AWS CLI DynamoDB – Useful Commands Examples
Amazon DynamoDB is a fully managed NoSQL database service provided by Amazon Web Services (AWS). It provides fast and predictable performance with seamless scalability. It supports document and key-value store models and provides a simple API for storing and retrieving data. It also has fine-grained data access controls to ensure that the data is securely stored and managed.
The AWS Command Line Interface (CLI) is a unified tool to manage your AWS services. With just one tool to download and configure, you can control multiple AWS services from the command line and automate them through scripts.
This article provides various examples of using AWS CLI DynamoDB commands that you can use during your day-to-day AWS activities.
Table of contents
AWS CLI Installation
You can install AWS CLI on Windows, macOS, and Linux. In addition to that, Amazon Linux AMI already contains AWS CLI as a part of the OS distribution, so you don’t have to install it manually.
Windows
For modern Windows distributions, we recommend you to use Chocolatey package manager to install AWS CLI:
# AWS CLI
choco install awscli
# Session Manager plugin
choco install awscli-session-manager
# AWS-Shell
choco install python
choco install pip
pip install aws-shell
macOS
To install AWS CLI on macOS, we recommend you to use brew package manager:
# AWS CLI
brew install awscli
# Session Manager plugin
brew install --cask session-manager-plugin
# AWS-Shell
pip install aws-shell
Linux
Depending on your Linux distribution, the installation steps are different.
CentOS, Fedora, RHEL
For YUM-based distributions (CentOS, Fedora, RHEL), you can use the following installation steps:
# AWS CLI
sudo yum update
sudo yum install wget -y
wget https://dl.fedoraproject.org/pub/epel/epel-release-latest-7.noarch.rpm
sudo yum install epel-release-latest-7.noarch.rpm
sudo yum -y install python-pip
sudo pip install awscli
# Session Manager plugin
curl "https://s3.amazonaws.com/session-manager-downloads/plugin/latest/linux_64bit/session-manager-plugin.rpm" \
-o "session-manager-plugin.rpm"
sudo yum install -y session-manager-plugin.rpm
# AWS-Shell
pip install aws-shell
Debian, Ubuntu
For APT-based distributions (Debian, Ubuntu), you can use slightly different installation steps:
# AWS CLI
sudo apt-get install python-pip
sudo pip install awscli
# Session Manager plugin
curl "https://s3.amazonaws.com/session-manager-downloads/plugin/latest/ubuntu_64bit/session-manager-plugin.deb" \
-o "session-manager-plugin.deb"
sudo dpkg -i session-manager-plugin.deb
# AWS-Shell
pip install aws-shell
Other Linux distributions
For other Linux distributions, you can use manual AWS CLI installation steps.
AWS CLI DynamoDB Commands
Usually, you’re using AWS CLI commands to manage DynamoDB when you need to automate DynamoDB operations using scripts or in your CICD automation pipeline. For example, you can configure the Jenkins pipeline to execute AWS CLI commands for any AWS account in your environment.
Managing DynamoDB tables
This article section will cover the most common examples of using AWS CLI commands to manage DynamoDB tables.
Create table
To create a DynamoDB table using AWS CLI, you need to use the aws dynamodb create-table command, which expects at least four arguments:
--table-name
– defines the DynamoDB table name--attribute-definitions
– a JSON-like definition of all table attributes and their types that you’re going to use in the DynamoDB table and indexes--key-schema
– specifies the attributes that make up the primary key for a table or an index (partition or sort keys)--billing-mode
– Controls how you are charged for read and write throughput and how you manage capacity. Allowed values:PROVISIONED
andPAY_PER_REQUEST
.
Let’s create a simple AWS demo DynamoDB table to store demo product catalog items.
Table name | Primary Key |
---|---|
ProductCatalog | Partition key: Id (Number) |
To create this DynamoDB table, use the following AWS CLI command:
aws dynamodb create-table \
--table-name ProductCatalog \
--attribute-definitions AttributeName=id,AttributeType=N \
--key-schema AttributeName=id,KeyType=HASH \
--billing-mode PAY_PER_REQUEST
Note: it is recommended to usePAY_PER_REQUEST
billing mode only for brand new tables when you don’t know the table’s scaling capacity upfront.
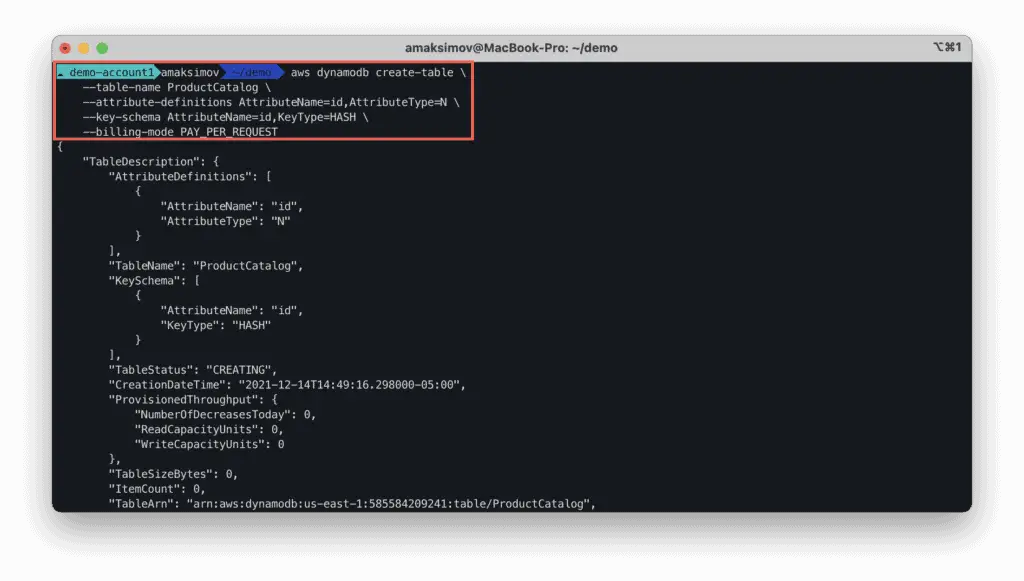
As a result, you’ll get the following DynamoDB table in your AWS console:
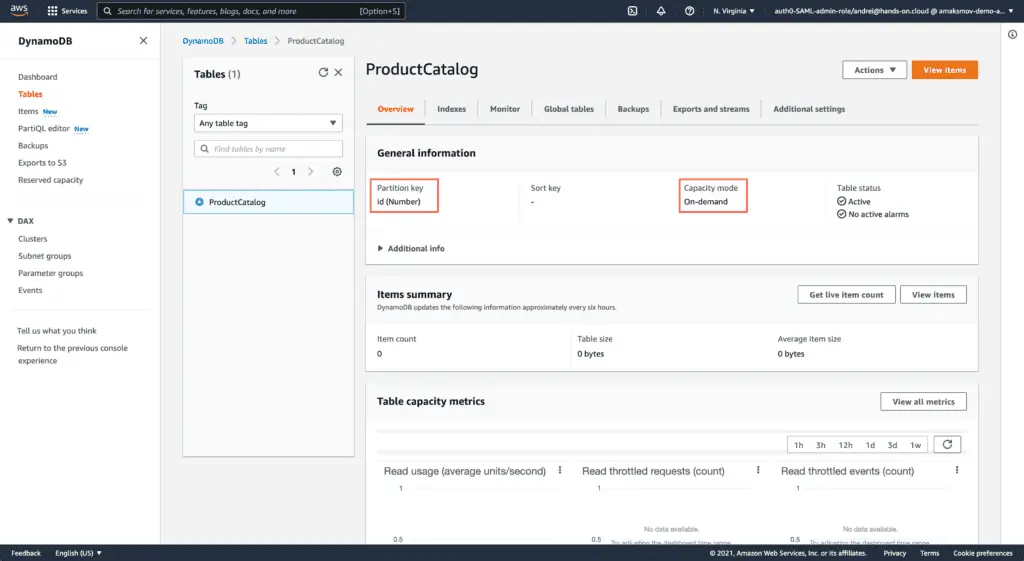
Now, let’s create a DynamoDB table for storing users’ accounts with the composite index.
Table name | Primary Key |
---|---|
MyWebsiteUsers | Partition key: user_id (String)Sort key: created_at (String) |
In the example below, we’ll use another billing mode where we’ll specify the exact amount of the read and write capacity units for the table:
aws dynamodb create-table \
--table-name MyWebsiteUsers \
--attribute-definitions AttributeName=user_id,AttributeType=S AttributeName=created_at,AttributeType=S \
--key-schema AttributeName=user_id,KeyType=HASH AttributeName=created_at,KeyType=RANGE \
--billing-mode PROVISIONED \
--provisioned-throughput ReadCapacityUnits=5,WriteCapacityUnits=5
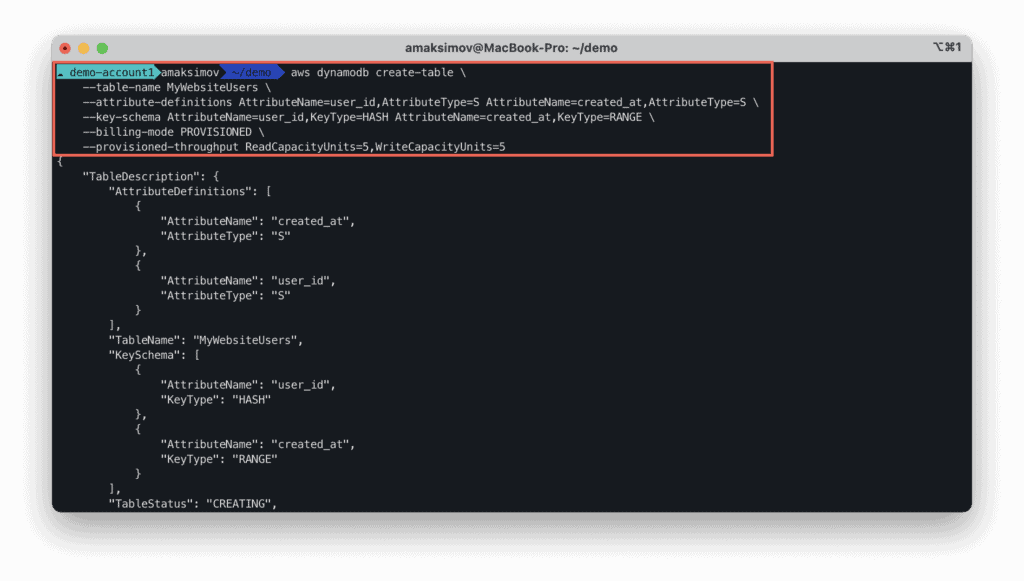
As a result, you’ll get the following DynamoDB table in your AWS console:
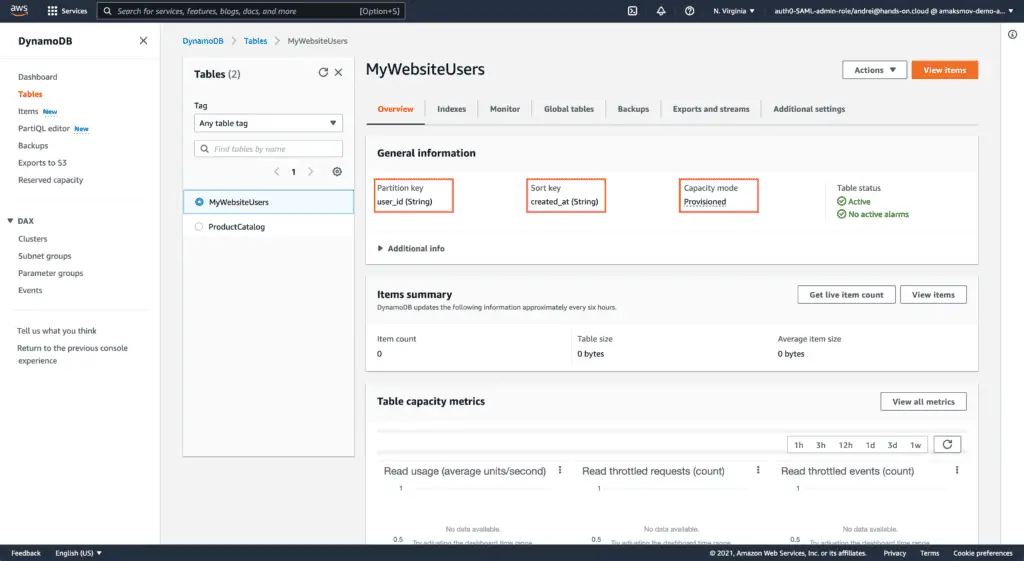
You can specify arguments’ values in a JSON syntax too. The following command will produce the same result as we got from the previous command execution:
aws dynamodb create-table \
--table-name MyWebsiteUsers \
--attribute-definitions '[{"AttributeName":"user_id", "AttributeType":"S"}, {"AttributeName":"created_at", "AttributeType":"S"}]' \
--key-schema '[{"AttributeName":"user_id", "KeyType":"HASH"}, {"AttributeName":"created_at", "KeyType":"RANGE"}]' \
--billing-mode PROVISIONED \
--provisioned-throughput '{"ReadCapacityUnits": 5, "WriteCapacityUnits": 5}'
List tables
To list DynamoDB tables using AWS CLI, you need to use the aws dynamodb list-tables command:
aws dynamodb list-tables
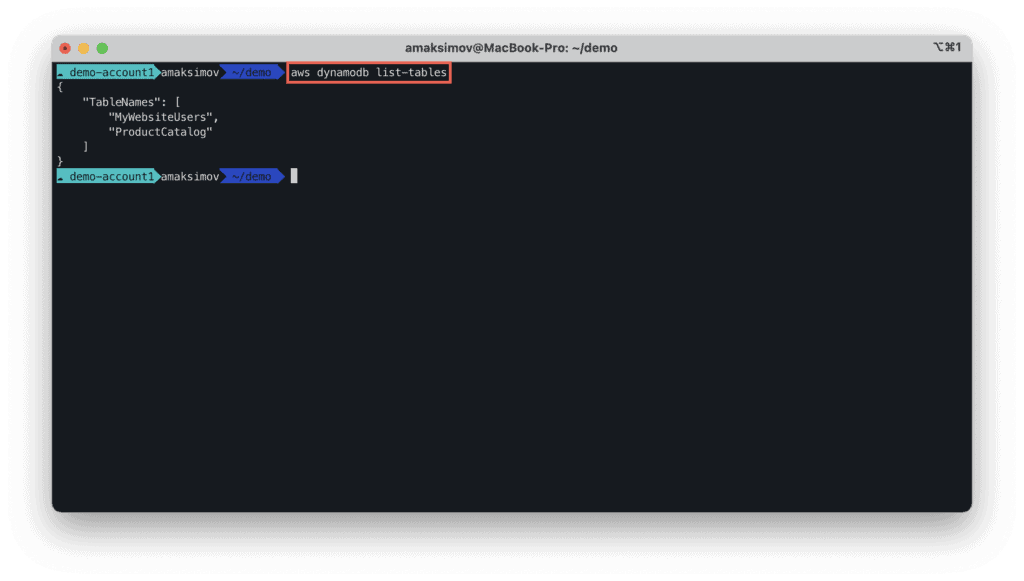
Describe table
To describe the DynamoDB table using AWS CLI, you need to use theaws dynamodb describe-table command with the required --table-name
argument. This command will return the table key schema, capacity mode, provisioned WCU and RCU, amount of items stored in the table, table status, consumed storage space, and much more.
aws dynamodb describe-table \
--table-name MyWebsiteUsers
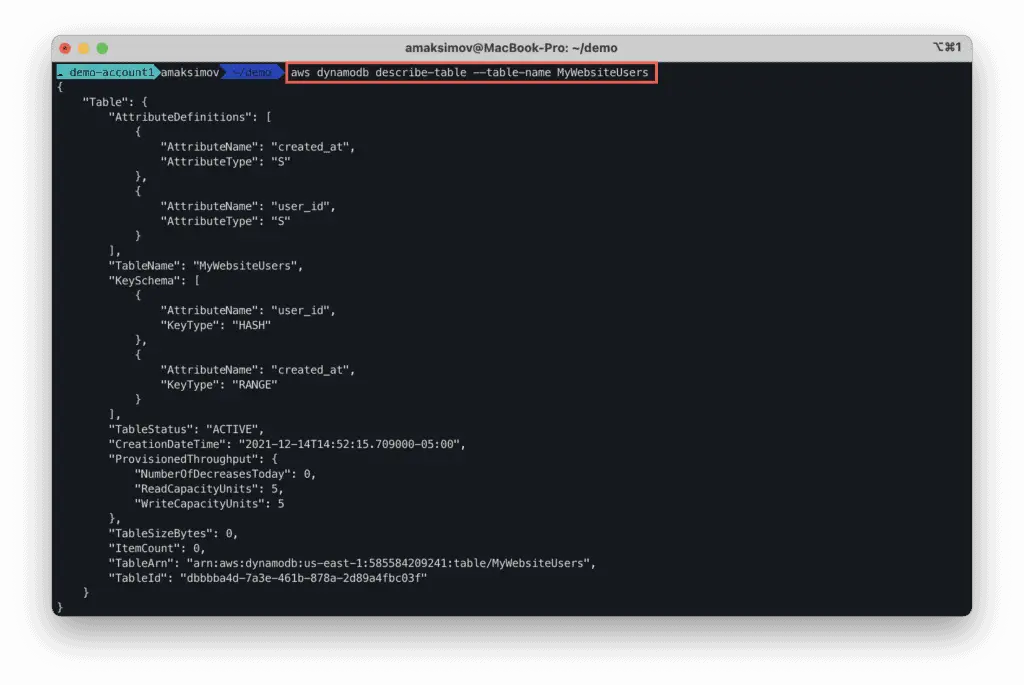
Delete table
To delete DynamoDB Table using AWS CLI, you need to use the aws dynamodb delete-table command with the required --table-name
argument:
aws dynamodb delete-table \
--table-name ProductCatalog
Note: this command removes the table, data, and related DynamoDB Streams.
Note: it might take a while to delete your table and its data; meanwhile, the table will be in the DELETING
state.
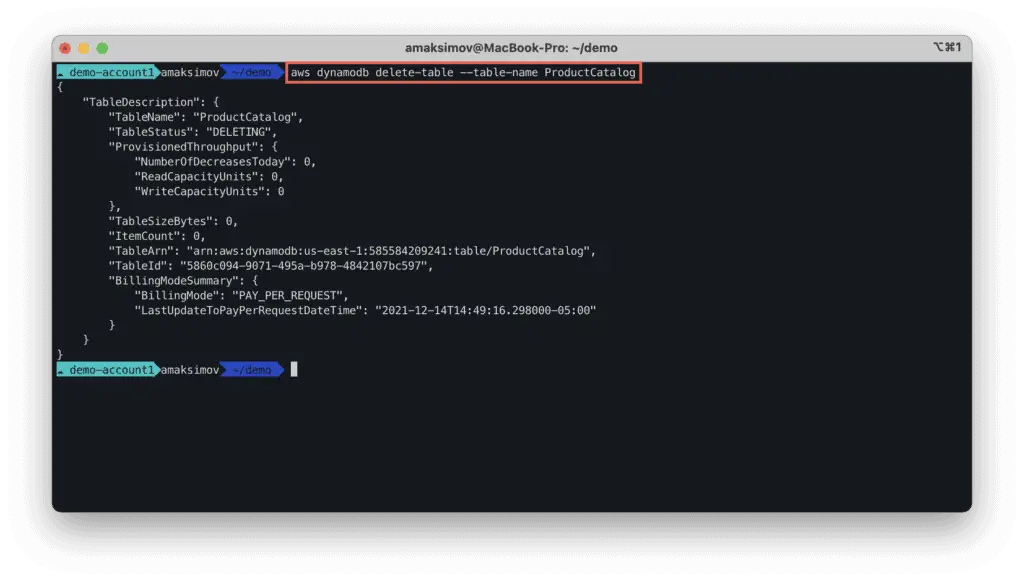
Backup table
To backup the DynamoDB table using AWS CLI, you need to use the aws dynamodb create-backup command with two required arguments:
--table-name - specifies the DynamoDB table to backup
--backup-name
- specifies the name of the to backup
aws dynamodb create-backup \
--table-name ProductCatalog \
--backup-name ProductCatalog-backup-$(date +"%m-%d-%Y")
Note: Check additional
date
command format options here.
The command above will request a full, on-demand backup of your DynamoDB table. The backup will contain all the data, table key structure, LSIs and GSIs, and information about streams and provisioned read and write capacity.
DynamoDB backup is an asynchronous operation and does not consume any of the provisioned throughputs.

List table backups
To list DynamoDB table backups using AWS CLI, you need to use the aws dynamodb list-backups command with
--table-name
argument.
The following command will list all on-demand backups initiated by the user or DynamoDB itself:
aws dynamodb list-backups \
--table-name ProductCatalog
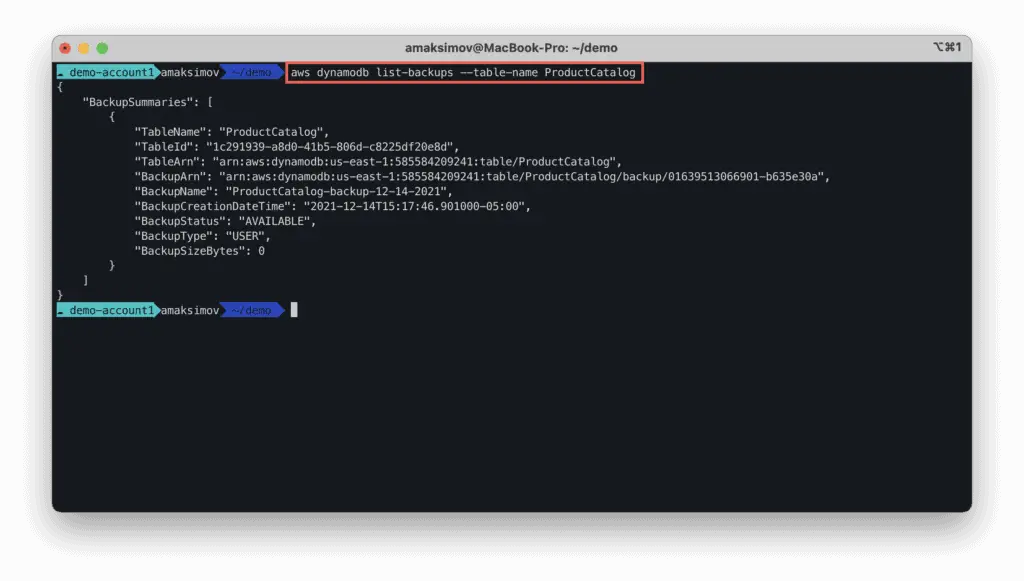
To specify the type of backup, you need to use the
--backup-type
argument, which accepts the following values:
USER
- On-demand backup created by you.SYSTEM
- On-demand backup automatically created by DynamoDB.ALL
- All types of on-demand backups (USER
andSYSTEM
).
In addition to that, you can use the
--time-range-lower-bound
and --time-range-upper-bound
arguments to list backups between two dates (in epoch format). It is convenient to use the date
command to convert date time to the timestamp:
# Linux
aws dynamodb list-backups \
--table-name ProductCatalog \
--time-range-lower-bound $(date -d '12/14/2021 14:30:00' +"%s") \
--time-range-upper-bound $(date -d '12/14/2021 20:00:00' +"%s") \
--backup-type USER
# macOS
aws dynamodb list-backups \
--table-name ProductCatalog \
--time-range-lower-bound $(date -jf "%Y-%m-%d %H:%M:%S" "2021-12-14 14:30:00" +%s) \
--time-range-upper-bound $(date -jf "%Y-%m-%d %H:%M:%S" "2021-12-14 20:00:00" +%s) \
--backup-type USER

Describe table backup
To describe the DynamoDB table backup using AWS CLI, you need to use the aws dynamodb describe-backup command with
--backup-arn
argument:
aws dynamodb describe-backup \
--backup-arn arn:aws:dynamodb:us-east-1:585584209241:table/ProductCatalog/backup/01639513066901-b635e30a
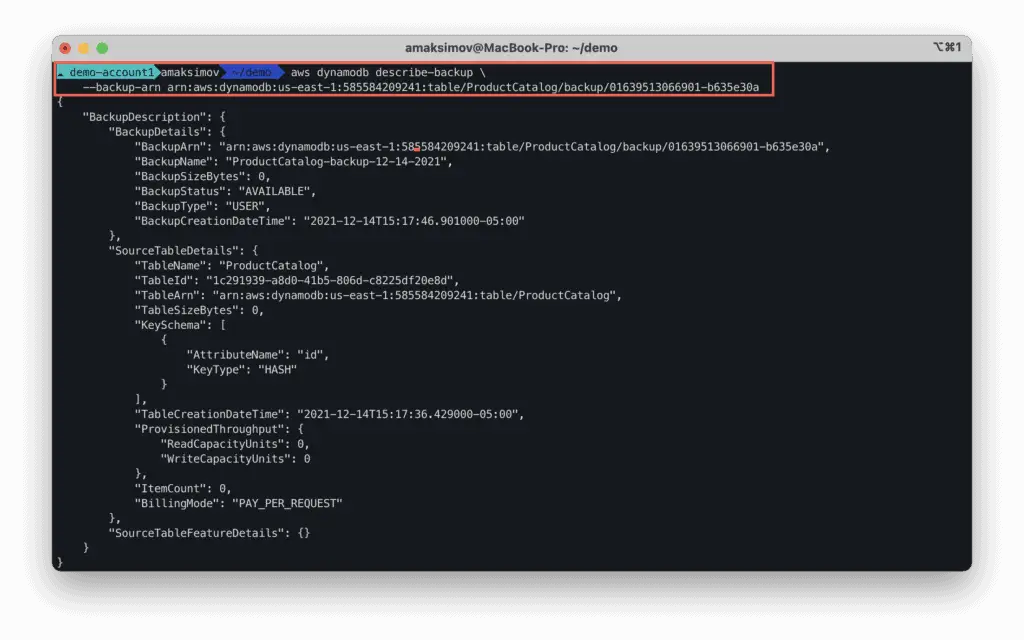
Restore table from backup
To restore the DynamoDB table from the backup using AWS CLI, you need to use the aws dynamodb restore-table-from-backup command, which requires the
--target-table-name
and --backup-arn
arguments:
aws dynamodb restore-table-from-backup \
--target-table-name ProductCatalog-restored \
--backup-arn arn:aws:dynamodb:us-east-1:585584209241:table/ProductCatalog/backup/01639513066901-b635e30a
DynamoDB the restore table from the backup operation does not set up table tags, autoscaling settings, streams, TTL, and CloudWatch Metrics.
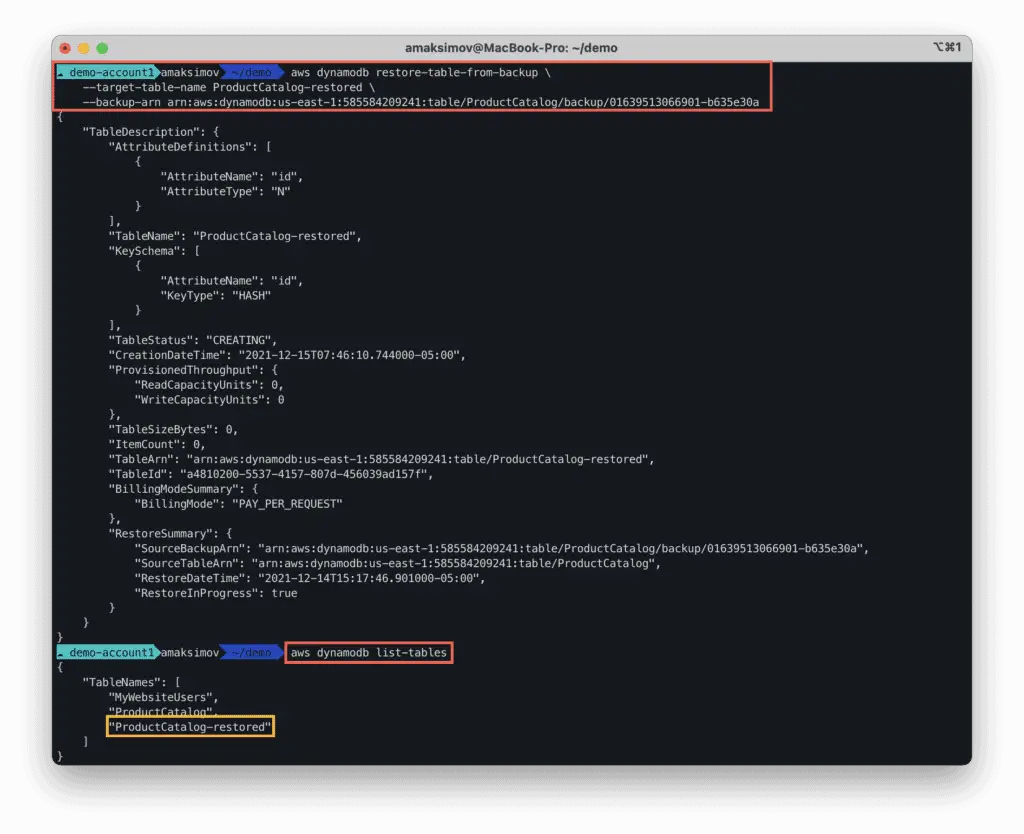
Managing DynamoDB table items
This section of the article will cover the most common examples of using AWS CLI commands to manage DynamoDB items.
Put item
To insert (put) an item to theDynamoDB table using AWS CLI, you need to use the aws dynamodb put-item command.
You can provide the item information in the form of JSON data structure in the terminal:
aws dynamodb put-item \
--table-name ProductCatalog \
--item '{"id": {"N": "100"}, "Title": {"S": "My-Product"}}'
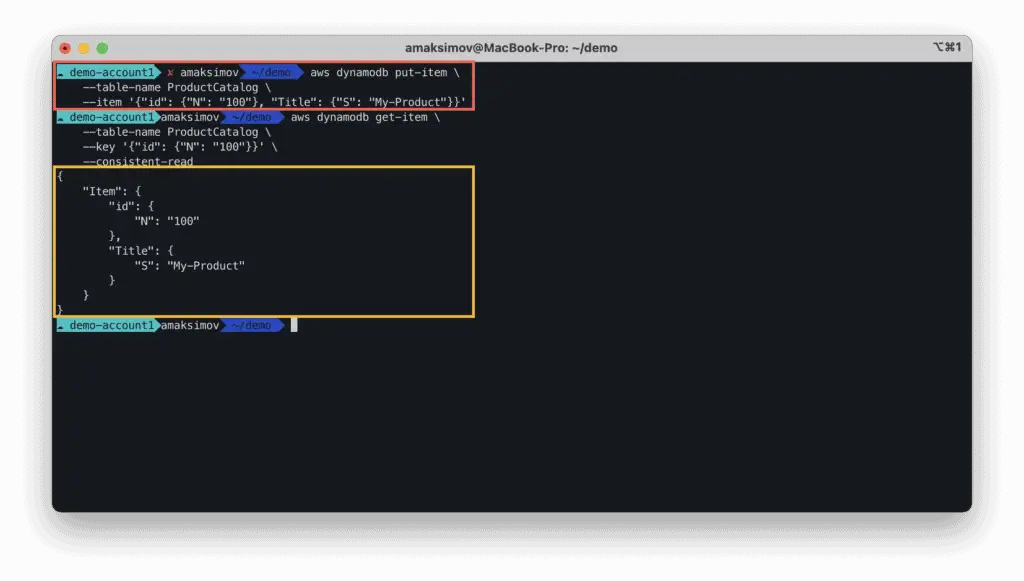
Alternatively, you can put the same JSON content of the DynamoDB item to the file (for example,
item.json
):
{"id": {"N": "100"}, "Title": {"S": "My-Product"}}
And insert it from the JSON file:
aws dynamodb put-item \
--table-name ProductCatalog \
--item file://item.json
Note: if the new item has the same primary key as one of the already existing items, an existing item with the same primary key will be completely overridden by the newly provided item.
Put multiple items (batch write item)
To write multiple items to DynamoDB at once using AWS CLI, you need to use the aws dynamodb batch-write-item command and provide it a list of items for
PutRequest
or DeleteRequest
operations in the JSON format using --request-items
argument.
The best way of doing this, is to create a JSON file (
request.json
) with the requested changes:
{
"ProductCatalog": [
{
"PutRequest": {
"Item": {
"id": {"N": "100"},
"Title": {"S": "My-Product"}
}
}
},
{
"PutRequest": {
"Item": {
"id": {"N": "101"},
"Title": {"S": "My-Product-1"}
}
}
},
{
"PutRequest": {
"Item": {
"id": {"N": "102"},
"Title": {"S": "My-Product-2"}
}
}
}
]
}
Now, you can run the following command to apply changes:
aws dynamodb batch-write-item \
--request-items file://request.json
Here's an execution output:
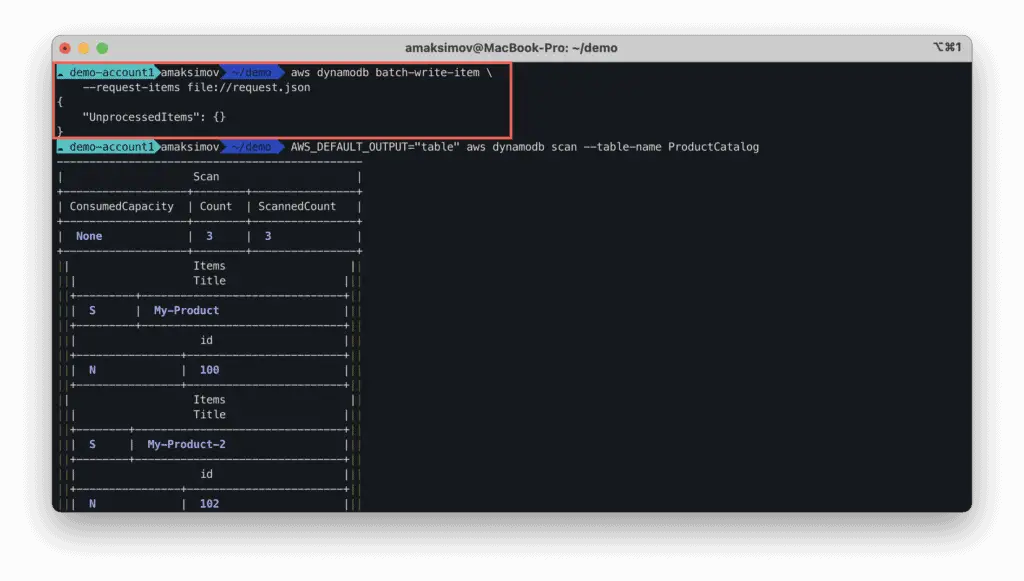
Get all items (Scan operation)
To get all items from theDynamoDB table using AWS CLI, you need to use the aws dynamodb scan command with at least
--table-name
argument provided:
aws dynamodb scan \
--table-name ProductCatalog
Here's an execution output:
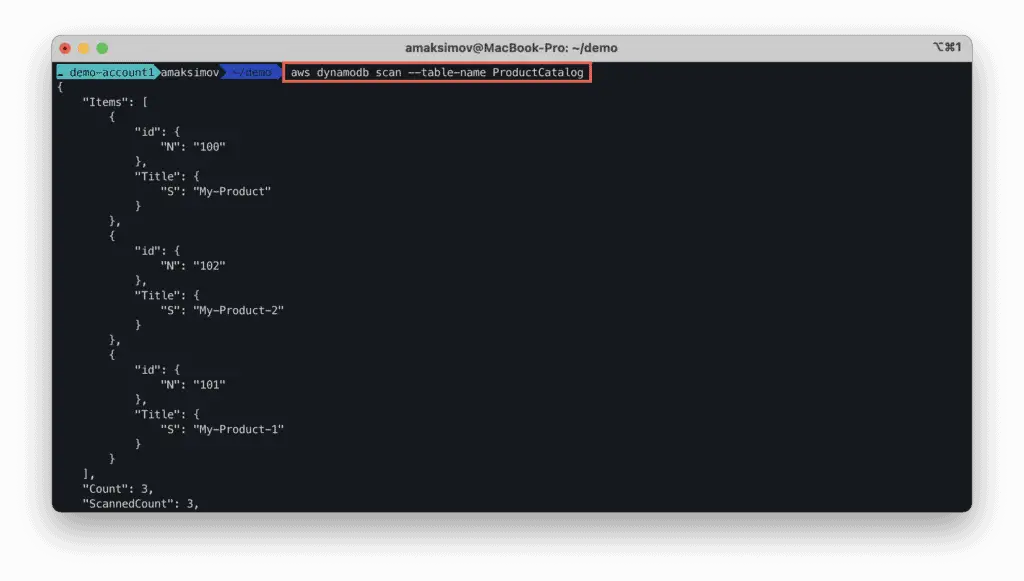
Note: you can use the
--starting-token
argument to query the next chunk of scanned data if your request is returning more data than specified.
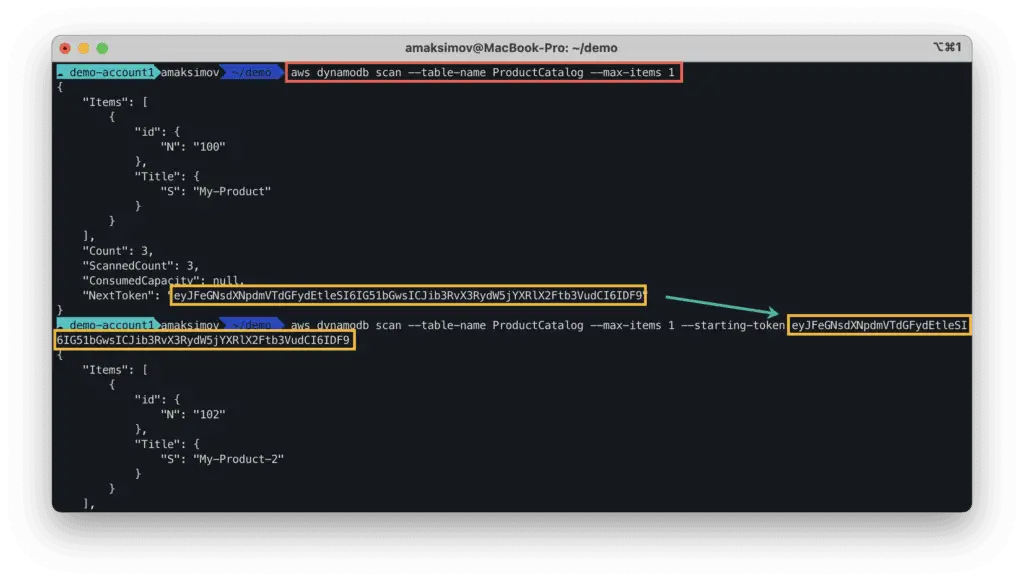
Get single item
To get a single item from DynamoDB using AWS CLI, you need to use the aws dynamodb get-item command with the
--key
argument:
aws dynamodb get-item \
--table-name ProductCatalog \
--key '{"id": {"N": "102"}}' \
--consistent-read
Note: you can use the optional
--consistent-read
argument to do a consistent read from the table.
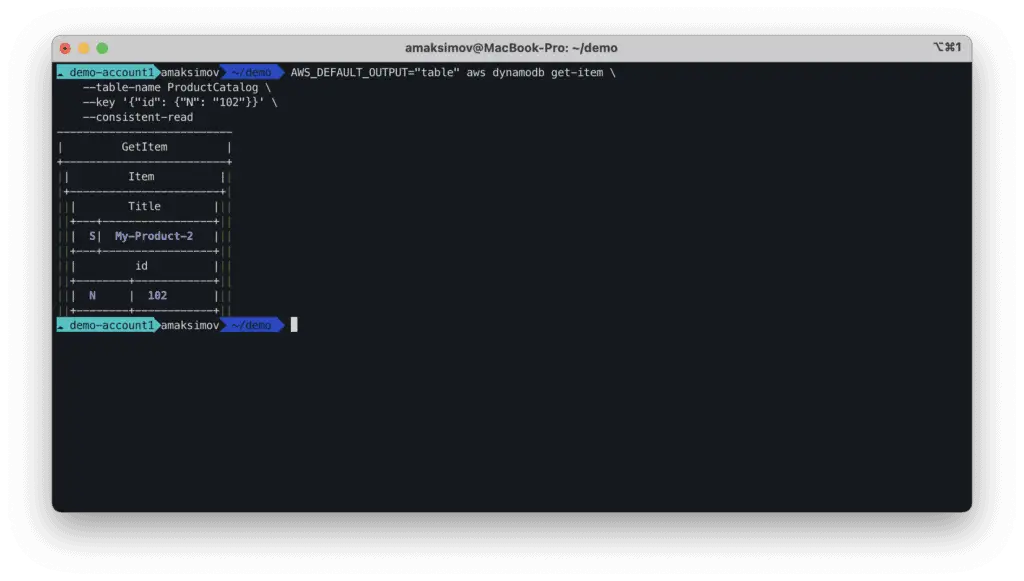
Delete item
To delete an item from DynamoDB using AWS CLI, you need to use aws dynamodb delete-item command with the required
--key
argument.
aws dynamodb delete-item \
--table-name NameOfTheTable \
--key '{"id": {"N": "102"}}'
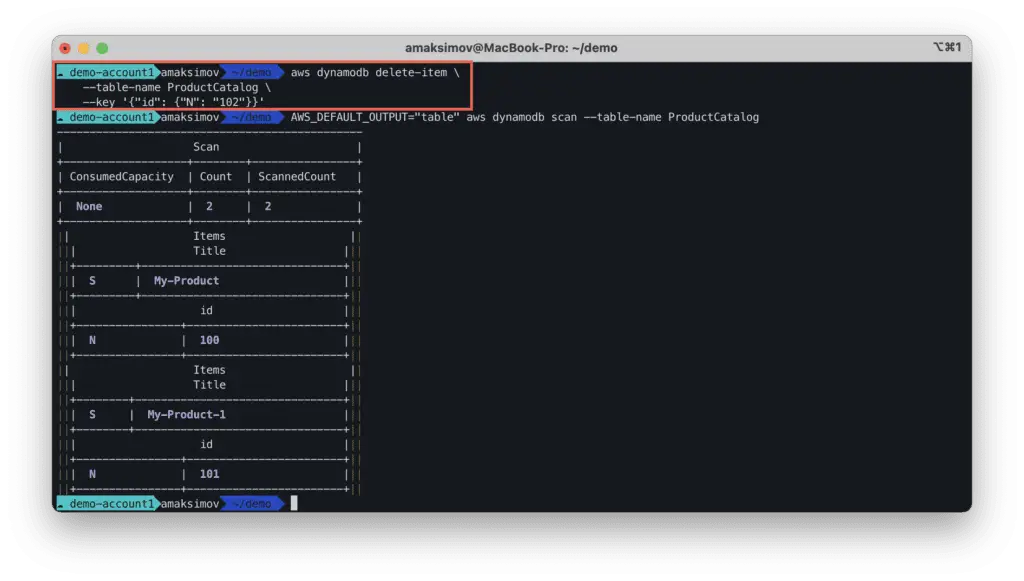
You can use the
--condition-expression
and --expression-attribute-values
arguments to delete items based on conditions. For more information, check out Condition Expressions documentation.
aws dynamodb delete-item \
--table-name ProductCatalog \
--key '{"id": {"N": "101"}}' \
--condition-expression 'attribute_not_exists(Title)'
Note: if thecondition expression is not satisfied, you'll get the following error message:
An error occurred (ConditionalCheckFailedException) when calling the DeleteItem operation: The conditional request failed
.
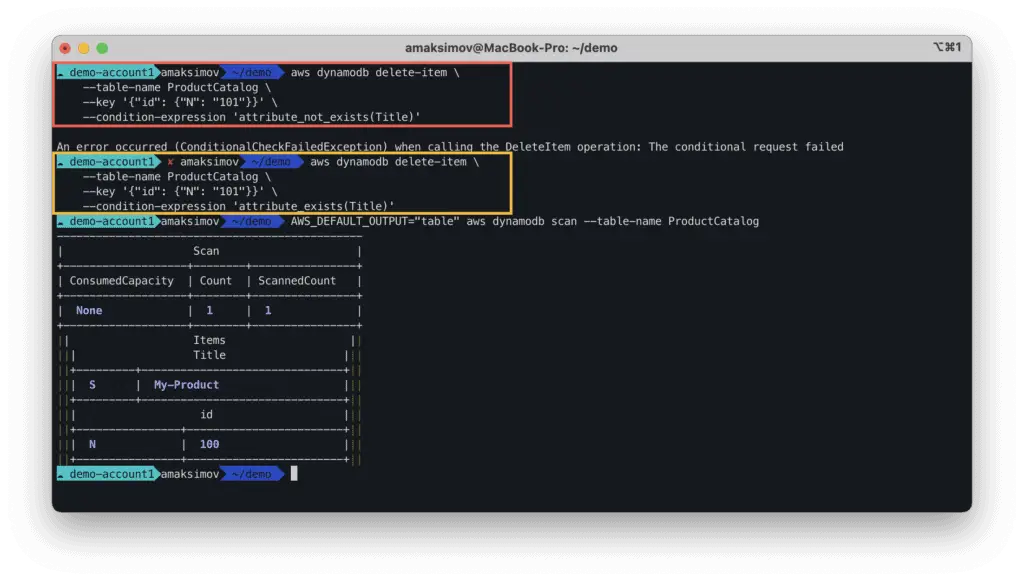
In our example, the first command failed, because the DynamoDB item had an existing
Title
attribute.
Query set of items
To query items from DynamoDB using AWS CLI, you need to use the aws dynamodb query command. You can query any DynamoDB table by the primary or secondary indexes:
aws dynamodb query \
--table-name ProductCatalog \
--key-condition-expression "id = :id" \
--expression-attribute-values '{":id": {"N": "101"}}'
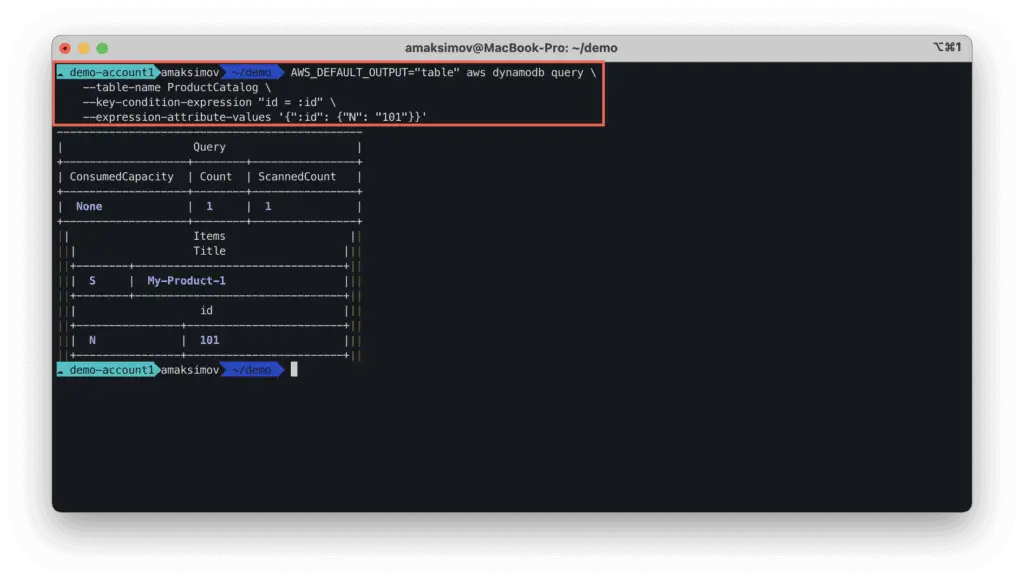
A couple of notes:
If you want to narrow down the query results on a non-index attribute, you can combine it with
--filter-expression
argument which acts in the same way as--condition-expression
argument of theaws dynamodb delete-item
commandThe
--filter-expression
argument is applied after the items have already been read and the process of filtering does not reduce consumed read capacity units
Update item
To update the DynamoDB item using AWS CLI, you need to use the aws dynamodb update-item command.
aws dynamodb update-item \
--table-name ProductCatalog \
--key '{"id":{"N":"101"}}' \
--update-expression "SET Title = :t" \
--expression-attribute-values '{":t": { "S": "Updated-Title" }}' \
--return-values ALL_NEW
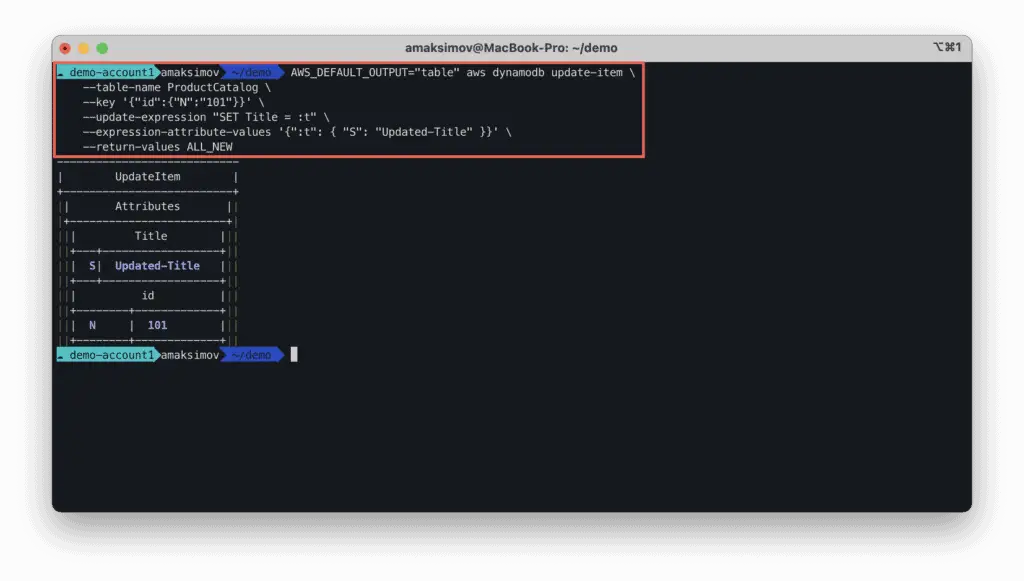
If you'd like to add additional attributes during the update operation, you need to specify them too:
aws dynamodb update-item \
--table-name ProductCatalog \
--key '{"id":{"N":"101"}}' \
--update-expression "SET Title = :t, Description = :d" \
--expression-attribute-values '{":t": { "S": "Updated-Title" }, ":d": {"S": "My description"}}' \
--return-values ALL_NEW
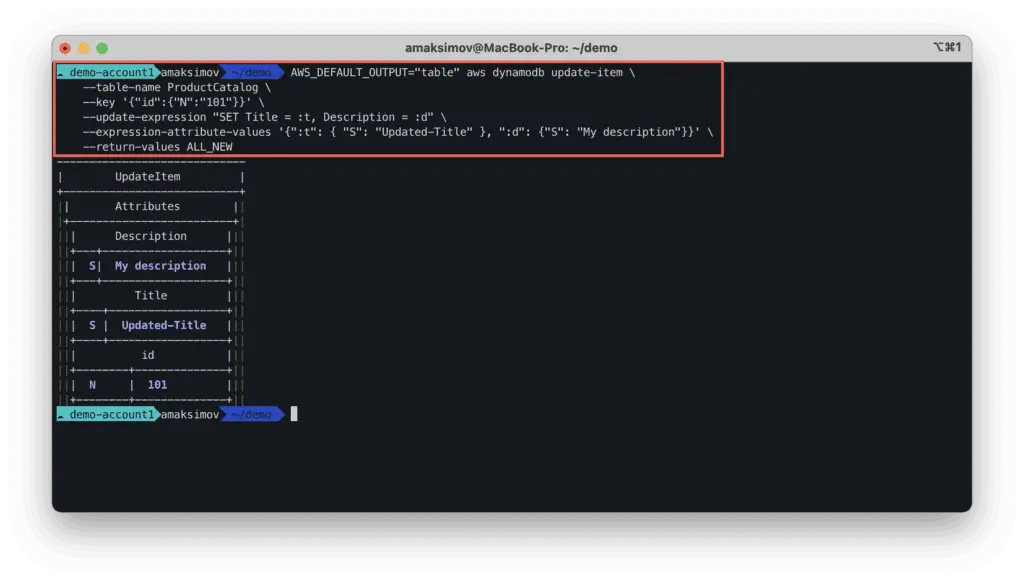
Increment item attribute
To update the DynamoDB item using AWS CLI, you need to use the aws dynamodb update-item command, but instead of using
update-expression
and expression-attribute-values
use the attribute-updates
shorthand:
aws dynamodb update-item \
--table-name ProductCatalog \
--key '{"id":{"N":"102"}}' \
--attribute-updates '{"amount_of_items": {"Value": {"N": "1"},"Action": "ADD"}}' \
--return-values ALL_NEW
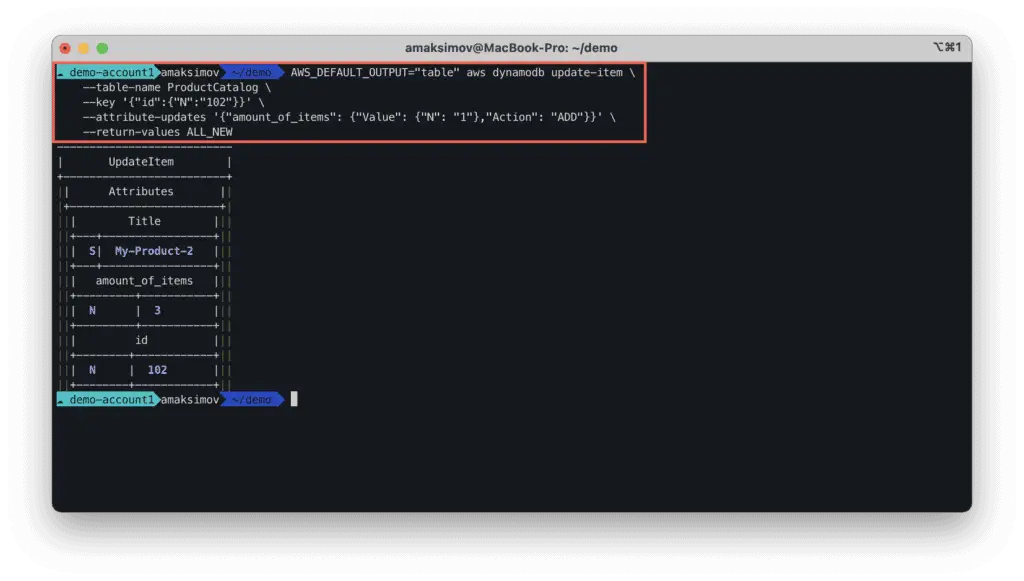
Summary
In this article, we've covered how to use AWS CLI to manage Amazon DynamoDB with many hands-on examples you can use during your day-to-day AWS activities.