Python Conditionals – Complete Tutorial
In the previous chapters, we reviewed Python program structure, variables, and basic data types and operators in Python. The examples we’ve used before were very simple and allowed us only to set up different variables. Now, let’s introduce Python conditionals which will help us to add logic to our programs.
Table of contents
If statement
In Python, we use if statements for decision-making operations. The statement contains a body of code that runs only when the condition is given in the if statement is True
.
Here’s the statement syntax:
if BOOLEAN EXPRESSION:
STATEMENTS
A couple of important notes:
- The colon (
:
) after the boolean expression is required - All statements within the if statement must be indented
Here’s a diagram to describe a program execution flow:
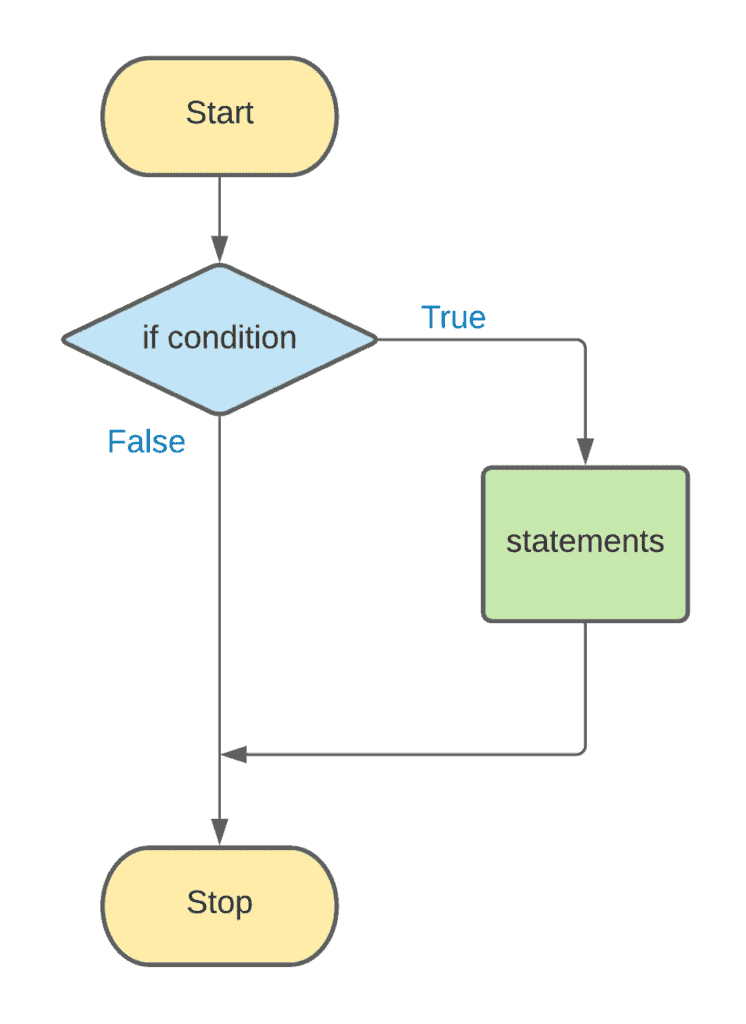
As an example, let’s try to check if the S3 bucket contains any objects:
#!/usr/bin/env python3
import boto3
s3_bucket_name = 'hands-on-cloud-python-course-src-bucket'
s3_resource = boto3.resource('s3')
s3_bucket = s3_resource.Bucket(s3_bucket_name)
s3_objects = list(s3_bucket.objects.all())
if len(s3_objects) > 0:
print(f'S3 bucket {s3_bucket_name} contains objects')
In this example in lines 11-12 we introduced an if statement, which checks if the number of items (built-in len() function) in s3_objects the list is greater than zero.
Here’s how you can execute the example above in Cloud9 IDE:
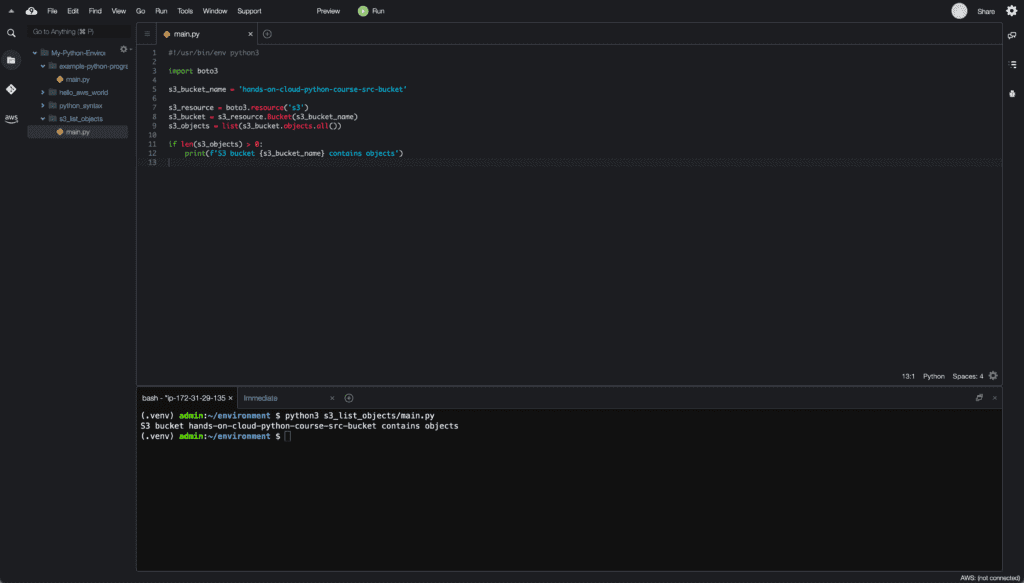
We’ll cover the boto3 library in more detail in the next chapters of the course.
If-else statement
The if statement can be extended with the optional else statement to execute some code if a boolean expression returned False
.
Here’s the statement syntax:
if BOOLEAN EXPRESSION:
STATEMENTS BLOCK 1
else:
STATEMENTS BLOCK 2
A couple of important notes:
- The colon (
:
) after the boolean expression and else statement is required - All statements within the if statement and else must be indented
Here’s a diagram to describe a program execution flow:
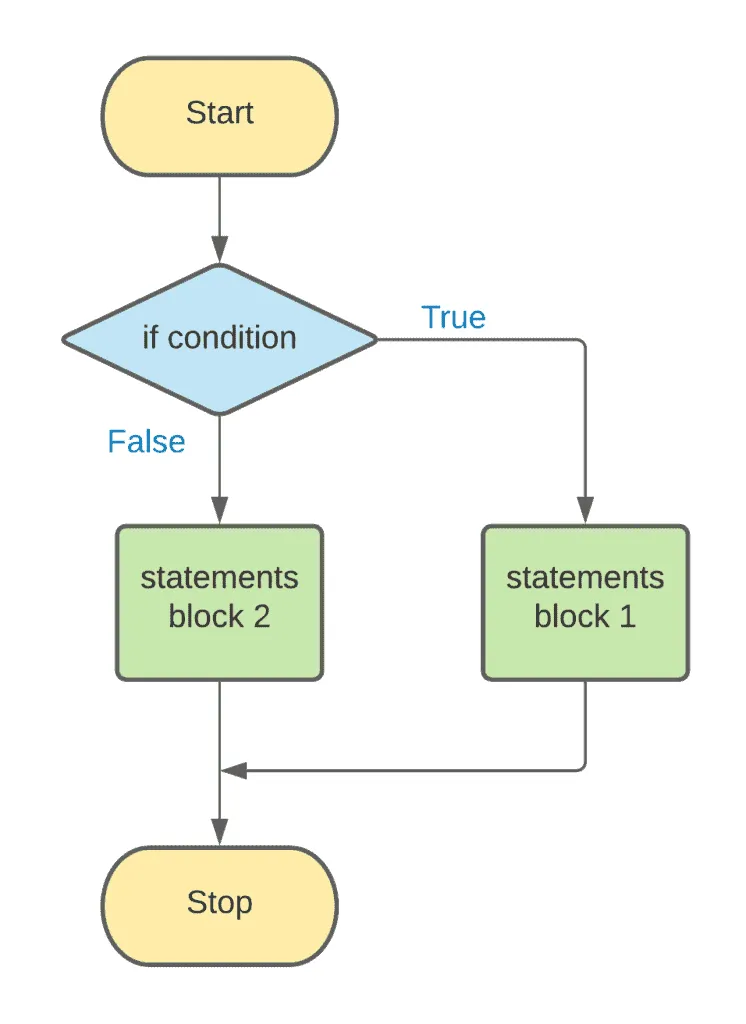
Let’s extend our previous example with the else statement:
#!/usr/bin/env python3
import boto3
s3_bucket_name = 'hands-on-cloud-python-course-src-bucket'
s3_resource = boto3.resource('s3')
s3_bucket = s3_resource.Bucket(s3_bucket_name)
s3_objects = list(s3_bucket.objects.all())
if len(s3_objects) == 0:
print(f'S3 bucket {s3_bucket_name} is empty')
else:
print(f'S3 bucket {s3_bucket_name} contains objects')
In this example in lines 11-14 we introduced an if-else statement, which checks allows us to check if the S3 bucket is empty or not.
Here’s how you can execute the example above in Cloud9 IDE:
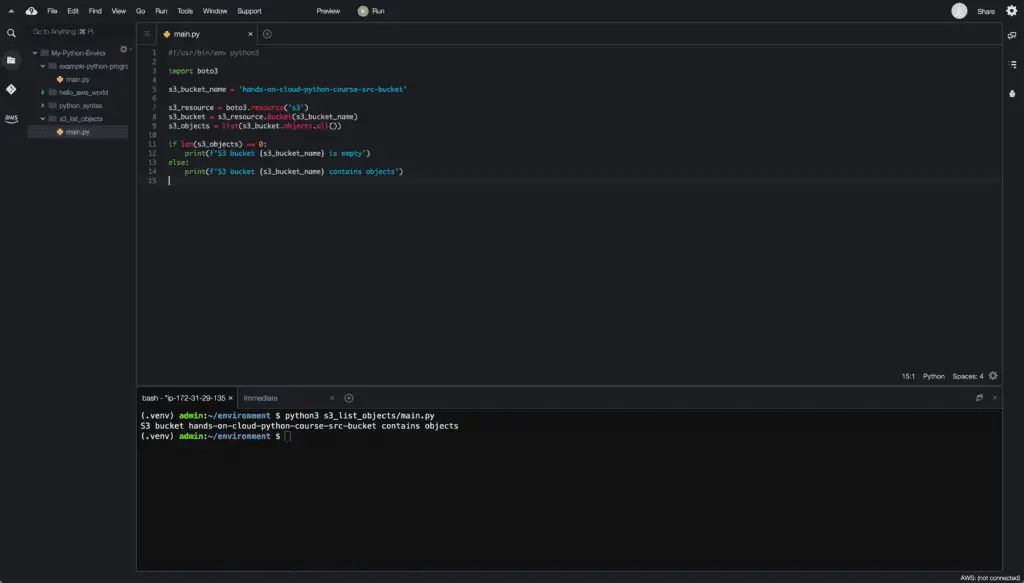
We’ll cover the boto3 library in more detail in the next chapters of the course.
Elif statement
The if statement can be extended with one or more optional elif statement blocks to add additional decision-making operations. The elif statement contains a body of code that runs only when the additional condition is True
.
Optional else statement can be added to execute some code if all previous boolean expressions returned False
.
Here’s the statement syntax:
if BOOLEAN EXPRESSION:
STATEMENTS BLOCK 1
elif BOOLEAN EXPRESSION:
STATEMENTS BLOCK 2
else:
STATEMENTS BLOCK 3
A couple of important notes:
- The colon (
:
) after the boolean expressions is and required after if, elif and else - All statements within the if, elif and else statement must be indented
Here’s a diagram to describe a program execution flow:
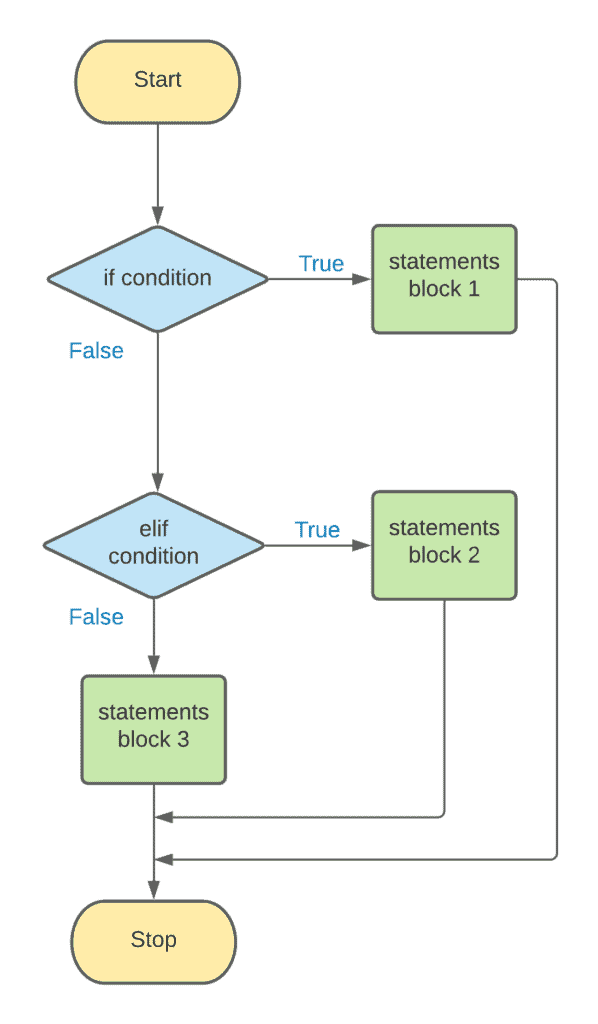
As an example, let’s try to classify the S3 bucket into three categories by the number of stored objects:
#!/usr/bin/env python3
import boto3
s3_bucket_name = 'hands-on-cloud-python-course-src-bucket'
s3_resource = boto3.resource('s3')
s3_bucket = s3_resource.Bucket(s3_bucket_name)
s3_objects = list(s3_bucket.objects.all())
if len(s3_objects) < 1000:
print(f'S3 bucket {s3_bucket_name} size is SMALL')
elif len(s3_objects) < 10000:
print(f'S3 bucket {s3_bucket_name} size is MEDIUM')
else:
print(f'S3 bucket {s3_bucket_name} size is LARGE')
In this example in lines 11-16, we introduced an if-elif-else statement, which classifies the S3 bucket by the number of stored objects.
Here’s how you can execute the example above in Cloud9 IDE:
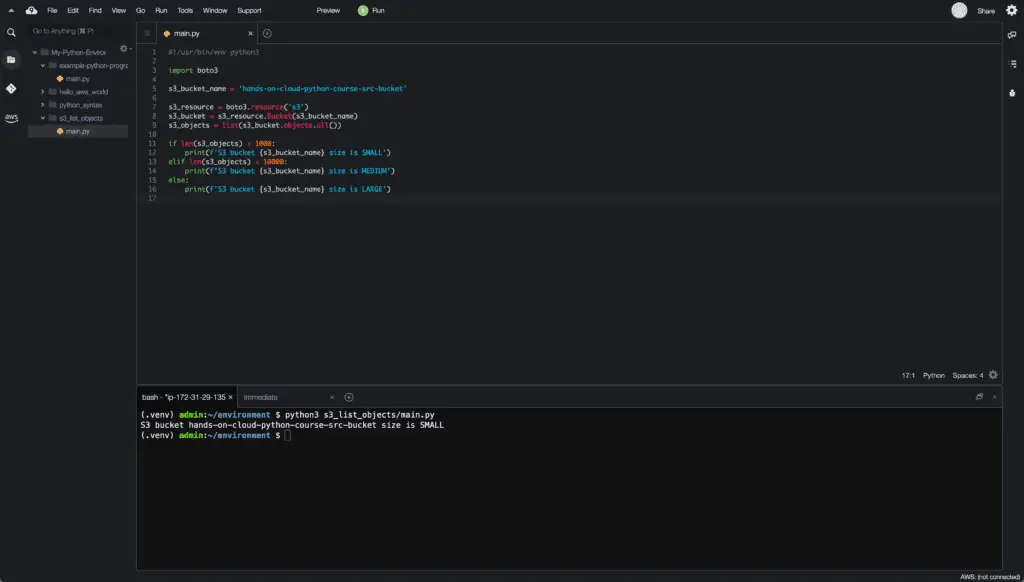
We’ll cover the boto3 library in more detail in the next chapters of the course.
Ternary operator
Ternary operators in Python perform an action based on a conditional statement result (true or false).
Their syntax is more concise than the syntax of traditional if-else statements.
Here’s the statement syntax:
expression_if_true if condition else expression_if_false
Let’s illustrate it on a simple example:
name = 'Andrei'
msg = f'Hi, {name}' if name else 'What is you name?'
print(msg)
print()
name = None
msg = f'Hi, {name}' if name else 'What is you name?'
print(msg)
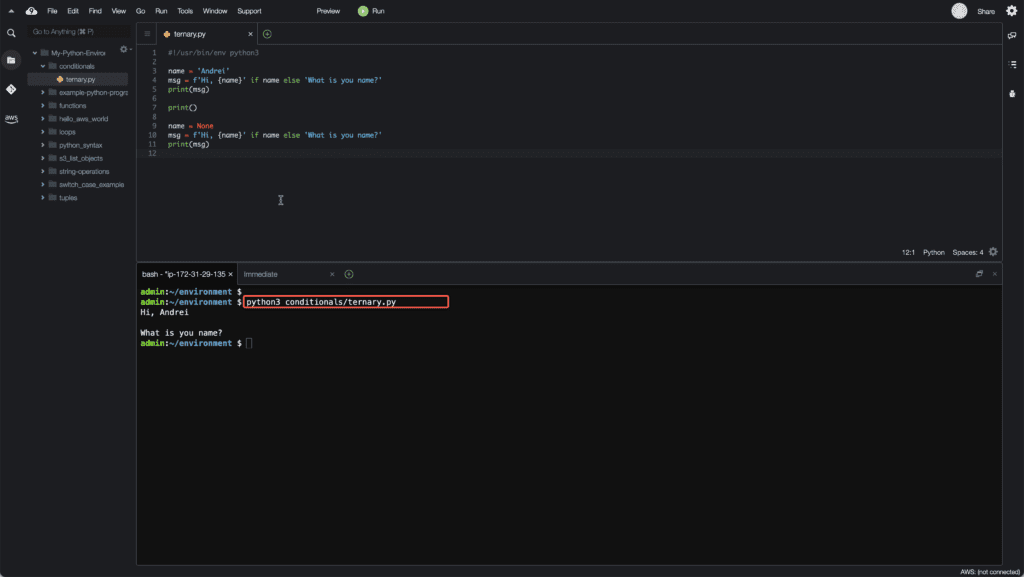
Switch case statement
A switch-case statement is another way of controlling program execution flow by comparing the switch case statement argument with predefined values to execute some code.
Here’s an illustration of this construct from other programming languages:
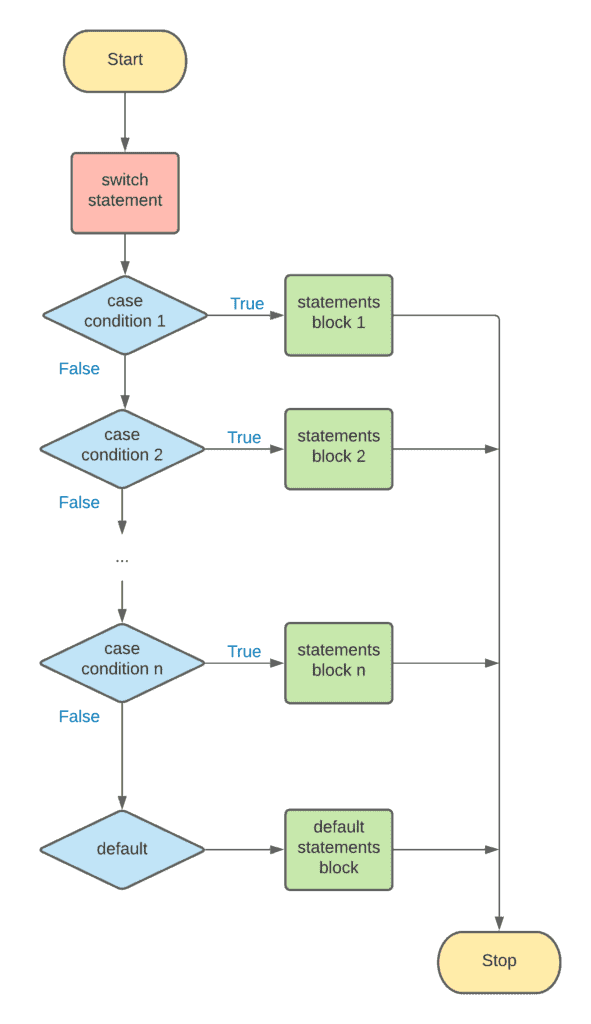
Python does not have a switch case statement, and you need to implement it yourself if you need it.
There are two ways of doing this:
- By using conditionals in function
- Or by using dictionary in function
We did not cover functions and dictionary operations in detail yet, but we still provide an implementation example for consistency.
Using conditionals to implement switch case statement
You’ve probably mentioned, that the switch-case flow diagram is similar to the if-elif-else statement.
Let’s use this similarity to implement switch case in Python:
#!/usr/bin/env python3
def switch(argument):
# case statement 1
if argument == "t3.nano":
print("Instance has 2 vCPU and 0.5 GiB RAM")
# case statement 2
elif argument == "t3.micro":
print("Instance has 2 vCPU and 1 GiB RAM")
# case statement 3
elif argument == "t3.small":
print("Instance has 2 vCPU and 2 GiB RAM")
# default
else:
print("Not supported instance type")
if __name__ == '__main__':
switch("t3.nano")
switch("t3.large")
Here’s how you can execute the example above in Cloud9 IDE:
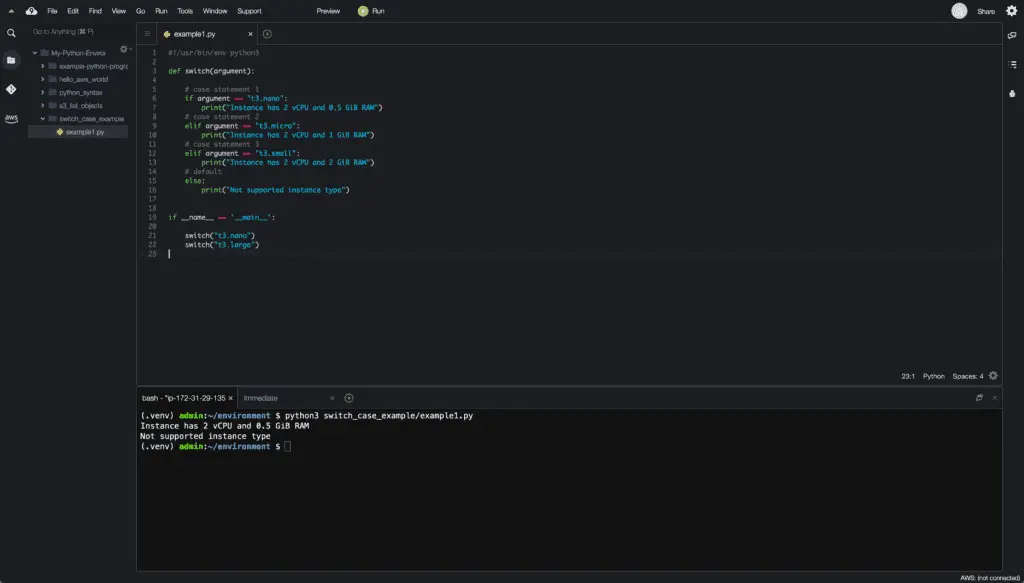
Using dictionary to implement switch case statement
In a similar way, you can use a Python dictionary to implement a switch case in Python.
Let’s modify our previous example to illustrate such an approach:
#!/usr/bin/env python3
def switch(argument):
messages = {
"t3.nano": "Instance has 2 vCPU and 0.5 GiB RAM",
"t3.micro": "Instance has 2 vCPU and 1 GiB RAM",
"t3.small": "Instance has 2 vCPU and 2 GiB RAM",
}
message = messages.get(argument, "Not supported instance type")
print(message)
if __name__ == '__main__':
switch("t3.nano")
switch("t3.large")
Here’s how you can execute example above in Cloud9 IDE:
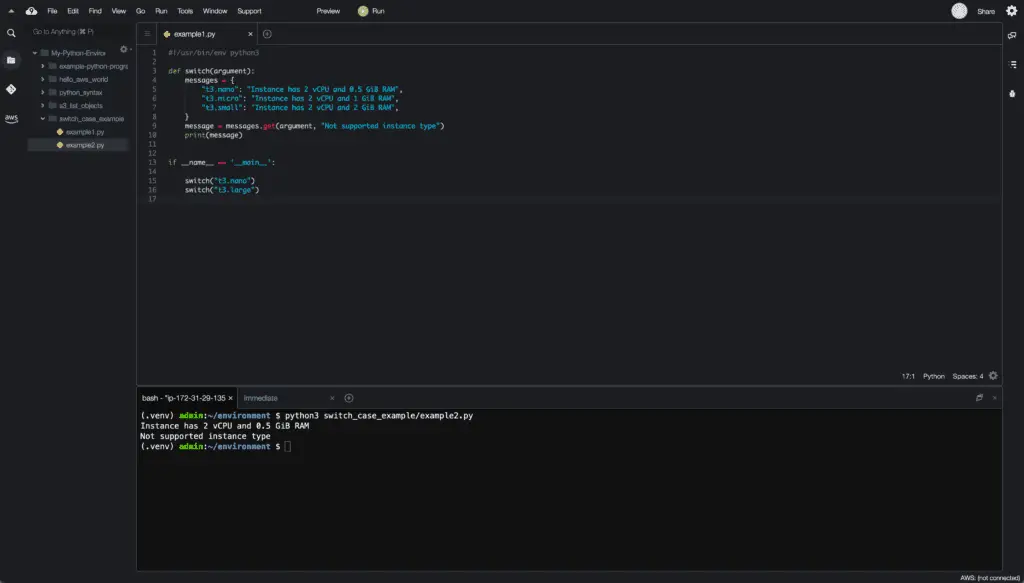
In the example above, we’re using messages
dictionary and its get() method to identify which message to print to describe EC2 instance resources.
The second argument to the get()
a function specifies a default value for our switch case implementation.