In this course section, we’ll describe what major components every Python program consists of and cover best practices for properly structuring and formatting your Python programs.
Program example
Here’s an example of a simple Python program that copies files from one S3 bucket to another.
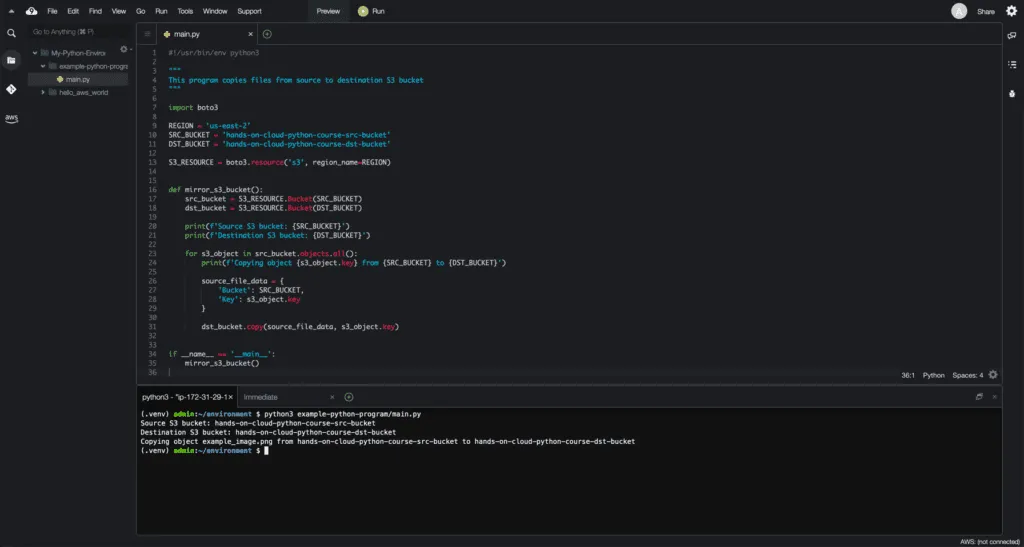
There’s no need to understand how this code works right now.
We’ll use this example to illustrate a typical Python program structure with the function:
#!/usr/bin/env python3
"""
This program copies objects from source to destination S3 bucket
"""
import boto3
REGION = 'us-east-2'
SRC_BUCKET = 'hands-on-cloud-python-course-src-bucket'
DST_BUCKET = 'hands-on-cloud-python-course-dst-bucket'
S3_RESOURCE = boto3.resource('s3', region_name=REGION)
def copy_objects():
src_bucket = S3_RESOURCE.Bucket(SRC_BUCKET)
dst_bucket = S3_RESOURCE.Bucket(DST_BUCKET)
print(f'Source S3 bucket: {SRC_BUCKET}')
print(f'Destination S3 bucket: {DST_BUCKET}')
for s3_object in src_bucket.objects.all():
print(f'Copying object: {s3_object.key}')
source_file_data = {
'Bucket': SRC_BUCKET,
'Key': s3_object.key
}
dst_bucket.copy(source_file_data, s3_object.key)
if __name__ == '__main__':
copy_objects()
Below we provided an explanation of all parts in more detail.
Shebang
#!/usr/bin/env python3
Line 1 of our program contains a shebang – a special syntax that allows Bash to understand which interpreter to run your program.
In our case, we’re telling Bash that our Python program needs to be executed using python3
interpreter (command).
It is a good practice to include shebang in your Python program code and allow program users to launch it without calling a Python interpreter first.
Program description
"""
This program copies objects from source to destination S3 bucket
"""
Lines 3-5 represent a multi-line comment used to describe the program’s purpose.
It is a good practice to provide a program description and leave comments on your program code to allow other developers to easily understand your code’s purpose and most important parts.
Reused code (modules)
import boto3
Line 7 imports a Python module called boto3.
This module contains a common Python code provided by Amazon Web Services, which allows Python developers to write software that uses AWS services.
In the Python world, modules are used to provide an ability to reuse the same code over and over again.
If you have a common code in your program, you can create a module and put your common code there.
Importing a module in your Python program allows you to access the code defined in the module.
Global variables
REGION = 'us-east-2'
SRC_BUCKET = 'hands-on-cloud-python-course-src-bucket'
DST_BUCKET = 'hands-on-cloud-python-course-dst-bucket'
S3_RESOURCE = boto3.resource('s3', region_name=REGION)
Lines 9-13 contain common global variables used in the entire Python program.
Those variables store information about AWS Region, source and destination S3 bucket names, and boto3 resource, which allows you to send API calls to the S3 service.
Function definitions
def copy_objects():
src_bucket = S3_RESOURCE.Bucket(SRC_BUCKET)
dst_bucket = S3_RESOURCE.Bucket(DST_BUCKET)
print(f'Source S3 bucket: {SRC_BUCKET}')
print(f'Destination S3 bucket: {DST_BUCKET}')
for s3_object in src_bucket.objects.all():
print(f'Copying object: {s3_object.key}')
source_file_data = {
'Bucket': SRC_BUCKET,
'Key': s3_object.key
}
dst_bucket.copy(source_file_data, s3_object.key)
Lines 16-31 contain a Python function definition.
In Python, functions are used to define reusable pieces of code, which can often be used in programs.
This function is responsible for copying all S3 objects from the source to the destination bucket.
Program entry point (main)
if __name__ == '__main__':
copy_objects()
Lines 34-35 allow us to define a Python program entry point.
Python interpreter reads the code of your program starting from the first line and tries to execute it immediately line by line.
In comparison with C, C++, or Java, for example, there is no main()
function in Python, so we have to use this special syntax to tell Python that it should start our program from running copy_objects()
function “first”.
Strictly speaking, this is not the first function that Python will execute in our program, but for simplicity of explanation, we’ll stick with this logic.