Python List – Complete Tutorial
Python lists are one of the most commonly used data structures that are mutable (can be changed) and designed to store an ordered sequence of elements. As a Cloud Automation Engineer, you’ll use lists extensively in your Python scripts and Lambda functions. This article covers Python list methods, list indices, operations with list items, iterating over multiple elements, and comparing, sorting, and transforming operations.
Table of contents
What is a list in Python?
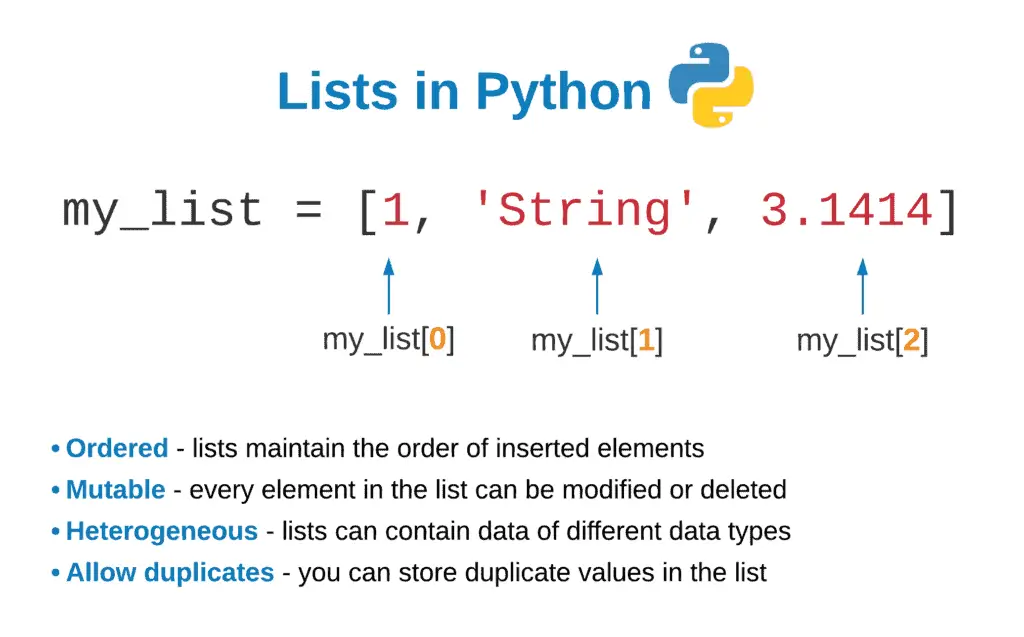
Python list is a mutable data structure that stores an ordered sequence of integers, strings, tuples, dictionaries, and other objects. Each element of a list is called an item. Lists in Python (and other programming languages) are defined by setting comma-separated items between square brackets [ ]
.
Python lists have the following characteristics:
- Ordered – lists maintain the order of inserted elements
- Mutable – every element in the list can be modified or deleted
- Heterogeneous – lists can contain data of different data types
- Allow duplicates – you can store duplicate values in the list
List vs. Tuple in Python
The difference between lists and tuples in Python is one of the most common questions on the internet.
Here’s a quick comparison:
List | Tuple |
---|---|
List is mutable | Tuple is immutable |
Iterations in the list are time-consuming | Iterations are much faster in tuples |
Consumes more memory | Consumes less memory |
Here’s some additional interesting information about the list and tuple differences.
List vs. Set in Python
The difference between lists and sets in Python is another common online question.
List | Set |
---|---|
The list is defined using square brackets [] | The set is defined using curly braces {} |
The list is an ordered collection of items | Set is an unordered collection of items |
Items in the list can be replaced or changed | Items in the set cannot be changed or replaced |
The list can store any data type | Set can store only immutable objects |
List vs. Dictionary in Python
The difference between lists and dictionaries in Python is also a popular online question.
List | Dictionary |
---|---|
A list is an ordered sequence of objects | A dictionary is an unordered collection of objects |
A list may contain any data types | The dictionary holds key:value pairs. The key can be of type string, number, or tuple. The value can be of any type. |
Items in lists are accessed by their index. | Items in dictionaries are accessed by keys and not by their position. |
How to create a list in Python?
You can create a Python list by putting comma-separated values inside square brackets []
. Items in a list do not have to be of the same type. The items can be integers, floats, strings, tuples, dictionaries, lists, and other objects. For the most efficient learning process, we strongly suggest you copy all Python code examples and execute them in the Python interpreter installed on your laptop.
Here’s an example of creating an empty Python list:
# Initialization of an empty list
empty_list = []
print(f"An empty list --> {empty_list}")
In the following example, we’ll create a list containing integer values:
list_integers = [1, 2, 3, 4]
print(f"A list with integers only --> {list_integers}")
Here’s a list of strings:
list_strings = ['String 1', 'String 2', 'String 3']
print(f"A list with strings only --> {list_strings}")
And finally, let’s define a list with values of different data types:
# A list containing integer, string, and float
list_mixed = [1, 'String', 3.1414]
print(f"A list containing mixed data types --> {list_mixed}")
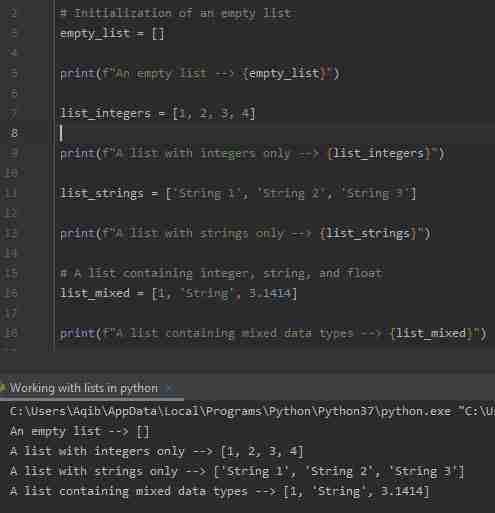
Accessing list items in Python
List items are indexed and can be accessed by referring to the index number. The index operator []
is used to access an item in a list. The index value must be a positive or negative integer number. Python uses zero-based indexing. That means the first element in the list has an index of 0, the second value has an index of 1, and so on.
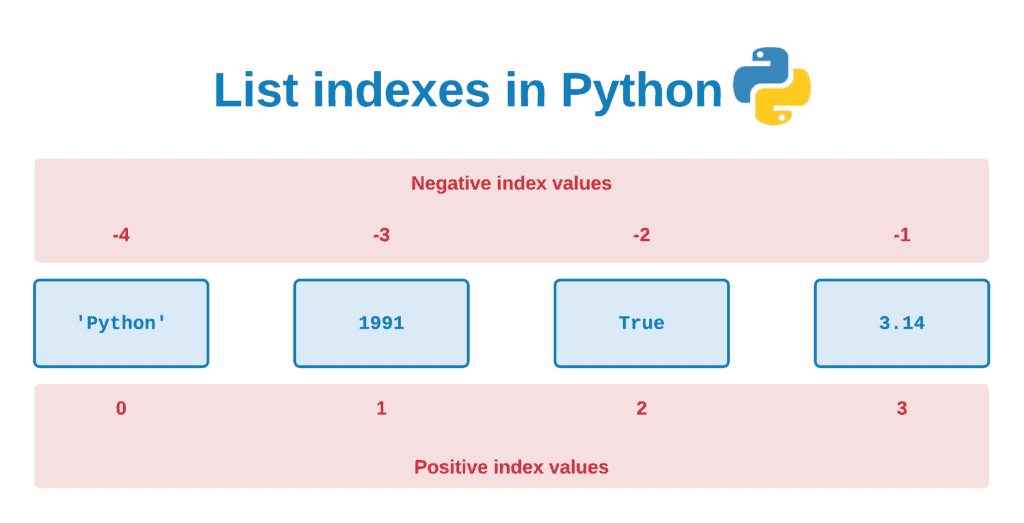
Accessing list elements using a positive index in Python
Here’s an example of using the positive index to get access to Python list elements:
hands_on_list = ['Python', 1991, True, 3.14]
# Python indexing starts at 0
print(f"Accessing first item --> {hands_on_list[0]}")
print(f"Accessing second item --> {hands_on_list[1]}")
print(f"Accessing fourth item --> {hands_on_list[3]}")
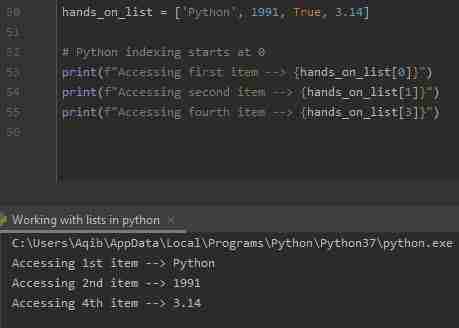
How to access list elements using a negative index in Python?
You can access Python list items using positive and negative index values. An index value of -1 points to the last item of a list, -2 to the second item from the end, and so on:
hands_on_list = ['Python', 1991, True, 3.14]
print(f"Accessing last item --> {hands_on_list[-1]}")
print(f"Accessing second last item --> {hands_on_list[-2]}")
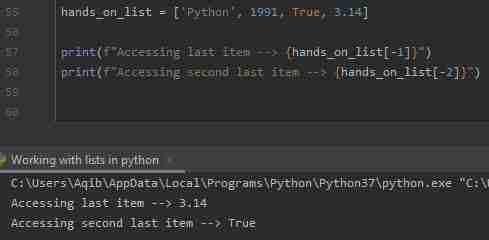
How to access a range of list elements (slicing) in Python?
A colon :
is used as the slicing operator for lists in Python. We can use a range of indices to access several items within that range. The code and output below explain the slicing concept.
Slicing operation examples:
[1:4]
– returns items within the index range from 1 to 4[1:]
– returns items from the list whose index is greater than 1[:3]
– returns items from the list whose index is less than 3[:]
– returns all elements from the list (a reference to the same object)
Let’s take a look at the code example:
hands_on_list = ['Python', 1991, True, 3.14, 'hand-on']
print(f"Accessing items from 2nd to 4th --> {hands_on_list[1:4]}")
print(f"Accessing items from 4th to 5th --> {hands_on_list[3:5]}")
print(f"Accessing items from 2nd to last --> {hands_on_list[1:]}")
print(f"Accessing items from start to 3rd --> {hands_on_list[:3]}")
print(f"Accessing all items --> {hands_on_list[:]}")
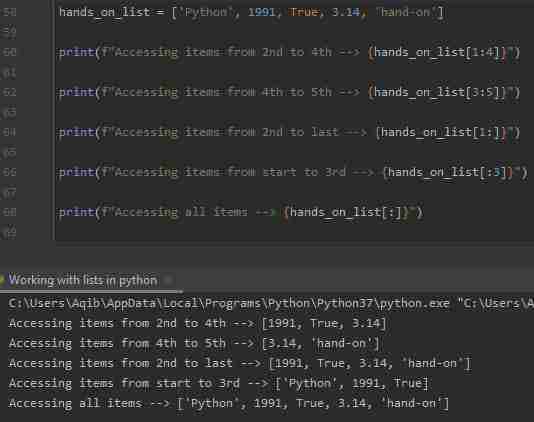
How to get the length of the list in Python?
You can use a built-in len()
method to get the length of a list in Python:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
print(len(hands_on_list))
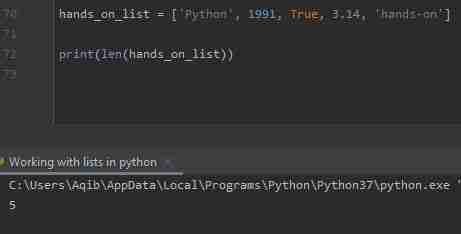
How to add items to the list in Python?
You can use an append()
method add (append) a single element to the end of the list:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
print(f"Original list --> {hands_on_list}")
hands_on_list.append('Lists Tutorial')
print(f"List after append/add --> {hands_on_list} \n")
To add (append) several items to an existing list, use an extend()
method:
hands_on_list_1 = ['Python3', 123, False, 0.234, 'hands-on']
print(f"Original list --> {hands_on_list_1}")
hands_on_list_1.extend(['Lists Tutorial', 'Dictionaries Tutorial'])
print(f"List after extend --> {hands_on_list_1} \n")
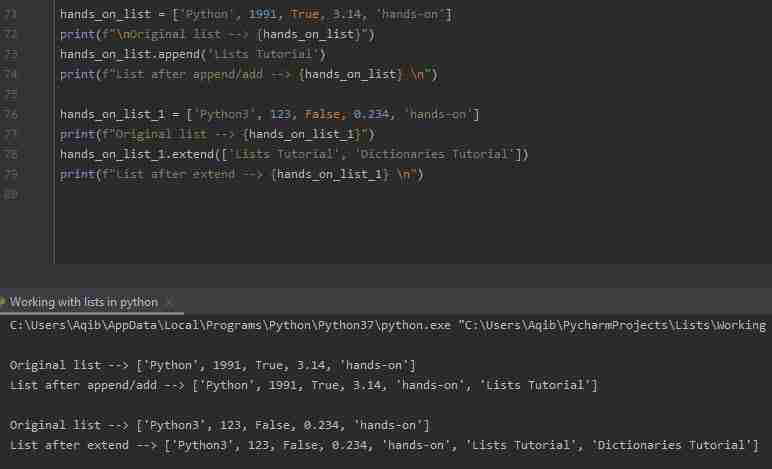
How to change list Items in Python?
In this section of the article, we’ll describe how to change a single item value and a range of item values in the list in Python.
How to change a list item value in Python?
We can change a specific item’s value in the list using the item index number:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
print(f"\nOriginal list --> {hands_on_list}")
hands_on_list[4] = 'https://www.hands-on.cloud'
print(f"List after alteration --> {hands_on_list}")
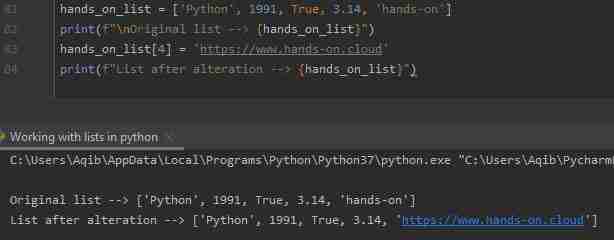
How to change a range of list item values in Python?
To change the range of items values, you need to define a list of item values for the specified range (slice operation):
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
print(f"\nOriginal List -->> {hands_on_list}")
hands_on_list[1:3] = [1992, False]
print(f"List after changing range of items values --> {hands_on_list}")

If you provide more items in a list than the number of items in the slice operation, then additional items will be added to the list. As a result, the length of the new list will be increased:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
print(f"\nOriginal List -->> {hands_on_list}")
hands_on_list[1:3] = [1992, False, 'New Item', 'Another Item']
print(f"List after changing range of items values --> {hands_on_list}")
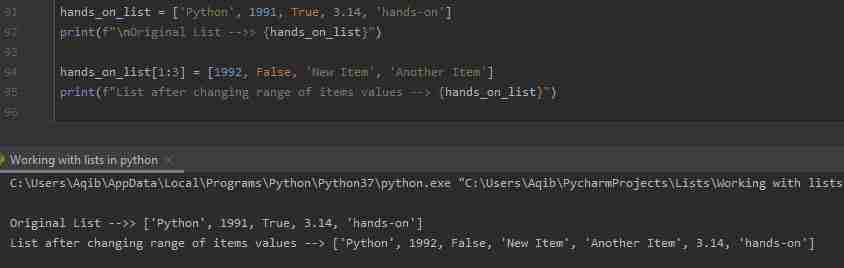
If we pass fewer items than specified in the slicing operation, then the remaining items, then the length of the new list will be decreased:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
print(f"\nOriginal List -->> {hands_on_list}")
hands_on_list[1:4] = [1992]
print(f"List after changing range of items values --> {hands_on_list}")
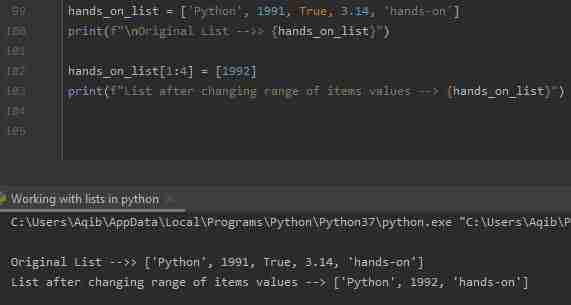
How to insert an item in a list in Python?
We can insert a new item to the list without replacing any of the existing values by using the insert()
method.
The insert()
method inserts an item at the specified index:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
hands_on_list.insert(1, 112)
print(hands_on_list)
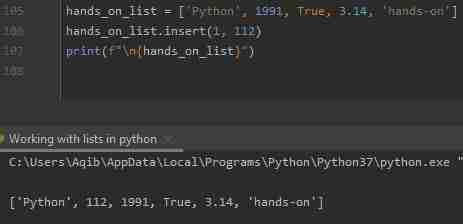
How to remove list Items in Python?
In this part of the article, we’ll cover several methods of removing elements from the Python list.
How to remove a specific item from the list in Python?
Any list in Python has a remove()
method that allows you to remove the specified item from the list.
Notes:
- The
remove()
method removed the item from the list by its value, not an index. - This method removes only a single occurrence of the item in the list. Only the first occurrence will be removed if the list contains several items of the same value.
- If the list does not have an item provided to the
remove()
method, Python will raiseValueError: list.remove(x): x not in list
exception.
Here’s an example:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
hands_on_list.remove(True)
print(f"\n{hands_on_list}")
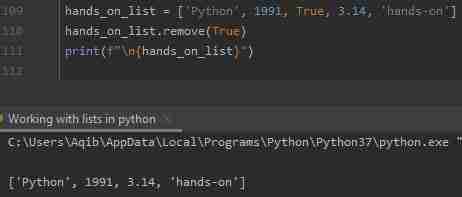
How to remove an item by the index from the list in Python?
Any list in Python has a pop()
method that allows you to remove the item from the list by the specified index.
Note: If we do not specify an index, then the pop()
method will remove the last item in the list.
Here’s an example:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
hands_on_list.pop(1)
print(f"\nRemoving Item at index 1 --> {hands_on_list}")
hands_on_list.pop()
print(f"\nRemoving last item of list --> {hands_on_list}")
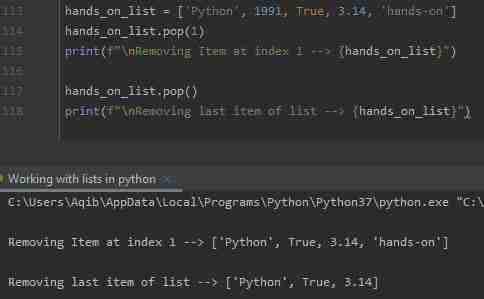
How to remove an item from the list using the ‘del’ keyword?
The del
keyword in Python allows you to remove an item from the list at a specified index.
You can also use the del
keyword to remove/delete the whole list too:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
del hands_on_list[0]
print(f"\n List after removing the item at index 0 --> {hands_on_list}")
del hands_on_list
print("Error would occur while printing the list because list no more exists...")
#print(hands_on_list)
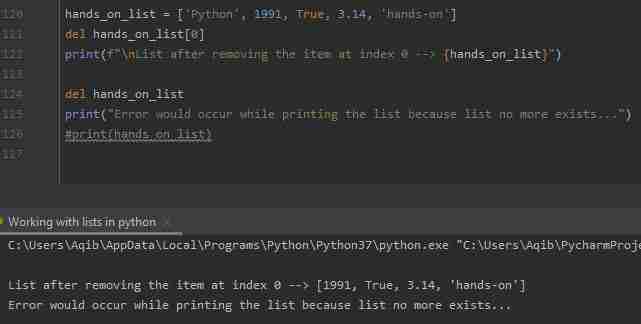
How to delete all elements from the list in Python?
You can use a clear()
method to delete all elements from the list:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
print(f"\nList before clearing --> {hands_on_list}")
hands_on_list.clear()
print(f"\nList after clearing --> {hands_on_list}")
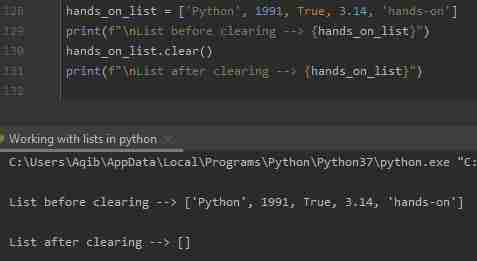
How to iterate over a list in Python?
You can iterate over a list in multiple ways. Let’s review those methods one by one.
Iterating over a list using for-loop
You can loop through a list by using a for-loop. All the items in the list will be accessible one by one in order.
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
for item in hands_on_list:
print(f"\n{item}")
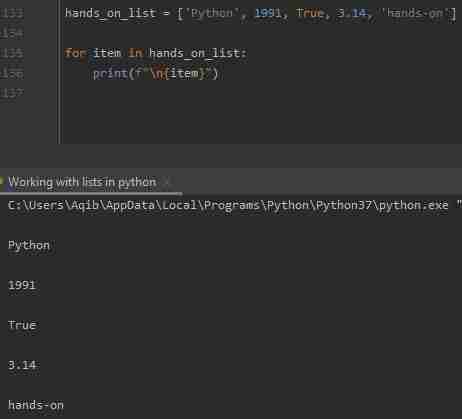
Iterating over a list using for-loop and range() function
If you need to have information about the item index during for-loop execution, you can use a range()
or len()
functions.
The range(start, stop, step)
function returns a sequence of numbers, starting from 0 by default, increments by 1 (by default), and stops before a specified number.
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
length_of_myList = len(hands_on_list)
for number in range(length_of_myList):
print(f"\n{ hands_on_list[number] }")
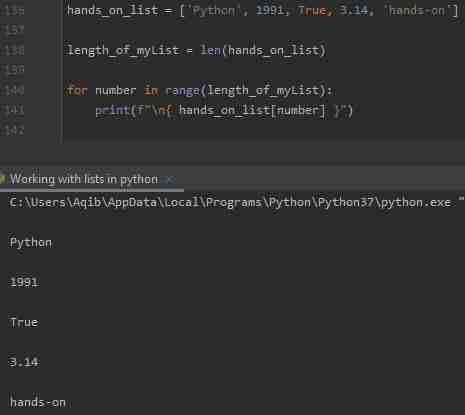
For example, if you need to get access to every second element from the list, you can slightly change the range()
arguments:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
length_of_myList = len(hands_on_list)
for number in range(1, length_of_myList, 2):
print(f"\n{ hands_on_list[number] }")
Iterating over a list using while-loop
You can loop through the list items by using a while-loop too:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
number = 0
length_of_myList = len(hands_on_list)
while number < length_of_myList:
print(f"\n{ hands_on_list[number] }")
number += 1
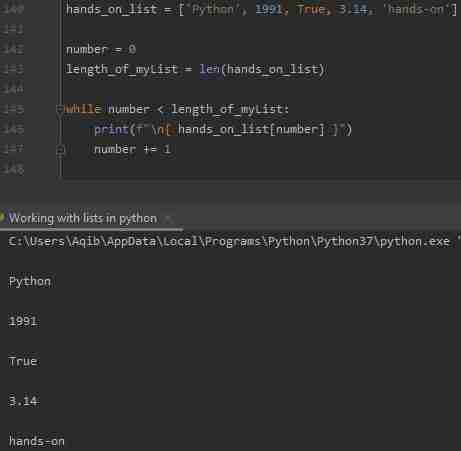
Iterating using list comprehension
A list comprehension consists of an expression followed by for statement inside square brackets and provides us with the shortest syntax for looping through a list.
The following one-line for-loop will print all items from the list one by one:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
[print(item) for item in hands_on_list]
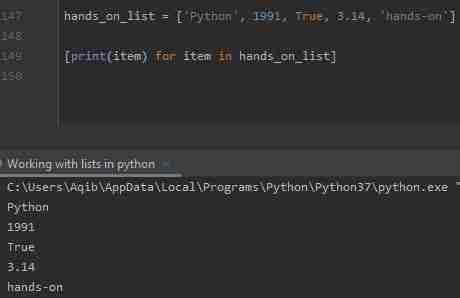
Using list comprehensions in Python
List comprehension allows you to create a new list from an existing list in the shortest possible way. List comprehension allows you to replace several lines of code with a single line.
Syntax: [expression for item in iterable]
You can add an if-condition if required, for example: [expression for an item in iterable if condition]
Let’s take a look at the example:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
new_hands_on_list = [item for item in hands_on_list]
print(f"\n New list contains all items of original list --> {new_hands_on_list}")
# With if condition
new_hands_on_list_1 = [item for item in hands_on_list if item == 1991]
print(f"\n New list contains that item which has 1991 value --> {new_hands_on_list_1}")
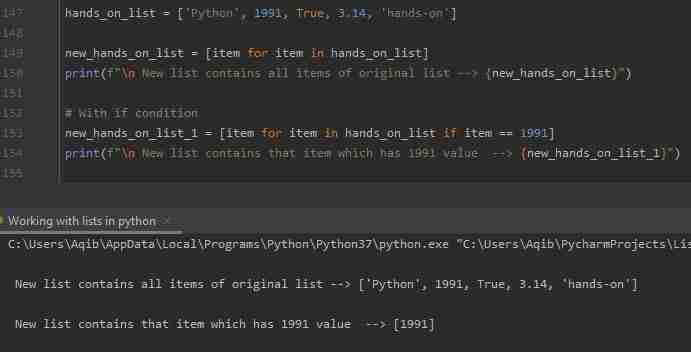
How to sort lists in Python?
A list class has a sort()
method that allows you to sort items in a list. By default, Python will sort the list in ascending order. If you need to sort the list in descending order, you have to provide reverse=True
argument to the sort()
method:
# Sort list alphabetically in ascending order
list_sorting = ['a', 'd', 'b', 'c']
list_sorting.sort()
print(list_sorting)
# Sort list alphabetically in descending order
list_sorting.sort(reverse=True)
print(list_sorting)
# Sort list numerically in ascending order
list_sorting = [3, 1, 4, 2]
list_sorting.sort()
print(f"\n{ list_sorting }")
# Sort list numerically in descending order
list_sorting.sort(reverse=True)
print(list_sorting)
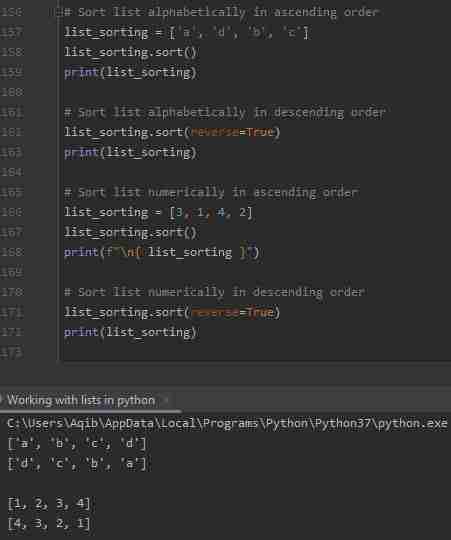
How to copy a list in Python?
As soon as variables of type list in Python store the reference to the list values in memory, you can’t make a copy of a list by using the equals (=
) operator.
After executing the statement list2=list1
, the variable list2
will store a reference to the variable list1
, and all changes made in list1
will be reflected in the list2
and vice versa.
So, you have to use built-in methods to copy a list in Python.
Using the copy() method
You can make a copy of a list by using the copy()
method:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
copied_list = hands_on_list.copy()
print(f"\n{copied_list}")
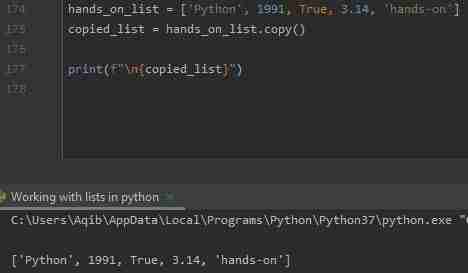
Using the list() method
Another way to make a copy of a list is to use the list()
method:
hands_on_list = ['Python', 1991, True, 3.14, 'hands-on']
copied_list = list(hands_on_list)
print(f"\n{copied_list}")
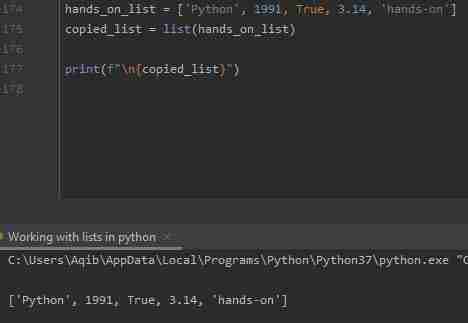
How to join (concatenate) lists in Python?
You can use several methods to join (concatenate) two or more lists. Let’s review those methods one by one.
Using the + operator
You can use the + operator to join (concatenate) two lists:
list_one = ['a', 'b', 'c']
list_two = ['d', 'e', 'f']
list_three = list_one + list_two
print(list_three)
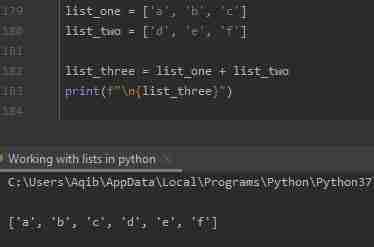
Using the append() method
The append()
is another method that allows you to join two lists by appending all the items from one list into another one by one:
list_one = ['a', 'b', 'c']
list_two = ['d', 'e', 'f']
for item in list_two:
list_one.append(item)
print(f"\n{list_one}")
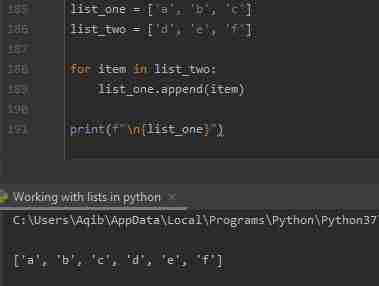
Using the extend() method
The extend()
is another method that allows you to join two lists by adding one list’s items to another:
list_one = ['a', 'b', 'c']
list_two = ['d', 'e', 'f']
list_one.extend(list_two)
print(f"\n{list_one}")
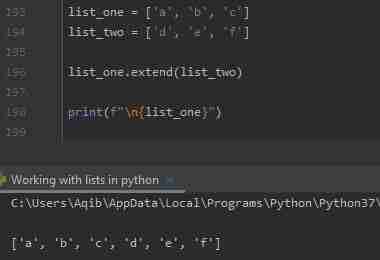
How to unpack lists in Python?
Suppose you have a list that you want to unpack. That’s easy. All you need to remember is that the number of variables from the left side must equal the number of items in a list from the right side. Otherwise, the Python interpreter will raise an ValueError: too many values to unpack
exception.
my_list = ['John', 'Samuel', 'Arnold', 'Watson']
name1, name2, name3, name4 = my_list
print(f"\nName1 --> {name1}, Name2 --> {name2}, Name3 --> {name3}, Name4 --> {name4}")
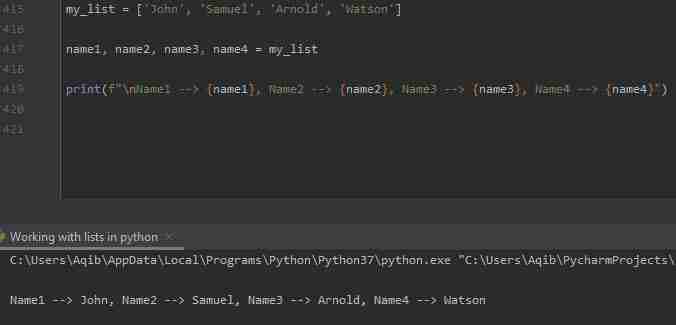
How to reverse a list in Python?
Python has a built-in reverse()
method, which reverses the elements of a list:
my_list = ['John', 'Samuel', 'Arnold', 'Watson']
print(f"\nOriginal List --> {my_list}")
my_list.reverse()
print(f"\nReversed List --> {my_list}")
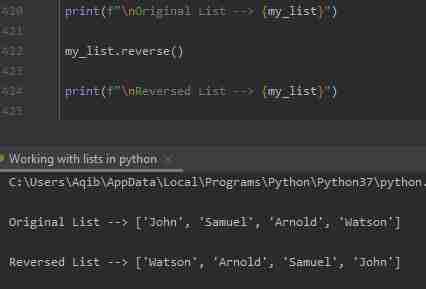
How to search items in the list in Python?
You can use in
operator to check if the list contains a particular item:
my_list = ['John', 'Samuel', 'Arnold', 'Watson']
if 'John' in my_list:
print("\nJohn exists")
else:
print("\nJohn does not exist")
if 'Johnny' in my_list:
print("\nJohnny exists")
else:
print("\nJohnny does not exist")
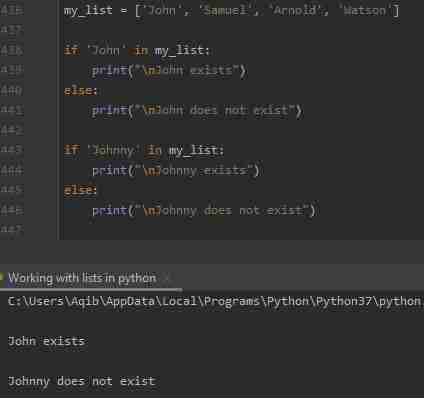
Multiplication of two lists in Python
You can do multiplication between two lists of an equal length. The item at the index of one list multiplies on the item of the same index of the other list.
Multiplication using zip()
my_list1 = [2, 5, 8]
my_list2 = [6, 2, 8]
multiplied_list = []
for num1, num2 in zip(my_list1, my_list2):
multiplied_list.append(num1 * num2)
print(f"\nMultiplied List --> {multiplied_list}")
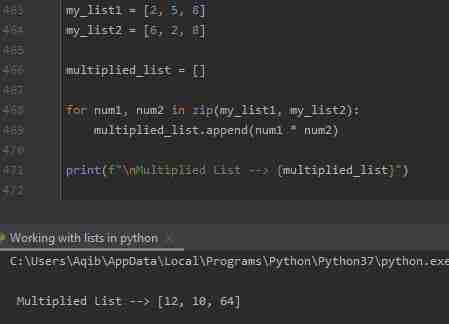
Multiplication using zip() and the list comprehension
The example above can be implemented in one line using list comprehension and zip() together:
my_list1 = [2, 5, 8]
my_list2 = [6, 2, 8]
multiplied_list = []
multiplied_list = [num1 * num2 for num1, num2 in zip(my_list1, my_list2)]
print(f"\nMultiplied List --> {multiplied_list}")
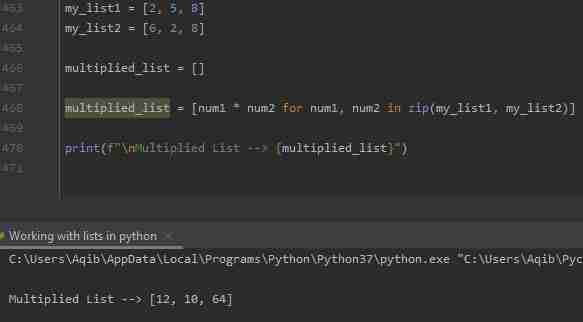
How to use enumerate() with lists in Python?
Python has an in-built enumerate()
method adds a counter to an iterable and returns the enumerated object. You can convert the returned enumerated object to a list or tuple.
Syntax: enumerate(iterable, start=0)
The enumerate()
method starts counting from start
value. If you omit the start
argument then enumerate()
method starts from 0:
# Initialization of a list
my_list = ['John', 'Samuel', 'Arnold', 'Watson']
#Enumerating the list
enumerateNames = enumerate(my_list)
print(f"\nType --> {type(enumerateNames)}")
# converting to list
print(f"\nEnumerated list with default start value --> {list(enumerateNames)}")
# changing the default counter
enumerateNames = enumerate(my_list, 10)
print(f"\nEnumerated list with start value 10 --> {list(enumerateNames)}")
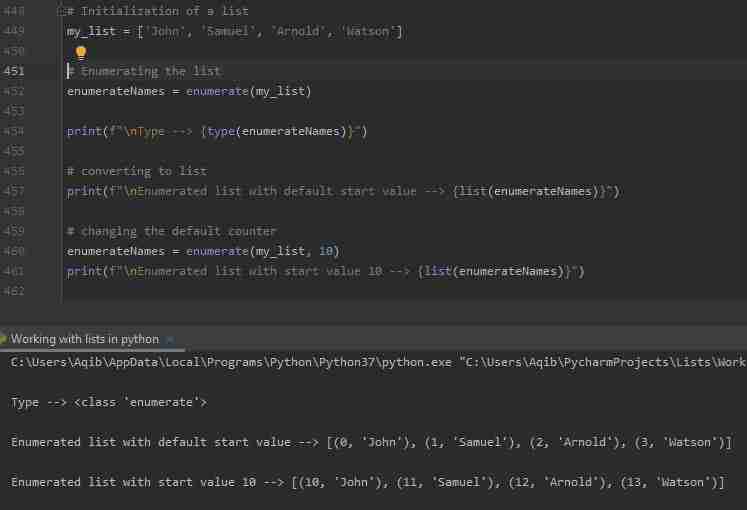
Computing XOR among list elements in Python
You can use several ways to perform bitwise operations among list elements. There are two methods for computing XOR among list elements.
Using reduce() + lambda + ‘ ^ ‘ operator
Here’s an implementation example ofXOR among list elements:
import functools
my_list = [1, 2, 1, 3, 4, 14]
# Original list
print(f"\nThe original list is --> {my_list} ")
# Using reduce() + lambda + "^" operator
xor = functools.reduce(lambda x, y: x ^ y, my_list)
# printing result
print(f"\nThe Bitwise XOR --> {xor} ")
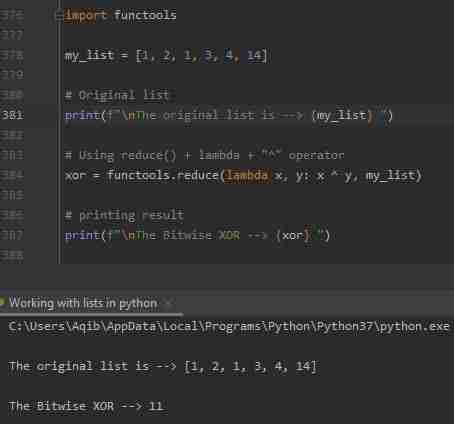
Using reduce and operator ixor
Here’s another implementation example ofXOR among list elements:
import functools
from operator import ixor
my_list = [1, 2, 1, 3, 4, 14]
# Original list
print(f"\nThe original list is --> {my_list} ")
# Using reduce() + lambda + "^" operator
xor = functools.reduce(ixor, my_list)
# printing result
print(f"\nThe Bitwise XOR --> {xor} ")
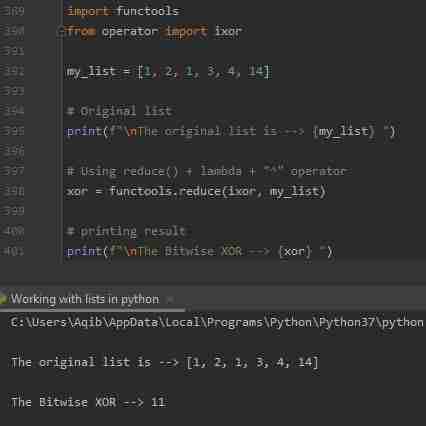
Union and intersection of two lists in Python
Let’s look at how you can get the union and intersection of two lists.
The union of two lists returns one list appended with another and ignores duplicate values.
The intersection of two lists returns all those elements which are common to both of the lists.
Here’s how you can do that:
list1 = [1, 4, 5, 7, 8, 12]
list2 = [2, 3, 5, 8]
list_union = list({i: i for i in list1 + list2}.values())
list_intersection = [i for i in list1 if i in list2]
print(f"\nUnion of two lists --> {list_union}")
sorted_union = sorted(list_union)
print(f"\nSorted Union of two lists --> {sorted_union}")
print(f"\nIntersection of two lists --> {list_intersection}")
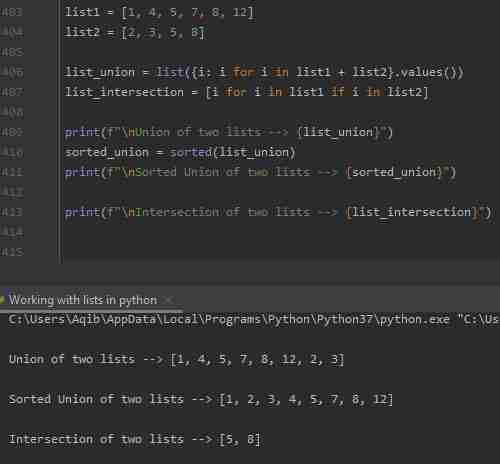
How to convert a list to a string in Python?
Several methods allow you to convert a list into a string. Let’s review them one by one.
Using iteration
Initialize an empty string, iterate through the list, and add the element for every index to the empty string:
list_one = ['a', 'b', 'c', 'd', 'e']
string_converted = ''
for item in list_one:
string_converted += item
print(f"\n{string_converted}")
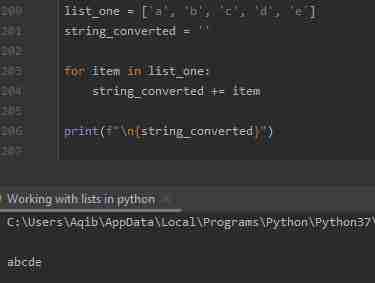
Using join() method
The join() method is a more straightforward way to convert a list into a string:
list_one = ['a', 'b', 'c', 'd', 'e']
string_converted = ' '
string_converted = string_converted.join(list_one)
print(string_converted)
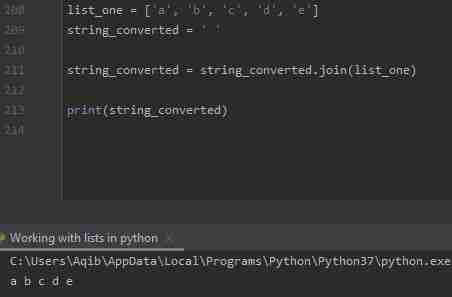
Using list comprehension
Another way to get a string from a list is to use a list comprehension:
list_one = ['a', 'b', 'c', 'd', 'e']
string_converted = ' '.join([str(elem) for elem in list_one])
print(f"\n{ string_converted }")
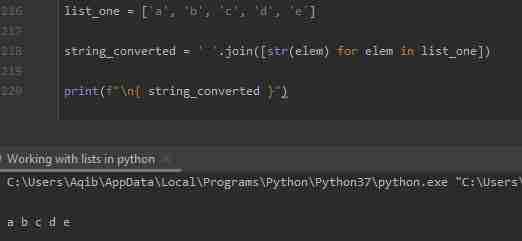
Using map() method
You can use a map()
method to convert the list into a string:
list_one = ['a', 'b', 'c', 'd', 'e']
string_converted = ' '.join(map(str, list_one))
print(f"\n{ string_converted }")
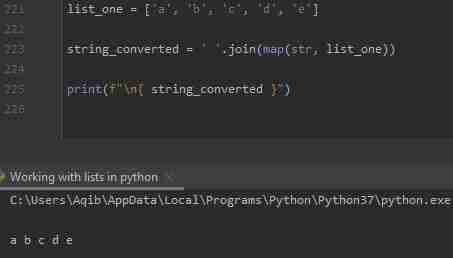
How to convert a list to a tuple in Python?
Several methods allow you to convert a list to a tuple. Let’s review them one by one.
Using tuple() method
Using a tuple()
method is the most straightforward way to convert a list into a tuple:
list_one = ['a', 'b', 'c', 'd', 'e']
tuple_converted = tuple(list_one)
print(f"\n{tuple_converted}")
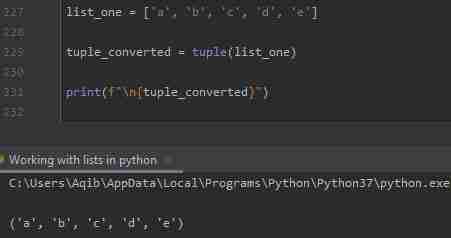
Using the list unpacking (*list, ) method
The list unpacking method is another way to convert the given list into a tuple.
list_one = ['a', 'b', 'c', 'd', 'e']
tuple_converted = (*list_one, )
print(f"\n{tuple_converted}")
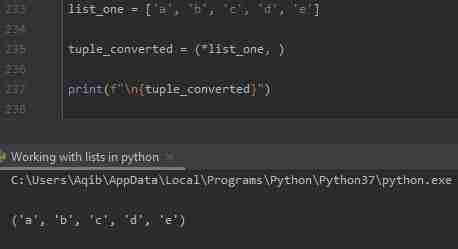
How to convert a list to a set in Python?
You can use a set(iterable)
method with iterable
as a list to convert it to a set and remove duplicates:
list_one = ['a', 'b', 'a', 'b', 'c']
set_converted = set(list_one)
print(f"\n{set_converted}")
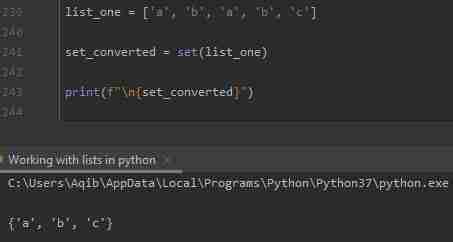
How to convert a list to a dictionary in Python?
Python allows you to convert a list to a dictionary. Let’s take a look at how you can do it.
Using zip() method
You can use a zip()
method to convert a list to a dictionary:
list_handsOn = ['name', 'hands-on', 'language', 'Python', 'Tutorial', 'Lists']
iterate_variable = iter(list_handsOn)
converted_dictionary = dict(zip(iterate_variable, iterate_variable))
print(f"\n{converted_dictionary}")
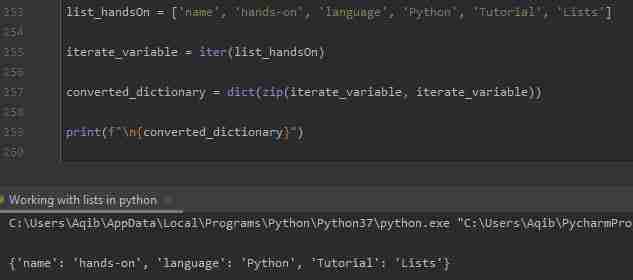
Using list comprehension
To convert a list to a dictionary, you can use list comprehension, but this method is hard to read and not recommended:
list_handsOn = ['name', 'hands-on', 'language', 'Python', 'Tutorial', 'Lists']
converted_dictionary = res_dct = {list_handsOn[i]: list_handsOn[i + 1] for i in range(0, len(list_handsOn), 2)}
print(f"\n{converted_dictionary}")
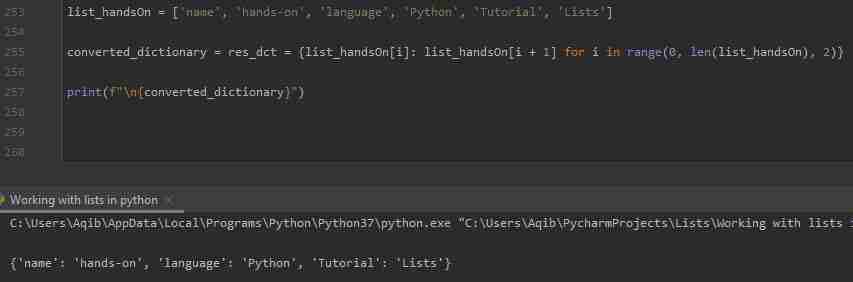
How to convert a list to a CSV file?
Python has a built-in csv
module. This module allows you to save Python lists into a CSV file.
The example code and output are given below:
# field names
csvFile_fields = ['Name', 'Grades']
# data rows of csv file
our_list = [
['John', 'B'],
['Samuel', 'A+'],
['Arnold', 'C'],
['Watson', 'A'],
['Sofia', 'B'],
['Catherine', 'C']
]
with open('list_to_CSV.csv', 'w') as file:
# using csv.writer method from CSV package
write = csv.writer(file)
write.writerow(csvFile_fields)
write.writerows(our_list)
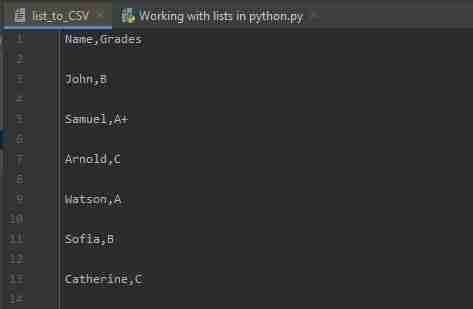
How to convert a list to a NumPy array?
Lists can be converted to NumPy arrays using the built-in functions in the Python NumPy library. NumPy package can be installed using the following command:
pip install numpy
The numpy
module provides you with two functions:
numpy.array()
numpy.asarray()
You can use both of them to convert a list into an array. The main difference between the two is that np.array()
will make a copy of the object (by default) and convert that to an array, while np.asarray()
will not.
Using NumPy array
The code below illustrates the usage of numpy.array()
:
import numpy
my_list = [1, 5, 6, 8, 12, 24, 78]
converted_array = numpy.array(my_list)
print(f"\nConverted Array --> {converted_array}")
print(f"\nConverted Array Type --> {type(converted_array)}")
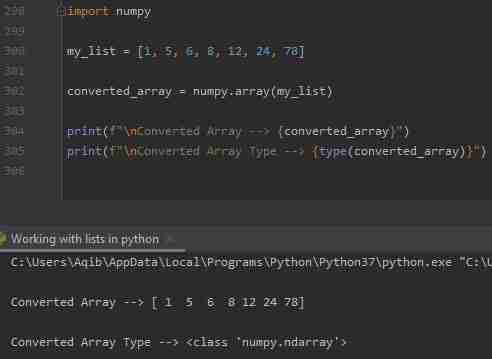
Using NumPy asarray
The code below illustrates the usage of numpy.asarray()
:
import numpy
my_list = [1, 5, 6, 8, 12, 24, 78]
converted_array = numpy.asarray(my_list)
print(f"\nConverted Array --> {converted_array}")
print(f"\nConverted Array Type --> {type(converted_array)}")
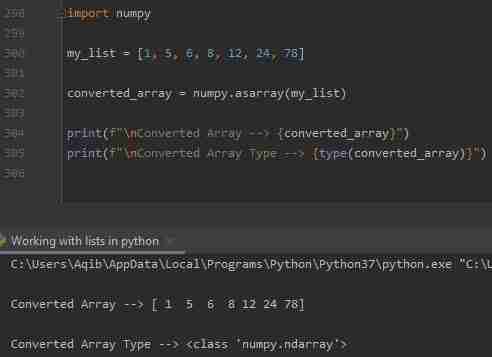
How to convert a list to a JSON in Python?
You must use a built-in JSON module to convert a list to a JSON data structure. This module has a json.dumps()
method to convert a list to a JSON string. The json.dumps()
function can take a list as an argument and return a JSON string.
List to JSON
Let’s convert a list containing different data types and convert it to a JSON string:
import json
my_list = [1, 5, 6, 'String 1', 'String 2', True, 78.45]
json_string = json.dumps(my_list)
print(f"\n{json_string}")
print(type(json_string))
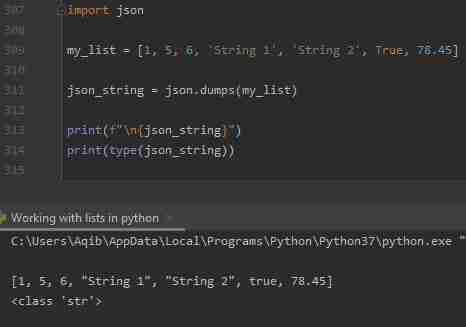
List of dictionaries to JSON
In the code below, we will convert a list of dictionaries to a JSON string:
import json
my_list = [{'a': 1, 'b': 2}, {'c': 3, 'd': 4}]
json_string = json.dumps(my_list)
print(f"\n{json_string}")
print(type(json_string))
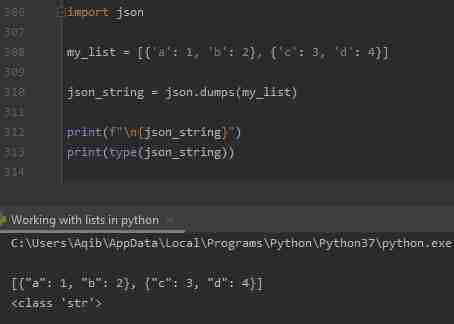
List of lists to JSON
Let’s take a look at how to convert a list of lists to a JSON string:
import json
my_list = [[{'a': 1, 'b': 2}], [{'c': 3, 'd': 4}]]
json_string = json.dumps(my_list)
print(f"\n{json_string}")
print(type(json_string))
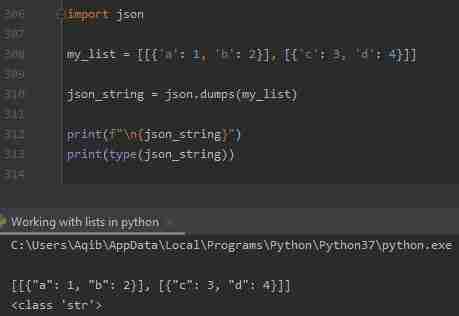
How to convert a list to XML in Python?
You can to use ElementTree
and xml.dom
to convert an existing list to an XML document. You can read, write, and manipulate XML files using these modules.
Using ElementTree
Here’s an example of using ElementTree for constructing an XML document:
import xml.etree.ElementTree as ET
my_list = ['John', 'Samuel', 'Arnold', 'Watson', 'Sofia', 'Catherine']
studentsNames = ET.Element("StudentsNames")
# create sub element
st = ET.SubElement(studentsNames, "StudentsNames")
# insert list element into sub elements
for user in range(len(my_list)):
usr = ET.SubElement(st, "student")
usr.text = str(my_list[user])
tree = ET.ElementTree(st)
# write the tree into an XML file
tree.write("List_To_XML.xml", encoding='utf-8', xml_declaration=True)
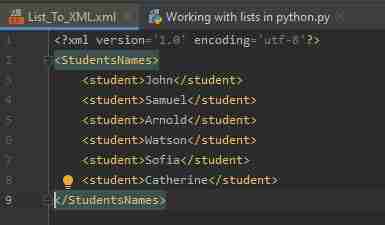
Using XML dom module
In this example, we’ll use the xml.dom
module to achieve a similar goal:
from xml.dom import minidom
my_list = ['John', 'Samuel', 'Arnold', 'Watson', 'Sofia', 'Catherine']
root = minidom.Document()
# creat root element
xml = root.createElement('root')
root.appendChild(xml)
for user in range(len(my_list)):
# create child element
productChild = root.createElement('Student' + str(user))
# insert user data into element
productChild.setAttribute('Name', my_list[user])
xml.appendChild(productChild)
xml_str = root.toprettyxml(indent="\t")
# save file
path_file = "List_To_XML_MiniDom.xml"
with open(path_file, "w") as f:
f.write(xml_str)
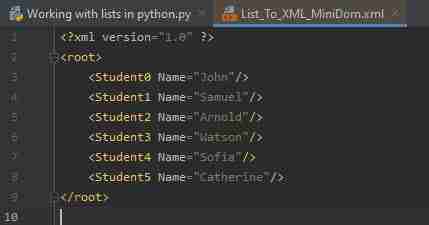
How to convert a list to Pandas DataFrame?
Converting a list to Pandas DataFrame can be done in multiple ways.
Using pandas.DataFrame() constructor
Here, you need to have a multi-dimensional list to create a DataFrame.
Note: multi-dimensional lists are also called nested lists.
# import pandas as pd
import pandas as pd
# list of strings
our_list = [['John', 'B'],
['Samuel', 'A+'],
['Arnold', 'C'],
['Watson', 'A'],
['Sofia', 'B'],
['Catherine', 'C']]
# Calling DataFrame constructor on list
converted_dataframe = pd.DataFrame(our_list, columns=['Name', 'Grade'])
print(converted_dataframe)
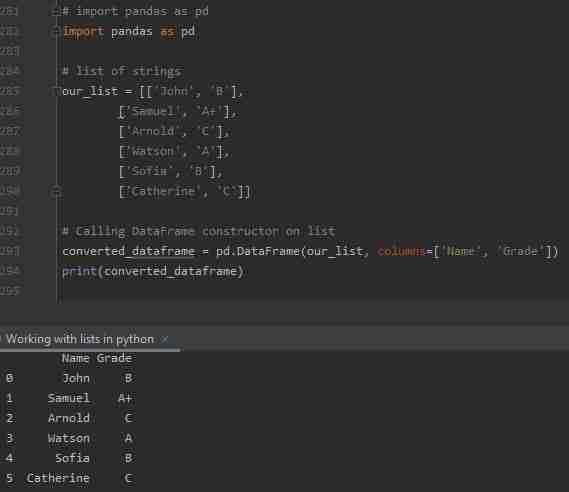
Using zip() andpandas.DataFrame() constructor
You can use two lists and the zip() Python built-in method to generate a Pandas DataFrame:
import pandas as pd
names = ['John', 'Samuel', 'Arnold', 'Watson', 'Sofia', 'Catherine']
grades = ['C', 'B', 'A', 'C', 'A+', 'A']
# Calling DataFrame constructor on list
converted_dataframe = pd.DataFrame(list(zip(names, grades)), columns=['Name', 'Grade'])
print(converted_dataframe)
Are lists ordered in Python?
In Python, all list items have a defined order. Two lists containing the same elements by a different index are not equal:
# Initialization of two lists with same items but with changed order
list1 = [1, 2, 3, 4]
list2 = [2, 1, 3, 4]
# Check if both lists are same
print(list1 == list2)
print(list1 is list2)
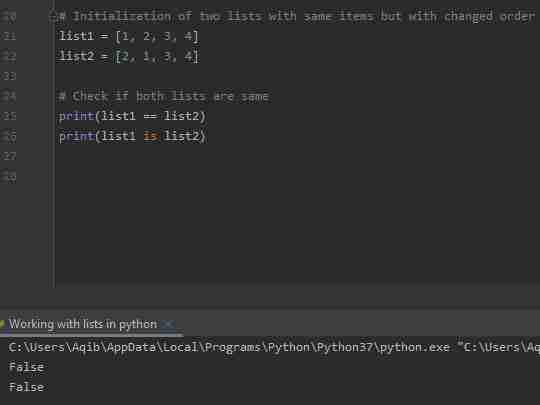
Are lists mutable (changeable) in Python?
In Python, a list is a mutable data structure. That means you can change, add, and remove items in the list after the list has been created.
hands_on_list = [1, 2, 3, 4]
hands_on_list.append(5)
print(f"Adding new item --> {hands_on_list}")
hands_on_list[0] = 'One'
print(f"Updating the item --> {hands_on_list}")
hands_on_list.remove(5)
print(f"Removing an item --> {hands_on_list}")
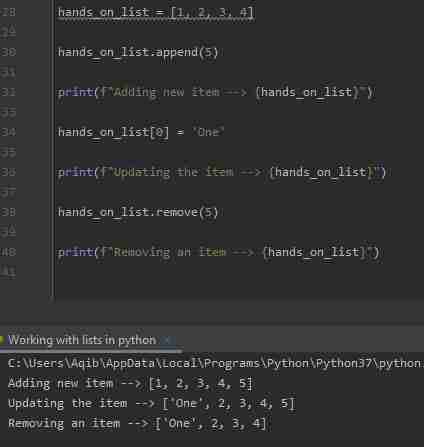
Are lists allow duplicates in Python?
As we discussed earlier in the article, lists are indexed so that lists can have items with the same values:
hands_on_list = [1, 1, 5, 1, 1, 2, 3, 4, 2]
print(hands_on_list)
print(hands_on_list[0])
print(hands_on_list[1])
print(hands_on_list[3])
print(hands_on_list[4])
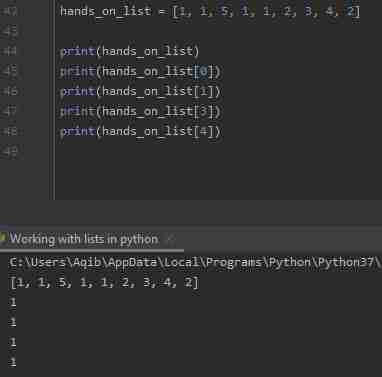
How to fill a list with zeros in Python?
You can initialize a fixed-size list with all zeros or any other value. Here’s an example:
n = 50
list_zeros = [0] * n
list_eights = [8] * n
print(f"\n{list_zeros}")
print(f"\n{list_eights}")
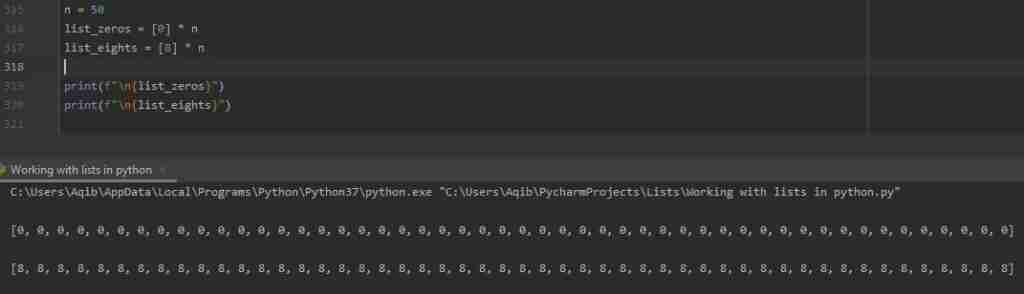
Built-in list functions and methods
Python has the following built-in functions and methods related to lists.
Functions
Function | Description |
---|---|
cmp(list_one, list_two) | Compares elements of two lists. |
list(seq) | Converts a given tuple into a list. |
len(list) | Returns the total length (number of items) of the list. |
min(list) | Returns an item from the list with minimum value. |
max(list) | Returns an item from the list with maximum value. |
Methods
Method | Description |
---|---|
list.append(item) | Appends an item to the list |
list.count(item) | Returns count of how many times an item occurs in the list |
list.extend(any_list) | Add elements of the any_list to the list |
list.index(item) | Returns the lowest index in the list that item appears |
list.insert(index, item) | Inserts object item into the list at offset index |
list.pop(item=list[-1]) | Removes and returns the last item or specified item from the list |
list.remove(item) | Allows to delete list elements |
list.reverse() | Reverses objects of the list in-place |
list.sort() | Sorts objects of the list |
Summary
This article covered Python lists, list item manipulation, iterating, comparing, sorting, and transformation operations.