Python Dictionary – Complete Tutorial
Learning how to use a Python dictionary is important because it allows you to store and efficiently organize data. Dictionaries are one of the most powerful data structures available in Python, as they allow users to access, insert, delete, and update items quickly. Additionally, dictionaries are commonly used for database manipulation, text parsing, and more.
This tutorial covers a Python dictionary in detail and answers the most common questions, like creating a dictionary and adding, accessing, and removing its elements. In addition, we’ll cover advanced topics like converting dictionaries to other formats (JSON and YAML), saving dictionaries to Excel, and much more. Let’s get started!
Table of contents
Dictionaries
A dictionary in Python is a data structure that stores key-value pairs. It is similar to a hash table or associative array in other programming languages and can store and access data efficiently. A dictionary uses keys as indexes to quickly retrieve the associated values.
Creating Python Dictionary
A dictionary can be created by placing items inside curly braces {}
separated by commas.
An item has a key and a corresponding value, colon (:
) separates each key from its associated value and is expressed as a pair (key: value
).
An empty dictionary without any items is written with two curly braces {}
.
Keys in a dictionary are unique, while values may not be.
The values of a dictionary can be of any type, but the keys must be of an immutable data type such as strings, numbers, or tuples.
If the script requires the key to be an integer/number, then quotes must not be applied; otherwise, the key will be a string.
So, while initializing a dictionary, the key data type must be handled properly.
Below is the code example to initialize different types of dictionaries.
# Initializing an empty dictionary
empty_dict = {}
print(empty_dict)
# Initializing a dictionary with string and integer keys
stringInteger_dict = {
'name': 'Python',
1: 'Language'
}
print(stringInteger_dict['name'])
# Initializing a dictionary with String, int, boolean, and list data types
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
print(differentDataTypes_dict)
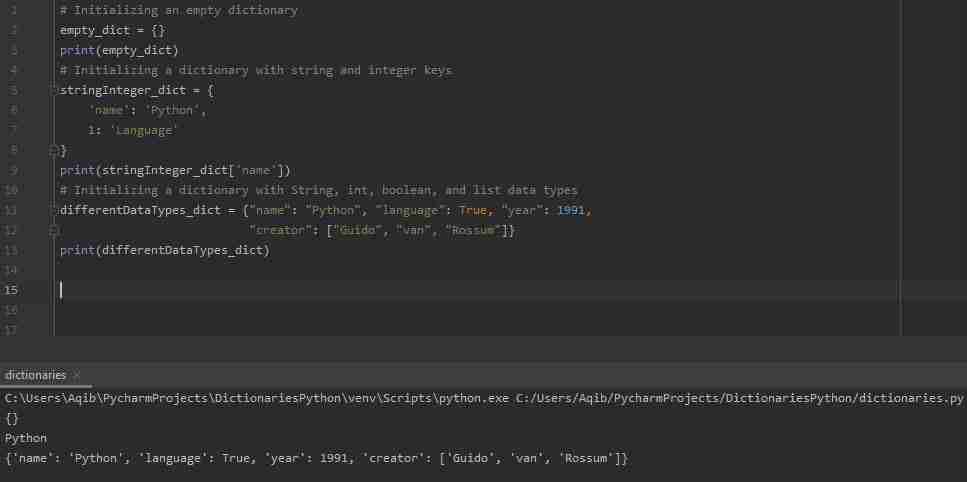
Copy and paste the above code into your editor; you will get the output in the above snapshot.
Access Dictionary Items
So, we created different types of dictionaries, and now it is time to access the dictionary items.
Unlike other data types that use indexes to access the values, a dictionary uses keys to access values.
We can query a python dictionary to get value against the provided key. A value is retrieved from a dictionary by specifying its corresponding key in square brackets ([]
) or with the get()
method.
The method to retrieve a value from a key is called Dictionary lookup. The reverse lookup is to find a key against the value.
If we use the square brackets []
, KeyError
is raised in case a key is not found in the dictionary.
On the other hand, the get()
method returns None if the key is not found.
# Initializing an empty dictionary
empty_dict = {}
print(empty_dict['name'])
# Initializing a dictionary with string and integer keys
stringInteger_dict = {
'name': 'Python',
1: 'Language'
}
print(stringInteger_dict['name'])
# Initializing a dictionary with String, int, boolean, and list data types
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
print(differentDataTypes_dict['creator'][-1])
In the below output, we get KeyError
as we used square brackets.
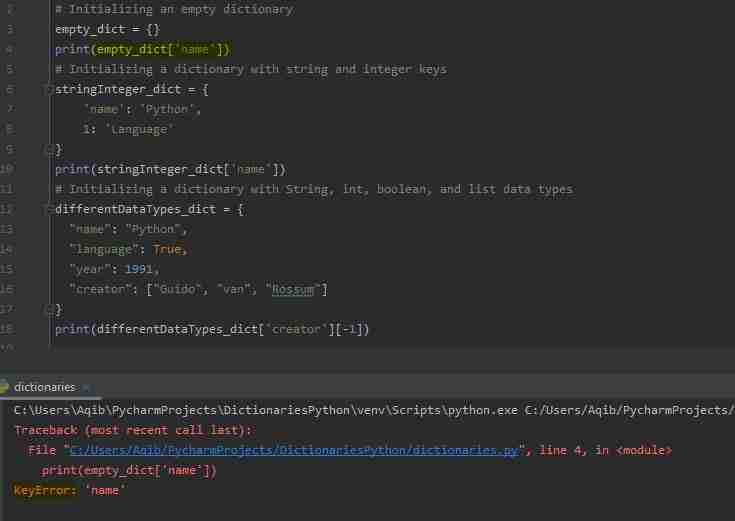
If we use get()
method then we will get None
output, as shown below, thus bypassing the KeyError
Python exception.
print(empty_dict.get('name'))
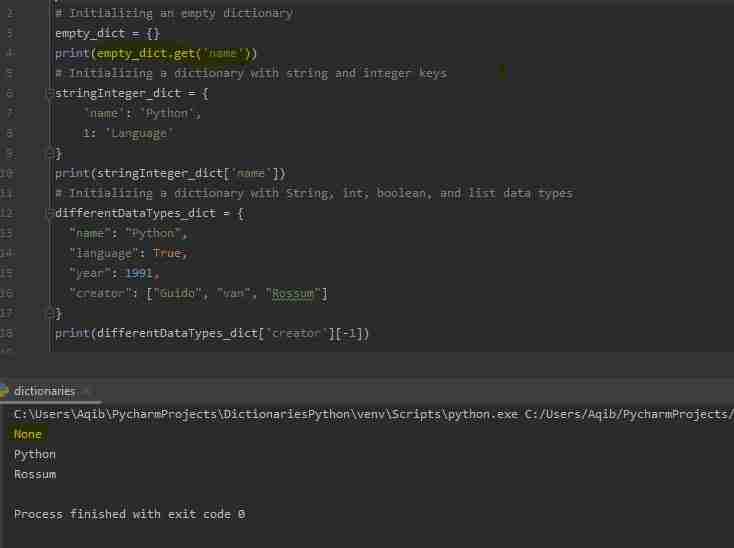
Are Dictionary Keys Case-Sensitive?
It is important to know that dictionary keys are case-sensitive.
The below code and example output explain this.
# Initializing dictionary with two key:value pairs
case_sensitivity = {'year': 1991, 'Year': 2021}
# The output will depend on the key passed.
# Keys names are same but are case-sensitive.
# One key starts with lower-case and the other with upper-case letter.
print(case_sensitivity['year'])
print(case_sensitivity['Year'])
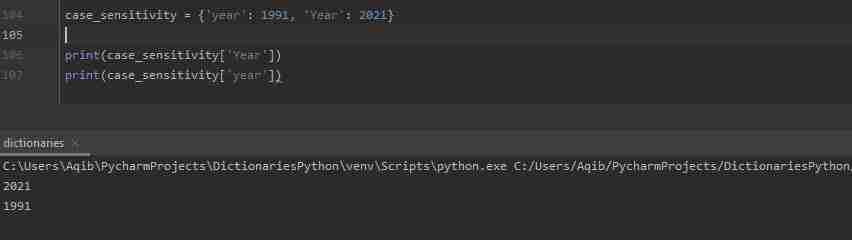
Dictionary Keys With Spaces
Keys can contain spaces.
keysWithSpaces = {'year 1991': 1991, 'Year 2021': 2021}
print(keysWithSpaces['Year 2021'])
print(keysWithSpaces['year 1991'])
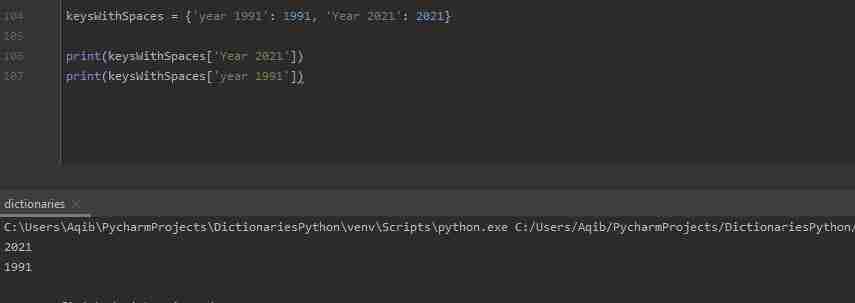
Can List be used as a dictionary key?
A dictionary key can be a tuple but not a list. The key must be of an immutable data type, such as strings, numbers, or tuples.
There is an explanation on this topic in the Python Wiki https://wiki.python.org/moin/DictionaryKeys.
Ordering, Changeability, and Duplicates
Dictionary items are ordered, changeable, and do not allow duplicates.
Dictionaries are unordered in Python 3.6 and the earlier versions, while in Python 3.7, dictionaries are ordered.
- Ordered means that the items have a specific order, and that order will not change. On the other hand, unordered means that the items do not have a specific order, we cannot refer to an item by using an index.
- Changeable means adding, changing, or removing items from the dictionary.
- When we say that dictionaries do not allow duplicates it means that a dictionary cannot have two items with the same key. If we use two same keys, the key will get the value placed last in the dictionary (see the below example output).
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1990, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
print(differentDataTypes_dict['year'])
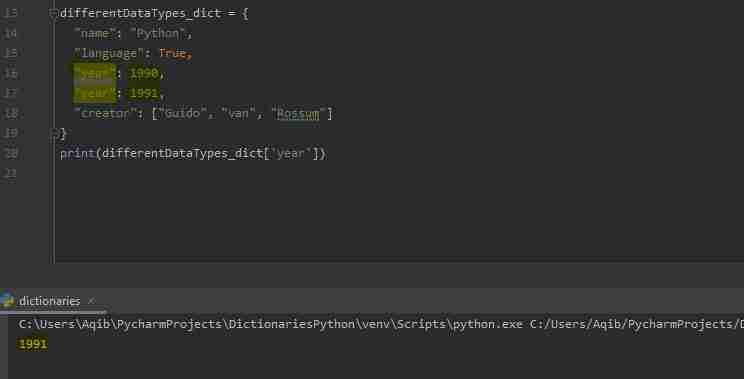
Length of Dictionary
To access the length of a dictionary, we use len()
method.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
print(len(differentDataTypes_dict))
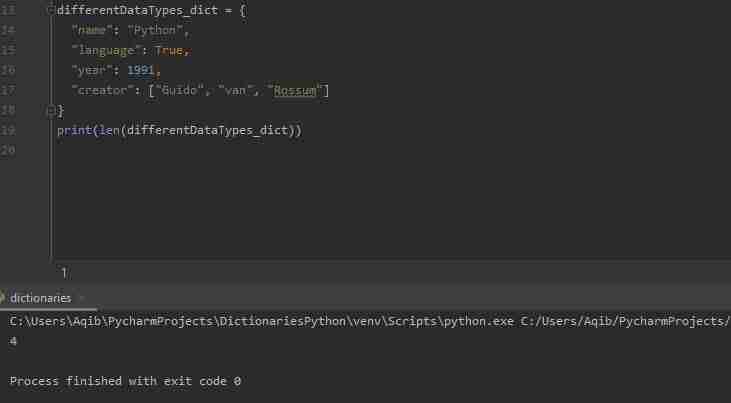
Changing Dictionary Items
A dictionary item’s value can be changed using the assignment operator; hence dictionaries are mutable.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1990, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
differentDataTypes_dict["name"] = "Python Programming Language"
print(f'Changed item value --> {differentDataTypes_dict["name"]}')
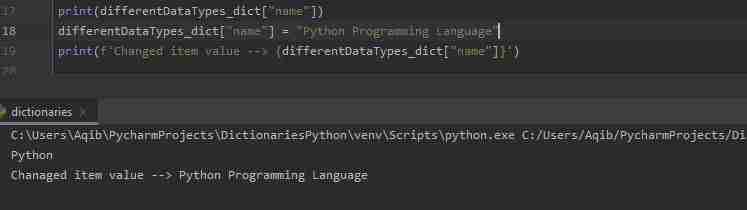
Adding/Appending Dictionary Items
We can add/append a new item in a dictionary using an assignment operator.
If the key is already present in a dictionary, then the existing value gets updated.
If the key is not present, a new item is added/appended to the dictionary.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1990, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
print(differentDataTypes_dict)
differentDataTypes_dict["website"] = "https://www.python.org/"
print(differentDataTypes_dict)

Removing Dictionary Items
Removing Specific Item
A specific item in a dictionary can be removed by using the pop()
method.
This method removes an item with the provided key and outputs the removed value.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
print(differentDataTypes_dict.pop("language"))
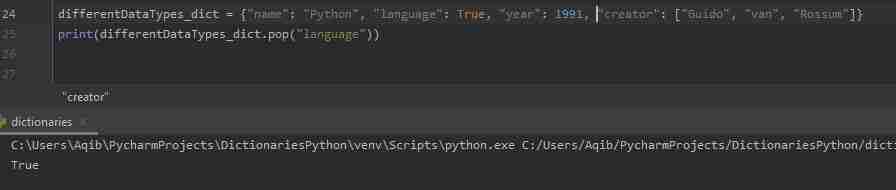
Removing Random/Last Item
The popitem()
method removes the last inserted item (in versions before 3.7, a random item is removed instead).
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
print(differentDataTypes_dict.popitem("language"))
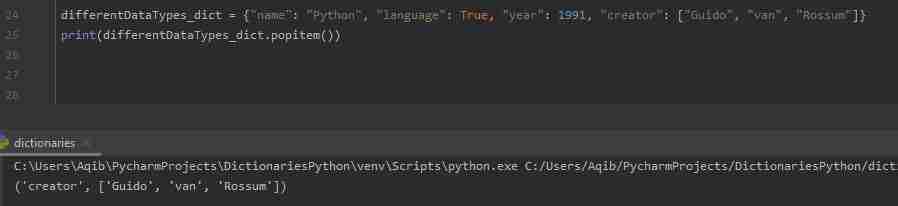
Emptying A Dictionary
The clear()
method empties a dictionary.
The resulting dictionary contains no items.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
differentDataTypes_dict.clear()
print(differentDataTypes_dict)
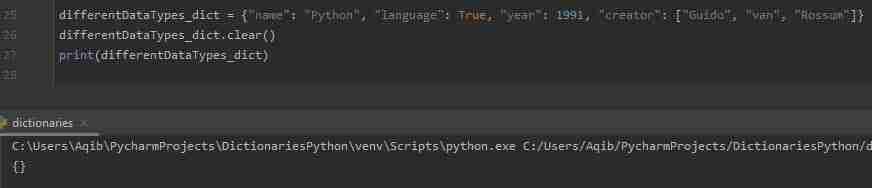
Deleting Whole Dictionary
The del
keyword is used to delete the dictionary completely.
Printing the deleted dictionary will raise an error because the dictionary does not exist anymore.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991,
"creator": ["Guido", "van", "Rossum"]}
del differentDataTypes_dict
print(differentDataTypes_dict)
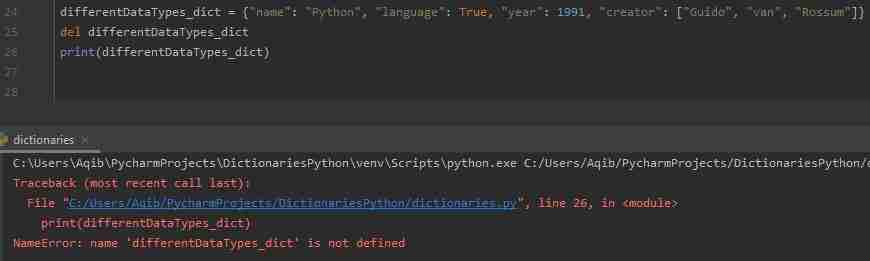
Copy A Dictionary
We cannot copy a dictionary simply by typing dict2 = dict1
, because dict2
will only be a reference to dict1
and changes made in dict1
will automatically also be made in dict2
.
There are ways to make a copy.
One way is to use the built-in dictionary method copy()
.
dict1 = {
"name": "Python",
"is_python": True,
"year": 1991
}
dict2 = dict1.copy()
print(dict2)
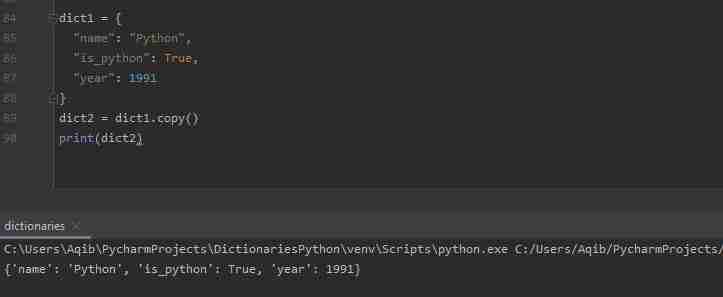
Another way to make a copy is to use the built-in function dict()
.
dict1 = {
"name": "Python",
"is_python": True,
"year": 1991
}
dict2 = dict(dict1)
print(dict2)
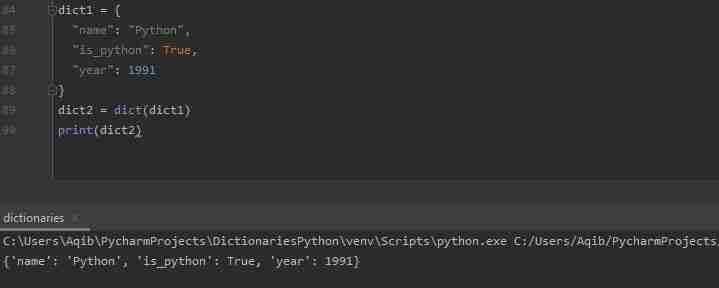
Iterating Through A Dictionary
A dictionary can be iterated using for loop. When looping through a dictionary, we can either return keys or values.
Return All Keys
A “for loop” can be used to print all keys, one by one, in a dictionary.
keys()
method can also be used to print the keys in a dictionary.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991, "creator": ["Guido", "van", "Rossum"]}
for keys in differentDataTypes_dict:
print(keys)
differentDataTypes_dict1 = {1: "a", 2: "b", 3: "c", 4: "d"}
for keys in differentDataTypes_dict1.keys():
print(keys)
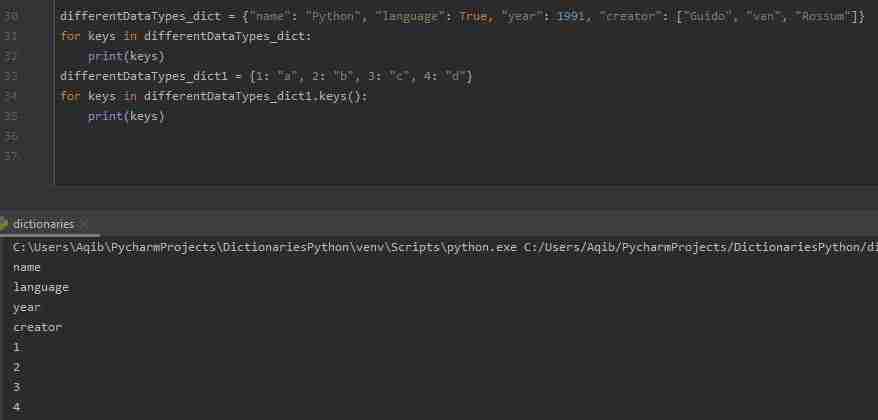
Return All Values
A for loop can be used to print all values, one by one, in a dictionary.
The values()
method can also be used to print the values in a dictionary.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991, "creator": ["Guido", "van", "Rossum"]}
for keys in differentDataTypes_dict:
print(differentDataTypes_dict[keys])
differentDataTypes_dict1 = {1: "a", 2: "b", 3: "c", 4: "d"}
for value in differentDataTypes_dict1.values():
print(value)
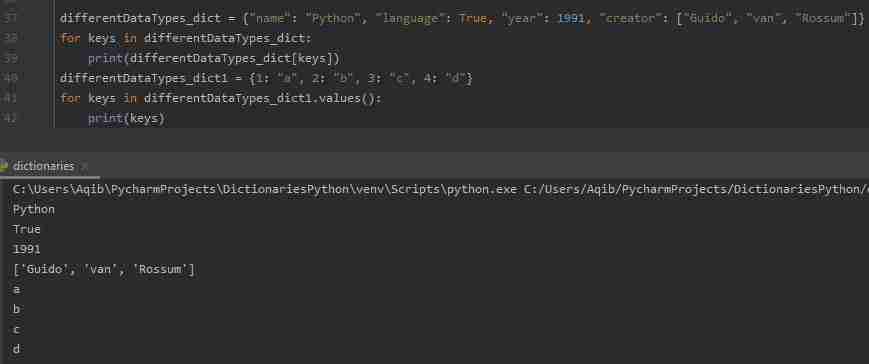
Loop through both keys and values
We can loop through both keys and values by using the items()
method.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991, "creator": ["Guido", "van", "Rossum"]}
for keys, values in differentDataTypes_dict.items():
print(f"{keys} --> {values}")
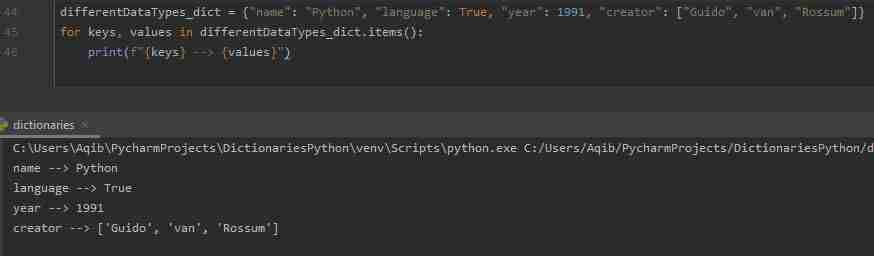
Sorting a Python Dictionary
To sort items in a dictionary sorted()
method can be used.
Sorting can be done using keys()
, values()
, and items()
.
The sorted keys will return when the keys()
method will be used in the sorted()
method.
The sorted values will return when values()
method will be used in the sorted()
method.
When items()
is used, the dictionary items will be returned sorted using keys and will show key:value
pair.
By default, sorted()
method will output the keys and values in ascending order.
However, if the output is needed to be shown in descending order, then we can use reverse=True
.
Sorting using keys()
differentDataTypes_dict = {3: "c", 1: "a", 4: "d", 2: "b"}
print(sorted(differentDataTypes_dict.keys()))
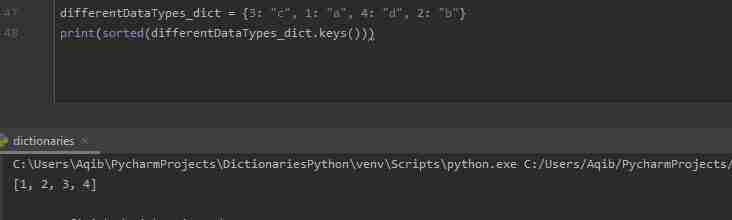
Sorting using values()
differentDataTypes_dict = {3: "c", 1: "a", 4: "d", 2: "b"}
print(sorted(differentDataTypes_dict.values()))
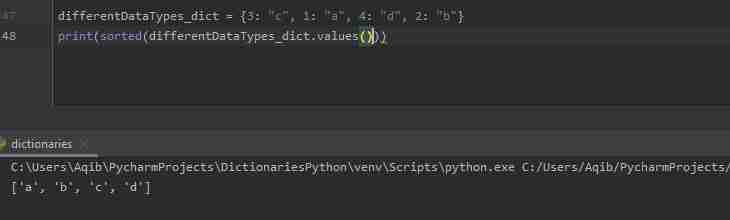
Sorting using items()
differentDataTypes_dict = {3: "c", 1: "a", 4: "d", 2: "b"}
print(sorted(differentDataTypes_dict.items()))
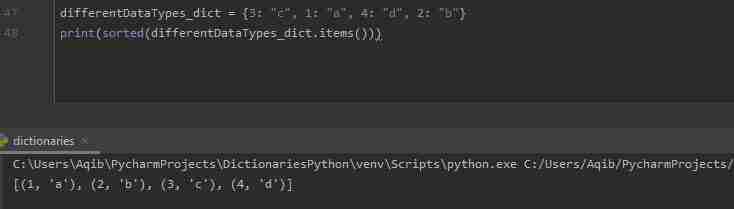
Sorting in descending order
differentDataTypes_dict = {3: "c", 1: "a", 4: "d", 2: "b"}
print(sorted(differentDataTypes_dict.keys(), reverse=True))
print(sorted(differentDataTypes_dict.values(), reverse=True))
print(sorted(differentDataTypes_dict.items(), reverse=True))
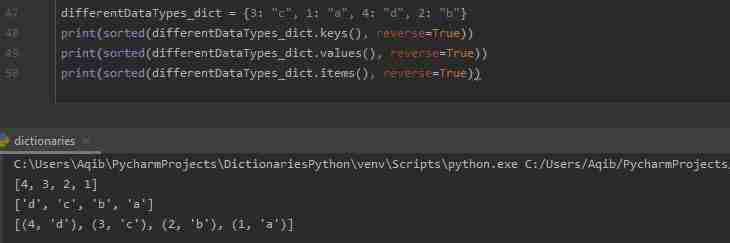
Merging Dictionaries
Two given dictionaries can be merged into a single dictionary. **
also called kwargs
and update()
can be used to merge two dictionaries.
Merge two dictionaries using the update() method
The update()
method would merge one dictionary with another.
If we have two dictionaries dict1
with dict2
then after using the update()
method the dict1
will have the contents of dict2
.
dict1 = {1: "a", 2: "b"}
dict2 = {3: "c", 4: "d"}
dict1.update(dict2)
print(dict1)
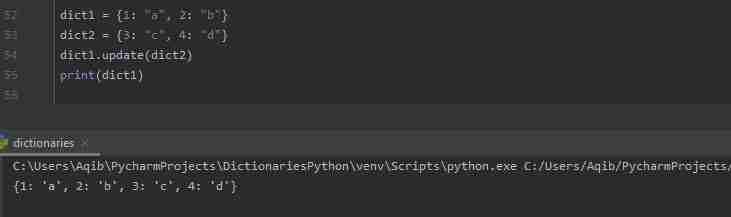
Merging dictionaries using the ** method (From Python 3.5 onwards)
The **
is called kwargs
in Python. Python versions 3.5 and above support this.
Using **
, the two dictionaries will be merged, and it will return the merged dictionary.
The use of **
in front of the variable will replace the variable with all its content.
dict1 = {1: "a", 2: "b"}
dict2 = {3: "c", 4: "d"}
dict3 = {**dict1, **dict2}
print(dict3)
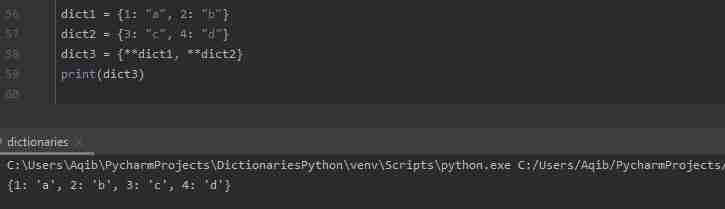
Dictionary Membership Test
Using the “in” keyword
If we want to test whether the key exists in a dictionary, we can use a membership test.
This test can be performed only on the key of a dictionary and not the value.
The membership test is done using the in
keyword.
When we check the key in the dictionary using in
keyword, the expression returns true if the key is present and false if not.
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991, "creator": ["Guido", "van", "Rossum"]}
print("name" in differentDataTypes_dict)
print("membership" in differentDataTypes_dict)
print("year" in differentDataTypes_dict)
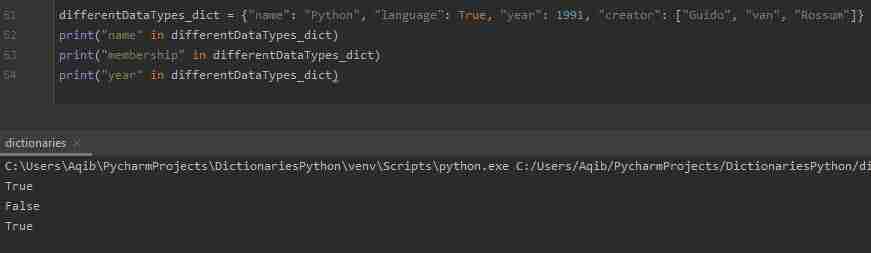
Enumerate Dictionary in Python
The enumerate()
Python returns an enumerate-type object and adds a counter variable to iterate over a list or some other type of collection.
It makes looping over such objects easier. We can also use the enumerate() function with dictionaries as well.
We can enumerate the keys of a dictionary and both keys and values of a dictionary.
The example code and output are given below.
# Example of enumerating the keys of a dictionary
print("*** Enumerating the keys ***")
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991, "creator": ["Guido", "van", "Rossum"]}
for i, k in enumerate(differentDataTypes_dict):
print(k)
# Example of enumerating through both keys and values of a dictionary
print("*** \nEnumerating through both keys and values ***")
for i, (k, v) in enumerate(differentDataTypes_dict.items()):
print(f"{k}: {v}")

Deep Copy And Shallow Copy A Dictionary In Python
Copying a dictionary creates a duplicate object through either a deep copy or a shallow copy.
Deep Copy
copy.deepcopy(dictionary)
returns a deep-copy of dictionary
.
Changes made to the original dictionary will not affect the deep-copied dictionary.
import copy
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991, "creator": ["Guido", "van", "Rossum"]}
copied_dict = copy.deepcopy(differentDataTypes_dict)
# Modifying objects in original dictionary
differentDataTypes_dict["name"] = "Python with hands-0n.cloud"
print(f"Original Dictionary --> {differentDataTypes_dict}")
print(f"\nCopied Dictionary --> {copied_dict}")
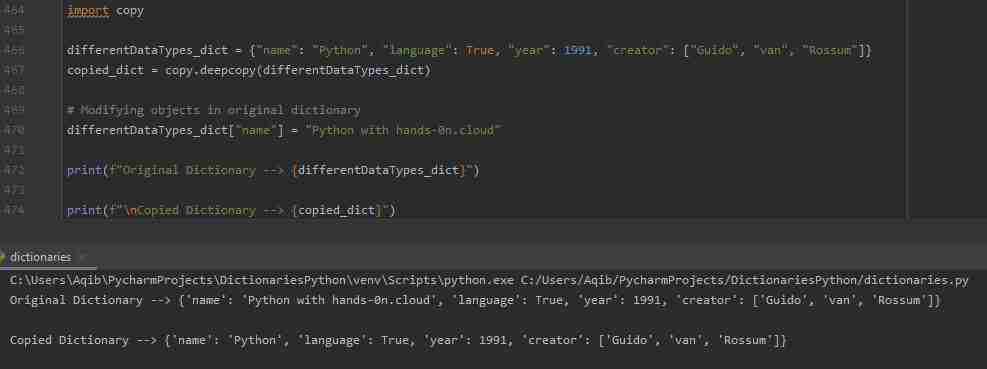
Shallow Copy
copy.copy(dictionary)
returns a shallow-copy of dictionary
.
Changes made to the original dictionary will affect the shallow-copied dictionary.
import copy
differentDataTypes_dict = {"name": "Python", "language": True, "year": 1991, "creator": ["Guido", "van", "Rossum"]}
copied_dict = copy.copy(differentDataTypes_dict)
# Modifying objects in original dictionary
differentDataTypes_dict["creator"][0] = "G."
print(f"Original Dictionary --> {differentDataTypes_dict}")
print(f"\nCopied Dictionary --> {copied_dict}")
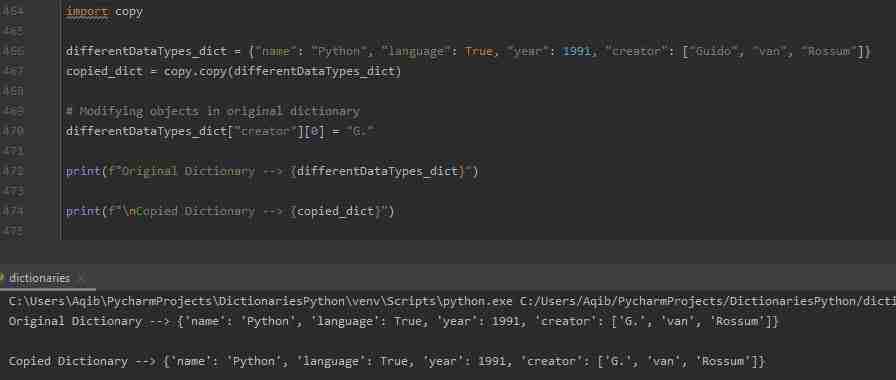
Nested Dictionaries
When one dictionary contains two or more dictionaries, this is called a nested dictionary.
languages = {
"language1": {
'name': 'Python',
'year': 1991
},
"language2": {
'name': 'C++',
'year': 1979
},
"language3": {
'name': 'Matlab',
'year': 1984
}
}
print(languages["language1"]["name"])
print(languages["language2"]["name"])
print(languages["language3"]["name"])
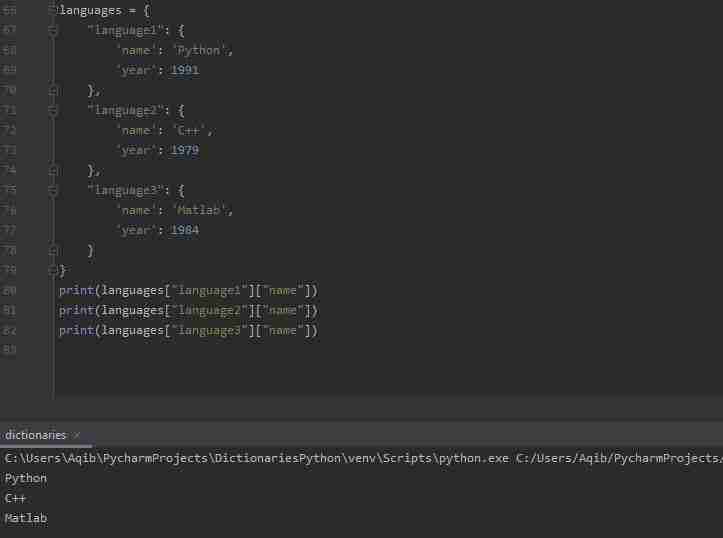
Swap Dictionary Keys And Values
There is a way to swap dictionary keys and values.
If we have two keys with the same values, then after swapping, the key will be the value, and the values will be a list of keys of the original dictionary.
Let’s take a look at the example code and output.
# initializing a dictionary
original_dictionary = {'a': 0, 'b': 1, 'c': 2, 'd': 3, 'e': 4, 'f': 5, 'g': 5, 'h': 6}
# Printing original dictionary
print(f"Original dictionary is : {original_dictionary}")
new_dict = {}
for key, value in original_dictionary.items():
if value in new_dict:
new_dict[value].append(key)
else:
new_dict[value] = [key]
# Printing new dictionary after swapping keys and values
print("Dictionary after swapping is : ")
for i in new_dict:
print(i, " :", new_dict[i])
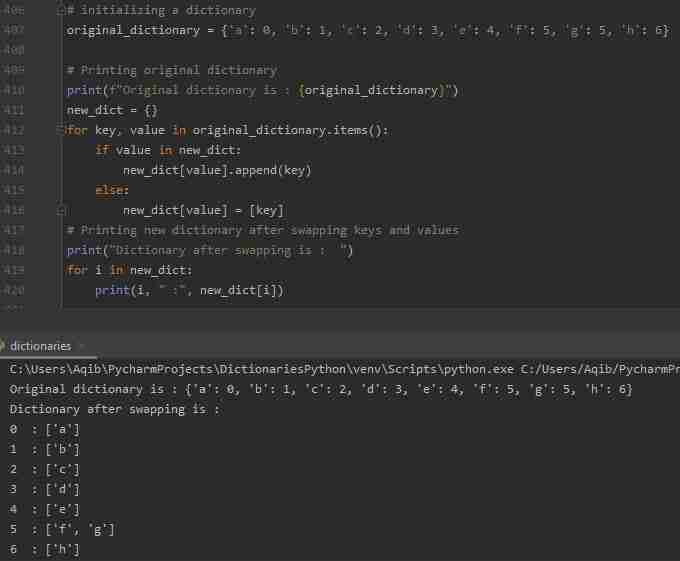
Rename A Dictionary Key
If a user wants to rename a key by preserving the value, this can be done using the pop()
method.
Let’s take a look at the example code and output.
# Initialization of a dictionary
dictionary = {"name": 'Python', "creation": 1991, "is_python": True}
newKey = "year"
oldKey = "creation"
dictionary[newKey] = dictionary.pop(oldKey)
print(dictionary)
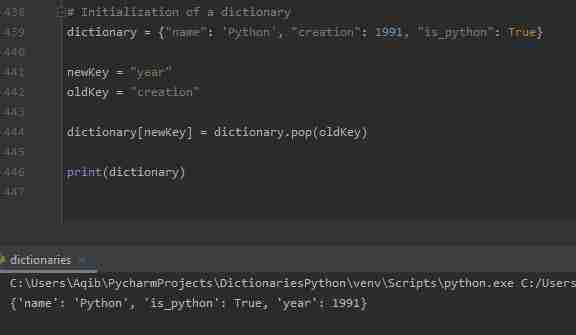
Reverse Dictionary Lookup
A reverse dictionary lookup returns a list containing each key in the dictionary that maps to a specified value.
dict.items()
is used to do a reverse dictionary lookup.
We will use for loop to iterate over each key:value
pair.
If the lookup value finds out, add the corresponding key to an empty list.
Let’s take a look at the example code and output.
# Initialization of a dictionary
dictionary = {'a': 0, 'b': 1, 'c': 2, 'd': 3, 'e': 1, 'f': 5, 'g': 6, 'h': 1}
# Value to look for
lookup_value = 1
# A list which will contain found keys
all_keys = []
# Procedure to find keys against the value
for key, value in dictionary.items():
if value == lookup_value:
all_keys.append(key)
print(all_keys)
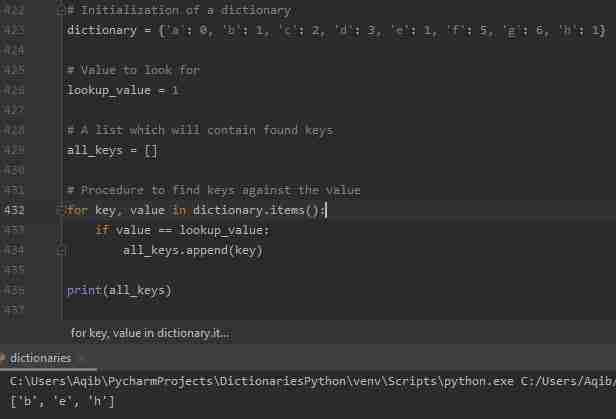
Convert Dictionary To JSON
Dictionary can be converted to JSON objects using python’s built-in package called json
.
To use this package, we have to import json
in our script, as shown below.
The functions used to convert a python dictionary into a JSON object are json.dumps() and json.dump().
json.dumps()
The syntax to use this function is:
json.dumps(your_dictionary, indent)
Parameters:
your_dictionary
– name of a dictionary that should be converted to a JSON objectindent
– defines the number of units for indentation
import json
your_dictionary = {
"name": "Python",
"created": 1991,
"language": True
}
# Serializing json
jsonObject = json.dumps(your_dictionary, indent=1)
print(jsonObject)
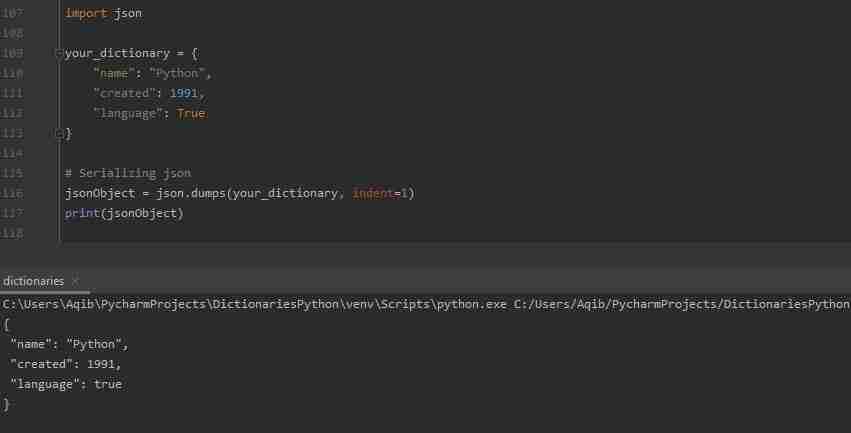
json.dump()
The syntax to use this function is:
json.dump(your_dictionary, filePointer)
Parameters:
your_dictionary
– name of a dictionary which should be converted to a JSON objectfilePointer
– pointer of the file opened in write or append mode.
import json
your_dictionary = {
"name": "Python",
"created": 1991,
"language": True
}
with open("DictionaryToJSON.json", "w") as outfile:
json.dump(your_dictionary, outfile)
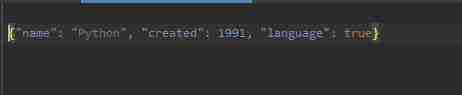
Literal Vs. Constructor
We can create a dictionary in two ways either by using literal, {}
, or by using a constructor, dict()
.
The literal is preferred over the constructor because it is faster.
The constructor is slower because it creates the object by calling the dict()
function.
On the other hand, the compiler turns the dictionary literal into BUILD_MAP
bytecode, saving the function call.
The code and output below demonstrate that the literal is much faster than the constructor.
import timeit
constructor_result = timeit.timeit(stmt='dict(one=1, two=2)')
print(f"Time taken by constructor --> {constructor_result}")
literal_result = timeit.timeit(stmt='{"one": 1, "two": 2}')
print(f"Time taken by literal --> {literal_result}")
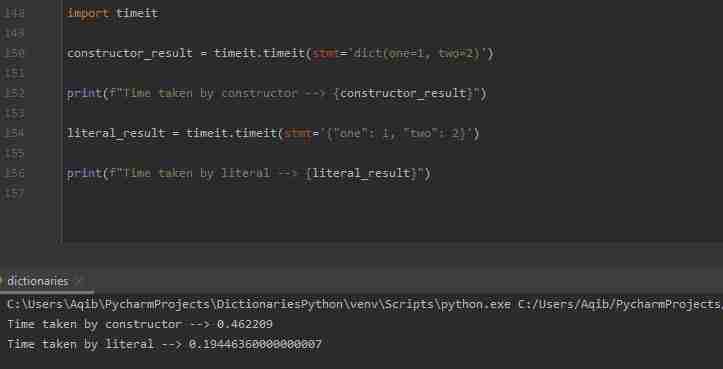
Difference Between Dictionary And List
List | Dictionary |
---|---|
A list is an ordered sequence of objects | Dictionary is an unordered collection of data values |
A single list may contain data types like Integers, Strings, as well as Objects | Dictionary holds key:value pair. Key can only be of type string, number, or tuple, and value can be of any type |
Items in lists are accessed via their position/index only | Items in dictionaries are accessed via keys and not via their position |
Dictionary Comprehension
Python allows dictionary comprehension, the same as that list comprehension.
We can also use dictionary comprehension to create dictionaries in a very brief way.
A dictionary comprehension takes the form – { key: value for (key, value) in iterable }
.
# List to represent keys
keys = ['a', 'b', 'c', 'd', 'e']
# List to represent values
values = [1, 2, 3, 4, 5]
# Dictionary comprehension
compDict = {key: value for (key, value) in zip(keys, values)}
print(compDict)
What is the zip() Function In Python Dictionary
In the above code zip()
function is used.
The zip()
function takes iterables, aggregates them in a tuple, and returns them.
The output of the above code is shown below.
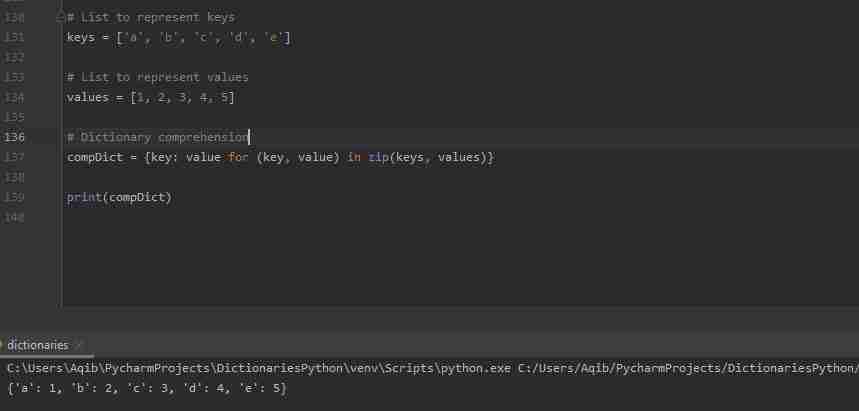
The above can also be done using dict(zip(keys, values))
.
The code and output are shown below.
# List to represent keys
keys = ['a', 'b', 'c', 'd', 'e']
# List to represent values
values = [1, 2, 3, 4, 5]
# Dictionary comprehension
compDict = dict(zip(keys, values))
print(compDict)
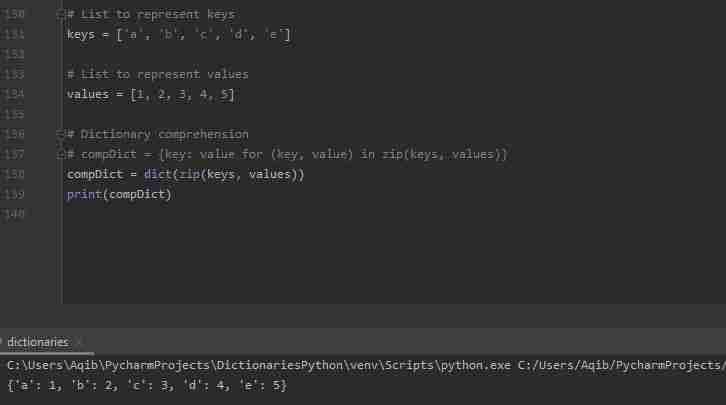
Let us look into another example that shows how dictionary comprehension works. Suppose we have a list of numbers. We will return the dictionary whose keys will be the numbers in the list, and values will be half the numbers in the list.
# Initializing a list of numbers
list_of_numbers = [2, 4, 6, 8, 10]
dict_Out = {x: x / 2 for x in list_of_numbers}
print(dict_Out)
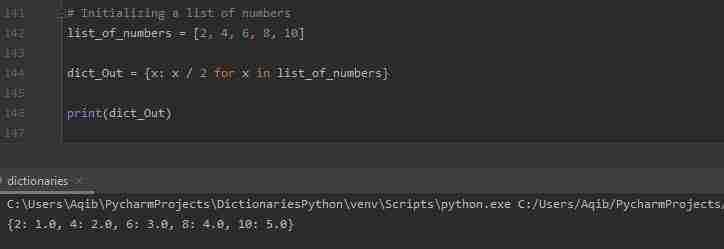
Dictionary And Yield Keyword
What is a yield?
The yield keyword in python works like a return. Instead of returning a value, it returns a generator object to the caller.
When a function is called, and the thread of execution finds a yield keyword in the function, the function execution stops at that line itself and returns a generator object to the caller.
What are Generators?
Generators are functions that return an iterable generator object. The values from the generator object are fetched one at a time instead of the full list together, and hence to get the actual values you can use a for-loop using the next() or list() method.
Generate A Dictionary From Yield
# Generator function
def generator_function():
yield 'a', 1
yield 'b', 2
# Returning a dictionary
def func(): return dict(generator_function())
print(func())
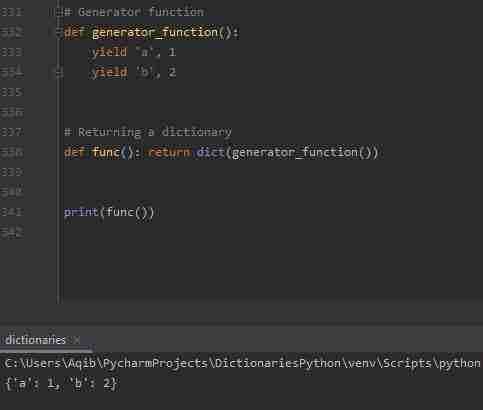
Pretty Print (Beautify) A Dictionary
To make the dictionary more readable, there is a Python module pprint()
which provides the capability to pretty print a dictionary.
In the example, we will use an array of dictionaries and would print the array using pprint()
.
# Initializing an array of dictionaries
dictionary_arr = [
{'Language': 'Python', 'year': 1991, 'is_python': True},
{'Language': 'C++', 'year': 1981, 'is_python': False},
{'Language': 'Matlab', 'year': 1984, 'is_python': False},
{'Language': 'R', 'year': 1995, 'is_python': False}
]
pprint.pprint(dictionary_arr)
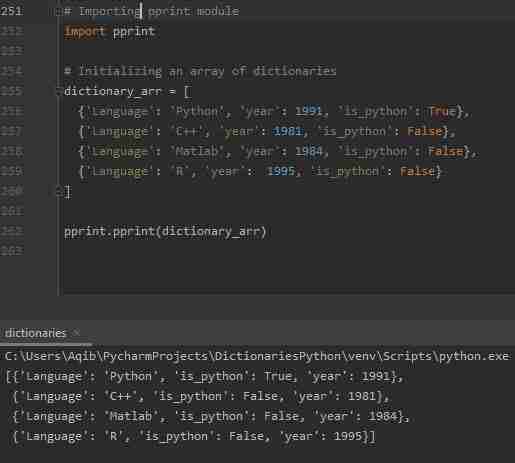
Convert Dictionary to String
The str()
function converts the dictionary into a string.
# Initializing a dictionary
dictionary = {
"name": "Python",
"created": 1991,
"language": True
}
#Converting dictionary into string
converted_dict = str(dictionary)
print(converted_dict)
print(type(converted_dict))
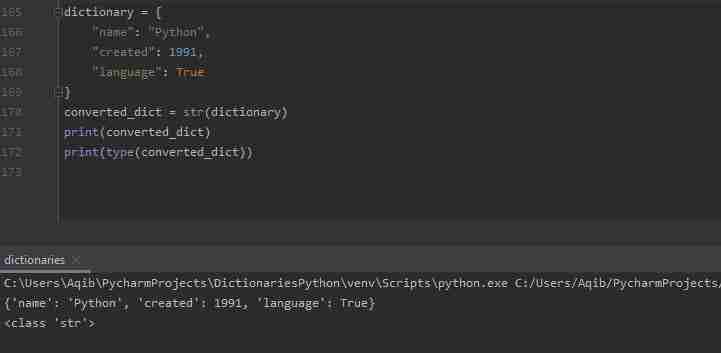
Copy/Save the Dictionary As CSV
CSV (comma-separated values) files are used to transfer data, in the form of a string, especially to any spreadsheet program like Microsoft Excel or Google spreadsheet.
Python dictionary can be copied/saved as a CSV file using Python’s in-built CSV module.
The code demonstrating how to save a dictionary as CSV is given below.
#import in-built csv module
import csv
# Initializing a dictionary
cars = [
{'No': 1, 'Company': 'Ferrari', 'Car Model': '488 GTB'},
{'No': 2, 'Company': 'Porsche', 'Car Model': '918 Spyder'},
{'No': 3, 'Company': 'Bugatti', 'Car Model': 'La Voiture Noire'},
{'No': 4, 'Company': 'Rolls Royce', 'Car Model': 'Phantom'},
{'No': 5, 'Company': 'BMW', 'Car Model': 'BMW X7'},
]
# We require 3 Columns in CSV file because the dictionary we initialized has 3 key:value pairs
csv_fields = ['No', 'Company', 'Car Model']
# Code to write a dictionary as CSV file
with open('Names.csv', 'w') as csvFile:
writer = csv.DictWriter(csvFile, fieldnames=csv_fields)
writer.writeheader()
writer.writerows(cars)
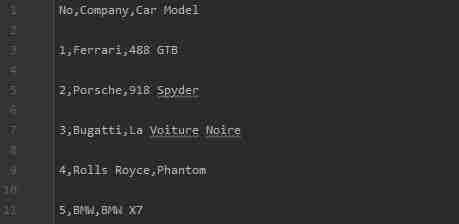
Convert Dictionary To List Of Tuples
There are several methods by which we can convert a dictionary to a list of tuples.
The code and output for each method are given below.
# Initializing a dictionary
dictionary = {'name': 'Python', 'year': 1991, 'is_language': True}
# Method to convert dictionary into list of tuple
converted_list = [(key, value) for key, value in dictionary.items()]
print(converted_list)
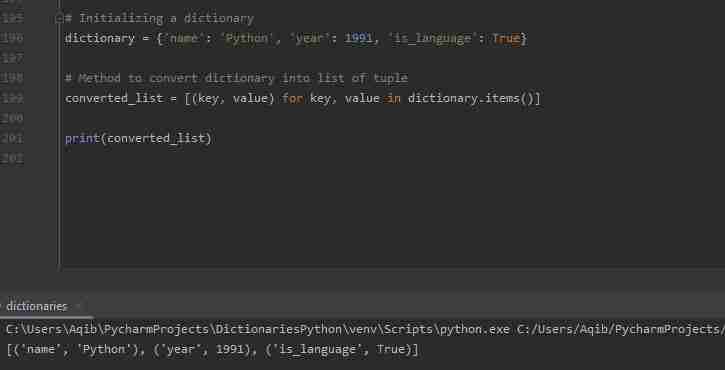
# Initializing a dictionary
dictionary = {'name': 'Python', 'year': 1991, 'is_language': True}
# Method to convert dictionary into list of tuple
converted_list = list(dictionary.items())
print(converted_list)
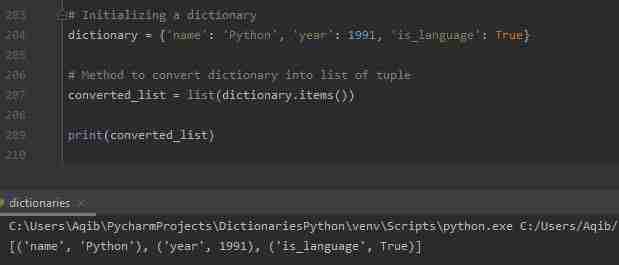
# Initializing a dictionary
dictionary = {'name': 'Python', 'year': 1991, 'is_language': True}
# Using zip function to convert dictionary into list of tuples
converted_list = zip(dictionary.keys(), dictionary.values())
converted_list = list(converted_list)
print(converted_list)
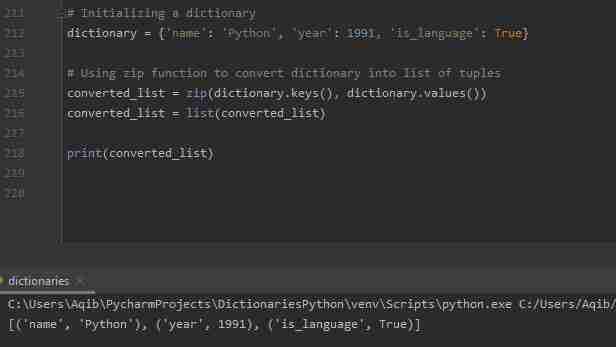
# Initializing a dictionary
dictionary = {'name': 'Python', 'year': 1991, 'is_language': True}
converted_list = []
# Iteration
for i in dictionary:
k = (i, dictionary[i])
converted_list.append(k)
print(converted_list)
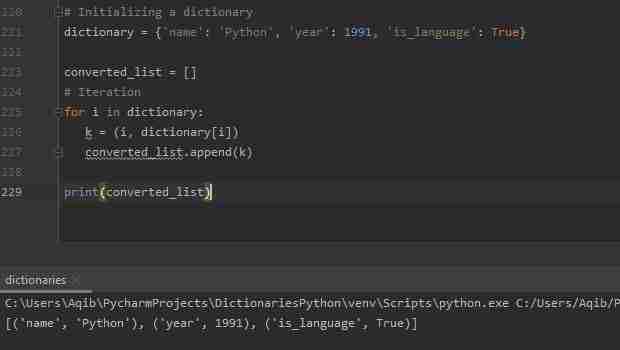
Convert Dictionary To XML
A module dicttoxml
can be used to convert a dictionary into an XML string.
The module can be installed using:
pip install dicttoxml
The example code and output are given below.
# Importing dicttoxml module
import dicttoxml
# Importing pprint for pretty printing
import pprint
# Initialization of dictionary
dictionary = {
'name': 'Python',
'language': True,
'year': 1991,
}
# Converting dictionary to XML string
xmlString = dicttoxml.dicttoxml(dictionary)
pprint.pprint(xmlString.decode())
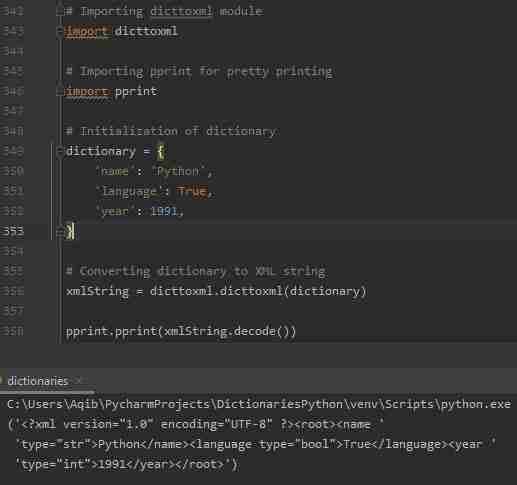
Convert Dictionary To Bytes
Python dictionary can be converted to bytes and vice versa. To achieve this, we have to import json
module.
The code and output are given below.
# initializing dictionary
dictionary = {'name': 'Python', 'year': 1991, 'is_language': True}
# Convert to bytes
to_bytes = json.dumps(dictionary).encode('utf-8')
# printing type and binary dict
print(f"Bytes --> {to_bytes}")
print(f"Type is --> {type(to_bytes)}")
# Convert bytes back to dictionary
to_dict = json.loads(to_bytes.decode('utf-8'))
# printing type and dict
print("**********************")
print(f"Dictionary --> {to_dict}")
print(f"Type is --> {type(to_dict)}")
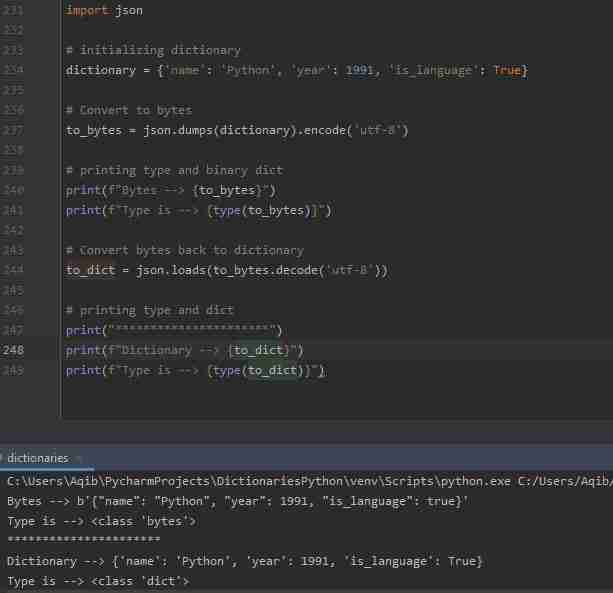
Dictionary And Sets
Python provides a lot of flexibility to handle different types of data structures.
There may be a need to convert one data structure to another for better use or analysis of the data.
Sets can be converted to a dictionary by using different methods.
The code and output for all such methods are given below.
# Initialization of Set 1
set1 = {1, 2, 3, }
# Initialization of Set 2
set2 = {'a', 'b', 'c', 'd'}
# Converting Sets to a dictionary
dictionary = dict(zip(set1, set2))
print(dictionary)
print(type(dictionary))
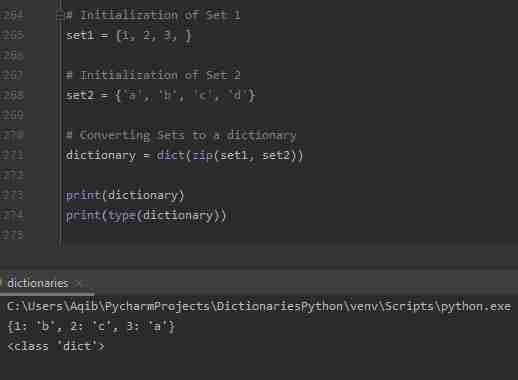
# Initialization of Set
set1 = {'a', 'b', 'c', 'd'}
# Converting Set to a dictionary
dictionary = dict.fromkeys(set1, 'Alphabet')
print(dictionary)
print(type(dictionary))
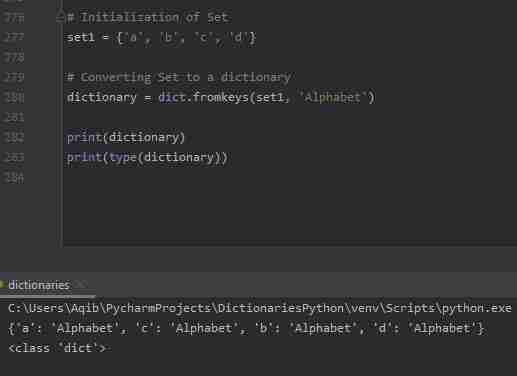
# Initialization of Set
set1 = {'a', 'b', 'c', 'd'}
# Converting Set to a dictionary
dictionary = {element: f'{element} is aplhabet' for element in set1}
print(dictionary)
print(type(dictionary))
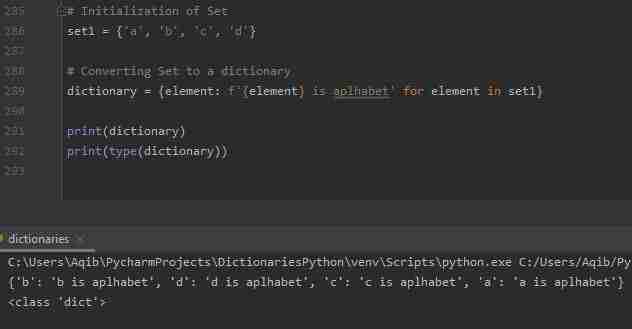
Dictionary And YAML
The dictionary can be converted to YAML and vice versa.
For this reason, we have to install a package pyYAML
:
pip3 install pyYAML
After installation of the package, we have to import yaml
module into our script.
The example code and output are given below.
# importing yaml
import yaml
# Initialization of Dictionary
dictionary = {
'name': 'Hands-on.cloud',
'languages': ['Python', 'Only Python', ],
'Other': {
'Linux': True,
'Docker': True,
},
}
# Converting dictionary into yaml stream and printing it
print(yaml.dump(dictionary, sort_keys=False))
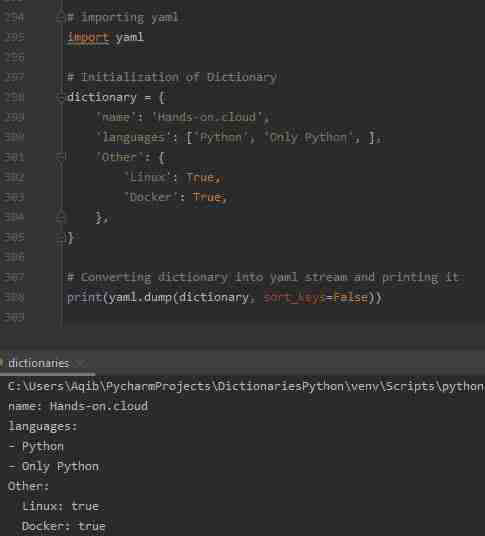
Now we will learn how to convert the YAML string into Python Object, i.e., a dictionary.
# Initialization of YAML string
yString = """
---
Pakistan:
capital: Islamabad
USA:
capital: Washington, D.C.
list_of_countries:
- Pakistan
- USA
"""
# Converting yaml stream into dictionary
dictionary = yaml.load(yString, Loader=yaml.SafeLoader)
print(dictionary)
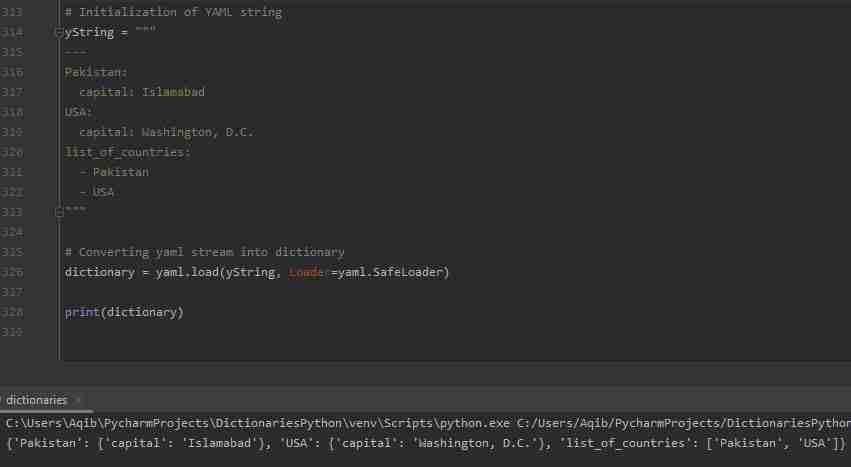
Convert Dictionary To Dataframe
Python dictionary can be converted to Pandas Dataframe by using class-method pandas.DataFrame.from_dict().
import pandas as pd
# Initializing a dictionary
dictionary = {'languages': ['Python', 'C++', 'Matlab', 'R'],
'Number of letters': ['6', '3', '6', '1']}
# Converting dictionary to Dataframe
data = pd.DataFrame.from_dict(dictionary)
print(data)
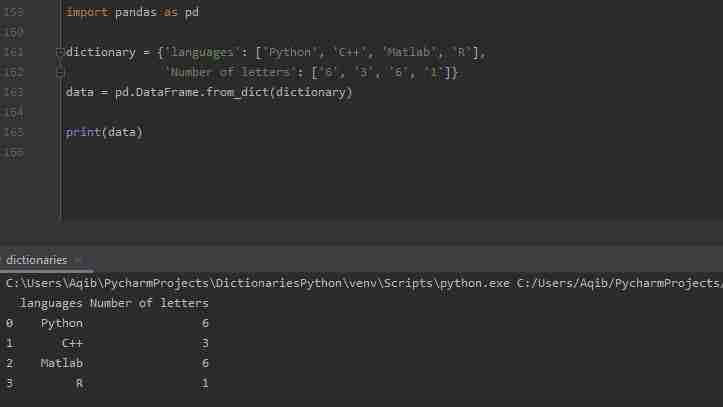
Creating Dictionary From Excel Data Using xlrd
We will learn how to create a dictionary from excel data. We have first to install xlrd
module using:
pip install xlrd
The Excel file in this example contains two rows and two columns.
The example code and output are given below.
import xlrd
dictionary = {}
wb = xlrd.open_workbook('ExcelToDictionary.xlsx')
sh = wb.sheet_by_index(0)
for i in range(2):
cell_value_class = sh.cell(0, i).value
cell_value_id = sh.cell(1, i).value
dictionary[cell_value_class] = cell_value_id
print(dictionary)
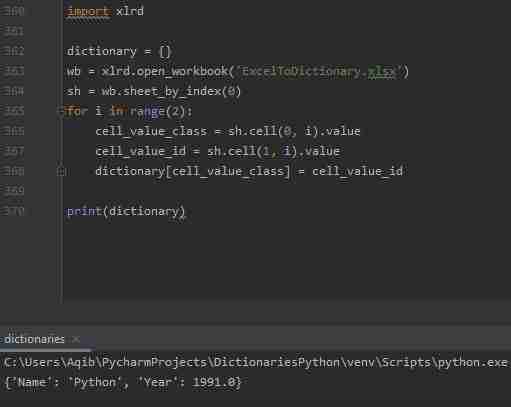
Create Excel File From Dictionary Using xlsxwriter
The dictionary can be saved as an Excel file.
The package used in this regard is xlsxwriter
.
Install this package using:
pip install xlsxwriter
Now, here’s an implementation example:
import xlsxwriter
workbook = xlsxwriter.Workbook('Excel_From_Dictionary.xlsx')
worksheet = workbook.add_worksheet()
my_dict = {'Bob': [10, 11, 12],
'Ann': [20, 21, 22],
'May': [30, 31, 32]}
col_num = 0
for key, value in my_dict.items():
worksheet.write(0, col_num, key)
worksheet.write_column(1, col_num, value)
col_num += 1
workbook.close()
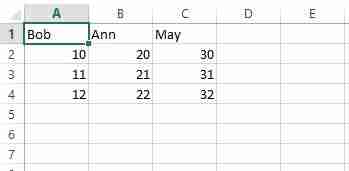
Dictionary Packing & Unpacking
Dictionary can be packed and unpacked using **
operator.
Dictionary Packing
def dictionary_func(**kwargs):
# Printing dictionary items
for key in kwargs:
print("%s = %s" % (key, kwargs[key]))
dictionary_func(name="Python", year=1991, is_python=True)

Dictionary Unpacking
def multiply(a, b):
print(a*b)
dictionary = {'a': 4, 'b': 5}
multiply(**dictionary)
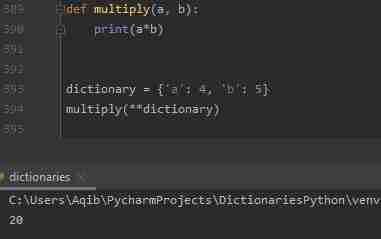
Built-in Dictionary Functions & Methods
Functions
str(anyDict)
: Produces a printable string representation of a dictionary.type(variable)
: In python, the functiontype()
returns the type of the passed variable. If a passed variable is a dictionary, then it would return a dictionary type.len(anyDict)
: Gives the number of items in the dictionary.cmp(anyDict1, anyDict2)
: Compares elements ofanyDict1
andanyDict2
.
Methods
anyDict.fromkeys()
: Creates a new dictionary with keys from seq and values set to value.anyDict.clear()
: Removes all elements of dictionary anyDict, and anyDict will be an empty dictionaryanyDict.copy()
: Returns a shallow copy of dictionary anyDictanyDict.keys()
: Returns list of dictionary anyDict’s keysanyDict.items()
: Returns a list of anyDict’s (key, value) tuple pairsanyDict.get(key, default=None)
: Returns value against the passed key or default if the key is not in the dictionaryanyDict.has_key(key)
: Returns true if the key is in dictionary anyDict, otherwise falseanyDict.values()
: Returns list of dictionary anyDict’s valuesanyDict.update(anyDict2)
: Adds dictionary anyDict2’s key-values pairs to anyDict