Python Sets – Complete Tutorial
If you need to find all unique elements from the list or find common or uncommon elements from two or more lists, Python sets are what you’re looking for. In this article, we’ll cover Python sets in detail and show how to get results of mathematical operations like union, intersection, symmetric difference, etc.
Table of contents
What is Python set?
A Python set is a collection of unordered, unindexed, and unchangeable items where all elements are unique (no duplicated elements).
There are two classes of sets that are available for you:
- set – this is a mutable collection of elements, so you can add or remove items in set when needed
- frozenset – this is immutable collection of elements, so you can’t change the set as soon as you’ve created it
To answer those questions, sets allow us to use mathematical set operations like union, intersection, symmetric difference, etc., which we’ll cover down below.
Advantages of Python sets
Python sets cannot store duplicate elements within them, so Python sets are highly useful for:
- Efficient removal of duplicate elements from a list and tuples
- Applying common mathematical operations like unions, intersections, differences, and asymmetric differences to collections of elements.
Sets vs lists and tuples
Here are two major differences between sets, lists, and tuples in Python:
- Lists and tuples store values in a sequence and can have duplicates.
- Sets store unordered values and can not have duplicates.
Managing Python sets
In this section, we’ll cover the basic operations with sets.
Creating Python set
You can create a set by putting all the set elements separated by commas inside curly braces {}
, or by using set()
class.
A set may consist of any number of elements of different types (integer, float, tuple, string, etc.), but it cannot contain mutable elements like lists, sets, dictionaries, or classes.
Here’s how you’re defining a set:
# elements of the same type
set_example = {3, 1, 2}
print(f'Example 1: {set_example}')
# elements of mixed type
set_example = {5.5, (1, 1, 0), "Joe"}
print(f'Example 2: {set_example}')
# set can't store duplicates
set_example = {1, 2, 3, 4, 3, 2}
print(f'Example 3: {set_example}')
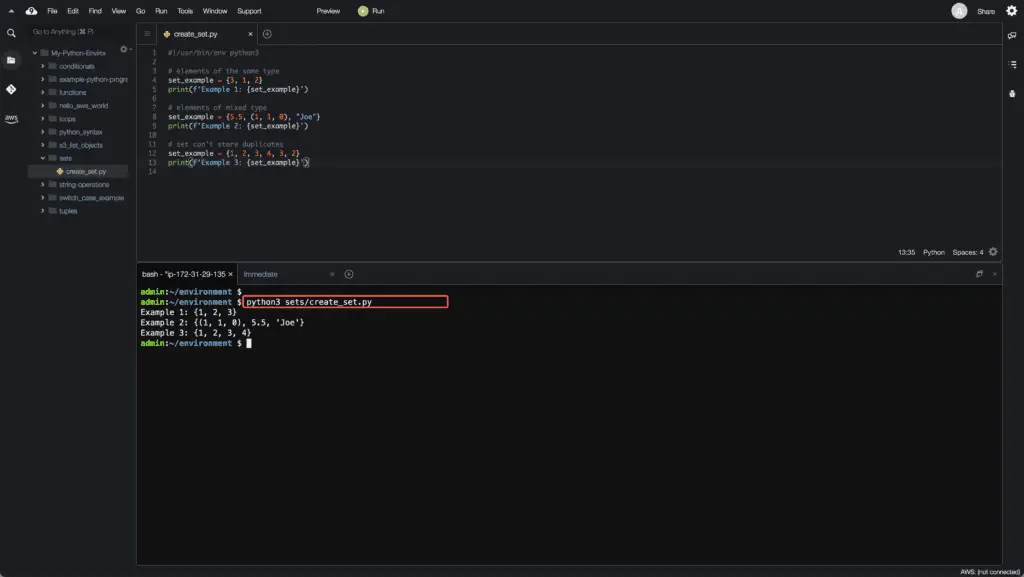
If you’d like to initialize an empty set, you can not use {}, because such syntax will create an empty dictionary.
Instead, use the set() class directly:
set_example = set()
print(set_example)
An attempt to store mutable elements in a set
set_example = {[1, 2], 3}
will throw an exception:
Traceback (most recent call last):
File "", line 1, in
TypeError: unhashable type: 'list'
Accessing elements in Python set
You can not access set items by referring to their index values, since sets do not have an index.
Instead, you can:
- Loop through the set items using a for loop
- Check if a specified value is present in a set, by using the in keyword
Here’s an example:
set_example = set(["hands", "on", "cloud"])
print(f'Example set: {set_example}')
# Accessing elements using
# for loop
for element in set_example:
print(f'Elements of set: {element}')
# Check if element exists in set
element = "cloud"
if element in set_example:
print(f'Element "{element}" exists in set')
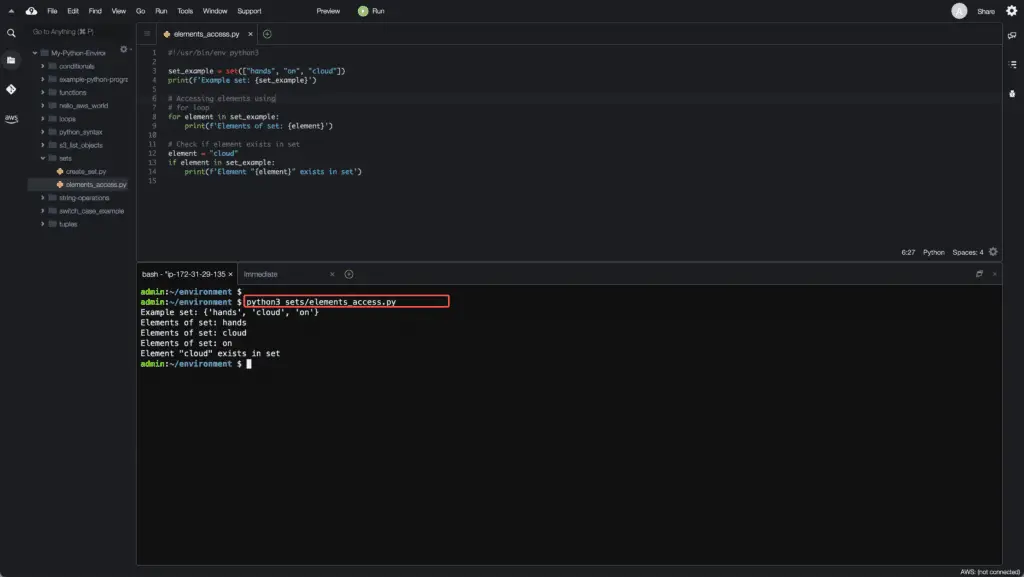
Adding elements to Python set
There are two methods available for you in the set class to add elements to the Python set:
add(element)
– adds one element at a timeupdate([elements_list])
– adds two or more elements to a set at a time from the list
Note: element of the type list cannot be used in the add()
method because the list is a mutable data type in Python.
Here’s an example:
set_example = set()
print(f'Initial set state: {set_example}')
# Adding elements to the set
set_example.add("hands")
set_example.add("on")
set_example.add("cloud")
set_example.add("313377")
print(f'Set state after adding elements: {set_example}')
# updating elements
set_example.update(["hands", True])
print(f'Set state after updating elements: {set_example}')
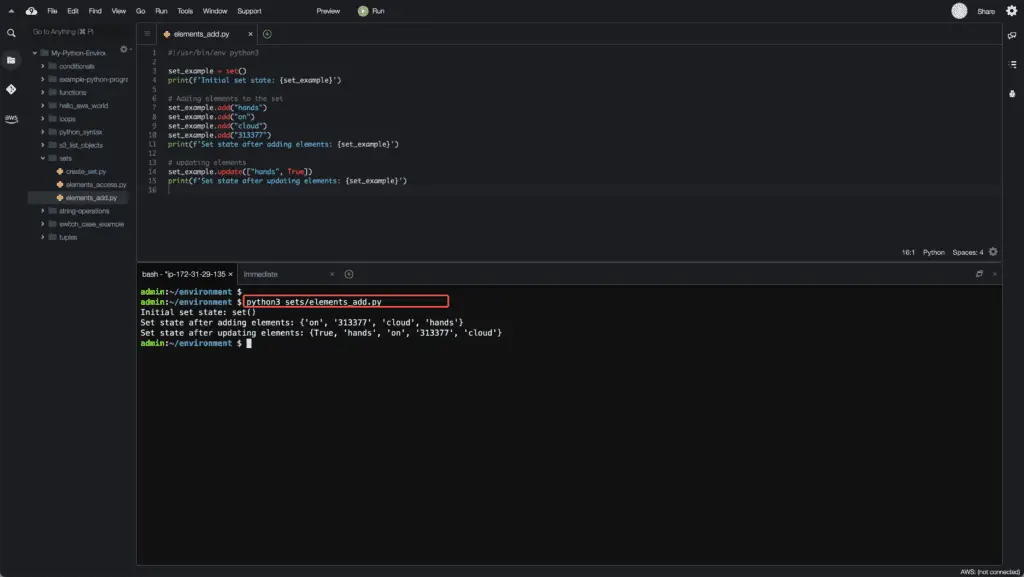
Removing elements from Python set
There are four methods available for you in the set class to remove elements to the Python set:
remove(element)
– removes element from the set or raises KeyError exception if the element does not existdiscard(element)
– deletes element from the set (the set ramains unchanged if there’s no such element in it)clear()
– removes all the elements from the setpop()
– removes last element from the set
set_example = set([1, 2, 3, 4, 5, 6, 7, 8, 9, 0])
print(f'Initial set state: {set_example}')
set_example.remove(1)
print(f'Set state after remove(): {set_example}')
set_example.discard(1)
set_example.discard(2)
print(f'Set state after discard(): {set_example}')
set_example.pop()
print(f'Set state after pop(): {set_example}')
set_example.clear()
print(f'Set state after clear(): {set_example}')
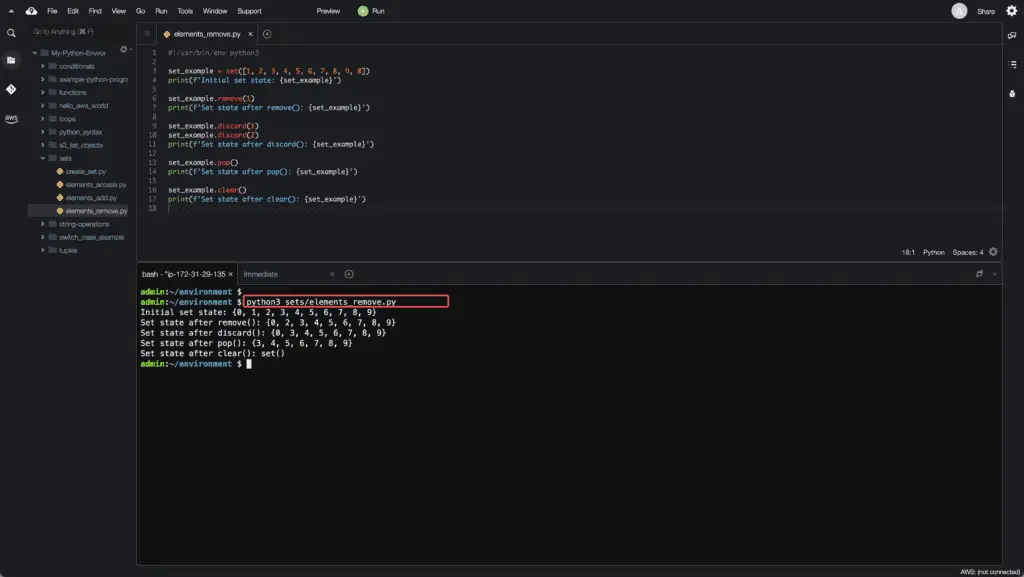
Iterating over sets in Python
There’s no way to access individual elements within a set by index, instead, you can iterate over set elements:
set_1 = {1, 2, 3, 4, 5}
for element in set_1:
print(f'Set element: {element}')
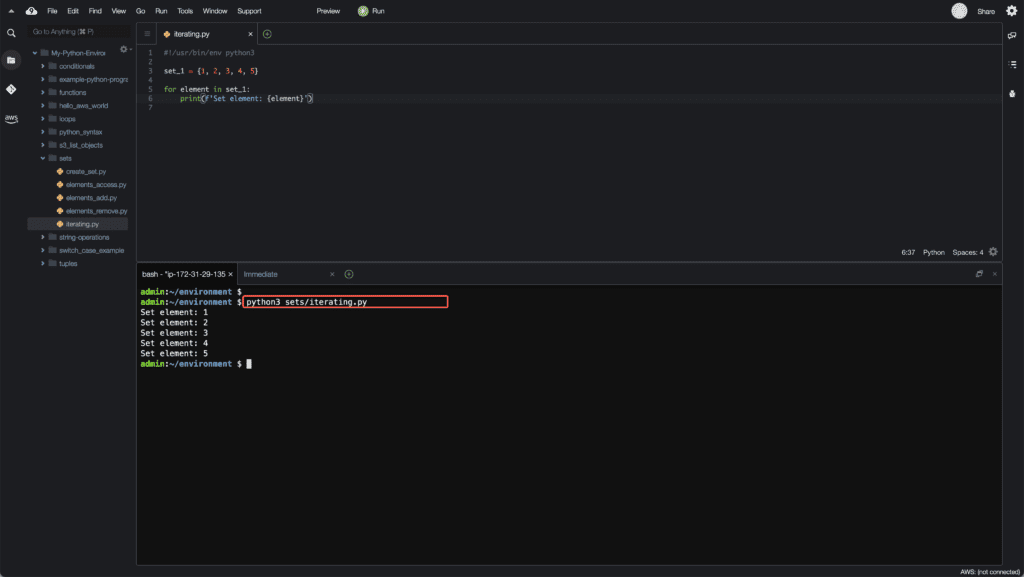
Test element membership in a set
You can check if an item exists in a set by using the in
keyword:
set_1 = {1, 2, 3, 4, 5}
element = "hands-on.cloud"
if element in set_1:
print(f'"{element}" belongs to the set {set_1}')
else:
print(f'"{element}" does not belong to the set {set_1}')
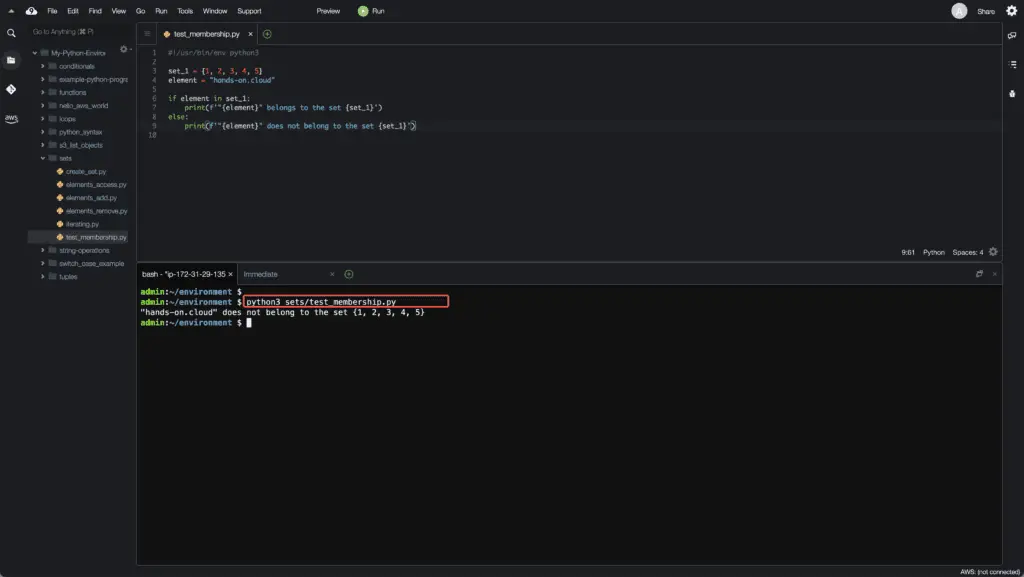
Ordering set values
If you need to get the values from the set in order, you can use the sorted
function to get an ordered list of set elements:
set_1 = {"x", "a", "g", "y", "t"}
ordered_list = sorted(set_1)
print(f'Set "{set_1}" elements in ordered form: {ordered_list}')
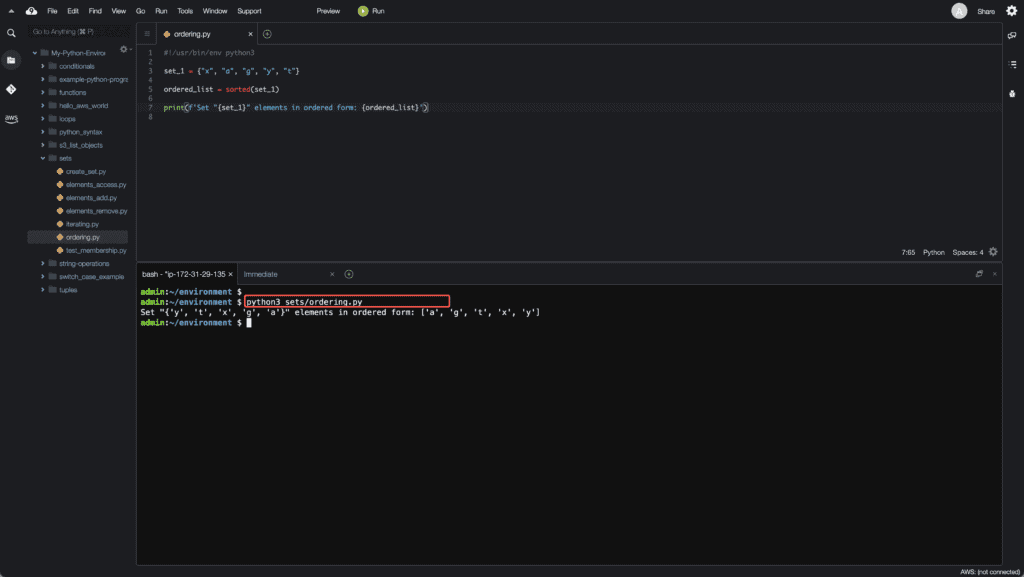
Remove duplicates from list
Sets are the fastest way to remove duplicates from a list. Let’s take a look, how to do it:
list_with_dups = ["x", "a", "a", "t", "t"]
list_without_dups = list(set(list_with_dups))
print(f'{list_with_dups} => {list_without_dups}')
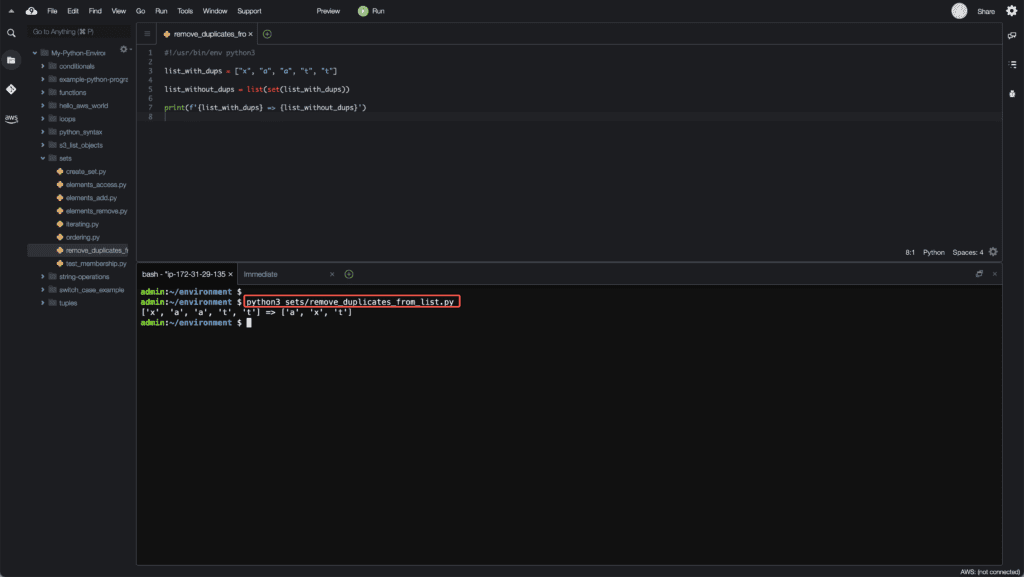
Set operations in Python
In this part of the article, we’ll review the fundamental operations through which sets can be combined and related to each other.
Union
The union operation on two sets produces a new set that contains all elements from both sets.

Here’s a code example:
set_1 = {1, 2, 3, 4, 5}
set_2 = {4, 5, 6, 7, 8}
result_set = set_1 | set_2
print(f'Result set: {result_set}')
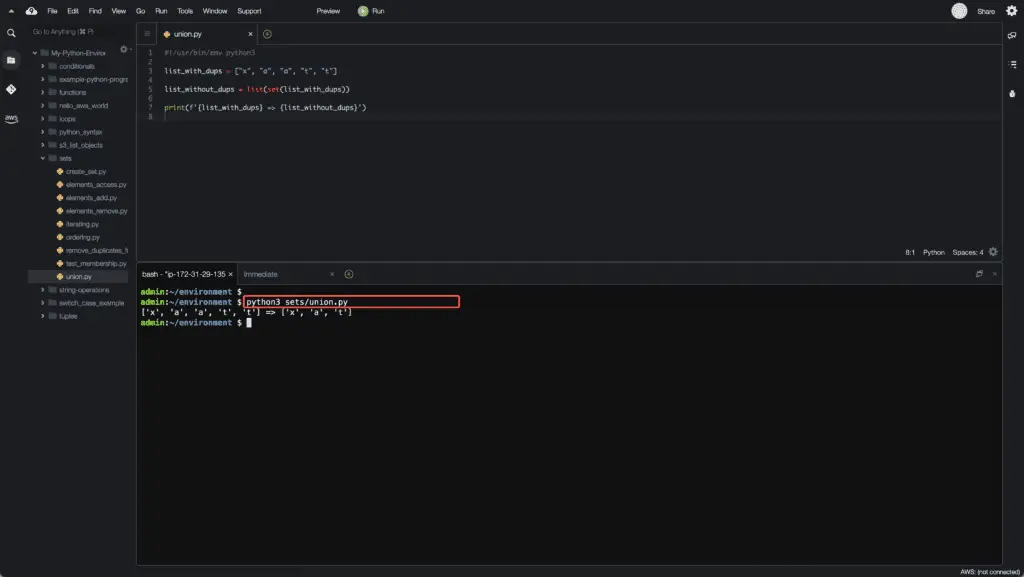
Intersection
The intersection operation on two sets produces a new set containing only common elements from both sets.
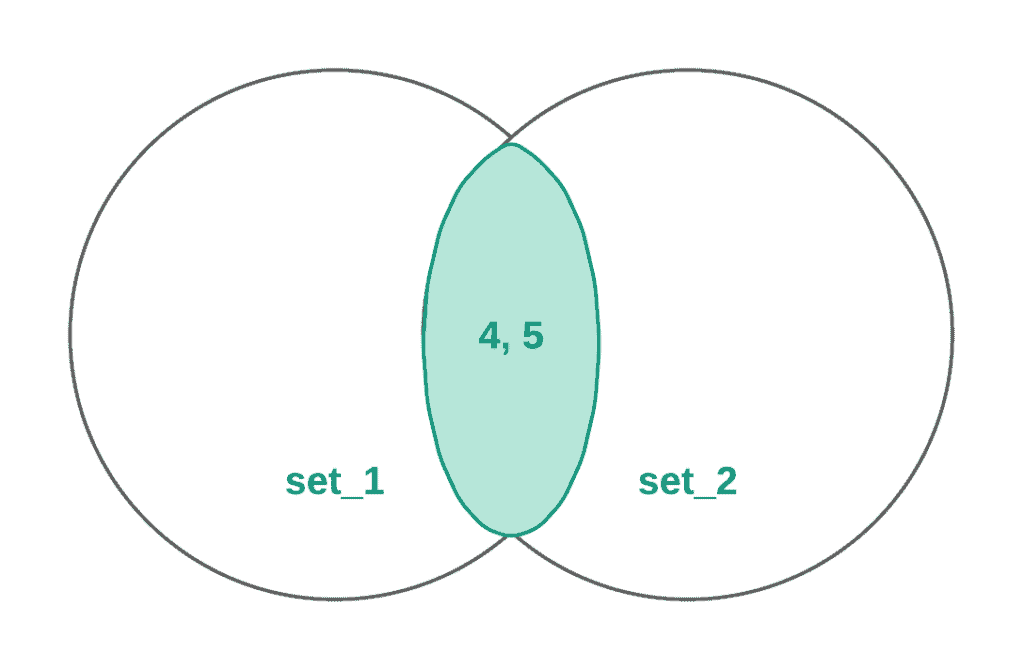
Here’s a code example:
set_1 = {1, 2, 3, 4, 5}
set_2 = {4, 5, 6, 7, 8}
result_set = set_1 & set_2
print(f'Result set: {result_set}')
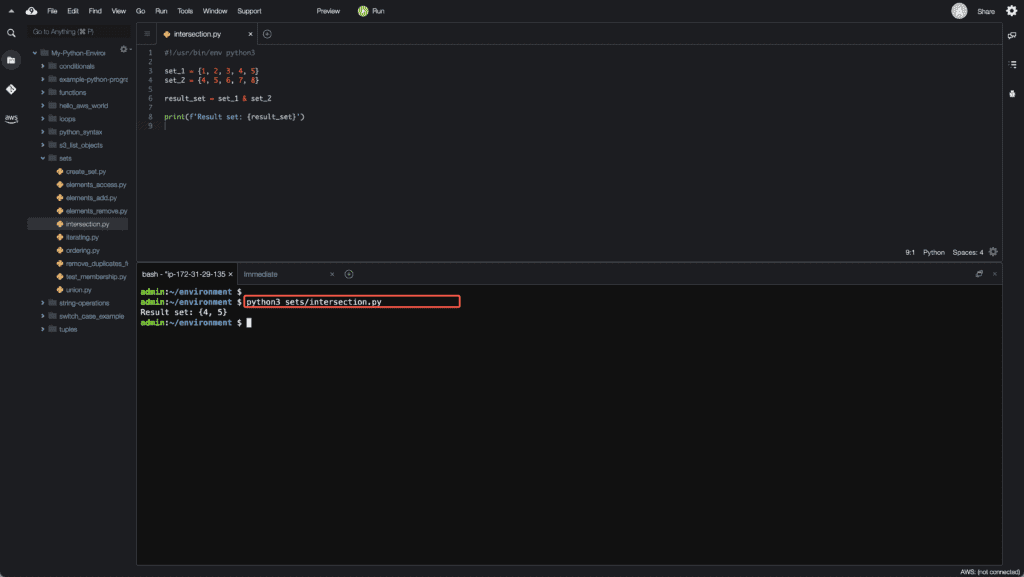
Difference
The difference operation between two sets produces a new set containing only the elements from the first set and none from the second set.
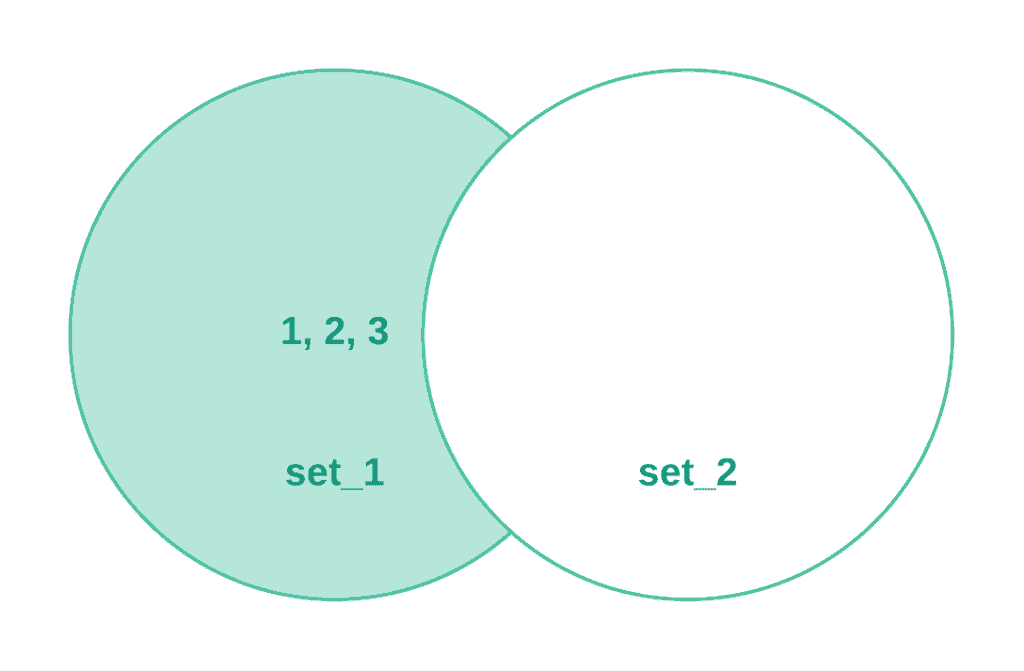
Here’s a code example:
set_1 = {1, 2, 3, 4, 5}
set_2 = {4, 5, 6, 7, 8}
result_set = set_1 - set_2
print(f'Result set: {result_set}')
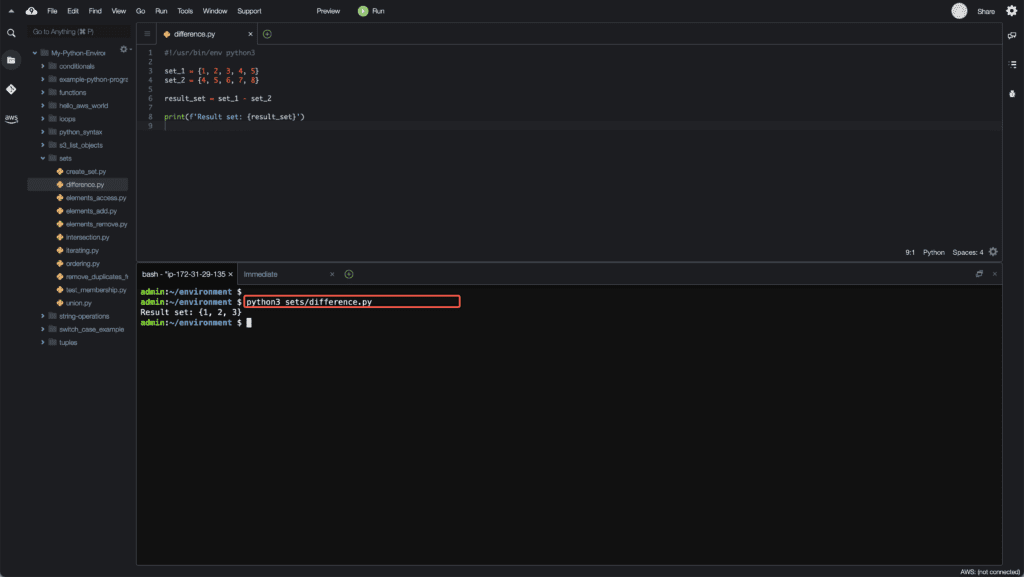
Symmetric difference
The symmetric difference operation between two sets produces a new set containing only the elements that belong to both sets at the same time.
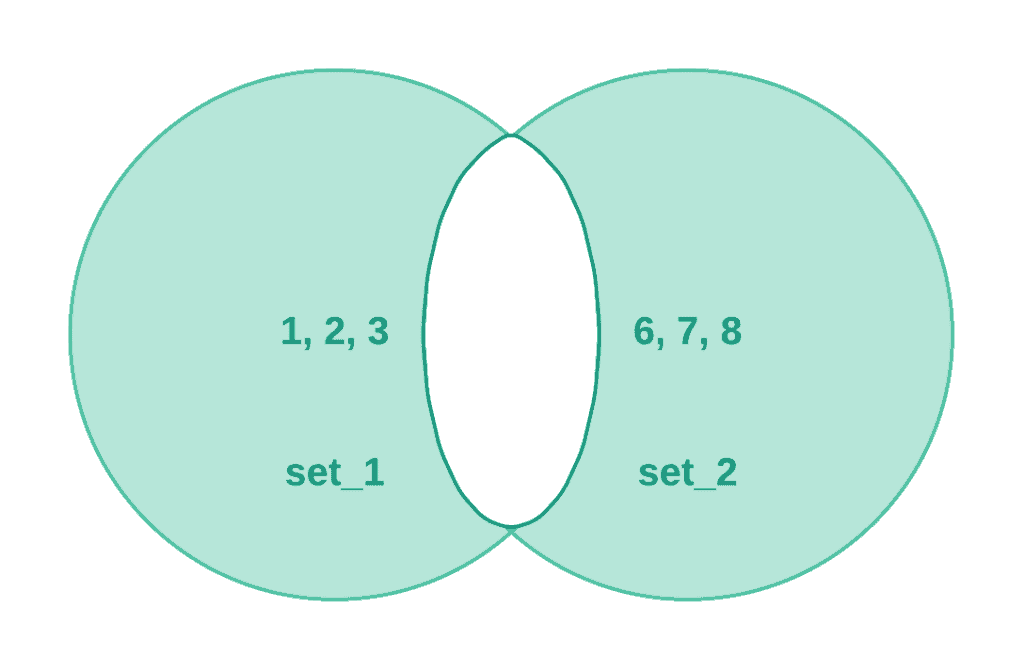
Here’s a code example:
set_1 = {1, 2, 3, 4, 5}
set_2 = {4, 5, 6, 7, 8}
result_set = set_1 ^ set_2
print(f'Result set: {result_set}')
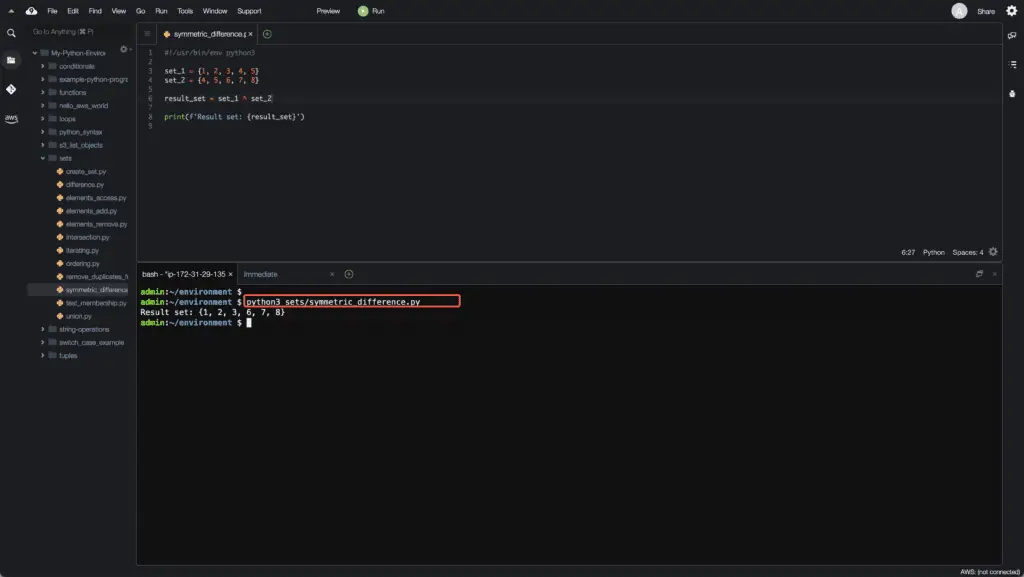
Comparing sets
You can check if one set is a subset or superset of another set. The result of the comparison operation is True
or False
:
set_1 = {1, 2, 3, 4, 5}
set_2 = {4, 5}
if set_2 <= set_1:
print(f'Set {set_1} is superset for set {set_2}')
if not set_2 >= set_1:
print(f'Set {set_1} is NOT superset for set {set_2}')
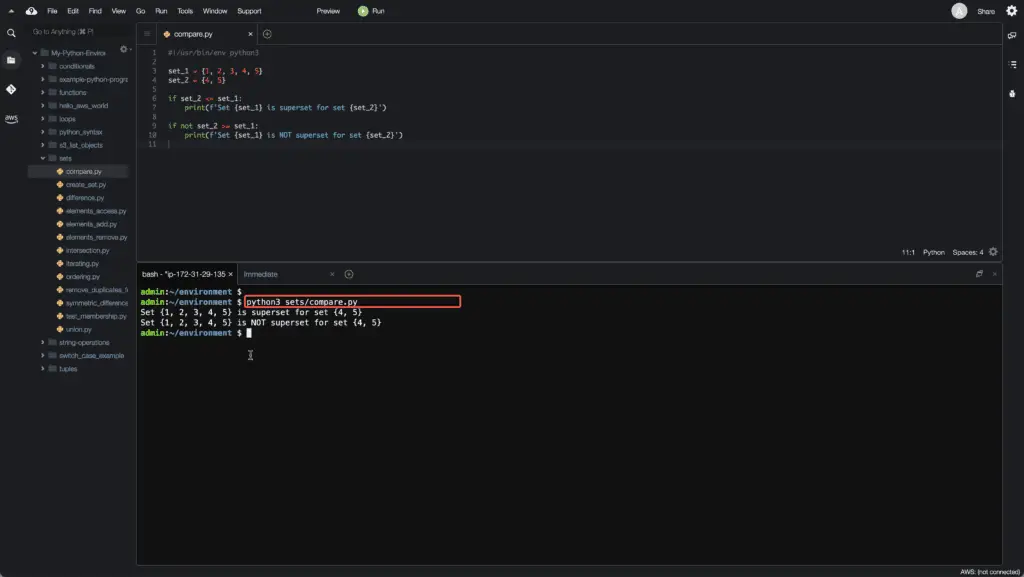
Frozenset in Python
The frozenset is a new class in Python that has the characteristics of a regular set, but its elements cannot be changed once frozenset has been initialized. In the same way, as tuples are immutable lists, frozensets are immutable sets. So, frozensets are useful in situations where you need to use a set, but a set itself should be immutable.
Frozensets are hashable and can be used as keys to dictionaries.
You can create a frozenset using the frozenset() class constructor:
set_1 = frozenset([1, 2, 3, 4, 5])
set_2 = frozenset([4, 5, 6, 7, 8])
Frozensets supports the same operations as regular sets:
# Union
result_set = set_1 | set_2
print(f'Result set: {result_set}')
# Intersection
result_set = set_1 & set_2
print(f'Result set: {result_set}')
# Difference
result_set = set_1 - set_2
print(f'Result set: {result_set}')
# Symmetric difference
result_set = set_1 ^ set_2
print(f'Result set: {result_set}')
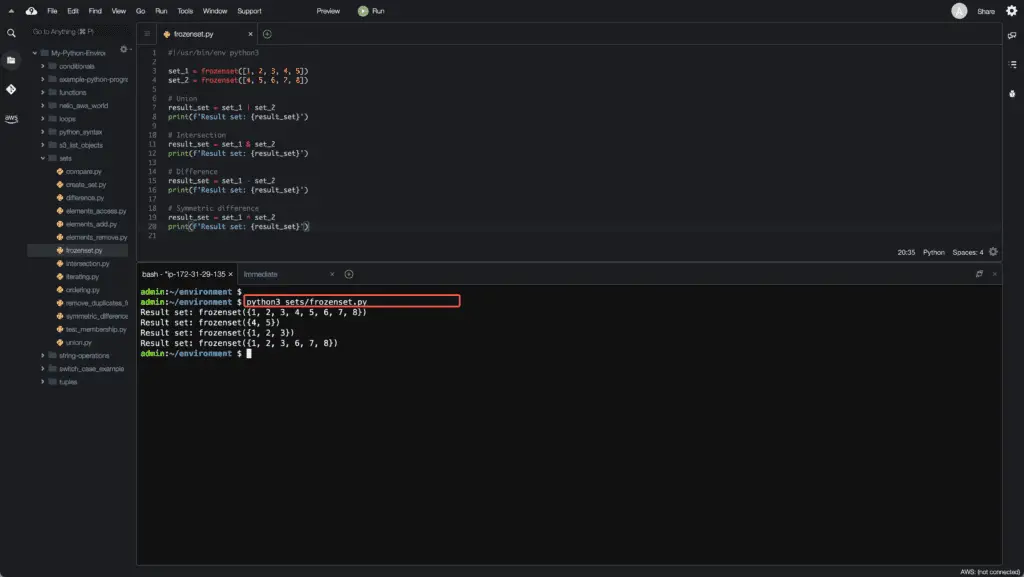
Summary
In this article, we’ve covered Python sets
, frozensets
and how to use them to get results of collections union, intersection, symmetric difference, etc.