Python Operators – The Ultimate Guide
Python operators are symbols that represent computations like addition, subtraction, multiplication, and division. They can also be used to compare values and perform logical operations. Examples of Python Operators include arithmetic operators ( + , – , * , / ), comparison operators ( == , != , > , < , >= , <= ), assignment operators (=), logical operators (and, or, not) and bitwise operators (&, |, ~, ^, << >>).
In this chapter, you’ll learn the basic Python operators. All of them are important because you’ll be using them daily as a Cloud Automation Engineer. We’ll use real-life examples wherever possible.
Table of contents
What are operators in Python?
Operators are special symbols in Python that allows you to do arithmetic or logical operations.
The values that an operator acts on are called operands.
For example:
>> 1 + 5
6
In the example above:
+
is the operator that does the sum operation.1
and5
are the operands6
is the result/output of the operation

Operator precedence
Operator precedence defines the order in which the interpreter processes operators. The following table summarizes the operator precedence in Python, from lowest to highest:
Operator precedence | Operator Category | Operator Example |
---|---|---|
1 | Logical | or |
2 | Logical | and |
3 | Logical | not |
4 | Relational | <,>,<=,>=,==,!= |
5 | Addition | +,- |
6 | Multiplication | *,/,//,% |
7 | Exponent/Power | ** |
Linke in complex mathematical expression, for example, multiplication takes precedence over addition and subtraction. Of course, you can use round brackets ( ) to control a specific operations order.
Assignment operators
Assignment Operators in Python are used for assigning the value of the right operand to the left operand.
There are multiple different assignment operators available for you in Python.
Let’s take a look at the most commonly used ones.
Simple assignment ( = )
The simple assignment operator assigns a value to a single or multiple variable(s).
Here are some examples:
>> x = 10
>> print(x)
10
>> x = y = z = 10
>> print(x)
10
>> print(y)
10
>> print(z)
10
>> x, y, z = 1, 2, 3
>> print(x)
1
>> print(y)
2
>> print(z)
3
Interpreter output at Cloud9 IDE:
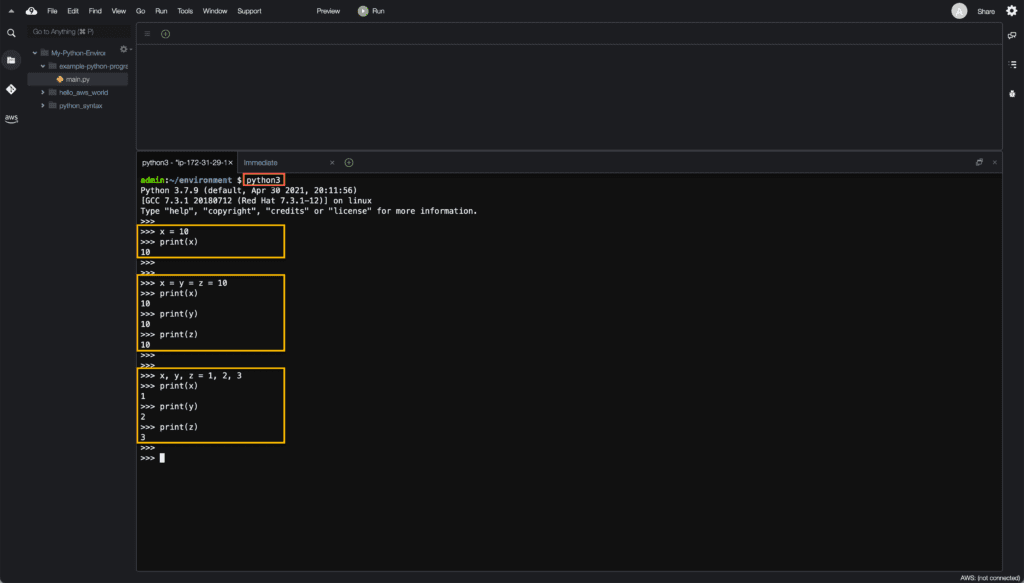
Here’s a classic example showing how to swap variable values:
>> x = 0
>> y = 1
>> x, y = y, x
>> print(x)
1
>> print(y)
0
Interpreter output at Cloud9 IDE:
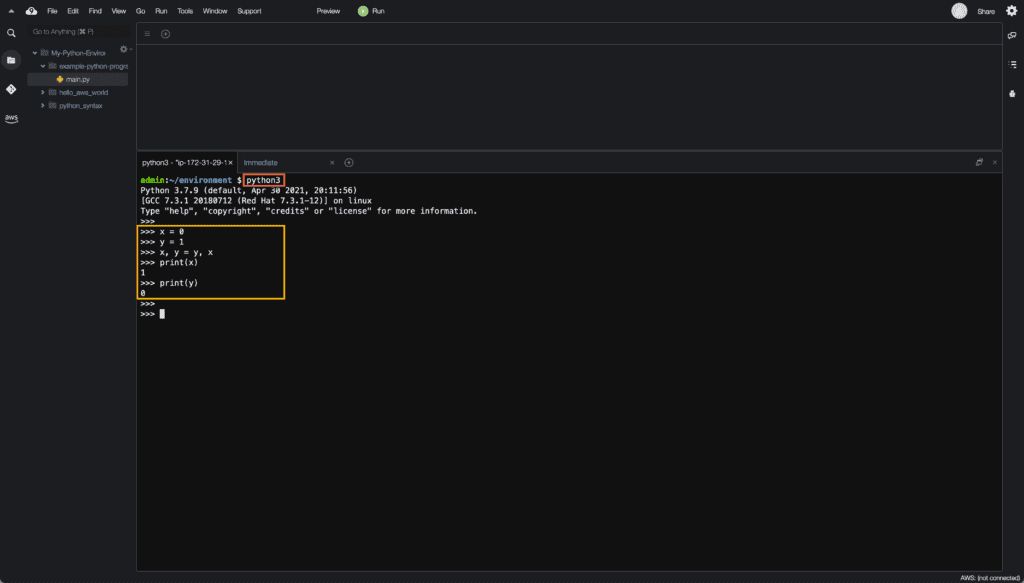
Increment assignment ( += )
The increment assignment operator adds a value to the variable and assigns the result to the same variable.
>> x = 15
>> x += 2
>> print(x)
17
The alternative way of doing an increment assignment is to use its longer form:
>> x = 15
>> x = x + 2
>> print(x)
17
Both examples will produce completely the same results.

Decrement assignment ( -= )
The decrement assignment operator subtracts a value from the variable and assigns the result to that variable.
>> x = 33
>> x -= 3
>> print(x)
30
# An alternative way of decrementing variable value
>> x = 33
>> x = x - 3
>> print(x)
30
Interpreter output at Cloud9 IDE:

Multiplication assignment ( *= )
The multiplication assignment operator multiplies the variable by a value and assigns the result to that variable.
Here’s an explanation example:
>> x = 10
>> x *= 3
>> print(x)
30
# An alternative way of multiplying a variable value
>> x = 10
>> x = x * 3
>> print(x)
30
Interpreter output at Cloud9 IDE:

Division assignment ( /= )
The division assignment operator divides the variable by a value and assigns the result to that variable.
Here’s an explanation example:
>> x = 10
>> x /= 2
>> print(x)
5.0
# An alternative way of dividing a variable value
>> x = 10
>> x = x / 2
>> print(x)
5.0
Interpreter output at Cloud9 IDE:
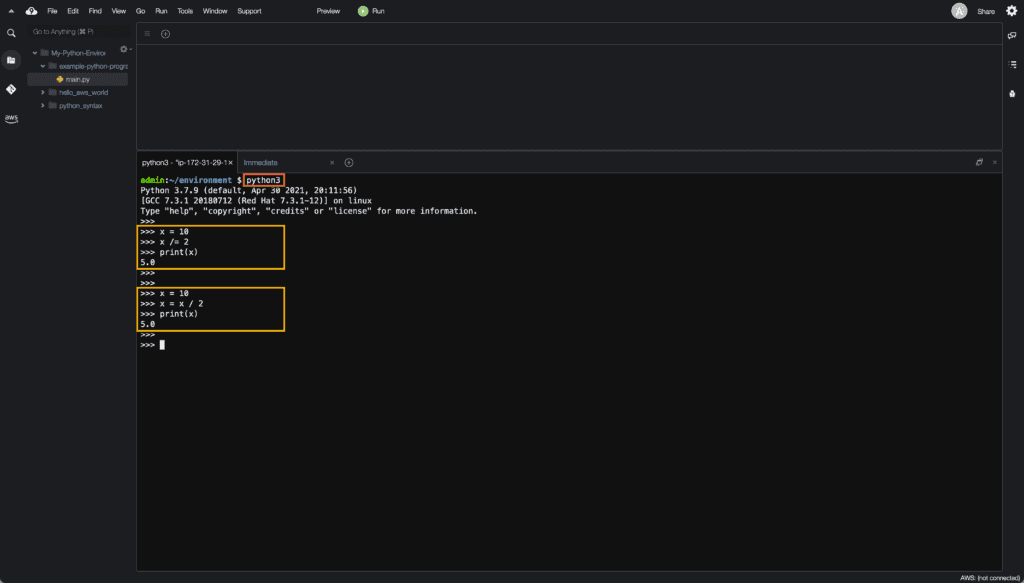
Other operators
Similarly to increment, decrement, multiplication and division operators, Python supports:
- The power assignment operator (
**=
) - The modulus assignment operator (
%=
) - The floor division assignment (
//=
)
But those operators are rarely faced in cloud automation activities.
Arithmetic operators
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, etc.
Addition ( + )
The addition operator returns the sum of two expressions.
For example:
>> 1 + 5
6
Interpreter output at Cloud9 IDE:

Subtraction ( – )
The subtraction operator returns the difference of two expressions.
>> 2 - 3
-1
Interpreter output at Cloud9 IDE:
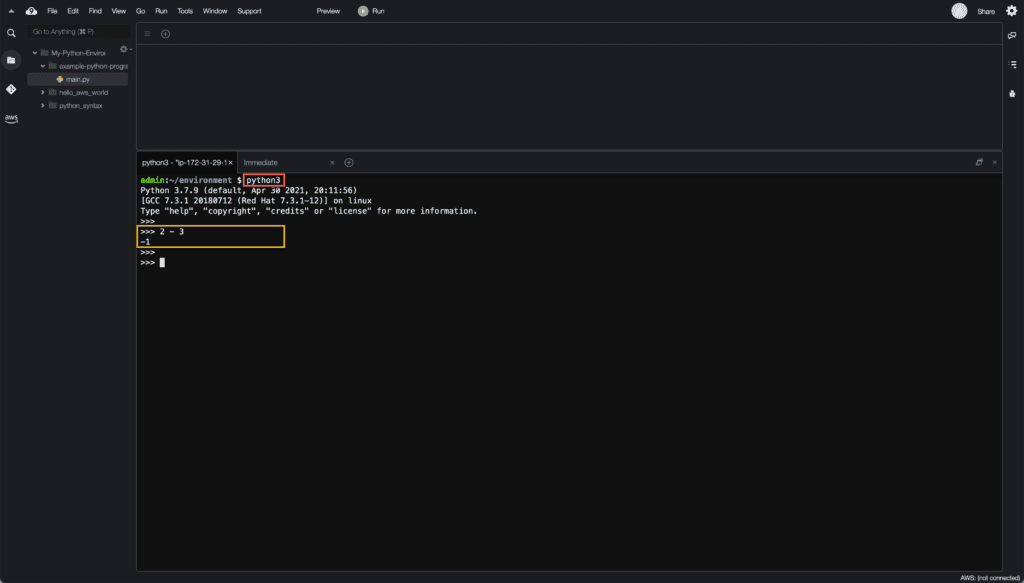
Multiplication ( * )
The multiplication operator returns the product of two expressions.
# integer multiplication example
>> 2 * 5
10
# float multiplication example
>> 2 * 5.0
10.0
Interpreter output at Cloud9 IDE:
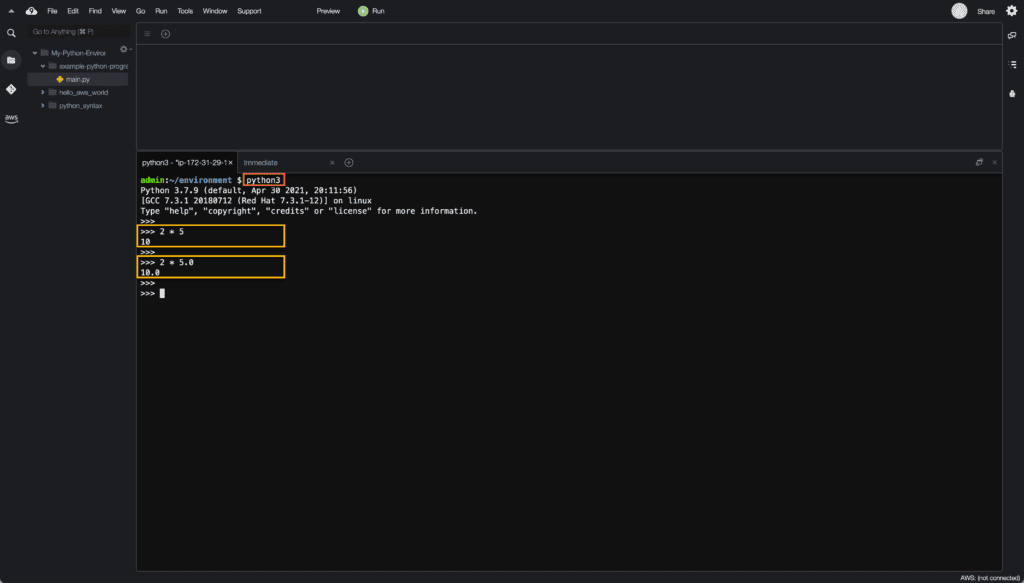
Power ( ** )
The power operator returns the value of a numeric expression raised to a specified power.
In addition to the power operator, Python has a pow() function, which acts in the same way.
Note: pow(0, 0)
and 0 ** 0
to be 1, as is common for programming languages.
>> 3 ** 3
27
>> 3 ** 3.0
27.0
>> 3.0 ** 3
27.0
>> 9 ** 0.5
3.0
>> 3 ** -3
0.037037037037037035
Interpreter output at Cloud9 IDE:
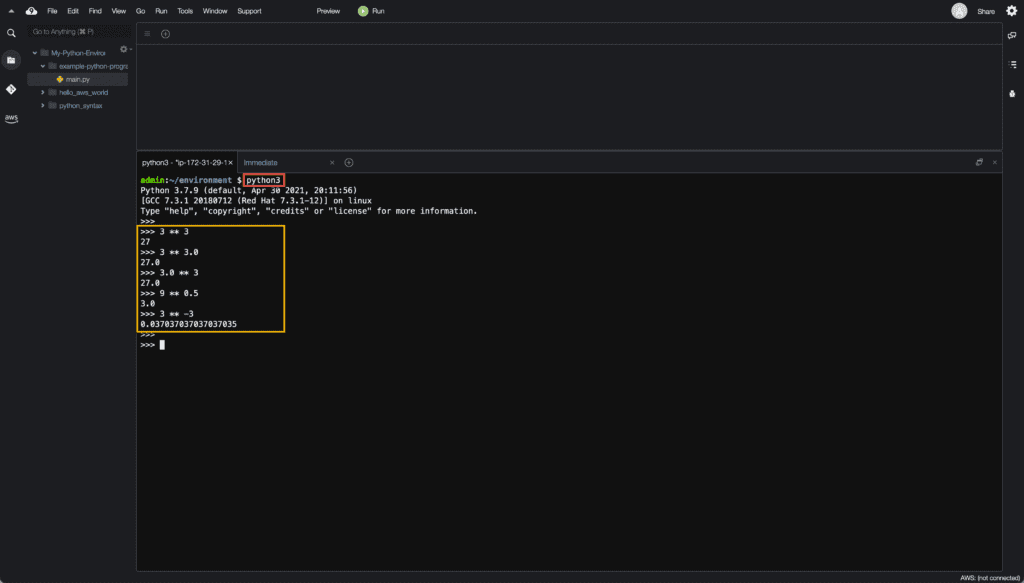
Division ( / )
The division operator returns the quotient of two expressions.
>> 3 / 1
3.0
>> 3 / 0.7
4.285714285714286
Interpreter output at Cloud9 IDE:

Other operators
Similarly to the mentioned arithmetic operators, Python supports:
But those operators are rarely faced in cloud automation activities.
Relational operators
Equal ( == )
The equal operator returns a Boolean value stating whether two expressions are equal or not.
# strings
>> 'xyz' == 'xyz'
True
>> 'Xyz' == 'xyz'
False
# integers
>> 1 == 1
True
>> 1 == 1.0
True
# objects
>> (1, 2) == 10
False
# variables
>> x = 5
>> y = 6
>> x == y
False
Interpreter output at Cloud9 IDE:
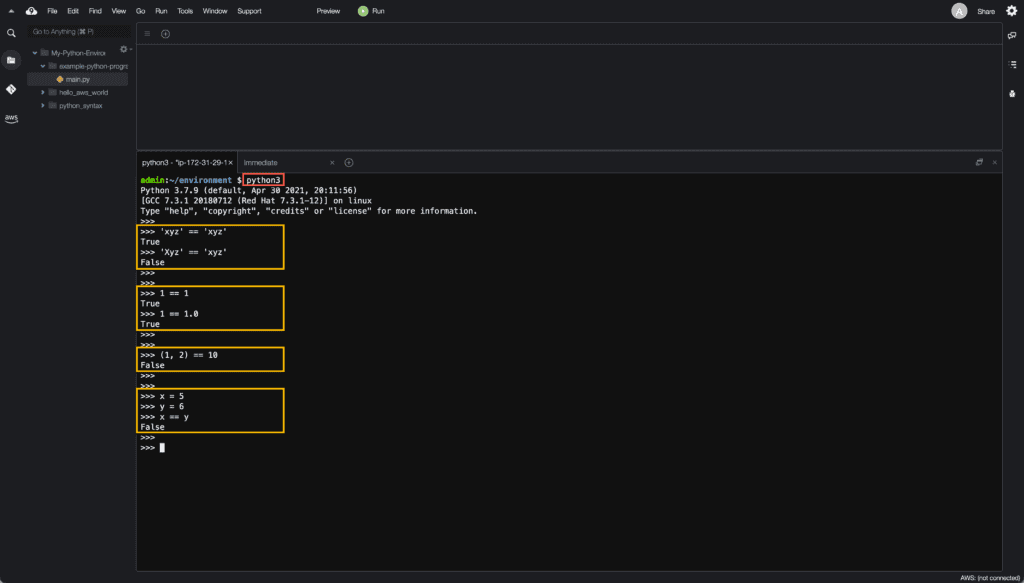
Not equal (!=)
The not equal operator returns a Boolean value stating whether two expressions are not equal.
# strings
>> 'xyz' != 'xyz'
False
>> 'Xyz' != 'xyz'
True
# integers
>> 1 != 1
False
>> 1 != 1.0
False
# objects
>> (1, 2) != 10
True
# variables
>> x = 5
>> y = 6
>> x != y
True
Interpreter output at Cloud9 IDE:

Greater than ( > )
Greater than operator returns a Boolean stating whether one expression is greater than the other.
>> 2 > 3
False
>> 4 > 3
True
Interpreter output at Cloud9 IDE:

Greater than or equal ( >= )
Greater than or equal operator is similar to greater than operator and returns a Boolean stating whether one expression is greater than or equal the other.
>> 3 >= 2
True
>> 3 >= 3
True
Interpreter output at Cloud9 IDE:
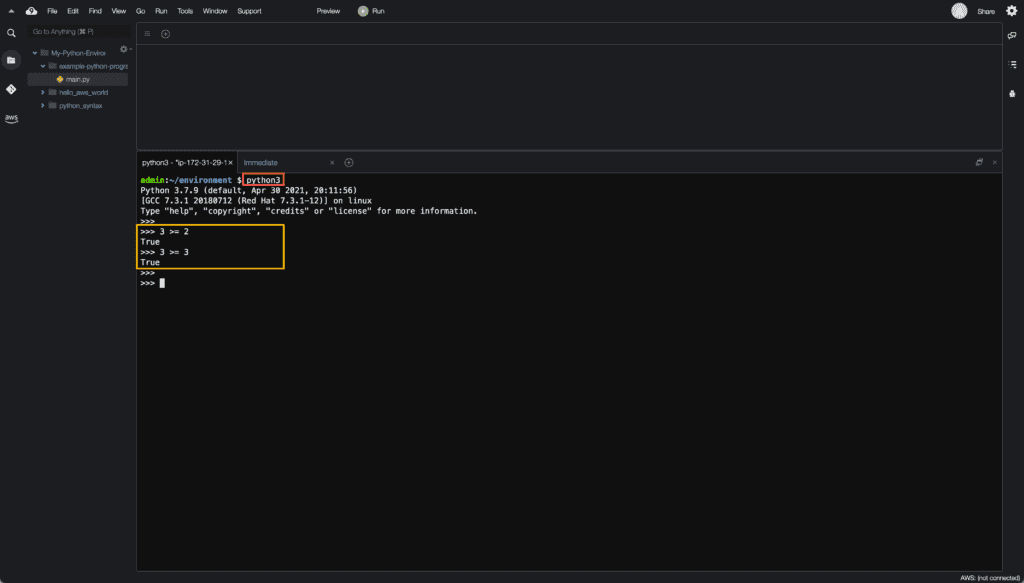
Less than ( < )
Less than operator returns a Boolean stating whether one expression is less than the other.
>> 3 < 3
False
>> 1 < 3
True
Interpreter output at Cloud9 IDE:

Less than or equal ( <= )
The less than or equal operator returns a Boolean stating whether one expression is less than or equal the other.
>> 4 <= 3
False
>> 3 <= 3
True
Interpreter output at Cloud9 IDE:
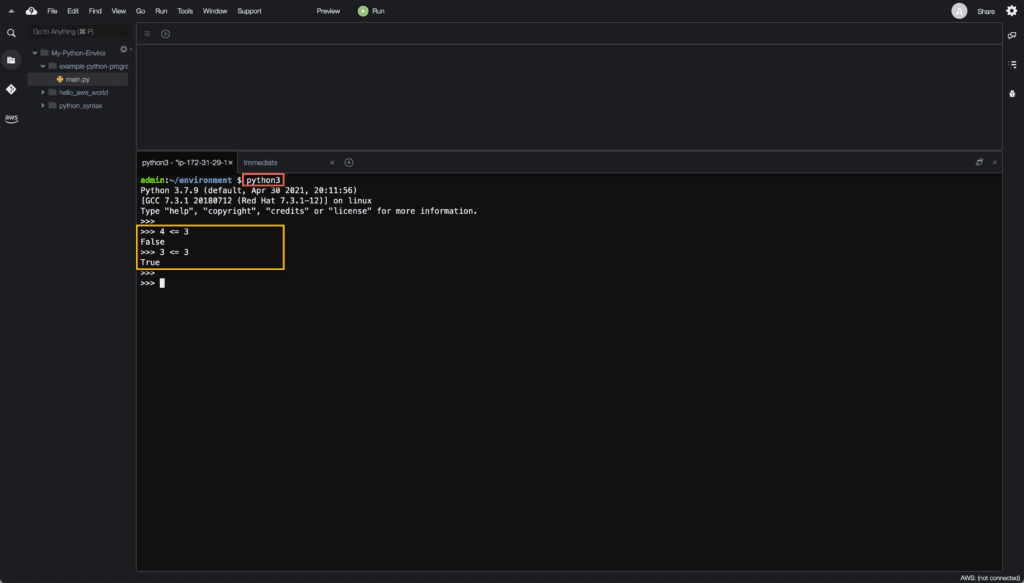
Boolean operators
There are three boolean operators in Python available: and, or and not.
Let’s review them one by one.
and
The and boolean operator returns the first operand that evaluates to False or the last one if all are True.
>> True and False
False
>> True and True
True
Interpreter output at Cloud9 IDE:
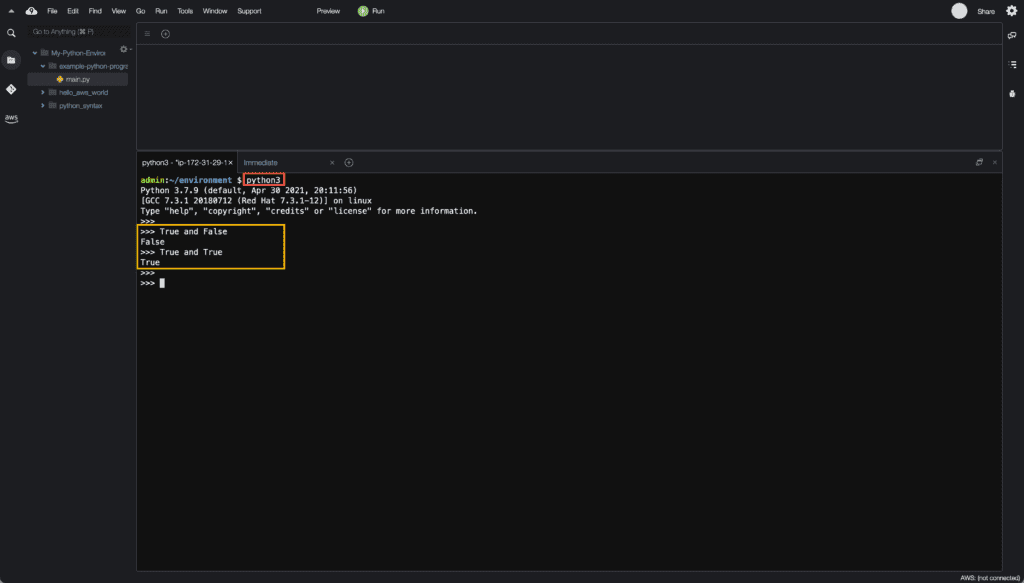
or
The or boolean operator returns the first operand that evaluates to True or the last one if all are False.
>> False or True
True
>> False or False
False
Interpreter output at Cloud9 IDE:
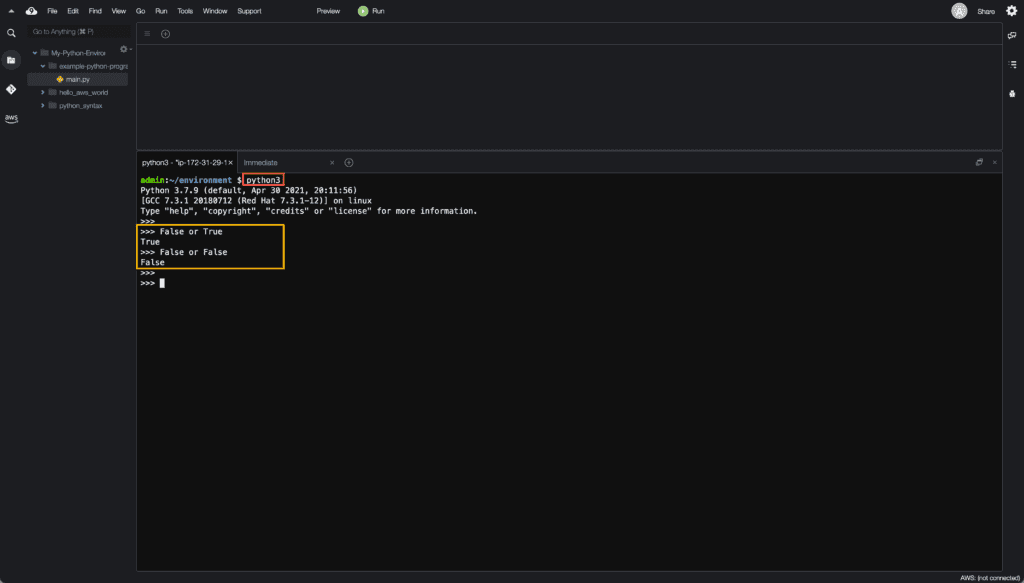
not
The not boolean operator returns a Boolean that is the reverse of the logical state of an expression.
# Example 1
>> not True
False
>> not False
True
# Example 2
>> True and not True
False
>> True and not False
True
>> True or not False
True
>> True or not True
True
Interpreter output at Cloud9 IDE:
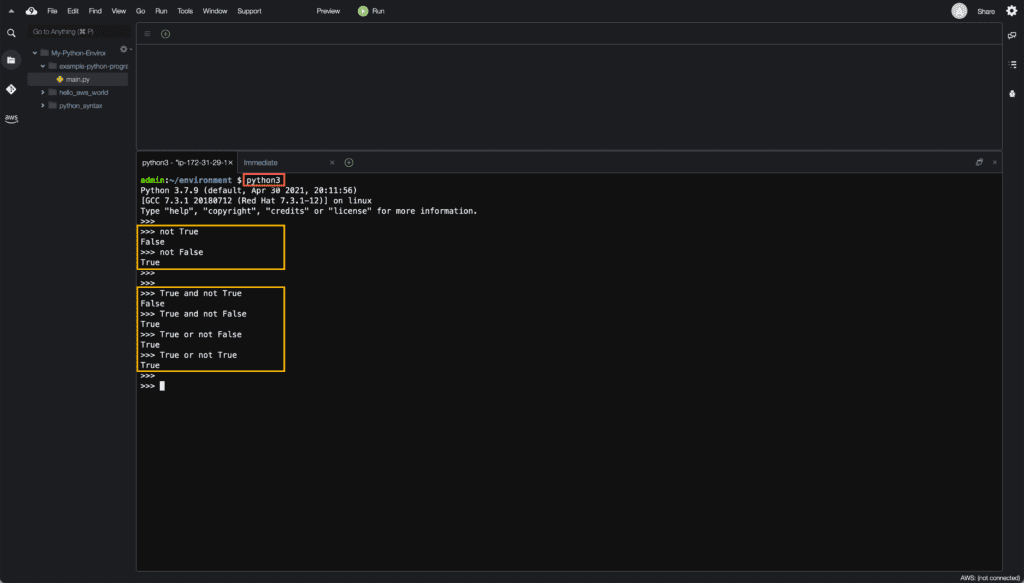
Membership operators
in
The in operator returns a Boolean stating whether the object exists in the container.
>> 'x' in 'xyz'
True
>> 5 in [3, 5, 7, 9]
True
Interpreter output at Cloud9 IDE:
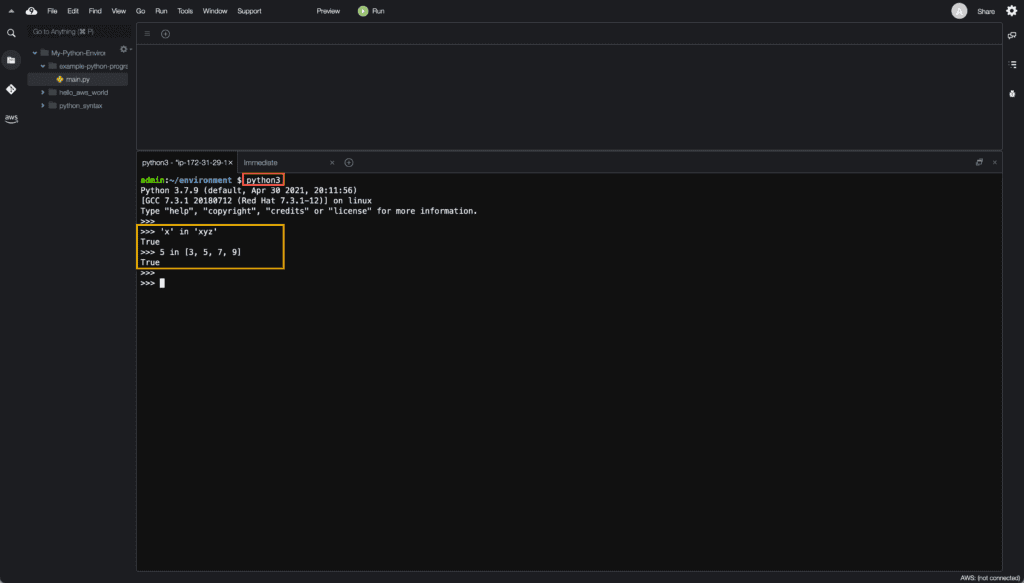
Conditional operator
if else
The if else conditional operator returns either value depending on the result of a Boolean expression.
In Python, conditional operator is similar to the if else statement.
It is also called a ternary operator since it takes three operands:
# Example 1
>> x = 5
>> y = 6
>> z = 1 if x < y else 0
>> print(z)
1
# Example 2
>> uptime = 100
>> status = 'green' if uptime > 99 else 'yellow'
>> print(status)
gren
Interpreter output at Cloud9 IDE:
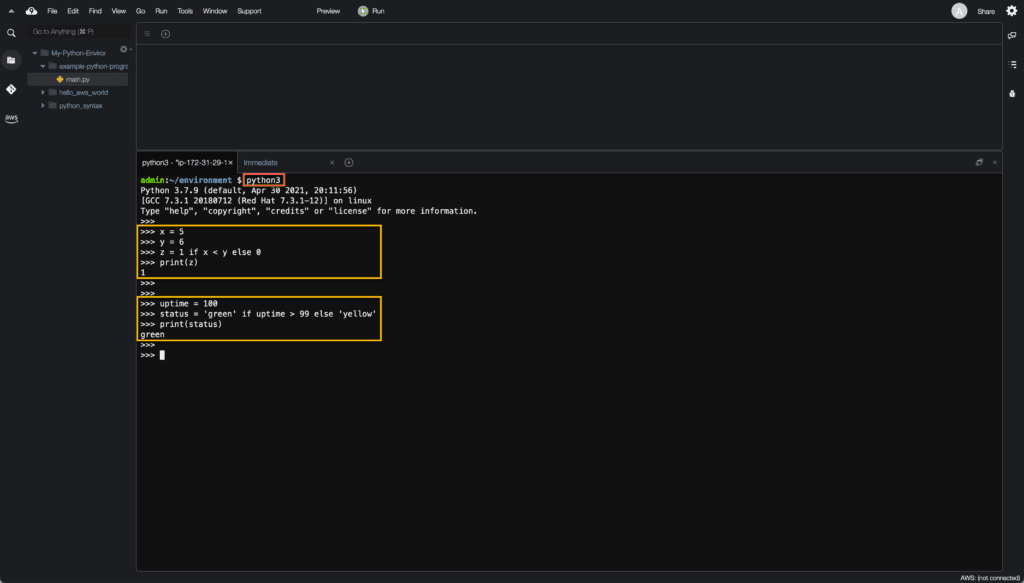
String operators
Concatenation ( + )
If concatenation operator used with strings or sequences, it will combine them:
# Example 1
>> greeting = "Hi, "
>> name = "Andrei"
>> message = greeting + name
>> print(message)
Hi, Andrei
# Example 2
>> list_1 = [0, 1]
>> list_2 = [2, 3]
>> result = list_1 + list_2
>> print(result)
[0, 1, 2, 3]
Interpreter output at Cloud9 IDE:
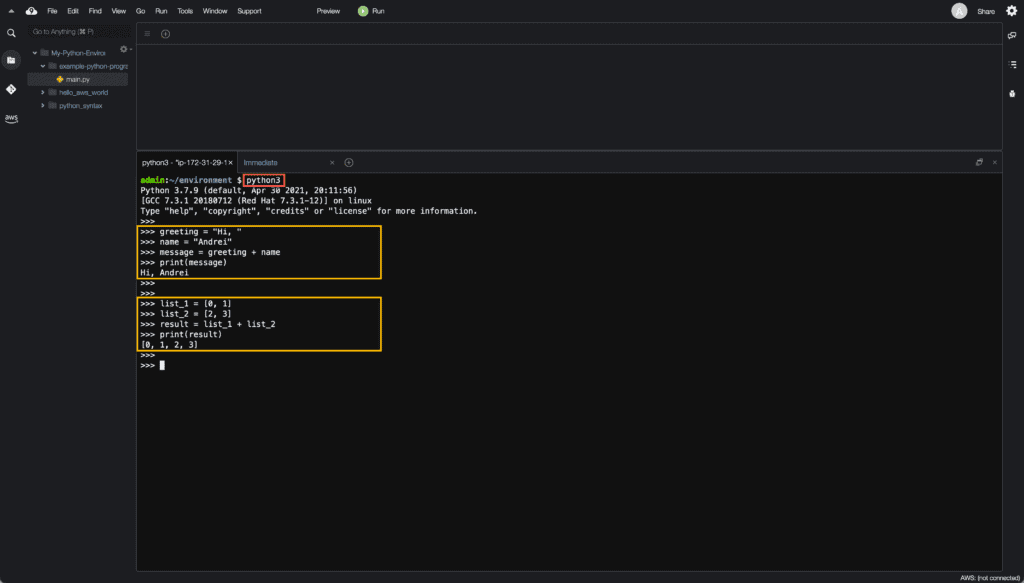
Multiple concatenations ( * )
The multiple concatenation operator returns a sequence self-concatenated specified amount of times.
# Example 1
>> message = '-' * 10
>> print(message)
----------
# Example 2
>> my_list = ['x', 'y', 'z']
>> result = my_list * 2
>> print(result)
['x', 'y', 'z', 'x', 'y', 'z']
Interpreter output at Cloud9 IDE:
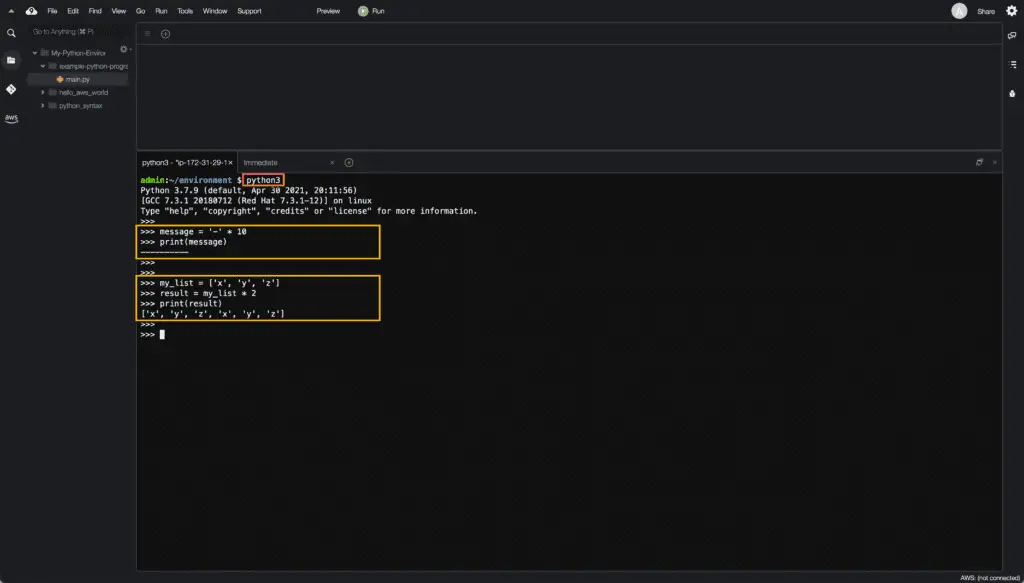