Mastering Machine Learning Modeling: A Complete Guide
Are you eager to unlock the potential of Machine Learning modeling? This comprehensive guide will give you everything you need about this cutting-edge technology. Whether a novice or an experienced data scientist, you’ll find insightful information about Machine Learning, the models used, and how to create them for your projects. Machine Learning modeling creates algorithms that learn from and make decisions or predictions based on data. It’s a critical aspect of data science and artificial intelligence, playing a significant role in various applications, from self-driving cars to personalized product recommendations.
This article explores Python’s critical role in Machine Learning modeling, its libraries, and how to code a Machine Learning model. Furthermore, we’ll delve into AWS SageMaker, a powerful platform that simplifies the process of building, training, and deploying Machine Learning models at scale. With real-world case studies, we’ll demonstrate Python and SageMaker in action. From understanding common challenges to sharing best practices, we’ll cover all facets of Machine Learning modeling, equipping you with the knowledge to harness its power effectively. Get ready to dive into the world of Machine Learning modeling!
Introduction to Machine Learning Modeling
Machine Learning modeling has emerged as a critical player in a world increasingly driven by data, transforming how we approach problem-solving and decision-making. Machine Learning models are the engines behind these intelligent systems, from powering recommendation systems on our favorite online platforms to detecting fraudulent transactions in financial institutions.
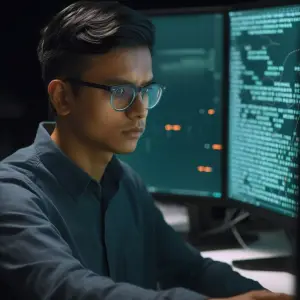
But what exactly is Machine Learning modeling?
Machine Learning modeling involves teaching a computer to recognize patterns and make predictions or decisions without being explicitly programmed. Instead, these models learn from data. The more high-quality data they have to learn from, the better their ability to make accurate predictions.
For instance, if we wanted to develop a Machine Learning model to predict the likelihood of customer churn in a telecom company, we would first need to feed our model with historical customer data. This data might include customer usage patterns, payment history, service issues, etc. Based on these inputs, our model would then learn the patterns that indicate a higher likelihood of customers discontinuing their service.
The versatility of Machine Learning modeling has been further enhanced by cloud-based platforms such as AWS SageMaker. SageMaker provides a robust and scalable environment to build, train, and deploy Machine Learning models. It abstracts away much of the complexity associated with Machine Learning modeling, making it more accessible to a broader audience.
For instance, let’s consider our earlier example of predicting customer churn. In a traditional Machine Learning setting, deploying such a model would involve several complex steps – from setting up the server infrastructure to implementing APIs that can interact with the model. With SageMaker, however, much of this process is simplified. Once your model is trained using Python in a Jupyter Notebook on SageMaker, it can be deployed with a single line of code:
predictor = model.deploy(initial_instance_count=1, instance_type='ml.m4.xlarge')
In this line, ‘model’ refers to the model we have trained, and ‘deploy’ is a method SageMaker provides to deploy the model. The parameters ‘initial_instance_count’ and ‘instance_type’ specify the number of instances we want for our model and the instance type, respectively.
This blog post aims to provide a comprehensive understanding of Machine Learning modeling, from the basic principles to the practical application using Python and AWS SageMaker. Let’s delve into the fascinating world of Machine Learning modeling and explore how it’s changing the technology and business landscape.
Understanding the Basics of Machine Learning
Before we delve into the specifics of Machine Learning modeling, it’s essential to understand some basic concepts that underpin this exciting field.
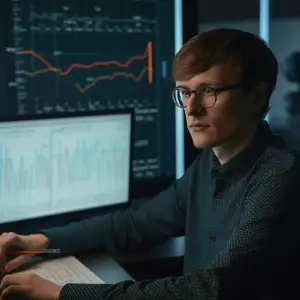
What is Machine Learning?
Machine Learning is a branch of artificial intelligence (AI) that empowers computers to learn from data and improve their performance over time without being explicitly programmed. It’s the science of making computers act intelligently by learning patterns and making decisions from data.
Key Concepts in Machine Learning
Several critical concepts form the backbone of Machine Learning:
- Data: This is the information from which our Machine Learning models learn. The quality, quantity, and variety of data significantly impact the performance of our models.
- Features: These are the variables or attributes in our data that our Machine Learning model uses to make predictions. For instance, in a customer churn prediction model, features might include customer age, tenure, service usage, etc.
- Models: A model is the mathematical representation of a real-world process. It’s a specific Machine Learning algorithm that’s been trained on data. For example, a decision tree or a neural network.
- Training: This is the process where our model learns from data. It involves providing our model with inputs (features) and desired outputs (labels) so the model can learn how to map the former to the latter.
- Evaluation: This process involves testing our model on new data (not used during training) to assess its performance.
- Prediction: Once a model is trained and evaluated, it can be used to predict outcomes for new, unseen data.
As a powerful and versatile programming language, Python offers numerous libraries like scikit-learn, Pandas, and Numpy that simplify these steps and streamline the Machine Learning process.
AWS SageMaker further simplifies this process by providing a fully managed platform to build, train, and deploy Machine Learning models. Using SageMaker’s built-in Jupyter notebooks, we can easily write Python code to load and process our data, select and train our models, and evaluate their performance. SageMaker also has built-in algorithms that can be used for model training, saving the hassle of coding algorithms from scratch.
For example, to train a Machine Learning model on SageMaker, you would need to specify the type of estimator (algorithm) you want to use and then call its ‘fit’ method:
from sagemaker import get_execution_role
from sagemaker.amazon.amazon_estimator import get_image_uri
from sagemaker.estimator import Estimator
# Specify the AWS image URI for the algorithm you want to use
image_uri = get_image_uri(boto3.Session().region_name, 'xgboost')
# Define the estimator
estimator = Estimator(image_uri,
get_execution_role(),
instance_count=1,
instance_type='ml.m5.2xlarge',
output_path='s3://{}/{}/output'.format(bucket, prefix),
sagemaker_session=sagemaker.Session())
# Fit the estimator to the training data
estimator.fit({'train': s3_input_train})
In this example, we’re using SageMaker’s implementation of the XGBoost algorithm to train our model. The ‘fit’ method uses the specified training data to trigger the training process.
In the next sections, we’ll delve deeper into the steps involved in creating Machine Learning models, focusing specifically on how we can leverage Python and SageMaker to simplify and optimize this process.
Exploring the Types of Machine Learning Models
As you venture further into Machine Learning, you’ll encounter various models designed to address different problems. While no one-size-fits-all model is perfect for all tasks, understanding the different Machine Learning models can help you select the best approach for your problem.
Supervised Learning
In supervised learning, we train our model on a labeled dataset, meaning that each data point in our training set includes both the input features and the correct output (label). The model aims to learn a mapping from inputs to outputs and use this to predict the output for new, unseen inputs. Common supervised learning algorithms include linear regression, logistic regression, support vector machines, and decision trees.
Unsupervised Learning
Unsupervised learning models learn from unlabeled data. Instead of predicting an output, these models typically aim to discover interesting patterns or structures in the data. Common tasks in unsupervised learning include clustering (grouping similar data points) and dimensionality reduction (simplifying the data without losing too much information). Examples of unsupervised learning algorithms include K-means clustering and principal component analysis (PCA).
Reinforcement Learning
In reinforcement learning, an agent learns to perform actions in an environment to maximize a reward signal. It’s a trial-and-error approach where the model learns from past actions and their outcomes. Reinforcement learning has been used successfully in various tasks that involve sequential decision-making, such as gaming and robotics.
AWS SageMaker offers built-in implementations for various Machine Learning models, from linear regression for supervised learning to K-means for unsupervised learning. Using SageMaker’s Python SDK, you can easily train and deploy these models. For instance, to train a K-means model on SageMaker, you would use the following code:
from sagemaker import KMeans
# Specify the number of clusters
num_clusters = 10
# Define the KMeans estimator
kmeans = KMeans(role=get_execution_role(),
instance_count=1,
instance_type='ml.m5.xlarge',
output_path='s3://{}/{}/output'.format(bucket, prefix),
k=num_clusters)
# Fit the estimator to the training data
kmeans.fit(kmeans.record_set(train_set))
In the following sections, we will look at the step-by-step process of creating Machine Learning models, focusing on supervised learning models, which are the most commonly used in Machine Learning. We’ll explore using Python and SageMaker to streamline this process and create powerful, efficient Machine Learning models.
Step-by-Step Guide to Creating Machine Learning Models
Creating an effective Machine Learning model involves a series of steps, each with significance and role in the overall process. We will break it down into four main stages: Data Collection, Data Preprocessing, Model Selection, Training, and Evaluation.
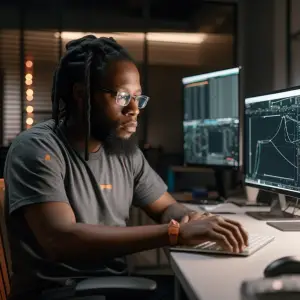
Data Collection
The first step in creating a Machine Learning model is gathering data. The quality and quantity of data directly impact how well your model will perform. Depending on your project, you might collect data from various sources such as databases, APIs, web scraping, or pre-existing datasets.
In Python, libraries like pandas make loading data from numerous sources straightforward. You can use the following code to load a CSV file into a pandas DataFrame, which is a 2-dimensional labeled data structure:
import pandas as pd
# Load data from a CSV file
df = pd.read_csv('path/to/your/data.csv')
Data Preprocessing
Once you’ve gathered your data, the next step is data preprocessing, which involves cleaning and transforming your data to prepare it for your Machine Learning model. This might involve handling missing values, encoding categorical variables, normalizing numerical variables, and more.
Python, with its wide array of libraries, simplifies this task. For example, you can use scikit-learn’s SimpleImputer
to fill in missing values:
from sklearn.impute import SimpleImputer
# Define our imputer
imputer = SimpleImputer(missing_values=np.nan, strategy='mean')
# Fit the imputer to the data
imputer.fit(df)
# Transform the data
df_imputed = imputer.transform(df)
Model Selection
Next, you must choose which Machine Learning model or algorithm to use. The choice depends on the nature of your task (e.g., classification, regression, clustering), the data’s characteristics, and the trade-offs you’re willing to make (e.g., accuracy vs speed).
With AWS SageMaker, you have numerous built-in algorithms, such as XGBoost, linear learner, K-means, and more. Using the SageMaker Python SDK, you can specify your algorithm of choice as follows:
from sagemaker.amazon.amazon_estimator import get_image_uri
from sagemaker.estimator import Estimator
# Specify the AWS image URI for the algorithm you want to use
image_uri = get_image_uri(boto3.Session().region_name, 'xgboost')
# Define the estimator
estimator = Estimator(image_uri,
get_execution_role(),
instance_count=1,
instance_type='ml.m5.2xlarge',
output_path='s3://{}/{}/output'.format(bucket, prefix),
sagemaker_session=sagemaker.Session())
Training and Evaluation
The final step involves training your selected model on your preprocessed data and evaluating its performance. Training involves feeding your data into the model so that it can learn from it, while evaluation involves testing your model on new data to assess how well it has learned.
In SageMaker, you can train and deploy your model using the fit
and deploy
methods, as shown below:
# Fit the estimator to the training data
estimator.fit({'train': s3_input_train})
# Deploy the model
predictor = estimator.deploy(initial_instance_count=1, instance_type='ml.m4.xlarge')
In the following sections, we’ll delve deeper into Python’s capabilities for Machine Learning modeling and how AWS SageMaker enhances this process, making it more streamlined and efficient.
Deep Dive into Python for Machine Learning Modeling
Python’s simplicity and extensive library ecosystem have made it a premier language for Machine Learning. In this section, we will explore some of Python’s key libraries for Machine Learning and walk through coding a Machine Learning model.
Python Libraries for Machine Learning
Several Python libraries play a crucial role in Machine Learning modeling. Here are a few notable ones:
- NumPy: This library supports large multidimensional arrays and matrices and a collection of mathematical functions to operate on these arrays.
- Pandas: Pandas is an open-source library providing high-performance, easy-to-use data structures, and analysis tools. It’s perfect for manipulating and analyzing data.
- Matplotlib & Seaborn: Both libraries are for data visualization, allowing you to create graphs and plots to understand data better.
- Scikit-Learn: Scikit-learn is a simple and effective data mining and analysis tool. It includes various classification, regression, and clustering algorithms and is built on NumPy, SciPy, and matplotlib.
- TensorFlow & PyTorch: These two are popular libraries for deep learning, a Machine Learning subfield focusing on neural networks.
Coding a Machine Learning Model in Python
Now, let’s walk through the coding of a Machine Learning model in Python. We’ll use Scikit-Learn to create a simple linear regression model:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Assume 'df' is a pandas DataFrame with features 'X' and target 'y'
X = df.drop('target_column', axis=1)
y = df['target_column']
# Split data into training set and test set
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Define the model
model = LinearRegression()
# Train the model
model.fit(X_train, y_train)
# Make predictions on the test set
predictions = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, predictions)
print('Mean Squared Error:', mse)
While Scikit-Learn is excellent for prototyping and small-scale projects, AWS SageMaker would better fit larger projects requiring more computational resources. In SageMaker, you can use a Jupyter Notebook to write your Python code and leverage SageMaker’s scalable infrastructure and built-in algorithms to train and deploy your models. The process of building a model in SageMaker has been illustrated in the previous sections.
As we proceed, we will introduce more tools and techniques for Machine Learning modeling using Python and AWS SageMaker, which will open up new avenues and possibilities for your projects.
Introduction to AWS SageMaker
AWS SageMaker is a fully managed service that allows developers and data scientists to quickly build, train, and deploy Machine Learning (ML) models. It removes the heavy lifting from each step of the Machine Learning process to make it easier to develop high-quality models.
Why SageMaker for Machine Learning Modeling?
Here are some reasons why SageMaker is an excellent choice for Machine Learning modeling:
- Ease of use: SageMaker provides a fully managed environment, making it easy to build, train, and deploy ML models.
- Scalability: SageMaker handles all resource management, so you can easily scale your training jobs to multiple instances.
- Integration with Python: SageMaker offers a Python SDK, allowing you to write Python code for your Machine Learning models and deploy them seamlessly.
- Built-in Algorithms: SageMaker comes with numerous built-in algorithms you can use, saving you the time and effort to code these algorithms from scratch.
- Model Tuning: SageMaker Automatic Model Tuning helps find the best version of a model by adjusting hyperparameters.
- Versatility: SageMaker supports Machine Learning and deep learning frameworks like TensorFlow, PyTorch, and MXNet.
Setting up a Machine Learning Environment in SageMaker
To set up your SageMaker environment, follow these steps:
- Create a SageMaker notebook instance: Go to the SageMaker console in AWS and create a new notebook instance. This is a fully managed ML compute instance running the Jupyter Notebook App.
- Open Jupyter: Once your instance is ready, open Jupyter, and you’ll see an interface where you can create new notebooks and access existing ones.
- Start coding: You can create a new notebook and start writing your Python code. You can import data, preprocess it, visualize it, train models, and deploy models, all from within your SageMaker notebook.
Building and Deploying Models with SageMaker
Building and deploying models with SageMaker involves selecting an algorithm, providing data, training the model, and deploying it. Here is a simplified code example for this process using SageMaker’s Python SDK:
from sagemaker import get_execution_role
from sagemaker.amazon.amazon_estimator import get_image_uri
from sagemaker.estimator import Estimator
# Specify the AWS image URI for the algorithm you want to use
image_uri = get_image_uri(boto3.Session().region_name, 'xgboost')
# Define the estimator
estimator = Estimator(image_uri,
get_execution_role(),
instance_count=1,
instance_type='ml.m5.2xlarge',
output_path='s3://{}/{}/output'.format(bucket, prefix),
sagemaker_session=sagemaker.Session())
# Fit the estimator to the training data
estimator.fit({'train': s3_input_train})
# Deploy the model
predictor = estimator.deploy(initial_instance_count=1, instance_type='ml.m4.xlarge')
In the following sections, we’ll discuss working with SageMaker’s key features in more detail and provide more examples to help you get started with this powerful tool.
Case Studies: Real-world Machine Learning Modeling with Python & SageMaker
This section will review a few case studies showcasing the power of Machine Learning modeling using Python and SageMaker.
Predicting Customer Churn
In one example, a telecommunications company wanted to predict customer churn. They used AWS SageMaker’s built-in XGBoost algorithm to train a model on their customer data. The features included information like customer demographics, service usage patterns, and customer complaints. They preprocessed the data using Python in a SageMaker notebook, handled missing values, and encoded categorical variables.
After training the model, they evaluated its performance and deployed it to an endpoint. Once deployed, the model generated churn predictions for active customers, allowing the company to address customer dissatisfaction proactively.
Forecasting Energy Demand
In another example, an energy company needed to forecast energy demand. They collected data on past energy usage and relevant factors like weather and time of day. They used SageMaker’s DeepAR algorithm, specially designed for time series forecasting.
The company used the Python SDK to train the DeepAR model in SageMaker, passing in their time series data and specifying other necessary parameters. Once trained, the model was deployed to generate energy demand forecasts, helping the company optimize its energy production and distribution.
Recommending Products
An e-commerce company wanted to implement a recommendation system to suggest products to its customers. They used SageMaker’s Factorization Machines algorithm, which is great for tasks like a recommendation.
The company collected data on past purchases and customer browsing history. They used Python in a SageMaker notebook to preprocess this data and format it for the Factorization Machines algorithm. They trained the model in SageMaker and then deployed it. The deployed model generated product recommendations for customers, enhancing their shopping experience and increasing sales.
These case studies illustrate the power of Machine Learning modeling with Python and AWS SageMaker. The combination of Python’s versatility and SageMaker’s scalability and ease of use makes it easier to build and deploy Machine Learning models for various applications.
Common Challenges in Machine Learning Modeling and How to Overcome Them
While Machine Learning modeling can yield powerful results, it also comes with challenges. This section will explore some common challenges and discuss potential solutions.
Lack of Quality Data
Challenge: Often, the success of a Machine Learning model largely depends on the quality of data it’s trained on. The available data is sometimes sparse, unbalanced, or riddled with errors and missing values.
Solution: Data preprocessing methods in Python can be used to handle these issues. Missing values can be handled using imputation, and errors can be fixed during data cleaning. Techniques like oversampling, undersampling, or SMOTE can be employed for unbalanced datasets. AWS SageMaker also provides built-in algorithms like ‘Linear Learner’ that work well with imbalanced data.
Choosing the Right Model
Challenge: With many Machine Learning models available, selecting the one that best suits your data and problem can be challenging.
Solution: A sound understanding of different Machine Learning models, their assumptions, and their strengths and weaknesses can guide you in choosing the right model. SageMaker makes this easier by selecting built-in algorithms for specific Machine Learning tasks.
Overfitting
Challenge: Overfitting occurs when a Machine Learning model learns the training data too well and performs poorly on unseen data because it fails to generalize.
Solution: Techniques such as cross-validation, regularization, and early stopping can help prevent overfitting. Additionally, SageMaker provides automatic model tuning, which optimizes the model’s hyperparameters to prevent overfitting.
Scalability
Challenge: Training Machine Learning models on large datasets can be computationally intensive and time-consuming, especially on limited resources.
Solution: AWS SageMaker provides a scalable environment to train your models, no matter how large your datasets are. You can easily specify the number and type of instances you need for your training jobs, and SageMaker takes care of the rest.
Model Deployment and Management
Challenge: Deploying a Machine Learning model into a production environment and managing it can be daunting.
Solution: SageMaker simplifies the deployment process. With just a few lines of Python code, you can deploy your trained model to an endpoint, where it can be used to make predictions. SageMaker also allows for version control and easy updates to your deployed models, making model management straightforward.
By understanding these challenges and their solutions, you can improve the quality of your Machine Learning models and make your modeling process more efficient and effective.
Best Practices for Machine Learning Modeling
Machine Learning modeling is a complex process that involves many decisions and steps. Following best practices can guide this process and lead to better results. Here are a few to consider:
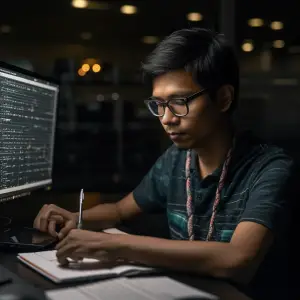
Understand the Problem and the Data
Before diving into modeling, take the time to understand the problem you’re trying to solve and the data you have available. Perform exploratory data analysis to understand the nature of your data, its quality, and its relevance to the problem.
Use Appropriate Data Preprocessing Techniques
Data preprocessing is a crucial step in the Machine Learning pipeline. Techniques like outlier detection, encoding categorical variables, imputing missing values, feature scaling, and feature engineering can drastically improve your model’s performance.
Split the Data
Splitting your data into a training and test set (and, optionally, a validation set) is essential. This allows you to assess how well your model generalizes to unseen data.
Choose the Right Model
Not all Machine Learning models are suited to all types of tasks. Be aware of the strengths and weaknesses of different models, and choose the most appropriate for your data and task. Remember, more complex models aren’t always better. A simpler model that’s easier to interpret might be more suitable, especially in the early stages of a project.
Validate and Fine-Tune Your Model
Use techniques like cross-validation to assess the robustness of your model and tune your model’s hyperparameters to optimize its performance. AWS SageMaker’s automatic model tuning feature can be highly beneficial for this.
Evaluate Your Model
Evaluate your model using appropriate metrics. Accuracy is not always the best metric; depending on the problem, you might want to consider others like precision, recall, F1-score, AUC-ROC, Mean Squared Error, etc.
Iterate
Machine Learning modeling is an iterative process. You might need to go back and forth between different steps, try different models, or rework your features. Don’t be afraid to experiment and iterate.
Plan production deployment
When you’re ready to deploy your model, consider the production environment. Will your model be used for batch predictions or real-time predictions? How will new data be handled? How often does the model need to be retrained? SageMaker provides features to simplify the deployment and management of your Machine Learning models.
Remember, these are general best practices. Depending on your specific problem and context, some might be more relevant. Following these practices can help you navigate the complex process of Machine Learning modeling and increase the likelihood of a successful project.
Conclusion: Harnessing the Power of Machine Learning Modeling
Machine Learning modeling is a powerful tool with the potential to revolutionize a wide range of domains, from healthcare and finance to entertainment and e-commerce. By learning how to build and deploy Machine Learning models effectively, you can leverage this power to solve complex problems, uncover insights, and create value in your work.
In this guide, we dove into the basics of Machine Learning, explored various Machine Learning models, and outlined a step-by-step process to create these models. We looked at how Python plays a vital role in Machine Learning modeling with its rich library and framework ecosystem. We also highlighted how AWS SageMaker simplifies the Machine Learning process, from development and training to deployment.
We examined real-world case studies of Machine Learning modeling with Python and SageMaker, discussed common challenges and ways to overcome them, and shared best practices for Machine Learning modeling.
The journey to mastering Machine Learning modeling is a continuous learning process. As new techniques and technologies emerge, there are always new things to learn and new ways to improve. But with a solid foundation in the basics, a practical understanding of the process, and the right tools at your disposal, you’re well on your way to harnessing the power of Machine Learning modeling.
FAQ
What is Modeling in machine learning?
What are the main 3 types of ML models?
What are the four models of machine learning?
What is the difference between machine learning and modeling?
References & Further Reading
Here are a few resources that will provide further insights and knowledge about machine learning modeling with Python and AWS SageMaker:
- Python for Data Analysis: This book by Wes McKinney provides a comprehensive introduction to data analysis with Python. It’s an excellent resource for understanding how to work with data in Python.
- Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow: This book by Aurélien Géron provides a practical guide to Machine Learning with Python. It covers a wide range of Machine Learning algorithms and techniques.
- AWS SageMaker Documentation: The SageMaker documentation is a thorough resource that provides detailed information about using SageMaker. Check out the Developer Guide for practical guidance and examples.
- Machine Learning on AWS: This page overviews AWS’s Machine Learning offerings, including SageMaker. It’s useful for understanding how Machine Learning fits into the broader AWS ecosystem.
- Scikit-Learn User Guide: The Scikit-Learn library is one of Python’s most widely used tools for Machine Learning. Their User Guide is excellent for understanding different Machine Learning models and techniques.
- Python Data Science Handbook: This book by Jake VanderPlas is an in-depth guide to the major Python tools used in data science, including NumPy, Pandas, Matplotlib, and Scikit-Learn.
- Deep Learning with Python: This book by François Chollet, the creator of Keras, provides a practical introduction to deep learning in Python.
By delving into these resources, you can deepen your understanding of Machine Learning modeling and gain new insights into leveraging these techniques. Happy learning!