Python Tuples – Complete Tutorial
A Python tuple is a collection of ordered and immutable objects. Python tuples are sequences, just like lists. The differences between tuples and lists are that the tuples cannot be changed (they are immutable Python objects), and they are faster and memory-efficient in compression with lists. Tuples are commonly used in parallel processing, Big Data, Machine Learning, and relational DB data management tasks. This tutorial will cover the tuples in detail and describe their purpose by providing examples to demonstrate how you can use them in real life.
Tuples
Python tuples, while useful for quick and dirty data composition and in many cases, should be replaced with named tuples, dataclasses, or regular classes.
Tuple vs. List
A Python tuple is a data structure in Python programming language similar to a list (a comma-separated list of values). The main difference between tuples and lists is that tuples are immutable, meaning they cannot be changed. Tuples are created by placing items inside parentheses, separated by commas. Items in a tuple can be accessed using indexing, just like a list. However, because tuples are immutable, attempting to modify a tuple will result in an error. Both tuples and lists can contain duplicate values. Tuples are often used to store data that should not be changed, such as the days of the week or the months of the year.
Both tuples and lists can contain nested data structures. For example, the tuple could have a nested tuple or a list as one of its elements. Tuples stored in other tuples as their elements are called nested tuples. Creating a nested tuple is similar to creating a nested list – we just have to specify additional nested parentheses enclosing the nested tuples.
We can create a nested tuple without using nested parentheses, but that is not recommended as it can be confusing for someone reading the code. Everything else about working with nested tuples is similar to working with nested lists – we can index them, slice them, concatenate them, etc. Creating Python tuples is quick and easy- once you get the hang of it, you’ll be able to create them in no time!
Tuples are the fastest data type to instantiate, particularly in the case of tuple literals:
python3 -m timeit '(1,2,3)'
50000000 loops, best of 5: 6.48 nsec per loop
python3 -m timeit '[1,2,3]'
5000000 loops, best of 5: 44.4 nsec per loop
On my machine, it’s more than 7 times faster.
Python tuples are always compared to lists, so here are the major differences:
- Tuples are fixed-length sequences of heterogeneous data (stores elements of different types)
- Lists are for arbitrary-length sequences of homogeneous data (stores data of the same type)
The best way to think about tuples is that lists are like the columns in a spreadsheet, and tuples are rows:
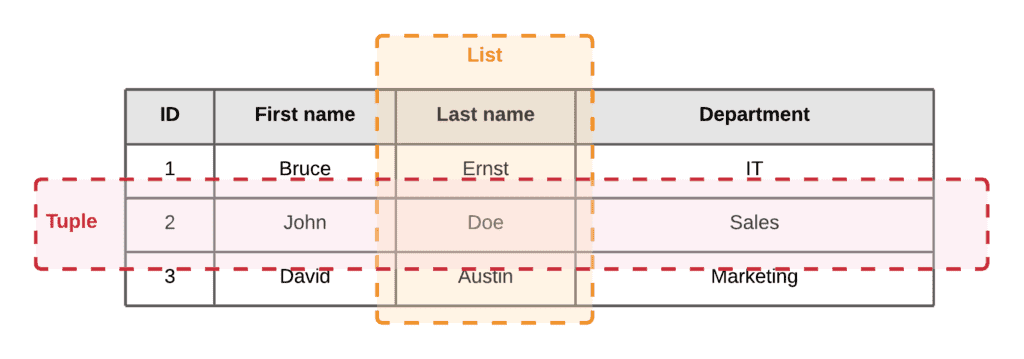
Creating a tuple
Creating a tuple is easy. Simply enclose the elements you want to include in parentheses. For example, when creating a tuple containing the integers 1, 2, and 3, you need to write: a = (1, 2, 3)
. You can also create tuples containing duplicate values. For example, a = (1, 2, 2)
is a valid tuple. However, if you try to create a tuple with duplicate values using the set()
function, only one copy of each value will be included in the new tuple. This is because Python sets cannot contain duplicate values.
Let’s take a look at how you can create a tuple:
# Empty tuple
record = ()
print(record)
# Tuple with mixed datatypes
record = (2, "John", "Doe", "Sales")
print(record)
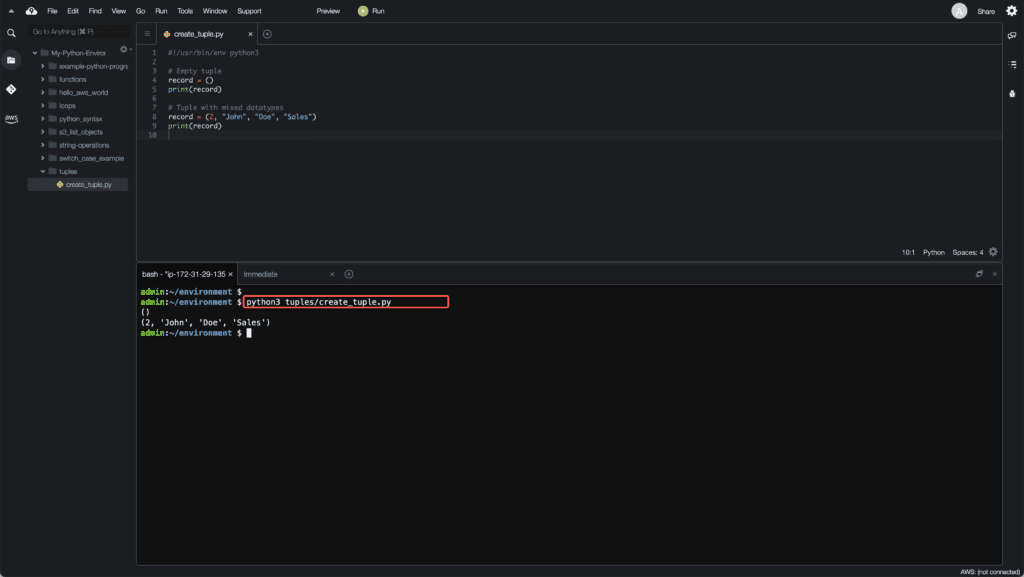
We’ve created an empty tuple and a tuple object containing mixed data types (int and str). After the second assignment, the record variable in the example above will store a reference to a new tuple (new data in memory).
If you’d like to define a tuple that consists of one element (having a single value), you can do it using the following notation:
# defining tuple of one element
a = 1,
# alternative way
a = tuple(1)
Packing and unpacking Python tuples
A tuple can be created without using parentheses.
This operation is known as tuple packing:
record = 2, "John", "Doe", "Sales"
print(record)
Wise versa, you can extract tuple values back into variables. This operation is called unpacking a tuple:
rec_id, rec_first, rec_last, rec_dep = record
print(f'ID: {rec_id}')
print(f'First name: {rec_first}')
print(f'Last name: {rec_last}')
print(f'Department: {rec_dep}')
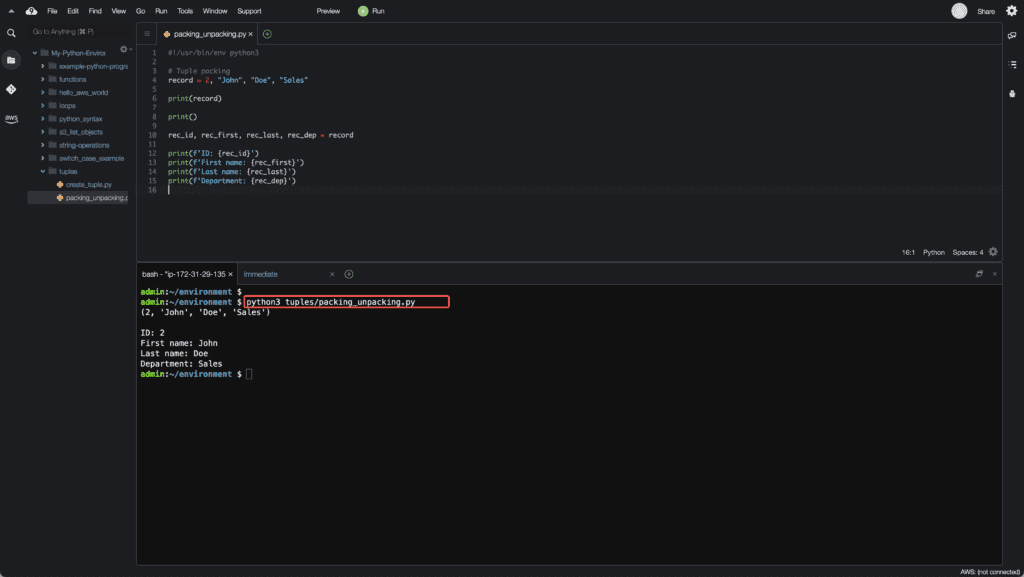
Note:
- During the tuple unpacking operation, the number of variables on the left-hand side should be equal to the number of values in a given tuple.
- If you’d like to skip some of the tuple elements, you may use
_
(underline character) instead of providing a variable. - In addition to that
*args
syntax (arbitrary variables number) is also available.
record = (2, "John", "Doe", "Sales")
# Skipping tuple element during unpacking
_, rec_first, rec_last, rec_dep = record
print(f'First name: {rec_first}')
print(f'Last name: {rec_last}')
print(f'Department: {rec_dep}')
print()
# Using arbitrary argument list during unpacking
rec_id, *args, rec_dep = record
print(f'ID: {rec_id}')
print(f'Department: {rec_dep}')
for arg in args:
print(f'arg = {arg}')
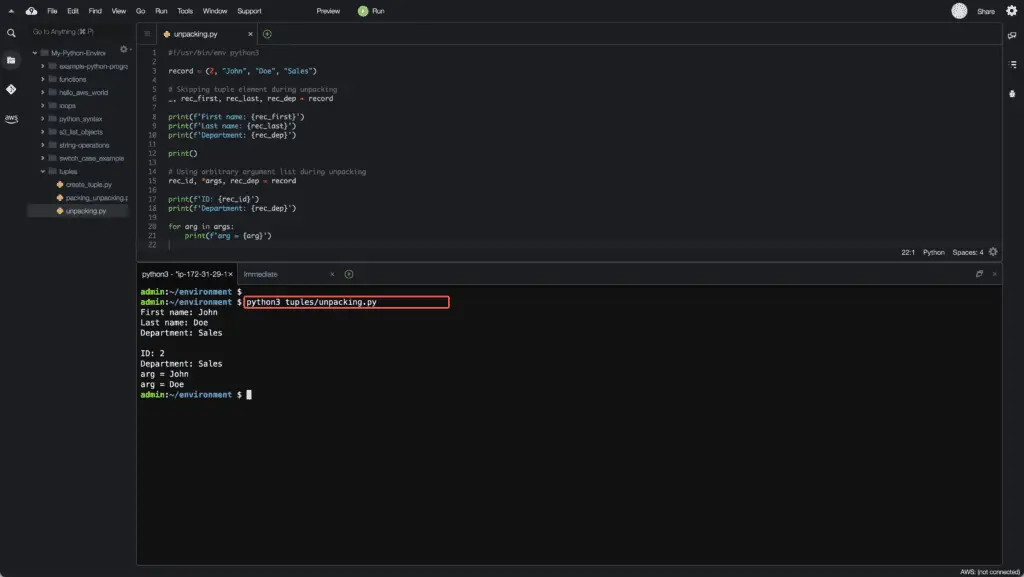
Access tuple elements
Now it’s time to look at how we can access the tuple elements.
We have several different options:
- Using the element index – you’ll get a single element of a tuple
- Using slicing operations – you’ll get a subset of tuple items and the return data structure type will be of class tuple
Accessing by index
You can use the index operator []
to access every item in a tuple, where the index starts from 0:
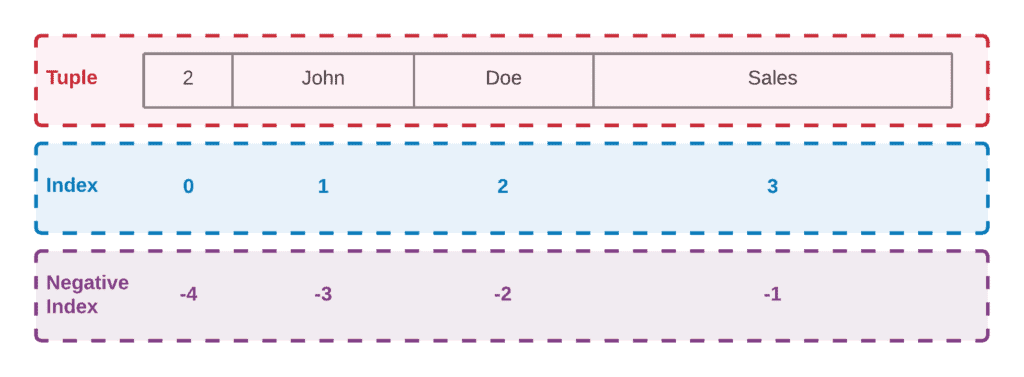
Note: trying to access an index outside of the tuple index range(in our case 5, 6, etc.) will raise an IndexError
Python exception.
record = (2, "John", "Doe", "Sales")
rec_id = record[0]
rec_first = record[1]
print(f'ID: {rec_id}')
print(f'First name: {rec_first}')
print()
rec_last = record[-2]
rec_dep = record[-1]
print(f'Last name: {rec_last}')
print(f'Department: {rec_dep}')

Tuple slicing
We can access the range of items in a tuple by using the slicing operator : (colon):
record = (2, "John", "Doe", "Sales")
# Elements from beginning to end
print(record[:])
# Elements from 2nd to 3rd
print(record[1:3])
# Elements from beginning to 2nd
print(record[:-2])
# Elements from 3rd to end
print(record[2:])
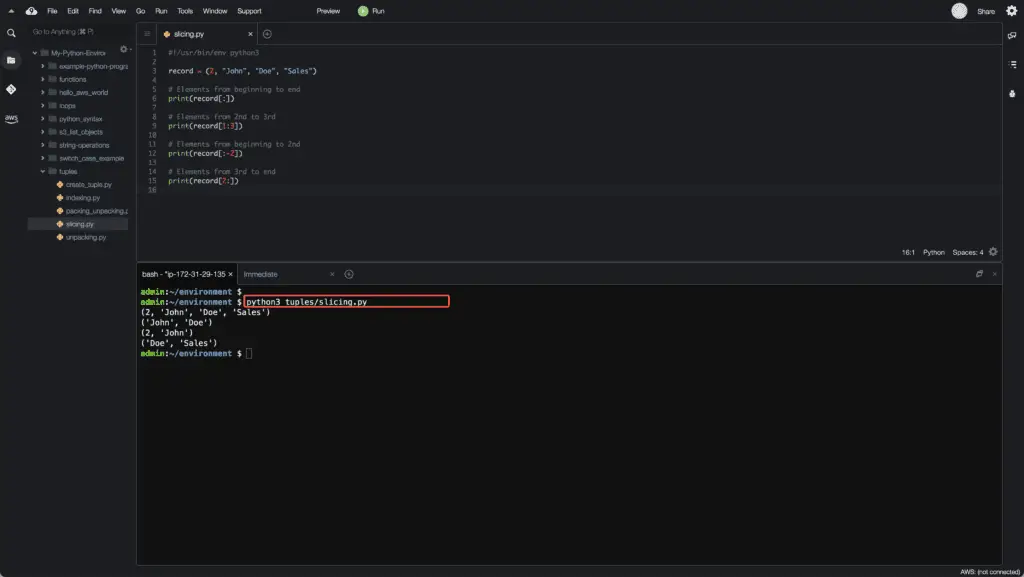
Each operation returns a subset of tuple items.
Other tuple operations
Modifying values
As soon as a tuple is immutable, you can’t modify its values directly, but you can convert it to the list to make the required changes:
record = (2, "John", "Doe", "Sales")
print(f'Original tuple: {record}')
record_list = list(record)
record_list[1] = 'Jane'
record = tuple(record_list)
print(f'Modified tuple: {record}')
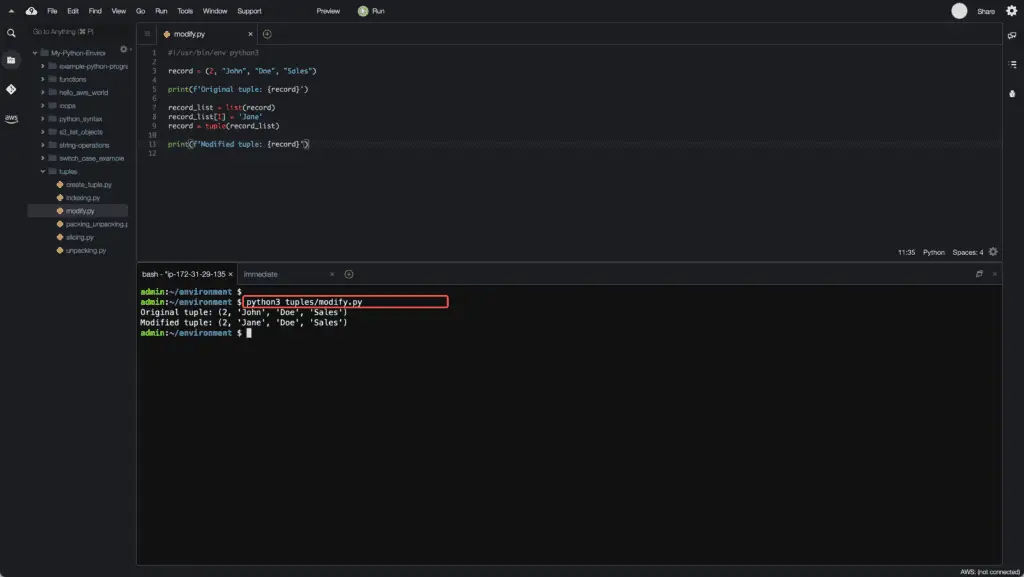
Deleting a tuple
To delete a tuple completely, you have to use del
keyword:
record = (2, "John", "Doe", "Sales")
del record
# NameError: name 'record' is not defined
# print(record)
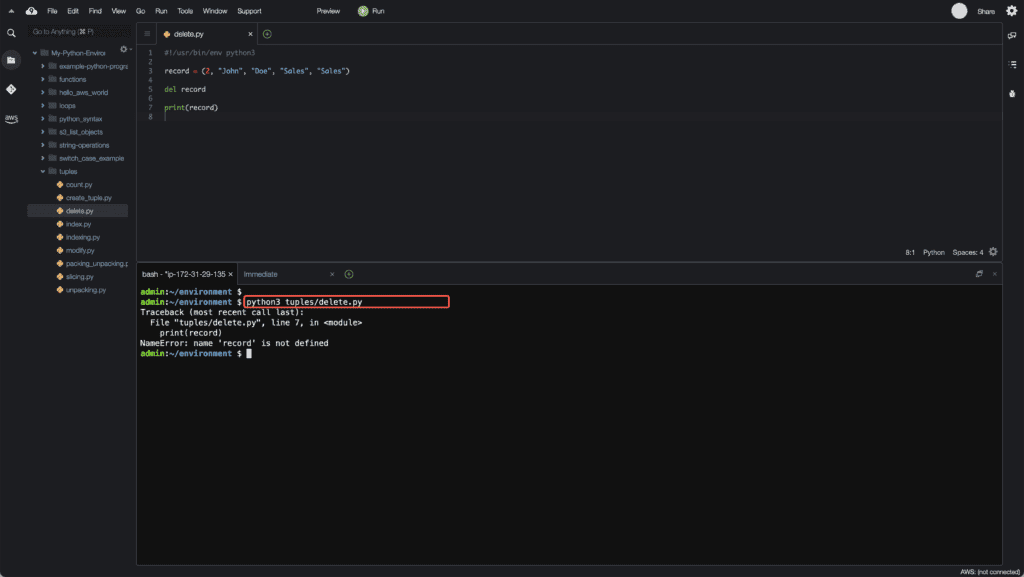
The code block above will delete a variable record
referencing a tuple.
Counting elements
To get several repeated elements in a tuple, use the following code:
record = (2, "John", "Doe", "Sales", "Sales")
items_count = record.count('Sales')
print(f'Items count: {items_count}')
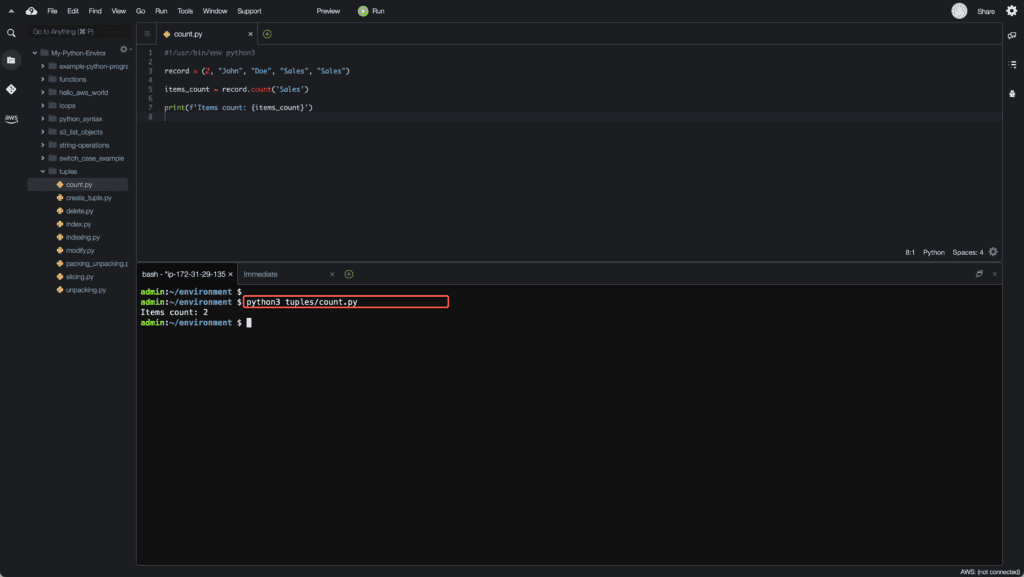
Getting element index
Tuple class also provides a convenient method to get element index value:
record = (2, "John", "Doe", "Sales", "Sales")
item_index = record.index('John')
print(f'Item index: {item_index}')
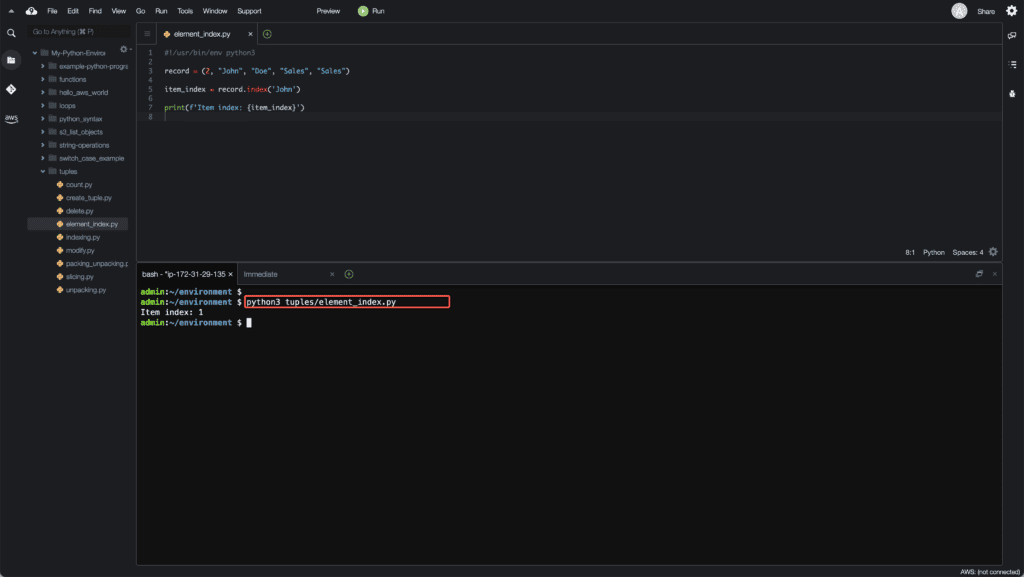
Check that element exists in a tuple
There’re two ways of checking that element exists in a tuple:
- By using
index()
method - By using
in
expression
The most straightforward way to do this is to use in
expression:
record = (2, "John", "Doe", "Sales")
element = 3
is_found = element in record
print(f'Is {element} found in tuple? => {is_found}')
element = 'John'
is_found = element in record
print(f'Is {element} found in tuple? => {is_found}')
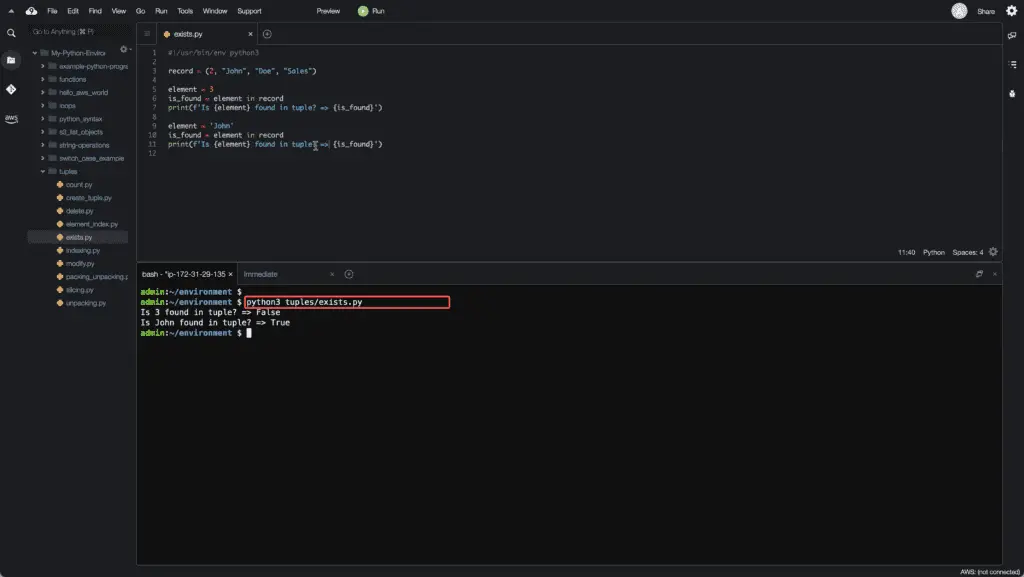
You may use index()
tuple method to check element membership in a tuple, but keep in mind that it will generate ValueError: tuple.index(x): x not in tuple exception, if the value not found:
record = (2, "John", "Doe", "Sales")
try:
element = 'IT'
item_index = record.index(element)
except ValueError:
item_index = None
finally:
if item_index:
is_found = True
else:
is_found = False
print(f'Is {element} found in tuple? => {is_found}')
We’ll cover Python exception handling in a future article.
Iterating over tuple
We can use for loop to iterate over an entire tuple:
record = (2, "John", "Doe", "Sales")
for item in record:
print(f'Item: {item}')
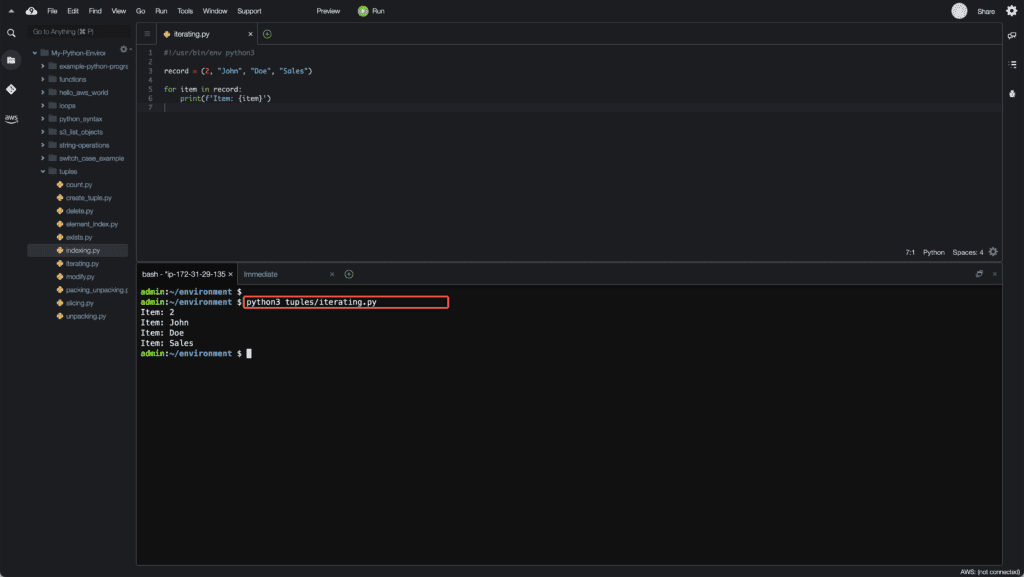
Named tuples
The major problem with tuples is that it is hard to remember which element has which index.
At this point, many developers start creating a class with read-only properties for storing the data and get access to ut using class.field
notation (for example, record.id
, record.first_name
, etc.).
It’s overkill to use a class for this purpose, and Python provides us with an elegant solution – named tuples.
Named tuples are easy-to-create and lightweight object types and we can reference their elements using object-like variables or the standard tuple syntax. A named tuple is immutable as a regular tuple, so you can not change its values as soon as you create it.
Creating named tuple
To create a named tuple, we need to import the namedtuple class from the collections module:
from collections import namedtuple
Record = namedtuple('Record', 'id first_name last_name department')
rec = Record(2, "John", "Doe", "Sales")
for item in rec:
print(f'Item: {item}')
print()
print(f'ID: {rec.id}')
print(f'First name: {rec.first_name}')
print(f'Last name: {rec.last_name}')
print(f'Department: {rec.department}')
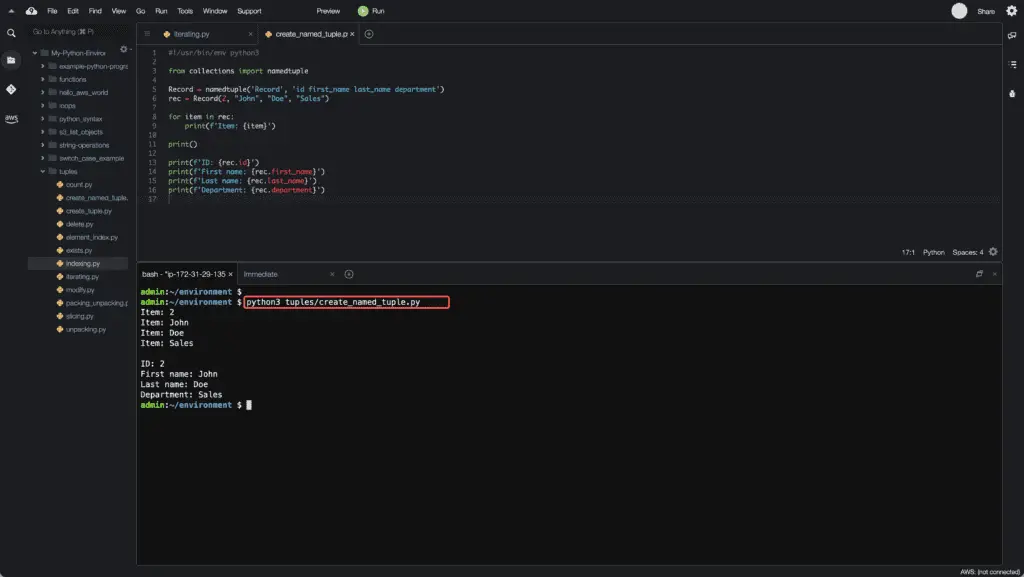