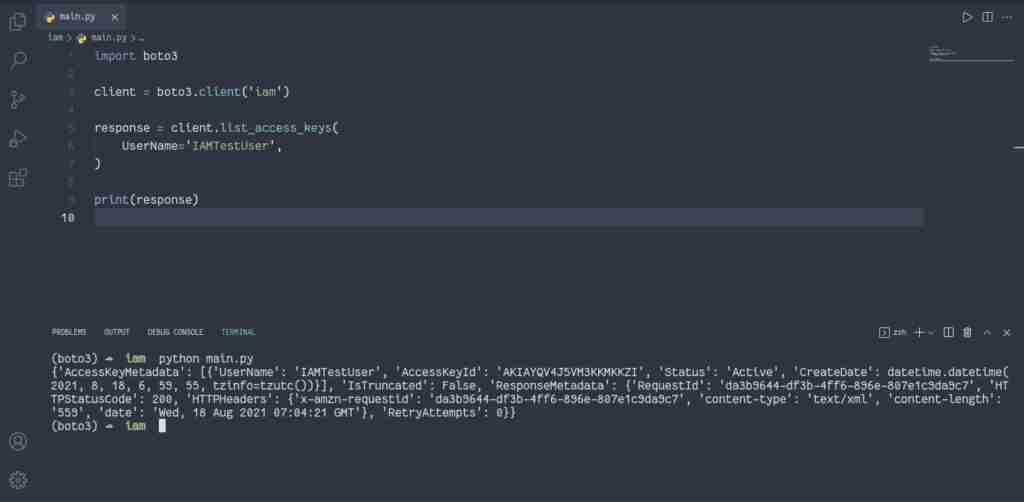
How to delete IAM access keys?
To delete IAM access, you can use the delete_access_keys() method of the IAM client that deletes the Access Key pair associated with the specified IAM user.
import boto3
client = boto3.client('iam')
response = client.delete_access_key(
UserName='IAMTestUser',
AccessKeyId='AKIAYQV4J5VM3KKMKKZI'
)
print(response)
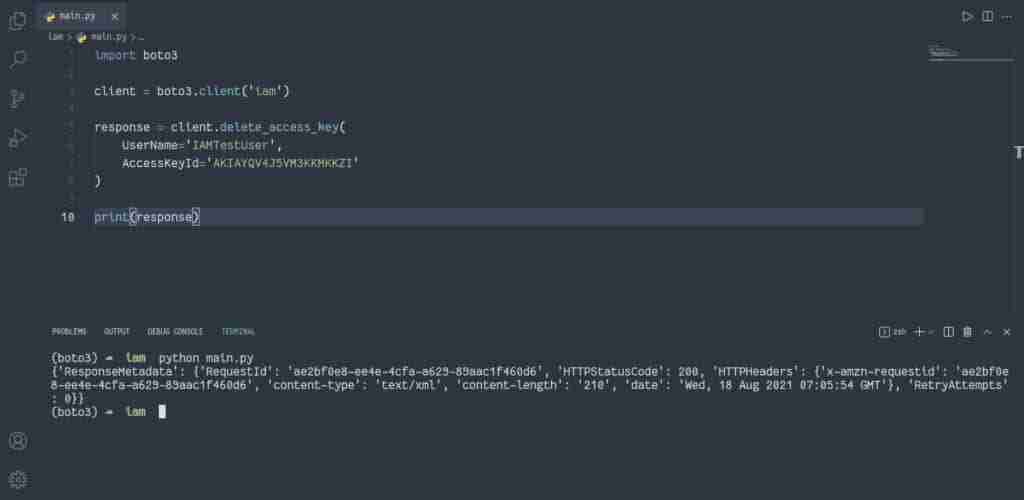
Managing IAM user login profile
IAM Login Profile creates a password for the specified user and provides access to AWS services through the AWS Management Console.
How to create login profile for IAM user?
To create the Login Profile for the IAM user, you need to use the create_login_profile() method of the IAM client that creates a password for the specified IAM user. A password allows an IAM user to access AWS services through the Management Console.
import boto3
client = boto3.client('iam')
response = client.create_login_profile(
UserName='IAMTestUser',
Password='test_password_for_user',
PasswordResetRequired=True
)
print(response)
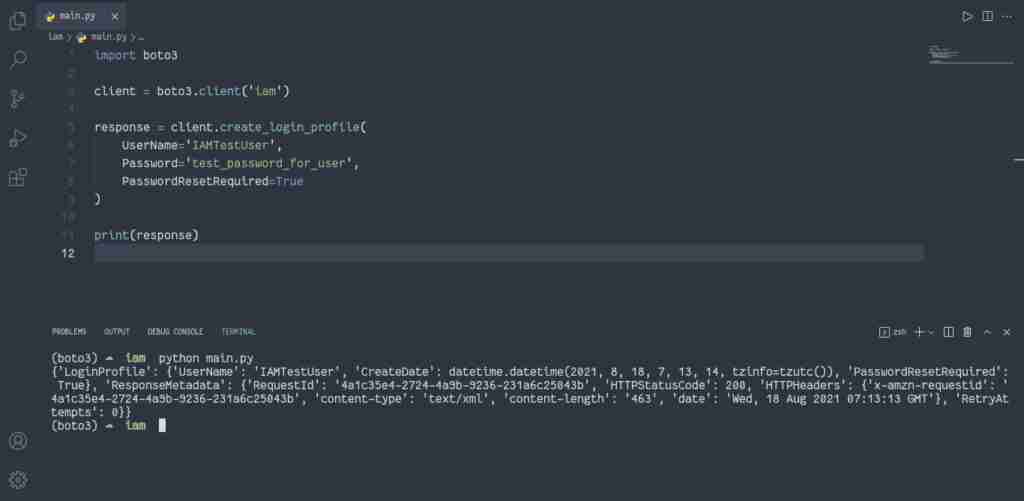
How to delete login profile from IAM user?
To delete the Login Profile from the IAM user, you need to use the delete_login_profile() of the IAM client that deletes the password for the specified IAM user and terminates the user’s ability to access Amazon Web Services services through the Management Console.
Note: Deleting a user’s password does not prevent accessing Amazon Web Services through the command-line interface or the API. To completely prevent users’ access to AWS, you must also either make all users’ Access Keys inactive or delete them.
import boto3
client = boto3.client('iam')
response = client.delete_login_profile(
UserName='IAMTestUser',
)
print(response)
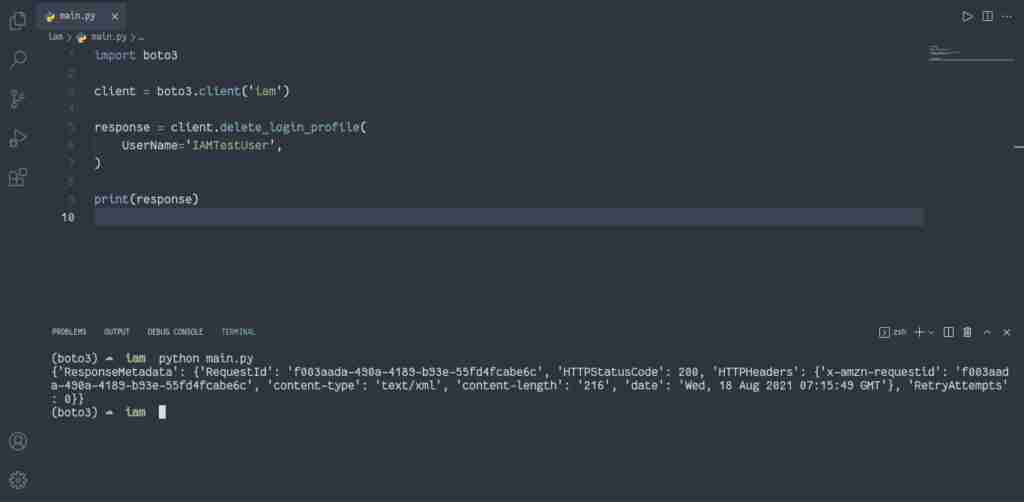
Generating IAM credentials report
IAM credentials report lists all users in your account and the status of their credentials usage, including passwords, access keys, and MFA devices.
Credential reports can assist you in auditing and compliance efforts. The report allows auditing credential lifecycle requirements, such as password and access key rotation. You can provide the report to an external auditor or grant permission to an auditor to download the report directly.
You can generate a credential report once every four hours. When you request a report, IAM first checks whether a report for the AWS account has been generated within the past four hours. If so, the most recent report is downloaded. If the most recent report for the account is older than four hours, or if there are no previous reports for the account, IAM generates and downloads a new report.
To generate the IAM credentials report, you need to use thegenerate_credential_report() of the IAM client:
import boto3
client = boto3.client('iam')
response = client.generate_credential_report()
print(response)
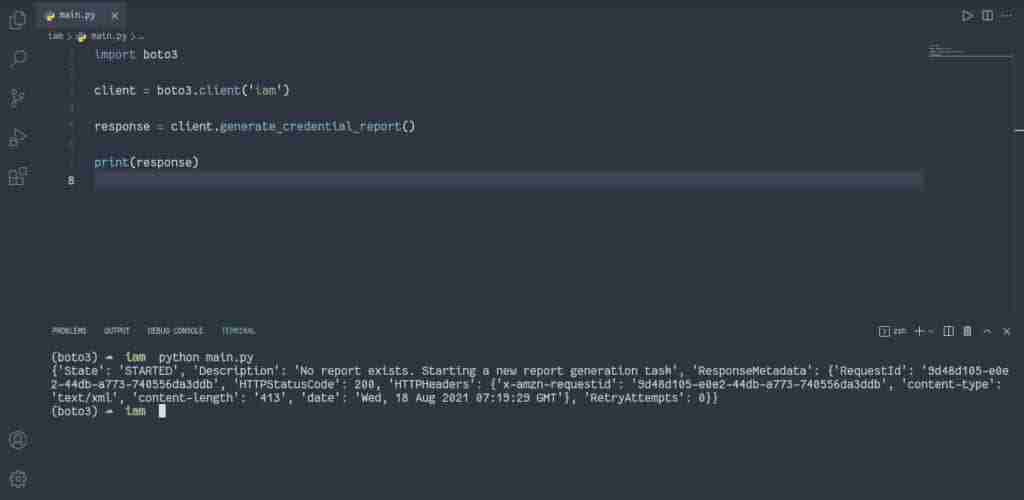
To download the IAM credentials report, you need to use theget_credential_report() of the IAM client:
import boto3
client = boto3.client('iam')
response = client.get_credential_report()
print(response)
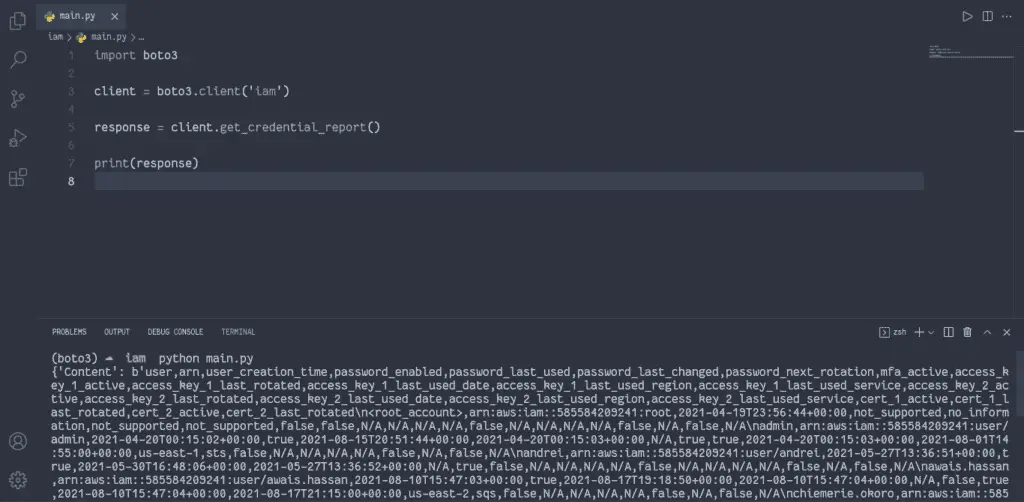
The IAM credential report contains data in CSV format, and it’s Base64-encoded, so you need to decode to string.
import boto3
client = boto3.client('iam')
response = client.get_credential_report()
report = response['Content'].decode("utf-8")
file = open('report.csv', 'w')
file.write(report)
file.close()
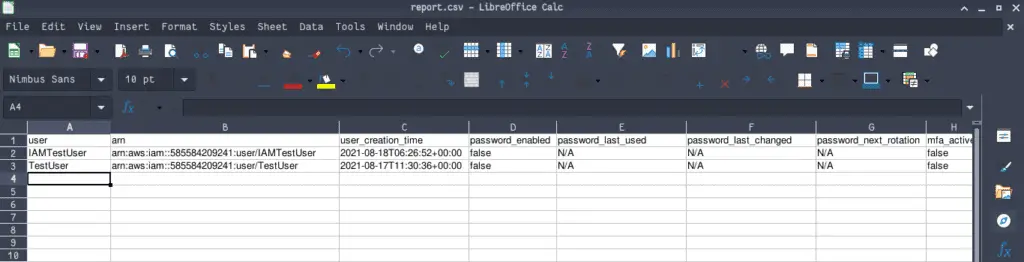
Summary
In this post, we’ve worked in depth with AWS IAM with the Python Boto3 library. We’ve explained using the Boto3 library to manage IAM Policies, Roles, Groups, and Users. We also created an EC2 instance with Boto3 and attached/associated IAM Instance Profile, which refers to the IAM Role. We looked at how assuming roles has better security than directly assigning permissions to a User or Group.