Managing AWS profiles is fundamental to using Boto3, the Python SDK for Amazon Web Services (AWS). This guide focuses on the configuration and practical use of AWS profiles in Boto3, which is essential for developers working with multiple AWS accounts or permission sets.
import boto3
session = boto3.Session(profile_name='power-user-account1')
s3_client = session.client('s3')
# or
s3_resource = session.resource('s3')
Understanding AWS CLI Profiles
AWS CLI Profiles contain configuration data that Boto3 uses to interact with AWS services. Profiles are a set of credentials and configuration values that include your aws_access_key_id
, aws_secret_access_key
, and default region.
Configure different profiles to use different IAM users or roles within the same or different AWS account.
You can store multiple profiles in the ~/.aws/credentials and ~/.aws/config files, allowing you to switch between different sets of credentials and configurations seamlessly.
Use AWS SSO or aws-vault for more secure AWS credentials management.
AWS Profiles Configuration
Your first step is to install Boto3 in a virtual environment.
python3 -m venv .venv
source .venv/bin/activate
pip install --upgrade pip
pip install boto3 awscli
After that, configure your AWS credentials using the AWS CLI:
aws configure
This command will prompt you for the aws_access_key_id
, aws_secret_access_key
, and default region and will save them to the ~/.aws/credentials file.
Example AWS Credentials File
[default]
aws_access_key_id = YOUR_DEFAULT_ACCESS_KEY
aws_secret_access_key = YOUR_DEFAULT_SECRET_KEY
[profile user2]
aws_access_key_id = YOUR_USER2_ACCESS_KEY
aws_secret_access_key = YOUR_USER2_SECRET_KEY
Setting up Boto3 AWS Profiles
Use AWS_PROFILE environment variable or directly pass the profile_name
variable to use the required AWS profile from your Python scripts. You can use multiple profiles through Boto3 Sessions.
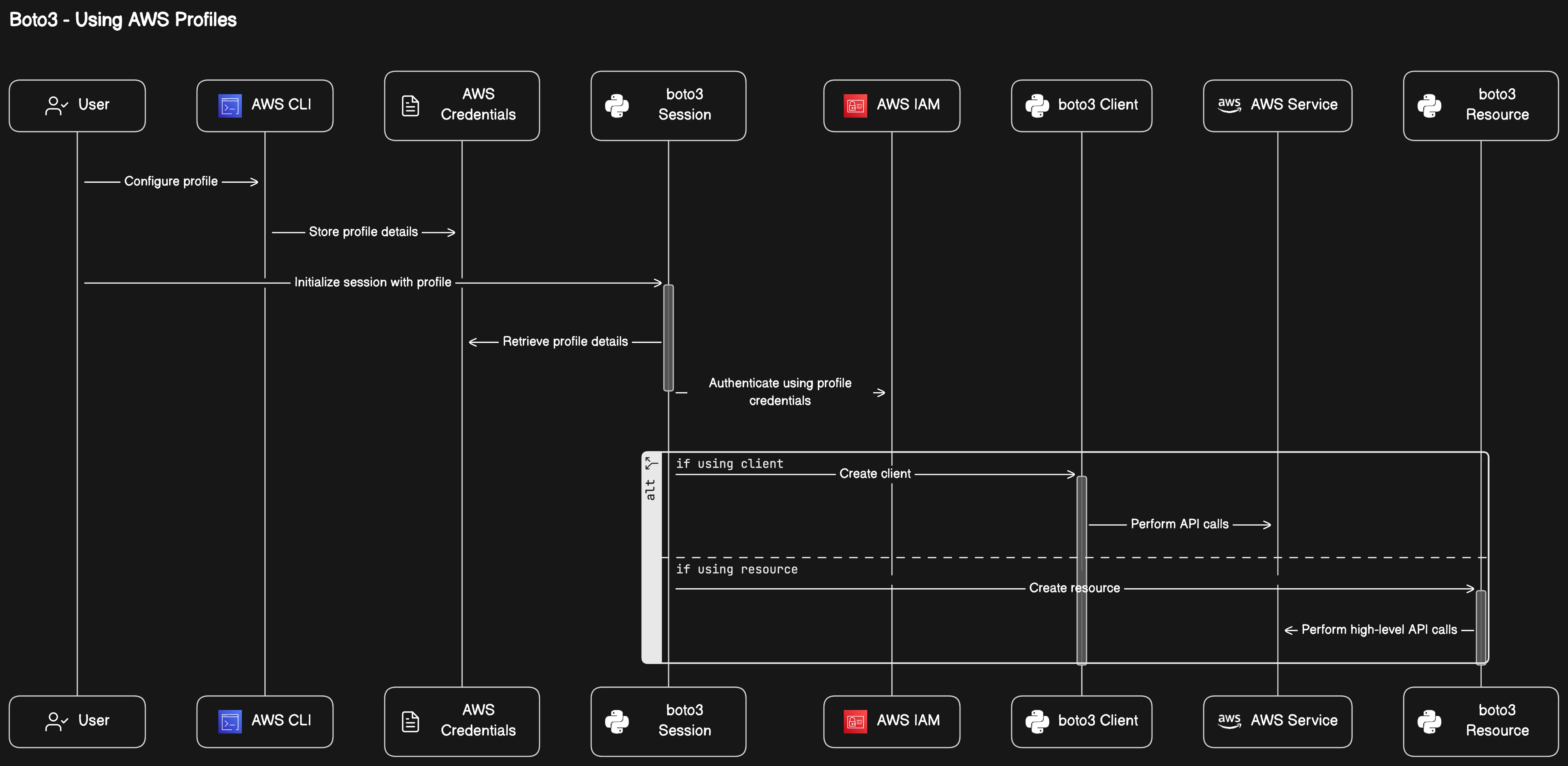
Boto3 session
Boto3 sessions offer a dynamic approach to managing AWS profiles. To create a session using a specific profile:
import boto3
session = boto3.Session(profile_name='user2')
This session will employ the ‘user2’ profile for AWS service interactions.
Boto3 client
To use a specific AWS profile for a Boto3 client, initialize the client within the context of a session.
import boto3
session = boto3.Session(profile_name='user2')
s3_client = session.client('s3')
# Perform S3 operations using 'user2' profile credentials
Boto3 resource
Similarly, Boto3 resources can be initialized using sessions to leverage specific AWS profiles.
import boto3
session = boto3.Session(profile_name='user2')
dynamodb = session.resource('dynamodb')
# Interact with DynamoDB using 'user2' profile credentials
Error Handling
Proper error handling in Boto3 enhances the robustness of Python scripts.
In the context of this topic, you can handle NoCredentialsError
exception:
import boto3
from botocore.exceptions import NoCredentialsError
try:
s3_client = boto3.client('s3')
# Operations with S3
except NoCredentialsError:
print("Credentials not available")
Conclusion
Understanding and effectively managing AWS profiles in Boto3 is vital for Python developers working with AWS. This guide explains the setup, selection, and usage of AWS profiles in both Boto3 sessions and individual service clients or resources, promoting a secure, organized, and efficient approach to AWS service management.